Table of Contents
- Introduction
- Understanding Next.JS
- Understanding React
- Integrating Next.JS with React
- Key Next.JS React Fundamentals
- Creating and Rendering Components in Next.JS and React
- State and Props in React
- Next.JS Routing System
- Handling Events in Next.JS and React
- Lifecycle Methods in React
- Server-Side Rendering in Next.JS
- Data Fetching Methods in Next.JS
- Building Static and Dynamic Pages in Next.JS
- Using CSS in JS with Next.JS and React
- Practical Examples
- Conclusion
- References
1. Introduction
In the fast-evolving world of web development, Next.JS and React have emerged as essential tools for developers. Understanding these technologies is crucial for building modern, scalable, and efficient web applications. Next.JS is a powerful framework built on top of React, offering features like server-side rendering, static site generation, and seamless integration with various tools and APIs. Meanwhile, React is a JavaScript library used for building user interfaces, particularly single-page applications where performance and interactivity are key.
Next.JS and React are like two sides of the same coin in the world of modern web development. While React handles the front-end, Next.JS provides the additional features and optimizations needed to create production-ready applications. As a developer, mastering these tools not only enhances your ability to build robust applications but also opens up opportunities to work on cutting-edge projects.
2. Understanding Next.JS
Next.JS is a React framework that simplifies the process of building web applications by providing a rich set of features out of the box. It's known for its ability to handle server-side rendering (SSR) and static site generation (SSG), which are crucial for improving application performance and SEO.
What is Next.JS?
Next.JS is an open-source framework created by Vercel (formerly known as Zeit). It was designed to make the development of React applications easier by providing a set of tools and conventions. At its core, Next.JS allows you to build web applications that are fast, scalable, and optimized for search engines.
Key Features of Next.JS
-
Server-Side Rendering (SSR): One of the standout features of Next.JS is SSR, which allows you to render pages on the server before sending them to the client. This improves the initial load time and SEO performance.
-
Static Site Generation (SSG): SSG allows you to pre-render pages at build time. This is ideal for content-heavy websites like blogs or e-commerce stores where the content doesn't change often.
-
API Routes: Next.JS provides a way to create API endpoints within your application. This is useful for handling server-side logic like form submissions, data fetching, and more.
-
Image Optimization: Next.JS includes an image component that optimizes images automatically. This improves load times and reduces bandwidth usage.
-
Automatic Routing: With Next.JS, pages are automatically mapped based on the file structure. This makes it easy to manage routes without the need for additional configuration.
-
Incremental Static Regeneration (ISR): ISR allows you to update static content after the site has been built, providing the flexibility of dynamic content while maintaining the performance benefits of static content.
Why Use Next.JS?
Next.JS is widely used in the industry due to its ability to handle complex applications with ease. It simplifies the development process, especially for developers working with React. The framework's built-in optimizations, like code-splitting and pre-fetching, ensure that applications are fast and responsive. Additionally, its seamless integration with various tools and APIs makes it a versatile choice for developers.
3. Understanding React
React is a JavaScript library for building user interfaces, particularly single-page applications where data changes dynamically over time. Created by Facebook, React has become one of the most popular libraries for front-end development due to its simplicity and flexibility.
What is React?
React is a component-based library that allows developers to create reusable UI components. These components manage their own state, making it easy to build complex UIs from small, isolated pieces of code. React is designed to be declarative, meaning that developers describe how the UI should look at any given time, and React takes care of updating the DOM to match that state.
Key Features of React
-
Component-Based Architecture: React encourages the creation of reusable components, which can be combined to build complex user interfaces. This modular approach simplifies the development process and makes the code more maintainable.
-
Virtual DOM: React uses a Virtual DOM to optimize updates to the actual DOM. When the state of a component changes, React creates a new Virtual DOM tree and compares it to the previous one. Only the differences are then applied to the real DOM, improving performance.
-
JSX: JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files. This makes it easier to create UI components and understand how they will be rendered.
-
Unidirectional Data Flow: React enforces a unidirectional data flow, meaning that data flows from parent components to child components. This makes it easier to debug and understand how data changes in your application.
-
React Hooks: Hooks are functions that allow you to use state and other React features in functional components. They simplify the way you manage state and side effects in your components.
Why Use React?
React's popularity stems from its simplicity, flexibility, and performance. It is widely adopted by large companies and startups alike due to its ability to build fast, responsive user interfaces. React's component-based architecture makes it easy to develop and maintain complex applications, while its Virtual DOM ensures that updates are efficient and performant. Additionally, React has a large ecosystem of tools, libraries, and community support, making it a solid choice for any front-end project.
4. Integrating Next.JS with React
While React handles the front-end aspects of a web application, Next.JS enhances React's capabilities by adding powerful features like server-side rendering, static site generation, and optimized performance. This section will explore how to integrate these two technologies to create a robust and efficient web application.
How Next.JS Enhances React
Next.JS builds on top of React, providing additional tools and conventions that make it easier to build production-ready applications. Some of the key enhancements include:
-
Automatic Routing: In a standard React application, you would typically use a library like React Router to manage routes. Next.JS, however, handles routing automatically based on the file structure in the
pages
directory. Each file corresponds to a route, which simplifies navigation within the app. -
Server-Side Rendering: React is primarily a client-side library, meaning that it renders components on the client. Next.JS adds the ability to render components on the server, improving performance and SEO.
-
Static Site Generation: Next.JS allows you to generate static HTML at build time, which can then be served to clients. This reduces the load on the server and improves the performance of your application.
-
API Routes: Next.JS provides a way to create API routes within the application. This eliminates the need to set up a separate backend server for handling API requests.
-
Performance Optimization: Next.JS includes several performance optimizations out of the box, such as code splitting, pre-fetching, and image optimization. These features ensure that your application loads quickly and efficiently.
Creating a Next.JS Application with React
To illustrate how Next.JS and React work together, let's go through the process of creating a simple Next.JS application.
-
Setting Up the Project:
First, you'll need to install Next.JS. You can do this using npm or yarn:
npx create-next-app my-<a href="/blog/building-a-high-performance-ecommerce-store-with-nextjs">nextjs</a>-app cd my-nextjs-app
This command will create a new Next.JS project with all the necessary dependencies.
-
Creating a Page Component:
In Next.JS, pages are stored in the
pages
directory. Create a new file calledindex.js
:import React from "react"; const HomePage = () => { return ( <div> <h1>Welcome to My Next.JS App!</h1> <p>This is a simple Next.JS application with React.</p> </div> ); }; export default HomePage;
This component will be rendered when you visit the root URL of your application.
-
Running the Application:
To start the development server, use the following command:
npm run dev
Your application will be available at
http://localhost:3000
.
4
. Adding Navigation:
Next.JS handles routing automatically, so adding new pages is as simple as creating a new file in the pages
directory. For example, you can create an about.js
file:
import React from "react";
import Link from "next/link";
const AboutPage = () => {
return (
<div>
<h1>About This App</h1>
<p>This app is built using Next.JS and React.</p>
<Link href="/">Go back to Home</Link>
</div>
);
};
export default AboutPage;
This creates a new page that you can navigate to by visiting http://localhost:3000/about
.
5. Key Next.JS React Fundamentals
Understanding the fundamentals of Next.JS and React is crucial for any developer looking to build modern web applications. In this section, we'll cover some of the most important concepts and features that you should be familiar with.
Creating and Rendering Components in Next.JS and React
Components are the building blocks of any React application. In Next.JS, components can be used to create pages, layout components, and even API routes. When creating components, it's important to understand how they are rendered in both client-side and server-side environments.
State and Props in React
State and props are two fundamental concepts in React that allow you to manage and pass data within your application. State is used to store data that can change over time, while props are used to pass data from parent components to child components. Understanding how to use state and props effectively is key to building dynamic and responsive UIs.
Next.JS Routing System
Next.JS simplifies routing by automatically mapping file paths to routes. This means that you don't need to configure a router manually; instead, you can create files in the pages
directory, and Next.JS will handle the rest. Additionally, Next.JS supports dynamic routing, allowing you to create pages with dynamic parameters.
Handling Events in Next.JS and React
Handling events in React is similar to handling events in regular HTML. However, React uses a synthetic event system, which means that events are normalized across different browsers. In Next.JS, you can handle events in both client-side and server-side environments, depending on your needs.
Lifecycle Methods in React
Lifecycle methods are functions that get called at different stages of a component's life. These include methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
. With the introduction of React Hooks, many of these lifecycle methods can now be replaced with hooks like useEffect
.
Server-Side Rendering in Next.JS
Server-side rendering (SSR) is one of the key features of Next.JS. It allows you to render pages on the server before sending them to the client, improving performance and SEO. In Next.JS, you can implement SSR by using the getServerSideProps
function in your pages.
Data Fetching Methods in Next.JS
Next.JS provides several methods for fetching data in your application, including getStaticProps
, getServerSideProps
, and getStaticPaths
. These methods allow you to fetch data at build time, request time, or on-demand, depending on your application's needs.
Building Static and Dynamic Pages in Next.JS
Next.JS allows you to build both static and dynamic pages with ease. Static pages are pre-rendered at build time, while dynamic pages are rendered on the server or client based on user input or other data. This flexibility makes Next.JS a powerful tool for building a wide range of applications.
Using CSS in JS with Next.JS and React
Next.JS supports several ways to style your application, including CSS Modules, Styled JSX, and popular CSS-in-JS libraries like styled-components and emotion. Each method has its advantages and trade-offs, and understanding how to use them effectively is important for creating maintainable and scalable applications.
6. Practical Examples
In this section, we'll provide practical examples and code snippets to help you understand the key concepts discussed in the blog. These examples will cover common use cases in Next.JS and React, such as creating components, handling state, and implementing server-side rendering.
7. Conclusion
Understanding the fundamentals of Next.JS and React is essential for any developer looking to build modern web applications. These technologies provide the tools and features needed to create fast, scalable, and maintainable applications. By mastering the concepts covered in this blog, you'll be well-equipped to tackle any web development project with confidence.
8. References
For more in-depth information, you can refer to the official documentation for Next.JS and React. These resources provide comprehensive guides and examples to help you further your understanding of these technologies.
About Prateeksha Web Design
Prateeksha Web Design is a leading web development company specializing in creating interactive and user-friendly websites. Their proficient team is well-versed in Next.JS React, a popular JavaScript library, and they ensure every developer is well-equipped with its fundamental aspects. The services include server-side rendering, static website generation, and creating API routes, ensuring a high-performing, SEO-friendly website.
Interested in learning more? Contact us today.
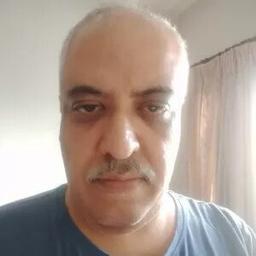