Understanding how to optimize applications is paramount in today's fast-paced web development environment, where speed and efficiency are more than mere buzzwords - it can make all the difference in terms of overall application performance. We will delve deep into Laravel data caching and Next.js server-side rendering (SSR), two powerful techniques which can significantly boost website performance - with our expert guide Prateeksha Web Design at hand to offer guidance in crafting high-performing, SEO-friendly sites!
Understanding Caching in Laravel
Laravel's elegant syntax and robust features make it the perfect platform to facilitate data caching - which can significantly reduce database loads while speeding up response times. Here's how Laravel can help:
1. Configuring Cache in Laravel
Before you start caching, you need to configure your cache settings. Laravel supports various cache drivers like Memcached, Redis, and even file-based caching. You can set your preferred cache driver in the .env
file:
CACHE_DRIVER=redis
This configuration tells Laravel to use Redis for caching, which is excellent for in-memory caching due to its speed.
2. Basic Caching Techniques
- Cache::put(): This method stores data in the cache for a specified duration. For instance:
Cache::put('key', 'value', 60); // Cache for 60 seconds
- Cache::remember(): This is a lazy loading strategy where data is fetched from the cache if available, or from the database if not, and then cached:
$posts = Cache::remember('posts.all', 30, function () {
return Post::all();
});
- Cache::forget(): To clear specific cache entries:
Cache::forget('posts.all');
3. Advanced Caching Strategies
-
Write-Through: Data is written to both the cache and the database simultaneously. This ensures data consistency but can be slower due to the double write operation.
-
Write-Around: Data is written directly to the database, bypassing the cache. This is useful when data is not frequently read.
-
Cache Aside: Data is fetched from the cache first. If not found, it's retrieved from the database and then cached for future requests. This is the most common strategy due to its simplicity and efficiency.
Server-Side Rendering (SSR) in Next.js
Now, let's shift gears to Next.js, a React framework that excels in performance optimization through SSR:
Why SSR in Next.js?
-
SEO Benefits: SSR allows search engines to crawl your pages more effectively since the content is already rendered on the server.
-
Faster Initial Load: Users see content faster as the server sends a fully rendered page, reducing the time to first paint.
-
Improved User Experience: SSR can lead to better performance metrics like First Contentful Paint (FCP) and Largest Contentful Paint (LCP).
Implementing SSR in Next.js
- getServerSideProps: This function is used to fetch data at request time on the server:
export async function getServerSideProps(context) {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: {
data,
},
};
}
- Dynamic Routes: Next.js allows for dynamic routes where SSR can be particularly beneficial:
// pages/posts/[id].js
import { useRouter } from 'next/router';
export async function getServerSideProps({ params }) {
const res = await fetch(`https://api.example.com/posts/${params.id}`);
const post = await res.json();
return { props: { post } };
}
function Post({ post }) {
// Render post data
}
Optimizing SSR in Next.js
- Caching: Implement caching strategies to reduce server load. Next.js provides built-in caching for pages:
export async function getServerSideProps({ res }) {
res.setHeader(
'Cache-Control',
'public, s-maxage=10, stale-while-revalidate=59'
);
// Fetch data
}
- Code Splitting: Use dynamic imports to load components only when needed, reducing the initial bundle size:
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(
() => import('../components/DynamicComponent')
);
- Image Optimization: Utilize Next.js's Image component for automatic image optimization:
import Image from 'next/image';
function Home() {
return <Image src="/image.jpg" width={500} height={500} alt="Description" />;
}
Prateeksha Web Design's Expertise
Prateeksha Web Design stands out with its deep understanding of both Laravel and Next.js:
-
Custom Laravel Solutions: They leverage Laravel's caching capabilities to build secure, scalable applications that perform seamlessly under load.
-
Next.js Mastery: Their team ensures that your Next.js applications are not only fast but also SEO-friendly, using SSR to its fullest potential.
-
Performance Optimization: From lazy loading to efficient data fetching, Prateeksha Web Design implements best practices to ensure your site's performance is top-notch.
Conclusion
By integrating data caching in Laravel with SSR in Next.js, you're setting your web application up for success in terms of speed, SEO, and user experience. Remember, the key is not just to implement these techniques but to do so thoughtfully, considering your application's specific needs.
Prateeksha Web Design can be your partner in this journey, ensuring that your website not only looks good but performs exceptionally well. Whether you're looking to optimize an existing site or build a new one from scratch, their expertise in both Laravel and Next.js will guide you to achieve the best results.
So, are you ready to make your website the fastest, most reliable, and engaging platform for your users? Let's dive into the world of caching and SSR with Prateeksha Web Design, and watch your site's performance soar!
About Prateeksha Web Design
Prateeksha Web Design offers expert services in optimizing data caching strategies for Laravel applications, ensuring efficient server-side rendering with Next.js. Our approach focuses on best practices for caching dynamic API responses, enhancing performance and reducing load times. We implement advanced techniques such as ETags and Cache-Control headers to manage cached data effectively. Additionally, we provide seamless integration between Laravel backends and Next.js frontends, maximizing responsiveness. Trust us to elevate your web applications with robust caching solutions tailored to your specific needs.
Interested in learning more? Contact us today.
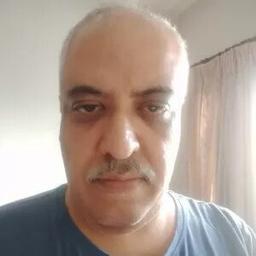