Hey there, future SaaS moguls! Are you ready to dive into the exciting world of building a multi-tenant SaaS platform? If you're nodding your head, then buckle up because we're about to embark on a journey through the realms of Laravel and Next.js, two powerhouse technologies that can make your SaaS dreams come true. And guess what? We've got the experts from Prateeksha Web Design guiding us through this adventure, ensuring we get the best insights and practices straight from the pros!
What's the Big Deal with Multi-Tenant SaaS?
First off, let's break down what we mean by multi-tenant SaaS. Imagine you're running a software service where multiple customers (or tenants) use the same application instance but with their own isolated data. This setup is like having an apartment building where each tenant has their own space, but they all share the same building infrastructure. Here's why this model rocks:
-
Cost Efficiency: You're not spinning up a new server for each customer. Instead, you're sharing resources, which means lower costs for you and your users.
-
Scalability: As your user base grows, your infrastructure can scale effortlessly. No need to worry about setting up new environments for each new tenant.
-
Maintenance: Updates, patches, and upgrades? Do it once, and it's done for all tenants. Talk about time-saving!
-
Customization: While tenants share the core application, they can still have personalized experiences through configurations.
Why Laravel for Your SaaS Backend?
Laravel isn't just another PHP framework; it's like the Swiss Army knife for web developers, especially when you're into SaaS:
-
Eloquent ORM: This feature makes database interactions a breeze. You can work with your database like you're dealing with PHP objects, which is super intuitive.
-
Artisan Console: Automate repetitive tasks with Laravel's command-line tool. It's like having a personal assistant for your development needs.
-
Blade Templates: For those dynamic, interactive front-ends, Blade is your go-to. It's lightweight and integrates seamlessly with Laravel.
-
Security: Laravel comes with built-in security features like CSRF protection, SQL injection prevention, and more, which are crucial for SaaS applications where security is paramount.
-
API Development: Laravel makes it straightforward to build RESTful APIs, which is essential for integrating with your Next.js frontend.
Next.js: The Frontend Powerhouse
Now, let's talk about Next.js, the React framework that's perfect for your SaaS frontend:
-
Server-Side Rendering (SSR): This isn't just about SEO; it's about providing a fast, smooth user experience right from the first load.
-
Static Generation: Pre-render pages at build time for even faster load times. This is gold for performance.
-
API Routes: Next.js allows you to create API routes directly within your project, making it super easy to connect with your Laravel backend.
-
Integration with Laravel: Next.js and Laravel can work together like peanut butter and jelly. Laravel handles the heavy lifting on the server, while Next.js takes care of the client-side rendering and interactivity.
Building Your Multi-Tenant SaaS with Laravel and Next.js
Step 1: Setting Up Your Development Environment
Before diving into the development of your multi-tenant SaaS platform with Laravel and Next.js, setting up your development environment correctly is crucial. Here's a detailed guide on how to prepare:
1. Install Laravel
Laravel, known for its elegant syntax and robust features, will serve as the backend for your SaaS application. Here's how to get started:
-
Prerequisites: Ensure you have PHP (version 8.1 or higher) and Composer installed on your system. If not, download PHP from the official PHP website and follow the installation instructions for your operating system. For Composer, visit the Composer download page and follow the setup instructions.
-
Installation:
-
Open your terminal or command prompt.
-
Navigate to the directory where you want to create your project.
-
Run the following command to create a new Laravel project:
composer create-project --prefer-dist <a href="/blog/top-laravel-packages-for-invoice-management-and-generation">laravel</a>/laravel your-project-name
-
Replace
your-project-name
with the name you want for your project. This command will download and set up Laravel with all its dependencies.
-
-
Verify Installation: After installation, navigate into your project directory:
cd your-project-name
Then, start the Laravel development server to ensure everything is working:
php artisan serve
You should see a message indicating that the server is running, typically on
http://127.0.0.1:8000
.
2. Setup Next.js
Next.js will be your frontend framework, providing server-side rendering and static generation capabilities:
-
Prerequisites: You need Node.js (version 12.22.0 or later) and npm (Node Package Manager) installed. If not, download Node.js from nodejs.org which includes npm.
-
Installation:
-
Open your terminal or command prompt.
-
Use the
create-next-app
CLI to set up a new Next.js project:npx create-next-app@latest your-frontend-name
-
Answer the prompts regarding TypeScript, ESLint, Tailwind CSS, etc., according to your project needs. This command will create a new Next.js application with all the necessary configurations.
-
-
Verify Installation: Navigate into your Next.js project:
cd your-frontend-name
Start the development server:
npm run dev
Your Next.js app should now be running on
http://localhost:3000
.
3. Database Setup
-
Choose Your Database: Laravel supports multiple databases like MySQL, PostgreSQL, SQLite, and SQL Server. For this guide, let's assume you're using MySQL:
-
Install MySQL: If not already installed, download and install MySQL from the official MySQL website.
-
Configure Laravel: Open the
.env
file in your Laravel project root directory. Update the database configuration:DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=your_database_name DB_USERNAME=your_username DB_PASSWORD=your_password
-
Create Database: Use MySQL command line or a GUI tool like phpMyAdmin to create the database you specified in the
.env
file.
-
4. Version Control with Git
-
Initialize Git: In both your Laravel and Next.js project directories:
git init
-
Add Files: Add all files to Git:
git add .
-
Commit Changes: Make your initial commit:
git commit -m "Initial commit"
-
Link to Remote Repository: If you have a remote repository set up (e.g., on GitHub):
git remote add origin your-repo-url git push -u origin main
This setup ensures that your development environment is ready for building a multi-tenant SaaS platform. Laravel will handle your backend logic, database interactions, and API endpoints, while Next.js will manage the frontend, providing a seamless user experience with its advanced features like server-side rendering. Remember, version control with Git is essential for tracking changes, collaborating with team members, and maintaining the integrity of your codebase over time.
Step 2: Architecting Your Multi-Tenant System
When building a multi-tenant SaaS platform with Laravel and Next.js, architecting your system to handle multiple tenants effectively is crucial. Here's a detailed explanation of how to approach this step, leveraging Prateeksha Web Design's expertise:
Database Design
-
Separate Databases or Schemas: Each tenant can have its own database or schema within a shared database. This approach ensures data isolation:
-
Separate Databases: Each tenant gets its own database. This provides the highest level of data isolation but increases complexity in terms of management and scaling. Laravel's migration system can be used to create databases for each new tenant. Here's how you might set this up:
// In a custom Artisan command or service provider public function createTenantDatabase($tenantId) { $databaseName = 'tenant_' . $tenantId; DB::statement("CREATE DATABASE $databaseName"); // Run migrations for the new database $this->call('migrate', ['--database' => $databaseName]); }
-
Shared Database with Separate Schemas: Tenants share the same database but have their own schema. This method reduces the overhead of managing multiple databases but still provides good isolation:
// Creating a new schema for a tenant public function createTenantSchema($tenantId) { $schemaName = 'tenant_' . $tenantId; DB::statement("CREATE SCHEMA $schemaName"); // Set the schema for migrations DB::connection('tenant')->setSchema($schemaName); $this->call('migrate', ['--database' => 'tenant']); }
-
-
Tenant ID Column: If using a shared database approach, add a
tenant_id
column to all tables that need to be tenant-specific. This allows for easy data segregation:Schema::create('users', function (Blueprint $table) { $table->id(); $table->unsignedBigInteger('tenant_id'); // Other fields... });
User Authentication
-
Custom Authentication: Laravel's built-in authentication can be extended to handle multi-tenant scenarios:
-
Tenant-specific Authentication: Users should authenticate against their tenant's database or schema. This can be managed through middleware or service providers:
// Middleware to set the correct tenant context public function handle($request, Closure $next) { $tenant = Tenant::where('domain', $request->getHost())->first(); if ($tenant) { $request->merge(['tenant' => $tenant]); // Switch database connection or schema DB::connection('tenant')->setSchema($tenant->schema_name); } return $next($request); }
-
Role-Based Access Control (RBAC): Implement roles and permissions to control what users can do within their tenant's environment. Laravel packages like
spatie/laravel-permission
can be used for this.
-
Tenant Isolation
-
Middleware: Use middleware to ensure that each request is processed in the context of the correct tenant:
// In your route file or service provider Route::middleware(['auth', 'tenant'])->group(function () { // Tenant-specific routes });
-
Service Providers: Service providers can be used to set up tenant context at the application level:
// In your TenancyServiceProvider public function boot() { $this->app->bind('tenant', function () { // Logic to determine the current tenant return Tenant::where('domain', request()->getHost())->first(); }); }
Customization
-
UI Customization: Allow tenants to customize their UI through configurations:
- Theme Settings: Store theme preferences in the tenant's database or configuration files.
- Custom CSS: Provide a way for tenants to upload or define custom CSS.
-
Business Logic Customization:
-
Plugins or Modules: Develop a plugin system where tenants can enable or disable features or add custom logic. This could be managed through a marketplace or directly within the application:
// Example of enabling a plugin $tenant->plugins()->attach($pluginId);
-
Configuration Files: Use Laravel's configuration system to allow tenants to set business rules or parameters:
// In a tenant-specific config file 'invoice_due_days' => 30, 'currency' => 'USD',
-
By following these architectural principles, Prateeksha Web Design ensures that your multi-tenant SaaS platform is not only scalable and secure but also flexible enough to meet the unique needs of each tenant. This approach leverages Laravel's robust features while providing a seamless experience for both developers and end-users.
Step 3: Integrating Laravel with Next.js
Integrating Laravel with Next.js for a multi-tenant SaaS platform involves several key steps to ensure seamless communication between the backend and frontend. Here's a detailed explanation of how to achieve this integration:
API Development in Laravel
-
Resource Controllers: Laravel's resource controllers provide a structured way to handle CRUD operations:
-
Create a Resource Controller: Use Artisan to generate a resource controller:
php artisan make:controller UserController --resource
-
Define CRUD Methods: Implement methods like
index
,show
,store
,update
, anddestroy
in your controller to handle different HTTP requests:public function index() { return User::where('tenant_id', auth()->user()->tenant_id)->get(); } public function store(Request $request) { $user = new User($request->all()); $user->tenant_id = auth()->user()->tenant_id; $user->save(); return response()->json($user, 201); }
-
-
API Routes: Define API routes in
routes/api.php
to map to your controllers:Route::apiResource('users', UserController::class);
-
CORS: Enable Cross-Origin Resource Sharing (CORS) in Laravel to allow requests from your Next.js frontend:
// In config/cors.php 'paths' => ['api/*'], 'allowed_methods' => ['*'], 'allowed_origins' => ['*'], 'allowed_headers' => ['*'],
Next.js API Routes
-
Create API Routes: Next.js allows you to create API routes directly within your project:
-
Setup API Route: Create a file in the
pages/api
directory, e.g.,pages/api/users.js
:import axios from 'axios'; export default async function handler(req, res) { const { method } = req; const baseUrl = process.env.NEXT_PUBLIC_API_URL; // Set this in your .env file switch (method) { case 'GET': try { const response = await axios.get(`${baseUrl}/api/users`); res.status(200).json(response.data); } catch (error) { res.status(500).json({ error: 'Failed to fetch users' }); } break; // Implement other HTTP methods as needed } }
-
-
Environment Variables: Use environment variables to manage your API URL:
# In .env.local NEXT_PUBLIC_API_URL=http://localhost:8000
Authentication
-
JWT (JSON Web Tokens):
-
Laravel: Use a package like
tymon/jwt-auth
to generate JWT tokens:// In your Laravel controller public function login(Request $request) { $credentials = $request->only(['email', 'password']); if (!$token = auth()->attempt($credentials)) { return response()->json(['error' => 'Unauthorized'], 401); } return $this->respondWithToken($token); } protected function respondWithToken($token) { return response()->json([ 'access_token' => $token, 'token_type' => 'bearer', 'expires_in' => auth()->factory()->getTTL() * 60 ]); }
-
Next.js: Store the JWT token in local storage or cookies and include it in the headers of API requests:
// In your Next.js API route or component const token = localStorage.getItem('token'); const response = await axios.get(`${baseUrl}/api/users`, { headers: { Authorization: `Bearer ${token}` }, });
-
-
OAuth: If using OAuth, you'll need to set up OAuth providers in Laravel and handle the OAuth flow in Next.js, which involves redirecting users to the provider for authentication and then back to your application.
State Management
-
Redux or React Query: For managing state in Next.js:
-
Redux: Use Redux for complex state management:
// In your Next.js component import { useSelector, useDispatch } from 'react-redux'; import { fetchUsers } from '../store/actions/userActions'; const UsersList = () => { const dispatch = useDispatch(); const users = useSelector((state) => state.users); useEffect(() => { dispatch(fetchUsers()); }, [dispatch]); return ( <ul> {users.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> ); };
-
React Query: For simpler state management or when dealing with server-side data:
import { useQuery } from 'react-query'; const UsersList = () => { const { data: users, isLoading, error, } = useQuery('users', () => fetch(`${process.env.NEXT_PUBLIC_API_URL}/api/users`).then((res) => res.json() ) ); if (isLoading) return 'Loading...'; if (error) return 'An error has occurred: ' + error.message; return ( <ul> {users.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> ); };
-
By following these steps, you ensure that your Laravel backend and Next.js frontend are tightly integrated, providing a seamless experience for your multi-tenant SaaS platform. This setup allows for efficient data fetching, secure authentication, and robust state management, all while leveraging the strengths of both frameworks.
Step 4: Deployment and Scaling
Deploying and scaling a multi-tenant SaaS platform built with Laravel and Next.js involves several strategic steps to ensure your application can handle growth, maintain performance, and provide a seamless user experience. Here's a detailed explanation of each aspect:
Cloud Services
-
Choosing a Cloud Provider:
- AWS (Amazon Web Services): AWS offers a wide range of services like EC2 for virtual servers, RDS for managed databases, and Elastic Beanstalk for easy deployment of web applications. AWS also provides tools like AWS Elastic Container Service (ECS) or Kubernetes for container orchestration.
- Azure: Microsoft Azure provides similar services with Azure Virtual Machines, Azure SQL Database, and Azure App Service for hosting web applications. Azure Kubernetes Service (AKS) can be used for container management.
- DigitalOcean: Known for its simplicity and developer-friendly approach, DigitalOcean offers Droplets (VPS), Managed Databases, and Kubernetes clusters. It's particularly appealing for smaller to medium-sized applications due to its straightforward pricing and ease of use.
-
Containerization:
- Docker: Use Docker to containerize your Laravel application. This ensures consistency across different environments by packaging your application with all its dependencies into containers. Here's how you might proceed:
- Dockerfile: Create a Dockerfile for your Laravel app to define the environment, install dependencies, and set up the application.
- Docker Compose: Use Docker Compose to manage multi-container applications, defining services like PHP-FPM, Nginx, and MySQL in a
docker-compose.yml
file. - Deployment: Push your Docker images to a registry like Docker Hub or AWS ECR, then deploy these containers to your chosen cloud service. This approach allows for easy scaling and updates.
- Docker: Use Docker to containerize your Laravel application. This ensures consistency across different environments by packaging your application with all its dependencies into containers. Here's how you might proceed:
CDN for Next.js
-
Vercel:
- Vercel, created by the team behind Next.js, offers zero-configuration deployments, automatic image optimization, and a global CDN. Here's how to deploy:
- Vercel CLI: Install and use the Vercel CLI to deploy your Next.js app directly from your local machine or CI/CD pipeline.
- Automatic Scaling: Vercel automatically scales your application based on traffic, ensuring performance and availability.
- Benefits: Besides scaling, Vercel provides serverless functions, which can be used for dynamic content or API routes, enhancing the performance of your Next.js frontend.
- Vercel, created by the team behind Next.js, offers zero-configuration deployments, automatic image optimization, and a global CDN. Here's how to deploy:
-
Netlify:
- Netlify is another excellent choice for deploying static sites and serverless functions:
- Deployments: Push your Next.js project to a Git repository, and Netlify can automatically deploy on every push.
- CDN: Netlify's global CDN ensures fast content delivery worldwide.
- Serverless Functions: Similar to Vercel, Netlify supports serverless functions for handling dynamic content.
- Netlify is another excellent choice for deploying static sites and serverless functions:
Database Scaling
-
Sharding:
- What is Sharding?: Sharding involves dividing your database into smaller, more manageable parts called shards. Each shard contains a subset of the data, typically based on a shard key like
tenant_id
. - Benefits:
- Scalability: Allows for horizontal scaling by adding more shards as data grows.
- Performance: Distributes load across multiple database instances, reducing the load on any single database.
- Isolation: Enhances tenant isolation, reducing the impact of one tenant's operations on others.
- Implementation:
- Key-Based Sharding: Use a hash function on a key to distribute data evenly across shards.
- Range-Based Sharding: Data is partitioned based on ranges of a key, which can be useful for time-series data or sequential IDs.
- Directory-Based Sharding: Use a lookup table to map keys to shards, offering flexibility in data distribution.
- What is Sharding?: Sharding involves dividing your database into smaller, more manageable parts called shards. Each shard contains a subset of the data, typically based on a shard key like
-
Replication:
- What is Replication?: Replication involves creating copies of your database to increase read performance and provide fault tolerance.
- Benefits:
- Read Scalability: Multiple read replicas can handle read requests, improving performance.
- High Availability: If one database instance fails, others can take over, minimizing downtime.
- Implementation:
- Read Replicas: Set up read replicas in your cloud service to distribute read operations.
- Multi-AZ Deployments: Use services like AWS RDS Multi-AZ for automatic failover and high availability.
Monitoring and Logging
-
New Relic:
- Features: Provides comprehensive monitoring including APM (Application Performance Monitoring), infrastructure monitoring, and real user monitoring (RUM).
- Error Monitoring: New Relic's Errors Inbox helps in tracking and managing errors across your application stack.
- Integration: Easily integrates with Laravel and Next.js through agents or SDKs, offering detailed insights into performance metrics, errors, and user interactions.
-
Sentry:
- Error Tracking: Sentry excels in real-time error detection, providing detailed error reports, stack traces, and the ability to track and manage errors as they occur.
- Performance Monitoring: Offers insights into performance issues, allowing you to trace slow transactions to specific API calls or database queries.
- Custom Alerts: Set up custom alerts for errors or performance issues, integrating with tools like Slack or Jira for team notifications.
By implementing these strategies, you ensure that your multi-tenant SaaS platform can scale efficiently, maintain high performance, and provide robust monitoring and error handling, all of which are critical for a growing application.
Real-World Examples and Prateeksha Web Design's Role
Prateeksha Web Design has been at the forefront of creating scalable, secure, and user-friendly SaaS platforms:
-
Case Studies: They've built platforms like Invoice Ninja for invoicing, Surf for subscription management, and many more, showcasing their prowess in Laravel and Next.js integration.
-
Expertise: Their team's deep understanding of multi-tenancy ensures that each tenant's data is secure and isolated, while still benefiting from shared infrastructure.
-
Custom Solutions: Whether it's a complex e-commerce platform or a simple SaaS tool, Prateeksha tailors solutions to fit the unique needs of each business.
Wrapping Up
Building a multi-tenant SaaS platform with Laravel and Next.js is not just about coding; it's about crafting an ecosystem where businesses can thrive. With Laravel's robust backend capabilities and Next.js's dynamic frontend, you're setting up for success. And with Prateeksha Web Design by your side, you're not just building software; you're creating a scalable, secure, and efficient business solution that can grow with your ambitions.
So, are you ready to start your SaaS journey? With the right tools, knowledge, and a bit of creativity, there's no limit to what you can achieve. Let's make your SaaS platform the next big thing!
About Prateeksha Web Design
Prateeksha Web Design specializes in developing robust multi-tenant SaaS platforms using Laravel and Next.js. Their services encompass custom architecture solutions, seamless user experience design, and scalable backend infrastructures. They prioritize security and performance, ensuring optimal resource management for multiple tenants. With expertise in API integration and real-time data handling, Prateeksha delivers efficient solutions tailored to client needs. Elevate your SaaS offerings with their innovative design and development strategies.
Interested in learning more? Contact us today.
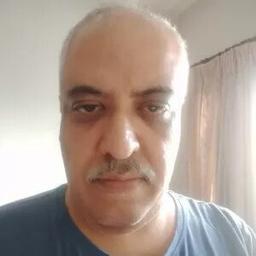