Dynamic routing is one of the most exciting features of Next.js, enabling developers to create dynamic web applications with ease. In this guide, we'll dive deep into how dynamic routing works, why it's a game-changer for web developers, and how you can master it. This comprehensive guide is packed with insights and tips to help both beginners and experienced developers understand the nuances of dynamic routing in Next.js. We'll also explore how Prateeksha Web Design's expertise can help small businesses harness the power of Next.js for building modern, dynamic websites.
What is Dynamic Routing?
Dynamic routing is a technique that allows you to define routes in your application that change dynamically based on user input, database content, or other external data sources. Unlike static routing, which requires developers to hard-code individual paths or URLs for each page, dynamic routing uses templates that adapt based on parameters passed to them. This makes it incredibly powerful for applications that need to handle a lot of unique pages without duplicating code.
Breaking It Down: Static vs. Dynamic Routing
Static Routing:
In static routing, every page has a fixed path defined explicitly by the developer. For example:
/about
leads to an About page./contact
leads to a Contact page.
Static routing is suitable for applications with a small number of pages where the structure doesn’t need to change often.
Dynamic Routing:
Dynamic routing, on the other hand, uses placeholders in the route definitions. These placeholders are replaced with actual values at runtime, creating pages dynamically. For instance:
/blog/[slug]
can handle URLs like/blog/how-to-code
or/blog/nextjs-tutorial
.
Why Do You Need Dynamic Routing?
Dynamic routing is essential when your application involves a large number of similar pages where creating separate static routes for each would be inefficient. Examples include:
- Blogs: Each post has a unique URL based on its title or ID.
- E-commerce: Product pages dynamically generated based on product IDs or names.
- Portfolios: Each project or client testimonial has a dedicated page.
By leveraging dynamic routing, you can avoid hardcoding paths and make your application more scalable and maintainable.
Benefits of Dynamic Routing in Next.js
-
Create Flexible User Experiences
Dynamic routing adapts content on the fly based on user inputs or parameters. This enables personalized experiences like showing product recommendations or user-specific dashboards. -
Reduce Redundancy
With dynamic routes, you can use a single file or template to manage similar content types. For instance, a single route template/product/[id]
can handle all your product pages instead of creating one for each product. -
Optimize SEO
Dynamic routing enables you to create meaningful and keyword-rich URLs, such as/blog/best-seo-practices
, which help search engines understand and rank your pages better.
Examples of Dynamic Routing in Action
Blog Application:
Suppose you’re building a blog platform. Without dynamic routing, you’d need to create separate files like these:
/pages/blog/post1.js
/pages/blog/post2.js
/pages/blog/post3.js
With dynamic routing, you can create a single file:
/pages/blog/[slug].js
When users visit /blog/my-first-post
, the [slug]
part dynamically picks up my-first-post
and renders the appropriate content.
E-Commerce Store:
Consider an online store with hundreds of products. Instead of creating:
/pages/product/shirt.js
/pages/product/shoes.js
/pages/product/watch.js
You can create one dynamic route file:
/pages/product/[id].js
This single route dynamically renders any product page based on the id
parameter.
Setting Up Dynamic Routes in Next.js
Step 1: Folder-Based Routing
Next.js uses a file-based routing system. This means that the structure of the /pages
directory directly maps to the structure of your site’s URLs. To create a dynamic route, use square brackets ([]
) to define placeholders for dynamic segments.
Example:
- File:
/pages/blog/[slug].js
- URL:
/blog/my-first-post
Here:
slug
is a dynamic parameter.- The file
/blog/[slug].js
serves as a template for all blog posts.
Step 2: Accessing Dynamic Parameters in Code
Once you’ve set up a dynamic route, you need to access the dynamic parameters (e.g., slug
) within your component. Next.js provides multiple ways to do this:
- Using
useRouter
:
TheuseRouter
hook lets you access route parameters directly in your component.
Example:
import { useRouter } from 'next/router';
const BlogPost = () => {
const router = useRouter();
const { slug } = router.query; // Access the dynamic 'slug' parameter
return <h1>Post: {slug}</h1>; // Render the content based on 'slug'
};
export default BlogPost;
Explanation:
- The
useRouter
hook gives you access to therouter
object. router.query.slug
retrieves the value passed in the URL.
- Using
getStaticProps
andgetServerSideProps
:
For static generation (SSG) or server-side rendering (SSR), Next.js allows you to fetch dynamic data for each route.
Example with SSG:
export async function getStaticPaths() {
const posts = await fetchPosts(); // Fetch post data from an API or database
const paths = posts.map((post) => ({
params: { slug: post.slug }, // Pass dynamic slug
}));
return { paths, fallback: false };
}
export async function getStaticProps({ params }) {
const post = await fetchPost(params.slug); // Fetch data for a single post
return { props: { post } }; // Pass post data as props
}
const BlogPost = ({ post }) => {
return <h1>{post.title}</h1>; // Render post content dynamically
};
export default BlogPost;
Key Points:
getStaticPaths
generates all possible dynamic paths at build time.getStaticProps
fetches the content for each path.
Static Generation (SSG) with Dynamic Routes
Static Site Generation (SSG) is a core feature of Next.js that pre-renders pages at build time. It generates HTML files for your application, ensuring excellent performance and SEO by serving fully-rendered pages directly from a CDN or server.
When combined with dynamic routing, SSG becomes a powerful tool for creating scalable applications that handle numerous dynamic pages—such as blogs, e-commerce product pages, or user profiles—without sacrificing performance.
The Role of getStaticPaths
and getStaticProps
in SSG with Dynamic Routes
To enable SSG for dynamic routes, you need two key functions in Next.js: getStaticPaths
and getStaticProps
. Let’s break these down.
getStaticPaths
: Defining Paths for Dynamic Routes
The getStaticPaths
function specifies the dynamic routes that should be statically generated at build time. It retrieves all the possible paths (URLs) your application should generate based on your data source (e.g., database or API).
Example:
Imagine a blog with posts fetched from an external API. Each post has a unique slug
(e.g., my-first-post
or nextjs-guide
).
Here’s how you define dynamic paths:
export async function getStaticPaths() {
// Fetch data (e.g., posts) from an API or database
const posts = await fetchPosts();
// Generate paths for each post
const paths = posts.map((post) => ({
params: { slug: post.slug }, // 'slug' corresponds to the dynamic part in [slug].js
}));
// Return paths and set fallback behavior
return { paths, fallback: false };
}
Key Points:
paths
: An array of objects representing the URLs to be generated. Example:[{ params: { slug: "my-first-post" } }, { params: { slug: "nextjs-guide" } }]
fallback
: Determines what happens when a user visits a path that wasn’t pre-rendered:false
: Only the specified paths are generated, and 404 errors are returned for other paths.true
: Unspecified paths are dynamically generated when first visited."blocking"
: Unspecified paths are dynamically generated but delay rendering until ready.
getStaticProps
: Fetching Data for Each Page
The getStaticProps
function is called for every path defined in getStaticPaths
. It fetches the necessary data to render the page.
Example:
Using the slug
parameter provided by getStaticPaths
, you can fetch detailed data for each blog post:
export async function getStaticProps({ params }) {
// Fetch detailed data for a specific post
const post = await fetchPost(params.slug);
// Pass the fetched data as props to the page component
return { props: { post } };
}
Key Points:
- The
params
object contains dynamic route parameters (e.g.,slug
). - The returned
props
object is passed to the page component as props.
Complete Example: Dynamic Blog with SSG
Here’s how it all comes together:
-
Define the dynamic route in your
/pages
folder:/pages/blog/[slug].js
-
Add
getStaticPaths
andgetStaticProps
:// Fetch all possible paths export async function getStaticPaths() { const posts = await fetchPosts(); const paths = posts.map((post) => ({ params: { slug: post.slug }, })); return { paths, fallback: false }; } // Fetch data for each path export async function getStaticProps({ params }) { const post = await fetchPost(params.slug); return { props: { post } }; } // Component rendering the blog post const BlogPost = ({ post }) => { return ( <div> <h1>{post.title}</h1> <p>{post.content}</p> </div> ); }; export default BlogPost;
Key Benefits of SSG with Dynamic Routes
-
Performance:
Pre-rendered pages are lightning-fast because they are served as static files. This is ideal for sites with heavy traffic. -
SEO:
Search engines can easily crawl and index fully-rendered pages, improving your site’s ranking. -
Cost Efficiency:
Hosting static files on a CDN (like Vercel or AWS) is more cost-effective than running server-side processes for every request. -
Scalability:
SSG handles scaling effortlessly. Once the pages are generated at build time, they can be served to millions of users without additional backend processing.
Incremental Static Regeneration (ISR): Enhancing SSG
One of the latest advancements in Next.js is Incremental Static Regeneration (ISR). It allows you to regenerate static pages after the site is built, ensuring your content stays fresh.
Key Features of ISR:
- Revalidation: Specify how often a page should be regenerated (e.g., every 60 seconds).
- No Full Rebuilds: Only updated pages are regenerated, saving time.
Example:
export async function getStaticProps() {
const data = await fetchData();
return {
props: { data },
revalidate: 60, // Regenerate the page every 60 seconds
};
}
When to Use SSG with Dynamic Routes?
- Static Content: Use SSG for pages with content that doesn’t change often, such as blogs, documentation, or product listings.
- SEO-Driven Pages: For better SEO, pre-rendered pages with keyword-rich URLs rank higher.
- High-Traffic Sites: Pre-generated pages ensure optimal performance under heavy loads.
Server-Side Rendering (SSR) vs. Static Site Generation (SSG)
SSG:
- Best for: Pages with mostly static content.
- Performance: Faster, as pages are pre-built.
- Data: Fetched at build time.
- Use Cases: Blogs, landing pages, documentation.
SSR:
- Best for: Pages with frequently changing or real-time content.
- Performance: Slower, as pages are built on request.
- Data: Fetched on every request.
- Use Cases: Dashboards, authenticated pages, dynamic user data.
Example of SSR:
export async function getServerSideProps({ params }) {
const post = await fetchPost(params.slug);
return { props: { post } };
}
Advanced Dynamic Routing Techniques
Dynamic routing in Next.js is incredibly versatile, and advanced techniques like catch-all routes, optional catch-all routes, and API routes with dynamic parameters push its capabilities further. These methods allow developers to handle complex URL structures and customize the behavior of their applications dynamically.
Catch-All Routes
Catch-all routes in Next.js handle multiple dynamic URL segments within a single route. They are defined by adding [...params]
in the file name inside the /pages
directory. This captures all additional segments in the URL as an array.
Example:
Imagine a documentation website where each section is nested, such as:
/docs/nextjs/dynamic-routing
/docs/react/hooks
/docs/javascript/closures
To create a single route that can handle all these URLs:
/pages/docs/[...slug].js
Code Implementation:
import { useRouter } from 'next/router';
const Documentation = () => {
const router = useRouter();
const { slug } = router.query;
return (
<div>
<h1>Documentation Page</h1>
<p>Segments: {slug ? slug.join(' / ') : 'Home'}</p>
</div>
);
};
export default Documentation;
Behavior:
- Visiting
/docs/nextjs/dynamic-routing
will result inslug = ['nextjs', 'dynamic-routing']
. - Visiting
/docs/javascript/closures
will result inslug = ['javascript', 'closures']
.
Why Use Catch-All Routes?
- They simplify routing for deeply nested structures.
- They allow a single route file to handle various segment combinations.
- They improve scalability, as you don’t need to define each nested route individually.
Optional Catch-All Routes
Optional catch-all routes are a variation of catch-all routes that allow the dynamic segment to be optional. They are defined using [[...params]]
in the file name.
Example:
For a route /docs
, you may want it to handle:
/docs
(no additional segments)/docs/nextjs
/docs/nextjs/dynamic-routing
To create an optional catch-all route:
/pages/docs/[[...slug]].js
Code Implementation:
import { useRouter } from 'next/router';
const Documentation = () => {
const router = useRouter();
const { slug } = router.query;
return (
<div>
<h1>Documentation Page</h1>
{slug ? (
<p>Segments: {slug.join(' / ')}</p>
) : (
<p>Welcome to the Documentation Home</p>
)}
</div>
);
};
export default Documentation;
Behavior:
- Visiting
/docs
results inslug = undefined
(or an empty array). - Visiting
/docs/nextjs
results inslug = ['nextjs']
. - Visiting
/docs/nextjs/dynamic-routing
results inslug = ['nextjs', 'dynamic-routing']
.
Why Use Optional Catch-All Routes?
- They allow a single route to serve both base and nested pages.
- They reduce the need for separate files for default and nested routes.
- They provide flexibility for handling varying URL depths.
API Routes with Dynamic Parameters
Dynamic routing isn’t limited to pages—it can also be applied to API routes in Next.js. This is particularly useful when building dynamic APIs that respond to different parameters in the URL.
Example:
For an API that retrieves post data by id
:
/pages/api/post/[id].js
Code Implementation:
export default function handler(req, res) {
const { id } = req.query; // Extract the dynamic parameter from the request
res.status(200).json({ postId: id }); // Return the response
}
Behavior:
- A request to
/api/post/123
will respond with:{ "postId": "123" }
Why Use Dynamic API Routes?
- They simplify API design for resource-based routing.
- They integrate seamlessly with dynamic frontend routes.
- They enhance flexibility by allowing parameterized requests.
Optimizing SEO with Dynamic Routes
To maximize SEO potential, dynamic routes should be designed with user-friendly and keyword-rich URLs. Here’s how to do it effectively:
1. Use Descriptive Paths
Instead of using generic dynamic segments (/product/[id]
), opt for meaningful ones like:
/product/[category]/[slug]
Example:/product/electronics/smartphone
.
2. Add Dynamic Meta Tags
Leverage the next/head
component to dynamically generate meta tags for each page. This ensures that search engines can crawl and rank your pages effectively.
Code Example:
import Head from 'next/head';
const BlogPost = ({ post }) => {
return (
<>
<Head>
<title>{post.title}</title>
<meta name="description" content={post.description} />
</Head>
<h1>{post.title}</h1>
</>
);
};
Benefits:
- Improves click-through rates by displaying relevant titles and descriptions in search results.
- Enhances content discoverability.
Latest Insights and Best Practices
Combining SSG and SSR
While SSG provides excellent performance, some pages may require real-time updates. Incremental Static Regeneration (ISR) bridges this gap by allowing static pages to refresh periodically.
Example:
export async function getStaticProps() {
return {
props: { data },
revalidate: 60, // Regenerate every 60 seconds
};
}
Benefits:
- Reduces rebuild times for large websites.
- Keeps content fresh without compromising performance.
Middleware for Advanced Routing
Middleware allows you to run logic before a request is completed. It’s ideal for scenarios like:
- Authentication: Redirect users based on login status.
- A/B Testing: Serve different versions of a page to users.
- Internationalization: Dynamically load content based on the user’s locale.
Example Middleware:
export function middleware(req) {
const url = req.nextUrl.clone();
const locale = req.headers['accept-language'].split(',')[0];
url.pathname = `/${locale}${url.pathname}`; // Redirect based on locale
return NextResponse.redirect(url);
}
Why Choose Prateeksha Web Design?
At Prateeksha Web Design, we specialize in creating dynamic, scalable, and SEO-friendly websites using Next.js. Our team can help small businesses build cutting-edge web applications that deliver seamless user experiences. Whether you need a blog, an e-commerce platform, or a dynamic portfolio, we ensure top-notch performance and reliability.
Why Trust Us?
- Proven expertise in Next.js development.
- Tailored solutions for small businesses.
- Commitment to SEO best practices for improved visibility.
Conclusion
Dynamic routing in Next.js is a powerful tool that allows developers to create modern, efficient, and user-friendly web applications. By mastering concepts like SSG, SSR, and ISR, you can build websites that are not only fast but also adaptable to changing content needs.
If you’re ready to take your web development game to the next level, consider partnering with Prateeksha Web Design. Let us help you build the future of your online presence. 🚀
About Prateeksha Web Design
Prateeksha Web Design offers expert services in implementing dynamic routing in Next.js, a popular JavaScript framework. Our team of skilled developers can create dynamic routes to enhance user experience and optimize website performance. We specialize in building custom routing solutions tailored to meet the specific needs of each client. With our expertise in Next.js, we can help you create dynamic and interactive web applications that drive engagement and conversions. Contact us today to learn more about our dynamic routing services.
Interested in learning more? Contact us today.
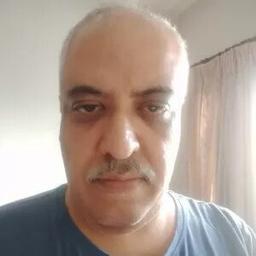