With the release of Next.js 14, the App Router has revolutionized how developers build dynamic websites and applications. Combining this cutting-edge framework with Markdown creates a powerful, modern blogging platform that is fast, scalable, and developer-friendly. In this guide, we’ll break down the entire process, ensuring you have the latest insights into Next.js blogging and Markdown.
Why Choose Next.js 14 and Markdown for Blogging?
Next.js 14 App Router simplifies routing and server-side rendering, allowing developers to create highly performant applications. Pairing it with Markdown for content management provides a lightweight, efficient solution for bloggers and small businesses.
Benefits of Next.js 14 App Router for Blogging:
- Simplified File Structure: Organize your app with a cleaner, component-based architecture.
- Server and Client Components: Choose server-side or client-side rendering as needed for each component, optimizing performance.
- Streaming and Suspense: Stream data directly to your components and render them incrementally for a seamless experience.
- SEO and Performance: Static site generation and server-side rendering ensure high search engine visibility and fast loading times.
Markdown's Role:
Markdown provides a lightweight, plain-text format for writing content, making it an excellent choice for managing blogs. Paired with tools like gray-matter
and react-markdown
, Markdown simplifies content creation and improves developer productivity.
Step 1: Setting Up Your Next.js 14 Project
To begin, set up a Next.js 14 project using the App Router.
-
Install Next.js 14:
npx create-next-app@latest my-blog-platform --experimental-app cd my-blog-platform npm run dev
-
Folder Structure: With the App Router, the
app/
directory is used instead ofpages/
. Insideapp
, you’ll define your routes, layouts, and components. -
Editor Setup: Use VS Code with extensions like ESLint and Prettier to format your code and enforce best practices.
Step 2: Adding Markdown Support
Markdown files will store your blog posts, making content creation intuitive. Here's how to integrate Markdown into your project:
-
Create a
posts/
Directory: Store your.md
files here. -
Install Dependencies:
npm install gray-matter react-markdown
gray-matter
: Parses frontmatter (metadata like title and date).react-markdown
: Converts Markdown content into React components.
-
Read Markdown Files: Create a utility function in
lib/getPosts.js
:import fs from "fs"; import path from "path"; import matter from "gray-matter"; const postsDirectory = path.join(process.cwd(), "posts"); export function getPosts() { const fileNames = fs.readdirSync(postsDirectory); return fileNames.map((fileName) => { const fullPath = path.join(postsDirectory, fileName); const fileContents = fs.readFileSync(fullPath, "utf8"); const { data, content } = matter(fileContents); return { slug: fileName.replace(/\.md$/, ""), metadata: data, content, }; }); }
Step 3: Building Dynamic Blog Routes
With the App Router, dynamic routing is more intuitive and integrated into the component-based structure.
-
Create a Dynamic Route:
- Inside the
app/
directory, create a folder structure likeapp/blog/[slug]/page.js
.
- Inside the
-
Fetch and Render Blog Content:
import fs from "fs"; import path from "path"; import matter from "gray-matter"; import ReactMarkdown from "react-markdown"; export async function generateStaticParams() { const postsDirectory = path.join(process.cwd(), "posts"); const filenames = fs.readdirSync(postsDirectory); return filenames.map((filename) => ({ slug: filename.replace(/\.md$/, ""), })); } export default async function BlogPost({ params }) { const { slug } = params; const filePath = path.join(process.cwd(), "posts", `${slug}.md`); const fileContents = fs.readFileSync(filePath, "utf8"); const { data, content } = matter(fileContents); return ( <div> <h1>{data.title}</h1> <p>{data.date}</p> <ReactMarkdown>{content}</ReactMarkdown> </div> ); }
-
Metadata for SEO: Utilize the
metadata
export for dynamic SEO metadata:export async function generateMetadata({ params }) { const { slug } = params; const filePath = path.join(process.cwd(), "posts", `${slug}.md`); const fileContents = fs.readFileSync(filePath, "utf8"); const { data } = matter(fileContents); return { title: data.title, description: data.excerpt, }; }
Step 4: Styling Your Blog with Tailwind CSS
Tailwind CSS is a popular utility-first framework that simplifies styling.
-
Install Tailwind CSS:
npm install -D <a href="/blog/understanding-tailwind-css-streamlining-web-design-with-utility-first-approach">tailwindcss</a> postcss autoprefixer npx tailwindcss init
-
Configure
tailwind.config.js
and add content paths:module.exports = { content: [ "./app/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}", ], };
-
Apply Tailwind Classes: Create reusable components for consistency.
- Example for a blog card:
export default function BlogCard({ post }) { return ( <div className="border p-4 rounded shadow-md hover:shadow-lg"> <h2 className="text-xl font-bold">{post.metadata.title}</h2> <p>{post.metadata.excerpt}</p> </div> ); }
- Example for a blog card:
Step 5: Adding Search Functionality
Enhance user experience with a search bar that filters blog posts.
-
Install
fuse.js
:npm install fuse.js
-
Implement Search:
- Add a search component that uses Fuse.js to filter blog posts by title or content.
import Fuse from "fuse.js"; import { useState } from "react"; export default function SearchBar({ posts }) { const [query, setQuery] = useState(""); const fuse = new Fuse(posts, { keys: ["metadata.title", "content"], threshold: 0.3, }); const results = query ? fuse.search(query).map((result) => result.item) : posts; return ( <div> <input type="text" placeholder="Search..." value={query} onChange={(e) => setQuery(e.target.value)} className="border p-2 rounded" /> <div> {results.map((post) => ( <BlogCard key={post.slug} post={post} /> ))} </div> </div> ); }
Step 6: Deploying Your Platform on Vercel
Deploying your blogging platform is straightforward with Vercel:
- Push Your Code to GitHub/GitLab.
- Connect to Vercel: Link your repository, and Vercel will handle the build and deployment process.
- Optimize for Performance: Use Vercel Analytics to monitor performance and make improvements.
Why Prateeksha Web Design is Your Ideal Partner
At Prateeksha Web Design, we specialize in creating high-performance, scalable web platforms using cutting-edge technologies like Next.js 14. Whether you're a small business or an individual blogger, we ensure your blogging platform is tailored to your needs, offering:
- Expert integration of Next.js blogging.
- Markdown-based content workflows for ease of use.
- Professional designs that engage and convert visitors.
Conclusion
With Next.js 14 App Router and Markdown, building a blogging platform has never been more efficient. By following this guide, you can create a modern, scalable, and SEO-friendly blog that caters to your audience. For those seeking a professional touch, Prateeksha Web Design is here to help turn your vision into reality. Let’s build something amazing together!
About Prateeksha Web Design
Prateeksha Web Design offers services to build a custom blogging platform using Next.js and Markdown, providing a seamless and user-friendly experience for bloggers. Our team of experts will help you create a dynamic and responsive website that showcases your content in a visually appealing way. We will also integrate features such as SEO optimization, social media sharing, and customizable layouts to enhance your blog's functionality. Contact us today to get started on building your dream blogging platform.
Interested in learning more? Contact us today. For more information about Next.js, visit the official Next.js website and to explore more about Markdown, check out Markdown Guide.
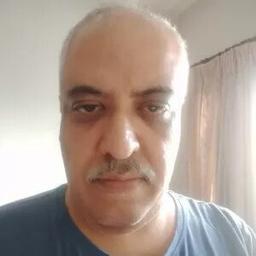