Authentication is the backbone of secure web applications, especially for developers using cutting-edge frameworks like Next.js. If you're a budding programmer or a small business owner curious about implementing robust Next.js JWT authentication, this blog is your comprehensive guide. With a casual yet professional tone, we'll explain complex concepts in an accessible way, aligning with EEAT (Expertise, Authoritativeness, and Trustworthiness) principles. Let’s dive in.
What is Authentication in Next.js? (Detailed Explanation)
Authentication is the process of confirming the identity of a user trying to access an application. Think of it as a digital ID check—just like showing your driver’s license to confirm your identity before entering a secured facility. In web development, this ensures that only the right people can access certain parts of your app, like admin dashboards or personalized user profiles.
Next.js simplifies this process through its powerful server-side rendering (SSR) capabilities and API routes. This React-based framework is designed to handle modern web applications efficiently and securely, making it an excellent choice for integrating authentication systems. By leveraging Next.js, you can manage authentication both on the client side (browser) and server side (API or middleware), ensuring a smooth and secure user experience.
Authentication Workflows in Next.js
There are several ways to implement authentication in Next.js:
-
Session-Based Authentication: Stores user sessions on the server and references them with cookies. While this is secure, it requires server-side storage, making it less ideal for highly scalable applications.
-
Token-Based Authentication (JWT): Uses stateless tokens like JSON Web Tokens (JWTs) to authenticate users. Since the token contains all the necessary user data, the server doesn’t need to store user state, making it lightweight and scalable.
-
Third-Party OAuth Providers: Integrations with providers like Google, Facebook, or GitHub allow users to log in without creating a new account. This is handled effectively with libraries like NextAuth.js.
In this guide, we focus on JWT (JSON Web Token) authentication because of its scalability, flexibility, and compatibility with modern applications.
Understanding JSON Web Tokens (JWT) in Detail
A JSON Web Token (JWT) is like a digital passport. It’s a small, secure package of information that verifies a user’s identity and contains their data in a compact, standardized format. The token is signed cryptographically, ensuring that it cannot be tampered with.
Structure of a JWT
A JWT consists of three parts, separated by dots (.
):
-
Header: This part specifies metadata about the token:
- Type of Token: For JWTs, this is always
JWT
. - Signing Algorithm: Indicates the cryptographic algorithm used to sign the token (e.g., HMAC SHA256).
Example:
{ "alg": "HS256", "typ": "JWT" }
- Type of Token: For JWTs, this is always
-
Payload: This is where the actual data (or claims) about the user resides. Claims are statements like:
- Issuer (iss): Identifies the entity issuing the token.
- Subject (sub): Specifies the user or entity the token refers to.
- Expiration Time (exp): Defines when the token expires.
- Custom Claims: Application-specific data like user roles, permissions, or preferences.
Example:
{ "sub": "1234567890", "name": "John Doe", "admin": true }
-
Signature: This ensures the token hasn’t been altered. It’s generated by encoding the header and payload, then signing them using a secret key (or private key in asymmetric encryption).
Example process:
HMACSHA256( base64UrlEncode(header) + "." + base64UrlEncode(payload), secret )
Advantages of JWT Authentication in Next.js
1. Scalability
Traditional session-based authentication requires storing user data on the server, often in a database or cache like Redis. This approach can become cumbersome as the application grows because the server needs to manage more sessions.
With JWT, the server does not store any session information. Once issued, the token is self-contained and can be validated on any server without additional lookups, making it perfect for distributed and microservice-based architectures.
2. Security
JWTs are cryptographically signed. If someone tries to tamper with the token’s data, the signature will not match, and the server will reject it. Additionally:
- Expiration times ensure that even if a token is stolen, it can only be used for a limited time.
- Custom claims allow fine-grained access control, such as defining roles (e.g., admin, editor, viewer).
3. Flexibility
JWTs are widely supported across APIs, microservices, and mobile apps. They’re sent in the HTTP Authorization
header, making them ideal for RESTful APIs and GraphQL backends.
Additionally, JWTs can be used for both authentication (verifying the user) and authorization (checking if the user has the required permissions). This dual functionality simplifies the workflow in complex applications.
How JWT Works in Next.js Applications
1. User Login
When a user logs in, their credentials are validated (e.g., comparing their password with the hashed password stored in the database). If successful, a JWT is generated and sent to the client.
2. Token Storage
The client stores the token, usually in localStorage or cookies:
- localStorage: Convenient for storing tokens, but prone to cross-site scripting (XSS) attacks.
- HttpOnly Cookies: More secure because they’re not accessible via JavaScript, mitigating XSS vulnerabilities.
3. Authenticated Requests
The client sends the JWT with every request to protected routes or APIs. This is typically done by adding the token to the HTTP Authorization
header as a Bearer Token:
Authorization: Bearer <your_jwt_token>
4. Token Verification
On the server, the token is validated by decoding it and verifying its signature using a secret key. If valid, the server grants access; otherwise, it rejects the request.
Why Use JWT with Next.js?
Scalability
The stateless nature of JWT allows Next.js applications to scale seamlessly. Since the server doesn’t need to maintain session data, horizontal scaling (adding more servers) becomes straightforward.
Security
JWT’s cryptographic signing ensures tamper-proof tokens. Combined with Next.js’s ability to handle server-side rendering (SSR) and middleware, you can implement robust access control for your application.
Flexibility
Whether you're building a single-page app, a microservices architecture, or even integrating with third-party APIs, JWT fits the bill. Its compatibility with Next.js API routes makes it easy to manage authentication workflows.
Next.js and JWT form a perfect combination for developers looking to build modern, secure, and scalable applications. By leveraging the strengths of both, you can deliver seamless authentication workflows that keep your application efficient and secure.
Prateeksha Web Design can help small businesses implement such robust solutions, ensuring that your application remains secure while providing a stellar user experience. Let us help you secure your web presence with Next.js!
Setting Up a Secure Next.js JWT Authentication Workflow
To establish Program Geek login setup with secure Next.js JWT authentication, follow these detailed steps:
1. Create a New Next.js Project
Start by initializing a Next.js application. If you haven’t installed Next.js, do so first:
npx create-next-app@latest jwt-auth-tutorial
cd jwt-auth-tutorial
After setup, run the development server:
npm run dev
2. Install Required Dependencies
Authentication involves encryption and secure token handling. Install these libraries:
npm install jsonwebtoken bcryptjs next-auth
- jsonwebtoken: To create and verify JWTs.
- bcryptjs: To hash passwords securely.
- next-auth: Optional, for integrating with OAuth providers.
3. Build a User Model
For simplicity, we’ll use a basic in-memory database. For production, connect to a database like MongoDB, PostgreSQL, or Firebase.
Create a file data/users.js
:
export const users = [];
This placeholder will store registered users. Replace it with your database logic later.
4. Create API Routes
Next.js provides API routes to handle backend logic directly within the framework. Create the following routes:
a. Register Route
Create pages/api/auth/register.js
:
import bcrypt from "bcryptjs";
export default async function handler(req, res) {
if (req.method !== "POST") return res.status(405).end();
const { username, password } = req.body;
const hashedPassword = await bcrypt.hash(password, 10);
users.push({ username, password: hashedPassword });
res.status(201).json({ message: "User registered successfully!" });
}
This route hashes the password and saves the user data securely.
b. Login Route
Create pages/api/auth/login.js
:
import jwt from "jsonwebtoken";
import bcrypt from "bcryptjs";
const SECRET_KEY = process.env.JWT_SECRET || "your-secret-key";
export default async function handler(req, res) {
if (req.method !== "POST") return res.status(405).end();
const { username, password } = req.body;
const user = users.find((u) => u.username === username);
if (!user) return res.status(404).json({ error: "User not found" });
const isValidPassword = await bcrypt.compare(password, user.password);
if (!isValidPassword) return res.status(401).json({ error: "Invalid credentials" });
const token = jwt.sign({ username }, SECRET_KEY, { expiresIn: "1h" });
res.status(200).json({ token });
}
This route validates user credentials and generates a JWT upon successful login.
c. Protected Route
Create pages/api/auth/protected.js
:
import jwt from "jsonwebtoken";
const SECRET_KEY = process.env.JWT_SECRET || "your-secret-key";
export default function handler(req, res) {
const { authorization } = req.headers;
if (!authorization) return res.status(401).json({ error: "Unauthorized" });
const token = authorization.split(" ")[1];
try {
const decoded = jwt.verify(token, SECRET_KEY);
res.status(200).json({ message: "Access granted", user: decoded });
} catch (error) {
res.status(401).json({ error: "Invalid token" });
}
}
This route verifies the JWT and grants access to authorized users.
5. Secure Your Application
- Environment Variables: Store your JWT_SECRET securely in
.env.local
. - HTTPS: Use SSL/TLS for encrypted communication.
- Token Expiry: Limit token lifespan to mitigate risks.
Integrating Frontend Authentication in Next.js
Authentication isn’t complete without a proper user interface. Follow these steps:
1. Create a Registration Form
In pages/register.js
:
import { useState } from "react";
export default function Register() {
const [username, setUsername] = useState("");
const [password, setPassword] = useState("");
const handleSubmit = async (e) => {
e.preventDefault();
await fetch("/api/auth/register", {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({ username, password }),
});
alert("User registered!");
};
return (
<form onSubmit={handleSubmit}>
<input placeholder="Username" onChange={(e) => setUsername(e.target.value)} />
<input type="password" placeholder="Password" onChange={(e) => setPassword(e.target.value)} />
<button type="submit">Register</button>
</form>
);
}
2. Create a Login Form
Similarly, create pages/login.js
:
import { useState } from "react";
export default function Login() {
const [username, setUsername] = useState("");
const [password, setPassword] = useState("");
const handleSubmit = async (e) => {
e.preventDefault();
const res = await fetch("/api/auth/login", {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({ username, password }),
});
const data = await res.json();
localStorage.setItem("token", data.token);
alert("Login successful!");
};
return (
<form onSubmit={handleSubmit}>
<input placeholder="Username" onChange={(e) => setUsername(e.target.value)} />
<input type="password" placeholder="Password" onChange={(e) => setPassword(e.target.value)} />
<button type="submit">Login</button>
</form>
);
}
1. Middleware for Authentication
Introduced in Next.js 12, middleware is a powerful feature that allows developers to run custom logic at the edge before a request is completed. It acts as a gateway for incoming requests, providing a seamless way to handle authentication dynamically.
How Middleware Enhances Authentication
- Dynamic Token Validation: Middleware can intercept requests to protected routes and verify JWTs or other authentication tokens in real time. For example:
import { NextResponse } from 'next/server'; export function middleware(req) { const token = req.cookies.authToken; // Example: Reading the JWT from cookies if (!token) { return NextResponse.redirect(new URL('/login', req.url)); // Redirect to login if no token } try { const isValid = verifyToken(token); // Custom function to validate the token if (!isValid) throw new Error('Invalid token'); } catch (error) { return NextResponse.redirect(new URL('/login', req.url)); // Redirect on invalid token } }
- Centralized Logic: Middleware allows authentication checks to be centralized, reducing redundant code in individual route handlers.
- Edge Runtime: Middleware runs at the edge (closer to the user), which speeds up requests and reduces server load.
Key Benefits
- Performance: Running logic at the edge reduces the latency of round trips to the server.
- Flexibility: Middleware can be tailored to handle complex authentication workflows, like token refreshing or role-based access control (RBAC).
- Ease of Use: Simplifies the implementation of global authentication checks without modifying every route.
2. Edge Functions
Edge functions are a revolutionary feature that takes server-side computation closer to the end user by running it on edge servers worldwide. This approach minimizes latency and enhances the overall performance of authentication workflows.
Authentication at the Edge
- Token Validation: Just like middleware, edge functions can validate JWTs. However, edge functions add the ability to handle more complex logic with better scalability.
- Access Control: Perform role-based checks and redirect unauthorized users without waiting for server-side processing.
How Edge Functions Improve Authentication
- Speed: With edge functions, authentication happens near the user, leading to faster responses and an improved user experience.
- Server Offloading: By moving authentication processing to the edge, the central server is freed up to handle other tasks, improving overall efficiency.
Example Use Case
For an international e-commerce application, edge functions can validate user tokens and serve region-specific content based on authentication claims (e.g., user roles or permissions).
3. Enhanced Security
Security is paramount in any authentication system, and Next.js continues to push boundaries with enhanced security measures and tools.
next-safe for Automatic Security Headers
The next-safe library simplifies implementing best-practice security headers in Next.js applications, reducing the risk of attacks like cross-site scripting (XSS) and clickjacking.
Key Features of next-safe
- Content Security Policy (CSP): Prevents malicious scripts from running in your application.
- X-Frame-Options: Mitigates clickjacking attacks by preventing your application from being embedded in iframes.
- Strict-Transport-Security (HSTS): Ensures your app is always accessed over HTTPS.
Secure Cookie Handling
With features like HttpOnly and Secure cookies, Next.js applications can ensure that tokens are stored safely and not accessible via client-side JavaScript.
Serverless Security Enhancements
Next.js integrates seamlessly with modern serverless platforms like Vercel and AWS Lambda, offering built-in security for handling sensitive user data.
Authentication Libraries and Frameworks
- NextAuth.js: Adds OAuth and session-based authentication with minimal configuration.
- Passport.js: Provides extensive strategies for different authentication methods.
- jsonwebtoken: Handles token generation and verification securely.
Why Choose Prateeksha Web Design for Next.js Projects?
When building applications that demand modern, secure, and scalable solutions, Prateeksha Web Design stands out as a trusted partner. Here’s how we can help:
Expert Knowledge in Next.js JWT Authentication
Our team has hands-on experience implementing cutting-edge authentication systems using Next.js and JWT. From setting up robust APIs to integrating third-party OAuth providers, we ensure that your application’s authentication layer is secure and efficient.
Reliable Implementation Tailored to Your Needs
Every project is unique. Whether you're a small business looking to protect sensitive customer data or a tech company building a multi-tenant platform, we design solutions that align perfectly with your goals.
Ongoing Support and Security Updates
Authentication is not a one-time setup. Our team provides ongoing support to keep your application secure, update dependencies, and adapt to new security threats.
Showcasing Authority and Trust
We bring a track record of delivering high-quality solutions that establish trust with your users. From implementing best practices in security to optimizing for performance, we ensure that your application meets the highest standards.
Conclusion
Implementing Next.js JWT authentication may seem daunting, but with the right approach, you can build secure and scalable applications. By following this guide, you’ve taken the first step towards mastering authentication workflows.
Looking for a trusted partner to build your Next.js project? Prateeksha Web Design combines expertise and creativity to bring your ideas to life. Reach out today and let us handle your web development needs!
About Prateeksha Web Design
Prateeksha Web Design offers expert services in implementing Next.js Authentication with JWT, providing a comprehensive tutorial for program geeks. Their services include setting up secure user authentication, integrating JWT tokens for secure data transmission, implementing login and registration functionalities, and handling user sessions seamlessly. With their expertise in Next.js and JWT authentication, Prateeksha Web Design ensures robust security measures for web applications.
Interested in learning more? Contact us today.
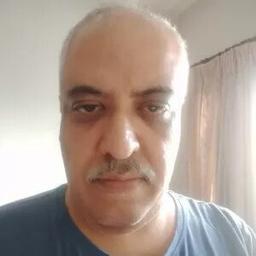