If you’re diving into ReactJS with Redux or wondering whether you should use React Hooks for state management instead, you’re in the right place. Choosing between React Redux and modern alternatives can feel like picking between a high-speed train (efficient but structured) and a sports car (agile but needs more handling).
By 2025, state management has evolved significantly, with Redux Toolkit, React Query, and newer players like Zustand gaining traction. So, should you stick with the tried-and-true Redux.js, or is it time to fully embrace React Hooks? Let’s break it down in a fun yet informative way.
1. What’s the Big Deal About State Management?
Imagine you’re planning a big family reunion. You’ve got different groups—parents, kids, cousins, grandparents—all needing different things. Some need updates about food, others about activities, and a few just want to know where the bathroom is. If everyone had to constantly ask you for information, it would be chaos.
Now, imagine if you had a centralized system—a group chat or a big whiteboard with all the necessary info. Suddenly, everyone stays informed without constantly checking in with you. That’s state management in a React app.
In React, your app consists of multiple components, each needing to share and update data efficiently. Without proper state management, things get messy, leading to unnecessary re-renders, inconsistent data, and debugging nightmares.
What is State in React?
State is the dynamic data of your application. It determines how your UI should look at any given moment. For example:
- If a user logs in, the state updates to show a different UI.
- When an item is added to a cart, the total price updates.
- Clicking dark mode switches the theme across the app.
In simple apps, managing state with React’s built-in features (useState
, useEffect
) is enough. But as your app grows, managing state across multiple components becomes complex. That’s where state management solutions like Redux, Context API, and Zustand come in.
Different Types of State in React Apps
Not all state is created equal! Let’s break it down:
1. Local State (Component-Level State)
👉 Best Managed With: React Hooks (useState
, useReducer
)
Local state is specific to a single component and doesn’t need to be shared.
📌 Example:
- A dropdown menu opening and closing (
useState
). - A form input value changing (
useState
). - A counter in a component (
useReducer
for more control).
2. Global State (Application-Wide State)
👉 Best Managed With: Redux, Context API, or Zustand
Global state affects multiple components and needs to be shared across the app.
📌 Example:
- User authentication (knowing if a user is logged in).
- Shopping cart items (multiple pages need access).
- Theme settings (dark mode toggled across the app).
3. Server State (Fetched from APIs)
👉 Best Managed With: React Query, SWR, or Redux Toolkit with RTK Query
This state comes from an external API and is updated asynchronously.
📌 Example:
- Fetching user profile data from a backend.
- Loading posts, comments, or products from an API.
4. UI State (Temporary UI Behavior)
👉 Best Managed With: React Hooks (useState
)
UI state determines small, temporary UI interactions.
📌 Example:
- Whether a modal is open or closed.
- A loading spinner appearing while an action is in progress.
2. React Hooks – The Lightweight Contender
When React Hooks arrived, they completely changed the way developers managed state in React applications. Before Hooks, we had to use class components and deal with this.setState()
, lifecycle methods (componentDidMount
, componentDidUpdate
), and prop drilling nightmares.
With React Hooks, state management became simpler, more readable, and more flexible—all within functional components.
How Hooks Help in State Management
Hooks allow you to manage state efficiently without external libraries like Redux. Here are the key hooks that help in state management:
1. useState
– The Simple State Manager
The useState
hook is the easiest way to manage local state. It’s perfect for small, component-specific data that doesn’t need to be shared across components.
📌 Example – Toggling a Modal
import { useState } from "react";
function ModalComponent() {
const [isOpen, setIsOpen] = useState(false);
return (
<div>
<button onClick={() => setIsOpen(!isOpen)}>
{isOpen ? "Close Modal" : "Open Modal"}
</button>
{isOpen && <div className="modal">This is a modal!</div>}
</div>
);
}
When to Use useState
?
✅ Managing form inputs, checkboxes, and UI elements.
✅ Tracking boolean states like open/closed, true/false.
✅ Handling counters or basic numerical state.
2. useReducer
– A Mini-Redux Alternative
While useState
is great for simple state updates, useReducer
is more powerful for complex state logic where actions determine the state. It’s like a lightweight Redux reducer but for a single component.
📌 Example – Using useReducer
for a Counter App
import { useReducer } from "react";
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case "increment":
return { count: state.count + 1 };
case "decrement":
return { count: state.count - 1 };
case "reset":
return initialState;
default:
return state;
}
}
function CounterComponent() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: "increment" })}>+</button>
<button onClick={() => dispatch({ type: "decrement" })}>-</button>
<button onClick={() => dispatch({ type: "reset" })}>Reset</button>
</div>
);
}
When to Use useReducer
?
✅ When state transitions follow complex logic (e.g., undo/redo functionality).
✅ When managing state objects instead of simple values.
✅ If you find yourself writing too many setState
functions inside useState
.
3. useContext
– Global State Without Redux?
The useContext
hook provides a simple way to share state across components without prop drilling. It’s a great alternative to Redux for small-scale global state management.
📌 Example – Using useContext
for Theme Mode
import { createContext, useContext, useState } from "react";
const ThemeContext = createContext();
function ThemeProvider({ children }) {
const [theme, setTheme] = useState("light");
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
}
function ThemedComponent() {
const { theme, setTheme } = useContext(ThemeContext);
return (
<div className={theme}>
<p>Current Theme: {theme}</p>
<button onClick={() => setTheme(theme === "light" ? "dark" : "light")}>
Toggle Theme
</button>
</div>
);
}
export default function App() {
return (
<ThemeProvider>
<ThemedComponent />
</ThemeProvider>
);
}
When to Use useContext
?
✅ For lightweight global state, like theme or authentication status.
✅ To avoid prop drilling when passing data deeply into components.
❌ Not ideal for frequently changing states (it causes unnecessary re-renders).
4. useEffect
– Handling Side Effects
In real-world applications, state isn’t just about managing data—it’s also about handling side effects like:
- Fetching data from an API.
- Subscribing to real-time events.
- Updating the DOM.
📌 Example – Fetching API Data with useEffect
import { useState, useEffect } from "react";
function FetchDataComponent() {
const [data, setData] = useState([]);
useEffect(() => {
fetch("https://api.example.com/data")
.then(response => response.json())
.then(json => setData(json));
}, []); // Empty array ensures this runs only once
return <div>{data.map(item => <p key={item.id}>{item.name}</p>)}</div>;
}
When to Use useEffect
?
✅ Fetching API data, handling subscriptions, or updating external states.
✅ Synchronizing components with external sources (e.g., localStorage, Firebase, WebSockets).
❌ Don’t use it for managing state logic (that’s what useState
and useReducer
are for).
3. React Redux – The Powerhouse for Global State
If React Hooks are the nimble, easy-to-use tools of the React ecosystem, then Redux is the Swiss Army knife—powerful, structured, and built for handling complex global state.
But here’s the catch—Redux isn’t always necessary. While it’s a go-to solution for large-scale applications, it comes with some baggage: boilerplate code, steep learning curves, and unnecessary complexity if overused.
That’s why Redux Toolkit in ReactJS has stepped in to simplify Redux and make it developer-friendly in 2025. Let’s break down how Redux works and when to use it.
How Redux Works (The Core Concepts)
Unlike useState
or useReducer
, Redux centralizes your entire application’s state in a single store. This makes data flow predictable, especially when dealing with a large number of components.
Here’s a simple breakdown of Redux’s key building blocks:
1. Redux Store – The Central Data Hub
Think of the Redux Store as a global database that holds the entire state of your application. Any component that needs data must subscribe to this store using useSelector
.
2. Redux Actions – The State Changers
Actions are simple objects that describe what should happen in the app. They don’t directly change the state but send instructions to reducers.
📌 Example: Action for Adding a Product to Cart
const ADD_TO_CART = "ADD_TO_CART";
const addToCart = (product) => ({
type: ADD_TO_CART,
payload: product,
});
This action tells the reducer that a new product should be added to the cart.
3. Redux Reducers – The State Updaters
Reducers listen to actions and update the state accordingly. They take the current state and the action, then return a new version of the state.
📌 Example: Cart Reducer
const initialState = {
cart: [],
};
const cartReducer = (state = initialState, action) => {
switch (action.type) {
case "ADD_TO_CART":
return { ...state, cart: [...state.cart, action.payload] };
default:
return state;
}
};
The reducer ensures that when ADD_TO_CART
is dispatched, the new product gets added without mutating the original state.
4. useSelector – Accessing the Store in Components
The useSelector
hook subscribes to the Redux store and retrieves state for a specific component.
📌 Example: Displaying Cart Items
import { useSelector } from "react-redux";
const CartComponent = () => {
const cart = useSelector((state) => state.cart);
return (
<div>
<h2>Shopping Cart</h2>
{cart.map((item, index) => (
<p key={index}>{item.name}</p>
))}
</div>
);
};
No prop drilling needed! Any change in the Redux store will automatically re-render components using useSelector
.
5. useDispatch – Sending Actions to Update State
The useDispatch
hook allows components to trigger Redux actions, updating the state in the store.
📌 Example: Adding an Item to the Cart
import { useDispatch } from "react-redux";
import { addToCart } from "../redux/actions";
const ProductComponent = ({ product }) => {
const dispatch = useDispatch();
return (
<div>
<h3>{product.name}</h3>
<button onClick={() => dispatch(addToCart(product))}>Add to Cart</button>
</div>
);
};
When the button is clicked, the addToCart
action is dispatched, updating the Redux store and automatically re-rendering the cart component.
When Should You Use Redux?
While Redux is powerful, it’s not always necessary. Here’s when Redux makes sense:
✅ Your app has a lot of shared/global state (e.g., user authentication, shopping cart, dark mode settings).
✅ You need a structured, predictable way to handle state updates.
✅ You require middleware support, such as Redux Saga or Redux Thunk (useful for API requests and async operations).
✅ You want state persistence across sessions (e.g., using Redux with localStorage).
🚨 But avoid Redux if your state is local to a single component. It’s overkill for small projects.
4. Redux vs Context API – Which One Should You Use?
A common debate among React developers is: Why not just use Context API instead of Redux?
Both Redux and Context API allow you to manage global state, but they work differently. Let’s compare:
Feature | Redux | Context API |
---|---|---|
Use Case | Large-scale apps with complex state | Small to medium apps with simple state |
Performance | Optimized with useSelector | Causes unnecessary re-renders |
Boilerplate | Requires actions, reducers, store | Minimal setup required |
Middleware Support | Supports Redux Saga, Thunk | No built-in middleware |
Best for | Shared, frequently updated data (e.g., authentication, cart, notifications) | Rarely changing global state (e.g., theme, language settings) |
When Should You Use Context API Instead of Redux?
✅ For small projects where you only need global state in a few places.
✅ For rarely changing global states like dark mode settings or user preferences.
✅ To avoid Redux’s boilerplate and learning curve.
🚨 But avoid Context API for frequently updated global state! If a value inside the Context changes often, it can cause unnecessary re-renders across the entire component tree.
The Ideal Approach in 2025: Combine Both!
In 2025, a common best practice is to combine Context API and Redux.
- Use Context API for rarely changing global states (like themes).
- Use Redux for high-frequency state updates (like shopping carts, notifications, and API calls).
5. Redux vs Zustand vs React Query – The 2025 Alternatives
State management is evolving, and newer alternatives to Redux.js are gaining traction:
🔹 Redux vs Zustand
- Zustand is a minimalistic state management library that reduces boilerplate code.
- Unlike Redux, Zustand doesn’t require actions and reducers—just pure functions.
- It’s faster and easier to implement.
👉 Use Zustand if you want a simple, lightweight alternative to Redux.
🔹 React Query vs Redux
- React Query is best for server-state management (e.g., API calls, caching).
- It automatically syncs with the server, making it more efficient than Redux for handling async data.
- If your state is mostly fetched from an API, React Query is the way to go.
👉 Use React Query if your app is data-heavy and relies on frequent API calls.
6. Redux Toolkit – Making Redux Less Painful
For years, developers have had a love-hate relationship with Redux. It was powerful, but the boilerplate code was overwhelming. Writing actions, reducers, and store setup separately made Redux feel like an overly complicated solution for state management.
Enter Redux Toolkit—the modern way to use Redux without the headaches. In 2025, Redux Toolkit (RTK) is the recommended way to use Redux because it eliminates unnecessary complexity while keeping the power of Redux’s centralized state management.
Why Redux Toolkit?
Redux Toolkit in ReactJS simplifies Redux in three key ways:
1. createSlice
– Fewer Files, Less Code
Instead of writing separate action types, action creators, and reducers, you can now define everything in a single file using createSlice
.
📌 Example: Managing a Counter with createSlice
import { createSlice } from "@reduxjs/toolkit";
const counterSlice = createSlice({
name: "counter",
initialState: { value: 0 },
reducers: {
increment: (state) => { state.value += 1; },
decrement: (state) => { state.value -= 1; },
reset: (state) => { state.value = 0; },
},
});
export const { increment, decrement, reset } = counterSlice.actions;
export default counterSlice.reducer;
👉 No more writing action types, action creators, and reducers separately!
2. configureStore
– Simplified Store Setup
With traditional Redux, setting up the store required manual configuration, applying middleware, and composing enhancers.
With Redux Toolkit, the configureStore
function automates these tasks while keeping everything structured.
📌 Example: Setting Up Redux Store
import { configureStore } from "@reduxjs/toolkit";
import counterReducer from "./counterSlice";
const store = configureStore({
reducer: {
counter: counterReducer,
},
});
export default store;
👉 It automatically enables the Redux DevTools extension and applies middleware like redux-thunk
by default.
3. Built-in Immer for Immutable Updates
Redux requires immutability, meaning you can’t modify state directly. Traditional Redux used spread operators (...state
) to update state, which could get messy.
With Immer (built into Redux Toolkit), you can write mutable-looking code that’s actually immutable.
📌 Example: Updating State with Immer
reducers: {
increment: (state) => { state.value += 1; }, // Looks mutable but is immutable under the hood!
}
👉 No need for return { ...state, value: state.value + 1 }
anymore!
7. When NOT to Use Redux
Despite all its power, Redux isn’t always the best choice. You should avoid using Redux if:
❌ Your app is small and doesn’t require global state.
❌ You don’t want to manage boilerplate code (even with Redux Toolkit, it still exists).
❌ You’re mainly dealing with API requests (consider React Query instead).
❌ You don’t want the extra memory Redux requires for state persistence.
Alternatives to Redux:
- React Hooks (
useState
,useReducer
) – For local component state. - Context API – For small global state (themes, authentication).
- Zustand – A minimal, lightweight Redux alternative.
- React Query – Best for fetching and caching API data.
8. Final Verdict – React Hooks vs Redux in 2025
So, which state management solution should you choose in 2025? The answer depends on your project size and requirements.
Use Case | Best Choice |
---|---|
Small app, minimal state | React Hooks (useState , useReducer ) |
Large app, complex global state | Redux Toolkit |
Frequent API calls, caching | React Query |
Simple global state (e.g., themes, auth status) | Context API |
Redux alternative with minimal setup | Zustand |
TL;DR:
🚀 React Hooks (useState
, useReducer
) – Best for local state management.
🚀 Redux Toolkit – Best for scaling large applications with structured state management.
🚀 React Query – Best for handling API data fetching and caching.
🚀 Zustand – A lightweight alternative to Redux for global state.
When Should You Stick with Redux Toolkit?
✅ If you need strict structure and predictable state updates.
✅ If your app has multiple global state dependencies (e.g., user authentication, shopping cart).
✅ If you need middleware like Redux Saga for complex async operations.
✅ If your app requires state persistence across sessions.
9. Need Help with Your ReactJS Project?
Whether you need help setting up React Redux, integrating Redux Toolkit, or deciding between Redux vs Context API, we’ve got you covered!
At Prateeksha Web Design, we specialize in:
🔥 Building scalable React & Next.js applications
💡 E-commerce website development in Mumbai
🚀 Modern web design tailored for high performance
Looking for the best web design company in Mumbai?
We create stunning, high-performing React Redux apps with the latest technologies.
Final Thought:
Choosing between React Hooks vs Redux depends on your app’s needs. If you value simplicity, go with Hooks. If you need scalability and structure, go with Redux Toolkit. And if you need expert guidance, Prateeksha Web Design is here to help! 🚀
About Prateeksha Web Design
Prateeksha Web Design offers specialized services in 2025 that focus on optimizing state management in React applications using Hooks and Redux. Their team provides comprehensive comparisons and tailored solutions, ensuring clients leverage the most effective approach for their projects. By emphasizing performance, scalability, and simplicity, they help businesses choose the right tool for state management. Additionally, Prateeksha's workshops and resources empower developers to enhance their skills in modern React practices. Ultimately, they aim to streamline user experiences and improve application efficiency.
Interested in learning more? Contact us today.
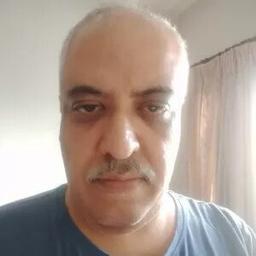