Table of Contents
- Introduction
- Understanding App Routing in Next.js
- Getting Started
- Setting Up Next.js
- Understanding Next.js Directory Structure
- Creating Pages
- Implementing App Router
- Advanced Routing Concepts
- Common Issues and Solutions
- Conclusion
Introduction
In the fast-paced world of web development, Next.js has emerged as a leading framework that simplifies the process of building React applications. It’s not just popular for its out-of-the-box features like server-side rendering (SSR) and static site generation (SSG) but also for its streamlined developer experience.
Next.js is often described as a "meta-framework" for React, meaning it takes the powerful but sometimes complex React library and adds a layer of abstraction to make it easier to work with. Developers choose Next.js because it provides a robust set of tools that simplify the complexities of modern web development, especially for production-ready applications.
In this comprehensive guide, we’ll delve into how to use the App Router in Next.js applications. Whether you’re new to Next.js or looking to deepen your understanding, this blog will walk you through every step needed to master routing in Next.js.
Understanding App Routing in Next.js
App routing is one of the fundamental aspects of web development, and in Next.js, routing is particularly unique because it’s file-based. This means that the structure of your project’s files directly influences how users navigate your application.
Traditional web applications often rely on client-side routers like React Router for managing navigation between pages. While effective, these routers can be complex to set up and maintain. Next.js simplifies this with a pages directory that automatically maps to the application's routes.
Why is routing important in Next.js? In simple terms, routing defines how users can move between different pages or views in your application. A well-structured routing system improves both the user experience and the performance of your app.
Next.js provides a simpler and more intuitive approach to routing compared to traditional methods. It’s not just about making things easier for developers; it’s also about creating more performant and SEO-friendly applications.
Getting Started
Before diving into the actual implementation of routing in Next.js, it’s crucial to ensure you have everything set up correctly.
Prerequisites
- Node.js: Ensure you have the latest LTS version of Node.js installed on your machine. You can download it from here.
- NPM or Yarn: These are package managers that come with Node.js. You’ll use them to install dependencies and run scripts.
- Basic Understanding of JavaScript and React: If you’re new to these technologies, it might be helpful to brush up on the basics of React before diving into Next.js.
- Code Editor: A good code editor like Visual Studio Code is recommended for working with Next.js projects.
Setting Up Your Environment
- Install Node.js: Follow the instructions on the Node.js website to install it on your operating system.
- Install a Code Editor: If you don’t already have one, download Visual Studio Code from [here](https://code.visualstudio.com/).
- Create a New Next.js Application: Once everything is installed, you can create a new Next.js project using the Create Next App utility, which we’ll cover in the next section.
With everything in place, you’re now ready to start building your Next.js application.
Setting Up Next.js
Creating a new Next.js project is a straightforward process, thanks to the create-next-app
command-line tool. This tool sets up a new Next.js project with all the necessary configurations and dependencies.
Step 1: Create a New Next.js Application
Open your terminal and run the following command:
npx create-next-app@latest my-<a href="/blog/building-a-high-performance-ecommerce-store-with-nextjs">nextjs</a>-app
Replace my-nextjs-app
with the name of your project. This command will create a new directory with that name, and set up a basic Next.js project inside it.
Step 2: Navigate to the Project Directory
Once the setup is complete, move into the project directory:
cd my-nextjs-app
Step 3: Start the Development Server
To start your Next.js application, run:
npm run dev
This command will start a development server at http://localhost:3000
. Open this URL in your browser, and you should see the default Next.js homepage.
Congratulations! You’ve just set up your first Next.js project. Now, let's dive into understanding how Next.js structures its files and directories, as this is crucial for routing.
Understanding Next.js Directory Structure
One of the first things you’ll notice in a new Next.js project is its directory structure. Unlike other frameworks that might require extensive configuration, Next.js projects follow a convention-over-configuration approach. Understanding this structure is key to effectively implementing routing.
Key Directories and Files
-
pages/
: This is the most important directory for routing. Each file inside thepages
directory automatically becomes a route in your application. For example, a file namedindex.js
inside thepages
directory maps to the/
route. -
public/
: This directory is where you store static assets like images, fonts, and other files. Files inpublic
are accessible from the root URL. -
styles/
: Contains global styles and CSS modules for the project. -
components/
: Typically, you would create this directory to store your React components. -
api/
: This is where you can define API routes, which are automatically mapped to/api/*
endpoints.
Pages Directory in Detail
The pages/
directory is central to routing in Next.js. Here’s how it works:
index.js
: Corresponds to the root route (/
).about.js
: Corresponds to/about
.[id].js
: Dynamic routes can be created by wrapping part of the filename in square brackets, like[id].js
, which will match any string in the URL and make it available as a query parameter.
Understanding this file-based routing system is crucial before we move on to creating pages and implementing advanced routing features.
Creating Pages
Pages in Next.js are the building blocks of your application’s routing structure. Creating pages is simple—just add a new JavaScript file to the pages/
directory, and it automatically becomes accessible via the URL that corresponds to its file path.
Creating Static Pages
Static pages are the simplest form of pages in Next.js. For example, to create an About
page, follow these steps:
- Create a new file named
about.js
inside thepages/
directory. - Add the following code to the file:
const About = () => {
return (
<div>
<h1>About Us</h1>
<p>This is the about page of our Next.js application.</p>
</div>
);
};
export default About;
- Access the page by navigating to
http://localhost:3000/about
.
Creating Dynamic Pages
Dynamic pages are useful when you need to generate pages based on dynamic data, such as user profiles or blog posts.
- Create a new file named
[id].js
inside thepages/
directory. - Add the following code to the file:
import { useRouter } from "next/router";
const Post = () => {
const router = useRouter();
const { id } = router.query;
return (
<div>
<h1>Post: {id}</h1>
<p>This is a dynamic route page with ID: {id}</p>
</div>
);
};
export default Post;
- Access the dynamic page by navigating to
http://localhost:3000/1
(or any other ID).
Creating pages is just the beginning. To fully harness the power of Next.js, you’ll need to understand how to implement routing effectively.
Implementing App Router
Routing in Next.js is intuitive, especially if you’re familiar with React. However, there are some unique features and best practices that set it apart from other frameworks.
Basic Routing with Link Component
The Link component in Next.js is essential for navigating between pages without refreshing the entire page, enabling a seamless user experience.
Here’s how to use it:
- Import the Link component from Next.js:
import Link from "next/link";
- Use the Link component in your JSX:
const Home = () => {
return (
<div>
<h1>Welcome to Our Site!</h1>
<Link href="/about">
<a>Go to About Page</a>
</Link>
</div>
);
};
export default Home;
Handling Dynamic Routes
Dynamic routing is where Next.js shines. By using dynamic route segments (like [id].js
), you can create highly flexible pages.
For instance, in a blog application, you might want to create a separate page for each blog post. By using dynamic routing, you can create one template and reuse it for every post.
import { useRouter } from "next/router";
const BlogPost = () => {
const router = useRouter();
const { id } = router.query;
return (
<div>
<h1>Blog Post ID: {id}</h1>
</div>
);
};
export default BlogPost;
Simply navigate to http://localhost:3000/post/1
(or any other ID), and the corresponding page will render.
Using Router API for Programmatic Navigation
Sometimes, you need to navigate programmatically, for example, after a form submission. The Router API provided by Next.js is perfect for this.
import { useRouter } from "next/router";
const Form = () => {
const router = useRouter();
const handleSubmit = () => {
// Perform form submission logic here
router.push("/success");
};
return (
<div>
<button onClick={handleSubmit}>Submit</button>
</div>
);
};
export default Form;
This code snippet shows how to use router.push
to navigate to a different page after a form is submitted.
Advanced Routing Concepts
Next.js also supports more advanced routing concepts, which are crucial for building complex applications.
Nested Routing
Nested routes are routes that are nested within other routes. While Next.js doesn’t support nested routes in the way some frameworks do (like Vue.js), you can achieve a similar effect by creating a folder structure in the pages
directory.
For example:
- pages/blog/index.js →
/blog
- pages/blog/[id].js →
/blog/:id
This structure creates a "nested" routing effect, where /blog
lists all posts, and /blog/:id
displays individual posts.
Catch-All Routes
Catch-all routes in Next.js allow you to capture multiple route segments in a single route.
// pages/docs/[...slug].js
import { useRouter } from "next/router";
const Docs = () => {
const router = useRouter();
const { slug } = router.query;
return (
<div>
<h1>Docs: {slug.join("/")}</h1>
</div>
);
};
export default Docs;
In this example, navigating to http://localhost:3000/docs/nextjs/routing
will capture all segments after /docs
and pass them as an array to the Docs
component.
Optional Catch-All Routes
Optional catch-all routes are similar to catch-all routes but allow the parameter to be optional.
// pages/docs/[[...slug]].js
import { useRouter } from "next/router";
const Docs = () => {
const router = useRouter();
const { slug } = router.query;
return (
<div>
<h1>Docs: {slug ? slug.join("/") : "Home"}</h1>
</div>
);
};
export default Docs;
Now, the slug
parameter can be omitted, and the route will still render the page.
Creating Custom 404 Pages
Custom 404 pages improve user experience by providing a more cohesive design when a page isn’t found.
- Create a
404.js
file inside thepages
directory. - Add your custom 404 message:
const Custom404 = () => {
return <h1>404 - Page Not Found</h1>;
};
export default Custom404;
This page will now display whenever a user navigates to a non-existent route.
Common Issues and Solutions
Even with a framework as user-friendly as Next.js, developers can encounter common issues while implementing routing. Here are some of the most frequent problems and how to solve them:
Page Not Found Errors
Problem: You’ve created a page, but when navigating to it, you encounter a 404 error.
Solution: Ensure the file is correctly named and placed in the pages/
directory. Remember, file names are case-sensitive, and even a small typo can lead to errors.
Dynamic Routes Not Working
Problem: You’ve set up a dynamic route, but it’s not behaving as expected.
Solution: Check that you’ve correctly used square brackets for dynamic segments. Also, ensure the component is correctly receiving the route parameters.
Link Component Not Working
Problem: You’re using the Link component, but clicking it doesn’t navigate to the expected page.
Solution: Ensure that the href
property is correctly set. Also, verify that you’re using the Link component correctly, wrapping it around an <a>
tag if necessary.
Using Router API Causes Errors
Problem: You’re using the Router API to navigate programmatically, but it’s causing errors or not working as expected.
Solution: Double-check the path you’re passing to router.push()
or router.replace()
. Ensure it’s a valid path within your application.
Conclusion
In this extensive guide, we’ve covered everything you need to know about implementing App Router in Next.js applications. From understanding the basics of Next.js routing to diving deep into advanced routing techniques, this blog has provided a comprehensive overview designed to help you master routing in Next.js.
Next.js makes routing simple yet powerful, allowing you to build complex and dynamic applications with ease. By understanding and utilizing the features discussed, you can create highly efficient and user-friendly web applications.
Whether you’re a beginner or an experienced developer, the concepts covered in this blog will help you navigate the world of Next.js page routing with confidence.
About Prateeksha Web Design
Prateeksha Web Design Company is renowned for providing innovative web solutions. They excel in creating Next.js applications, involving the App Router for seamless navigation. Their step-by-step guide service educates clients on using the App Router, from initial setup to advanced features. This fosters a user-friendly experience and empowers clients to manage their digital platform effectively.
Interested in learning more? Contact us today.
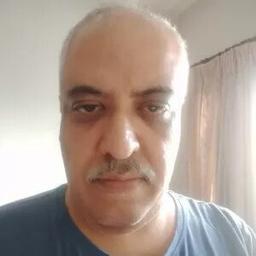