Table of Contents
- Introduction
- [The Basics of Headless WordPress](#the-basics-of-headless-wordpress)
- Overview of Next JS
- Setting Up Headless WordPress
- Implementing Next JS
- Building a Site with Headless WordPress and Next JS
- Troubleshooting and Common Issues
- Case Studies and Examples
- Conclusion
- Regular Updates
Introduction
In today’s rapidly evolving web development landscape, Headless WordPress and Next JS have emerged as powerful tools for building modern, dynamic websites. But what exactly are these technologies, and why are they becoming so popular?
Headless WordPress refers to using WordPress solely as a Content Management System (CMS) without relying on its traditional front-end rendering capabilities. Essentially, it means decoupling the backend (where you manage content) from the frontend (what the users see). This approach provides developers with greater flexibility, allowing them to use WordPress to manage content while building the front-end using any technology they prefer.
On the other hand, Next JS is a popular JavaScript framework built on React that excels in creating server-side rendered (SSR) and statically generated applications. It simplifies the development process by handling routing, code-splitting, and many other aspects of web development, making it a perfect companion to a headless CMS like WordPress.
This comprehensive guide will take you through the process of effectively using Headless WordPress and Next JS together, from setting up your environment to building a complete website. Whether you’re a developer looking to modernize your workflow or just someone curious about these technologies, this guide will equip you with the knowledge to harness their full potential.
The Basics of Headless WordPress
What Does 'Headless' Mean?
When we talk about a "headless" CMS, we’re essentially referring to a system where the content management backend (the “body”) is separated from the presentation layer or frontend (the “head”). In traditional setups, WordPress would manage both the backend and the frontend, meaning it would control everything from content creation to how that content is displayed on your site.
In a headless configuration, WordPress is only responsible for managing and storing content. The data is then accessed via an API (usually REST API or GraphQL) and can be displayed using any frontend technology. This approach gives developers the freedom to use modern frameworks like React, Angular, or in this case, Next JS, to design and build the frontend.
Benefits of Headless WordPress
-
Flexibility: Since the frontend is decoupled from WordPress, developers have the freedom to choose any technology to create the user interface, leading to highly customized user experiences.
-
Performance: A headless setup can improve site performance because the frontend can be optimized independently from WordPress, utilizing faster and more efficient technologies.
-
Scalability: Headless architectures often scale better, as the backend and frontend can be scaled independently, allowing for more efficient resource management.
-
Future-Proofing: As frontend technologies evolve, you can update or completely change the frontend without altering your content management system.
How It Differs from Traditional WordPress
In a traditional WordPress setup, the entire website, including content management and rendering, is handled by WordPress. This monolithic structure means any changes to the site’s functionality or design often require WordPress plugins, themes, and PHP code adjustments.
In contrast, a headless setup transforms WordPress into a purely backend solution, where it acts as a content repository. The frontend is entirely separate, typically built using modern JavaScript frameworks like Next JS, offering far more flexibility in design and performance optimization.
Overview of Next JS
What is Next JS?
Next JS is a powerful framework built on top of React that enables developers to create server-side rendered (SSR) and static sites with ease. It streamlines the process of building React applications by providing built-in features like routing, server-side rendering, static site generation, and API routes.
Key Features of Next JS
-
Server-Side Rendering (SSR): Unlike traditional React apps that are fully client-side, Next JS allows rendering pages on the server before sending them to the client. This results in faster initial load times and better SEO performance.
-
Static Site Generation (SSG): With SSG, Next JS can pre-render pages at build time, ensuring that the generated HTML is ready to be served quickly, improving performance, especially for content that doesn’t change often.
-
API Routes: Next JS provides a way to create API endpoints directly within the app, making it easier to handle backend logic without needing an external server.
-
File-Based Routing: Routing in Next JS is based on the file system, simplifying the process of creating new pages by simply adding files to the
pages
directory. -
Automatic Code Splitting: This feature ensures that only the necessary code is loaded for each page, reducing the amount of JavaScript that needs to be downloaded and improving page load times.
Why Use Next JS with Headless WordPress?
When combined with a headless WordPress setup, Next JS can pull content from WordPress and render it dynamically on the frontend. This combination leverages the strengths of both technologies: WordPress’s robust content management capabilities and Next JS’s modern web development features. Together, they provide a powerful, flexible, and efficient way to build high-performance websites.
Setting Up Headless WordPress
1. Preparing Your WordPress Site
Before you can use WordPress as a headless CMS, you need to ensure that it’s set up correctly.
-
Install WordPress: Start by installing WordPress on your server or locally. If you’re new to WordPress, most hosting providers offer one-click installation options.
-
Choose a Hosting Provider: Select a hosting provider that supports modern PHP versions and provides good performance. Popular options include WP Engine, Kinsta, and SiteGround.
-
Install Essential Plugins: To use WordPress as a headless CMS, you’ll need to install plugins that enable REST API or GraphQL functionality. Popular choices include WPGraphQL and Advanced Custom Fields (ACF). These plugins allow you to create custom content types and retrieve content programmatically.
2. Configuring WordPress for Headless Use
-
Enable REST API: WordPress comes with a REST API built-in, which allows you to access content programmatically. Make sure it’s enabled and accessible by checking your WordPress site’s
/wp-json/
endpoint. -
Configure Permalinks: For the REST API to function correctly, your permalinks need to be set to “Post name” or another option that isn’t default. This can be done in the WordPress dashboard under Settings > Permalinks.
-
Set Up WPGraphQL: If you prefer using GraphQL over REST API, install and activate the WPGraphQL plugin. This will provide a GraphQL endpoint that you can query to retrieve content from WordPress.
-
Manage Content: Use WordPress’s familiar interface to create and manage content. Even though the frontend is decoupled, the content management process remains the same.
3. Preparing for Integration with Next JS
-
Create Custom Fields: Use ACF or similar plugins to create custom fields for your posts and pages. This is especially useful if you need to store and retrieve complex data structures.
-
Generate API Keys: Depending on your setup, you might need to generate API keys for authentication when accessing WordPress data from the Next JS frontend. This can be done through plugins like Application Passwords.
-
Test API Endpoints: Before moving on to integrating with Next JS, test your API endpoints using tools like Postman to ensure they’re returning the expected data.
Implementing Next JS
1. Setting Up a New Next JS Project
To get started with Next JS, you’ll need to set up a new project.
-
Install Node.js: Ensure Node.js is installed on your machine. You can download it from the official Node.js website.
-
Create a Next JS App: Use the following command to create a new Next JS project:
npx create-next-app headless-wp-<a href="/blog/building-a-high-performance-ecommerce-store-with-nextjs">nextjs</a> cd headless-wp-nextjs
This command sets up a new Next JS project with all the necessary dependencies.
-
Run the Development Server: Start the development server using:
npm run dev
This command will start the server, and you can view your project by navigating to
http://localhost:3000
in your browser.
2. Integrating with Headless WordPress
Now that your Next JS project is up and running, it’s time to integrate it with your headless WordPress site.
-
Install Axios or Fetch: Next JS supports both Axios and the native Fetch API for making HTTP requests. Install Axios using:
npm install axios
Or you can use the built-in
fetch()
function available in modern JavaScript. -
Fetch Data from WordPress: Create a new page in your Next JS project (e.g.,
pages/blog.js
). Use Axios or Fetch to retrieve data from the WordPress REST API or GraphQL endpoint. Here’s a basic example using Axios:import axios from "axios"; export async function getStaticProps() { const res = await axios.get( "https://your-wordpress-site.com/wp-json/wp/v2/posts" ); return { props: { posts: res.data, }, }; } export default function Blog({ posts }) { return ( <div> <h1>Blog</h1> {posts.map((post) => ( <div key={post.id}> <h2>{post.title.rendered}</h2> <div dangerouslySetInnerHTML={{ __html: post.content.rendered }} /> </div> ))} </div> ); }
This code fetches posts from WordPress and displays them in a Next JS page.
-
Handle Dynamic Routing: Next JS makes it easy to create dynamic routes. For example, to display individual blog posts, you would create a file in
pages/blog/[slug].js
and usegetStaticPaths
andgetStaticProps
to fetch and display the content based on the post’s slug.
3. Optimizing for Performance and SEO
-
Implement Incremental Static Regeneration (ISR): ISR allows you to create or update static pages after you’ve built your site. This ensures your content is always fresh without requiring a full rebuild.
-
Optimize Images: Use Next JS’s built-in
<a href="/blog/how-to-optimize-images-in-nextjs-with-the-image-component">next/image</a>
component to optimize images for better performance. This component automatically handles image resizing, lazy loading, and more. -
Add Meta Tags: Use the
next/head
component to add SEO-friendly meta tags to your pages. This includes title tags, descriptions, and Open Graph tags for better social sharing.
Building a Site with Headless WordPress and Next JS
1. Creating Pages and Components
-
Page Creation: Start by creating the necessary pages for your site. This might include a homepage, about page, blog, and contact page. Each page should be a separate file within the
pages
directory in your Next JS project. -
Component Reusability: Break down your pages into reusable components. For example, create components for headers, Footers, navigation menus, and content sections. This modular approach makes it easier to manage and update your site.
2. Managing Routing and Navigation
-
Static Routing: Next JS uses a file-based routing system, which means the file structure in the
pages
directory determines your site’s URLs. For example,pages/about.js
would be accessible athttp://yoursite.com/about
. -
Dynamic Routing: For content-driven sites, you’ll need dynamic routing to handle pages generated from WordPress content. For example, to display individual blog posts, you’ll create a
[slug].js
file within thepages/blog
directory and usegetStaticPaths
to generate paths for each post.
3. Including Dynamic Content
-
Fetching Content: Use Next JS’s
getStaticProps
orgetServerSideProps
to fetch Dynamic Content from WordPress. You can also useSWR
(Stale-While-Revalidate) for data fetching in client-side components. -
Rendering Content: Ensure your components can render dynamic content effectively. This might involve parsing HTML from WordPress or rendering custom components for different content types.
4. Tips and Best Practices
-
Content Caching: Implement caching strategies to reduce the load on your WordPress server and improve site performance.
-
SEO Optimization: Use structured data and Next JS’s SEO features to ensure your site is well-optimized for search engines.
-
Accessibility: Make sure your site is accessible by adhering to WCAG guidelines. This includes using semantic HTML, ensuring proper color contrast, and making your site navigable by keyboard.
<a href="/blog/how-to-install-shopifycli-on-linux">Troubleshooting and Common Issues
1. API Authentication Issues
- Solution: If you’re using private data from WordPress, ensure you’ve correctly configured API authentication. This might involve using OAuth or API keys.
2. Data Fetching Problems
- Solution: Ensure your WordPress API endpoints are accessible and returning the correct data. Use tools like Postman to debug API responses.
3. Build Errors in Next JS
- Solution: Common build errors might arise from incorrect imports or unresolved dependencies. Carefully check your code and use Next JS’s error messages to identify issues.
4. CORS Issues
- Solution: If you encounter Cross-Origin Resource Sharing (CORS) issues, configure your WordPress server to allow requests from your Next JS domain.
5. Performance Bottlenecks
- Solution: If your site is slow, consider using Next JS’s profiling tools to identify performance bottlenecks. Optimize images, enable lazy loading, and minimize JavaScript where possible.
Case Studies and Examples
1. E-Commerce Site
- Example: An online store using Headless WordPress to manage product information and Next JS to deliver a fast, interactive shopping experience.
2. Blog Platform
- Example: A content-heavy blog that uses WordPress for content management and Next JS for fast page loads and SEO optimization.
3. Corporate Website
- Example: A corporate website where WordPress handles the content and Next JS powers a dynamic, modern frontend that reflects the company’s branding.
4. Portfolio Site
- Example: A designer’s portfolio that uses WordPress to manage projects and Next JS to display them in a visually engaging way.
Conclusion
In this guide, we’ve explored the powerful combination of Headless WordPress and Next JS, delving into their individual benefits and how they work together to create high-performance, scalable websites. By following the steps outlined, you can set up your own headless CMS and build a modern web application that leverages the strengths of both technologies.
Experiment with different configurations, explore additional features, and take advantage of the flexibility that this setup offers. Whether you’re building a blog, an e-commerce site, or a corporate website, the possibilities with Headless WordPress and Next JS are virtually limitless.
Regular Updates
The world of Web Development is ever-evolving, and both Headless WordPress and Next JS are continually being updated with new features and improvements. To keep your skills sharp and your projects up-to-date, regularly check for updates to both WordPress and Next JS. Stay engaged with the developer communities, participate in forums, and keep an eye on the latest trends in web development.
About Prateeksha Web Design
Prateeksha Web Design Company is a leading provider of cutting-edge website design and development services. One of its key offerings is a comprehensive guide to Headless WordPress with Next JS. This service offers an innovative approach to website building, leveraging the flexibility of WordPress and the high-performance capabilities of Next JS. It enables businesses to create fast, scalable, and SEO-friendly websites that cater to their unique needs.
Interested in learning more? Contact us today.
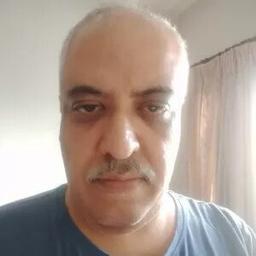