How to Implement React Portals in Next.js Projects Effectively
React Portals are an incredibly powerful tool in React for rendering components outside their parent DOM hierarchy. By leveraging this feature in Next.js, you can achieve cleaner code and implement advanced UI patterns effectively. This blog dives into the depths of React Portals and their integration with Next.js, focusing on practical applications and best practices. Whether you are a beginner or an experienced developer, you will gain valuable insights into this versatile feature. Plus, we’ll highlight how Prateeksha Web Design can help small businesses create stunning, high-performance websites.
What Are React Portals?
In React, components are typically rendered within their parent DOM hierarchy. However, there are situations where you need a component to appear outside of that structure, such as when creating modals, tooltips, or popovers. React Portals allow you to render elements into a different part of the DOM while maintaining their React context.
How React Portals Work
React Portals utilize the ReactDOM.createPortal(child, container)
method, which takes two arguments:
- Child: The React component you want to render.
- Container: The DOM node where the component should be rendered.
Here’s a simple example:
import ReactDOM from "react-dom";
function MyPortalComponent({ children }) {
return ReactDOM.createPortal(
children,
document.getElementById("portal-root")
);
}
In this case, the child component will render inside the #portal-root
element, regardless of its parent in the React tree.
Why Use React Portals in Next.js?
Next.js, a popular React framework, is known for its focus on server-side rendering, static site generation, and excellent performance. Integrating React Portals into Next.js projects allows you to:
- Enhance User Experience: Use portals to create modals, lightboxes, and notifications without disrupting the main application flow.
- Simplify Codebase: Keep the DOM structure organized while implementing complex UI patterns.
- Improve Accessibility: Ensure components like modals are placed at the top of the DOM hierarchy, avoiding z-index and positioning issues.
Setting Up React Portals in a Next.js Project
Step 1: Create a Portal Root in the DOM
First, add a div
in your pages/_document.js
file to serve as the root for your portal components. The _document.js
file is where you can customize the HTML structure of your Next.js application.
// pages/_document.js
import { Html, Head, Main, NextScript } from "next/document";
export default function Document() {
return (
<Html>
<Head />
<body>
<Main />
<div id="portal-root"></div>
<NextScript />
</body>
</Html>
);
}
The #portal-root
div will act as the container for all your portal components.
Step 2: Build a Portal Component
Now, create a reusable Portal component to handle the portal logic. Here’s how you can do it:
import ReactDOM from "react-dom";
const Portal = ({ children }) => {
if (typeof window === "undefined") {
return null; // Return null during <a href="/blog/next-js-vs-traditional-ssr-why-next-js-is-the-future-of-seo-friendly-web-apps">server-side rendering</a>
}
const portalRoot = document.getElementById("portal-root");
return portalRoot ? ReactDOM.createPortal(children, portalRoot) : null;
};
export default Portal;
This component ensures compatibility with Next.js, where server-side rendering can cause issues with DOM-related operations.
Step 3: Using the Portal Component
Here’s how to use the Portal component in your application. Let’s create a modal as an example:
import React, { useState } from "react";
import Portal from "./Portal";
const Modal = ({ onClose }) => (
<div className="modal-backdrop">
<div className="modal-content">
<p>This is a modal!</p>
<button onClick={onClose}>Close</button>
</div>
</div>
);
const App = () => {
const [isOpen, setIsOpen] = useState(false);
return (
<div>
<button onClick={() => setIsOpen(true)}>Open Modal</button>
{isOpen && (
<Portal>
<Modal onClose={() => setIsOpen(false)} />
</Portal>
)}
</div>
);
};
export default App;
Key Considerations When Using React Portals in Next.js
- Server-Side Rendering (SSR): Since portals interact with the DOM, they should only render on the client side. Handle SSR with conditional rendering, as shown in the
Portal
component example. - Styling: Ensure your portal components have their own styles to avoid conflicts with the rest of the application.
- Event Bubbling: React Portals still participate in React's event bubbling, meaning event listeners will behave as if the portal component is part of the React tree.
Advanced Applications of React Portals in Next.js
1. Modals and Popups
Using portals for modals ensures they are rendered outside the main application DOM hierarchy, preventing issues with z-index and positioning.
2. Notifications
Portals are ideal for displaying global notifications or toast messages at the top level of your application.
3. Tooltips
Use portals to create tooltips that appear in specific areas of your application without disrupting the existing DOM structure.
Recent Advancements in React Portals and Next.js
With the latest updates in React and Next.js:
- Improved Performance: React’s concurrent rendering capabilities enhance the performance of portals in large applications.
- Integration with CSS-in-JS: Tools like Emotion and Styled-Components now offer better support for styling portal components.
- Server Components: Next.js has introduced server components, allowing developers to manage rendering logic more effectively alongside portals.
Benefits of Partnering with Prateeksha Web Design
At Prateeksha Web Design, we specialize in React.js and Next.js development. Our team of experts can help your small business leverage technologies like React Portals to build interactive and efficient web applications.
- Expertise in Modern Web Development: With decades of experience, we create scalable, SEO-friendly, and high-performance websites.
- Tailored Solutions: We understand the unique challenges of small businesses and craft Custom Solutions that meet your needs.
- Dedicated Support: From initial consultation to post-launch support, we are with you every step of the way.
Contact us today to explore how React Portals and other cutting-edge technologies can transform your online presence!
Conclusion
Implementing React Portals in a Next.js project might seem complex at first, but with the right guidance and tools, it can significantly enhance your application's usability and design. Whether you’re creating modals, tooltips, or notifications, portals provide an elegant solution to render components outside the parent DOM while maintaining their React context.
By following the strategies and insights outlined in this guide, you can confidently integrate React Portals into your Next.js projects, improving both developer experience and end-user satisfaction.
And remember, if you need expert assistance in building modern, scalable web applications, Prateeksha Web Design is here to help!
About Prateeksha Web Design
Prateeksha Web Design offers services to effectively implement React Portals in Next.js projects. This includes creating encapsulated UI components, managing complex UI interactivity, and ensuring seamless integration with the Next.js framework. They optimize performance by leveraging server-side rendering capabilities of Next.js. Their team of experts also provide robust error handling and maintain code consistency across the project.
Interested in learning more? Contact us today.
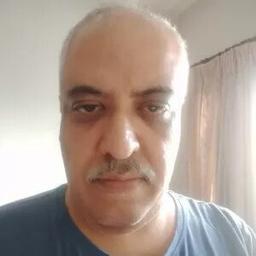