How to Use Next.js Incremental Static Regeneration (ISR) Effectively
Introduction to Incremental Static Regeneration (ISR)
Next.js is a powerful React framework widely embraced for its dynamic capabilities in building modern web applications. One of its standout features is Incremental Static Regeneration (ISR), which combines the benefits of static site generation (fast loading speeds) and server-side rendering (dynamic content updates). ISR allows developers to update static content without rebuilding the entire site, ensuring both speed and freshness.
For small businesses looking to optimize their online presence, particularly those with e-commerce platforms or frequently updated blogs, ISR can be a game-changer. At Prateeksha Web Design, we specialize in building Next.js-powered websites that leverage ISR to maximize performance and user experience.
What is Incremental Static Regeneration (ISR)?
Incremental Static Regeneration (ISR) is one of the most exciting features of Next.js, designed to balance the speed of static content delivery with the flexibility of dynamic updates. To truly understand ISR, let’s break it down by first understanding the two foundational concepts it builds upon: Static Site Generation (SSG) and Server-Side Rendering (SSR).
Static Site Generation (SSG)
SSG is the process of pre-building your website's pages during the build process. These pages are then stored as static files and served to users directly from a content delivery network (CDN) or web server. Here's why SSG is a popular choice:
-
Performance:
- Because pages are pre-built, the server doesn’t need to process any requests to generate the content. This results in lightning-fast load times for users.
-
Scalability:
- Static files are easy to cache and serve globally via CDNs, which means your site can handle large amounts of traffic without additional server load.
-
SEO Benefits:
- Pre-built HTML ensures that search engines can crawl and index your content more effectively.
However, SSG has a significant limitation: content cannot be updated dynamically without rebuilding the entire website. For example, if you add a new blog post, you must rebuild the entire site for the new post to appear.
Server-Side Rendering (SSR)
SSR generates your web pages on the server at request time, meaning the server fetches the necessary data and builds the page every time a user accesses it. This approach enables dynamic content updates, making it ideal for applications where data changes frequently.
Benefits of SSR include:
-
Dynamic Updates:
- The server retrieves the most up-to-date data for each request, ensuring the user always sees fresh content.
-
SEO-Friendly:
- Since pages are fully rendered before they’re sent to the browser, search engines can easily crawl and index the content.
But SSR has its drawbacks:
- Slower Page Loads: Generating pages on-demand adds processing time, which can result in slower load times compared to pre-built static pages.
- Increased Server Load: The server must handle every request, which can become resource-intensive as traffic scales.
Where ISR Comes In
Incremental Static Regeneration (ISR) is a hybrid approach introduced in Next.js that combines the advantages of both SSG and SSR:
- Like SSG, ISR pre-builds static pages to ensure fast initial load times.
- Like SSR, ISR allows these static pages to be updated dynamically without needing to rebuild the entire site.
How ISR Works
-
Pre-Building at Build Time:
- During the initial build process, Next.js pre-generates as many pages as possible and stores them as static files. These pages are served instantly to users.
-
Dynamic Updates with Revalidation:
- ISR allows you to define a
revalidate
interval (e.g., every 60 seconds). This tells Next.js to regenerate the static page with fresh data at regular intervals.
Example:
- A blog post page is pre-built at build time.
- After the
revalidate
interval (e.g., 60 seconds), if someone visits the page, Next.js checks if there’s new data available. If there is, it regenerates the page in the background while continuing to serve the existing static page to users. - Once the new page is built, it replaces the old static version, ensuring subsequent visitors see the updated content.
- ISR allows you to define a
-
On-Demand Generation:
- ISR can also handle pages that weren’t pre-built at build time. These pages are generated on the first request and then cached for future requests. This is particularly useful for large sites with thousands of pages, where building all pages upfront isn’t practical.
Why ISR is a Game-Changer
ISR combines the performance of SSG with the flexibility of SSR to deliver a seamless experience. Let’s summarize how ISR bridges the gap between SSG and SSR:
Aspect | Static Site Generation (SSG) | Server-Side Rendering (SSR) | Incremental Static Regeneration (ISR) |
---|---|---|---|
Performance | Very fast | Slower due to request-time rendering | Fast due to pre-built pages served statically |
Dynamic Updates | Requires full site rebuild | Real-time for every request | Dynamic updates at intervals or on-demand |
Scalability | High | Limited by server capacity | High |
SEO-Friendly | Yes | Yes | Yes |
Best For | Static content | Frequently updated content | Sites needing a mix of static and dynamic content |
Real-World Use Cases for ISR
-
E-commerce Platforms:
- Product pages can display up-to-date inventory or pricing without the overhead of SSR.
- Example: An online store shows the latest stock count for a product using ISR.
-
Blogs and News Sites:
- New articles can be added and updated dynamically while maintaining fast load times for readers.
- Example: A news website updates headlines and featured articles every minute.
-
Marketing Websites:
- Content updates like testimonials or promotional banners can be made without rebuilding the entire site.
- Example: A corporate website adds a new client success story, and it appears live within minutes.
ISR is a highly efficient solution for modern web applications, especially for businesses looking to deliver fast, dynamic, and SEO-friendly experiences. With its ability to blend the benefits of SSG and SSR, ISR is an essential tool for any developer working with Next.js. At Prateeksha Web Design, we help businesses harness ISR to create websites that are not only performant but also highly scalable and future-proof.
Key Concepts Behind ISR
To implement Incremental Static Regeneration (ISR) effectively, it’s essential to understand its core mechanisms. These concepts enable developers to deliver fast, dynamic, and scalable websites. Let’s delve into each one:
1. Revalidation Time
Revalidation Time is at the heart of ISR. It determines how frequently Next.js checks for new data and regenerates the static page to reflect those updates.
How It Works:
- When defining the
revalidate
property in thegetStaticProps
function, you set an interval (in seconds) that dictates how often Next.js should regenerate the page in the background. - Until the revalidation time elapses, the currently cached version of the page is served to users.
Example:
export async function getStaticProps() {
const data = await fetch("https://api.example.com/posts");
return {
props: { data },
revalidate: 60, // Check for updates every 60 seconds
};
}
- If the
revalidate
time is set to60
seconds:- For the first request after a build, the pre-built static page is served.
- Subsequent requests within the 60-second window serve the same cached version.
- After 60 seconds, the next request triggers a background regeneration process that fetches fresh data and updates the page.
Benefits of Revalidation Time:
- Efficient Updates: Only regenerate pages when necessary, minimizing server workload.
- Fast User Experience: Users don’t experience delays since old versions are served while updates occur in the background.
2. Fallback Pages
When you have a large number of pages or don’t know all the page paths during the build process, fallback pages allow you to generate these pages dynamically upon request.
How It Works:
Fallback behavior is controlled through the fallback
option in getStaticPaths
:
-
Static Paths: Pre-defined paths are generated during the build process.
export async function getStaticPaths() { return { paths: [{ params: { id: "1" } }, { params: { id: "2" } }], fallback: "blocking", }; }
-
Fallback Modes:
fallback: false
:- Only pre-defined paths are generated, and any non-predefined paths return a 404.
fallback: true
:- Pages not pre-built are generated on demand when requested by the user. While the page is being built, a placeholder is shown.
fallback: 'blocking'
:- Similar to
true
, but the user waits until the page is fully generated before it’s served.
- Similar to
Example:
For an e-commerce site with thousands of products, you may not pre-build every product page. Using fallback: 'blocking'
, you can generate a new page dynamically the first time it’s requested, then cache it for future requests.
Benefits of Fallback Pages:
- Scalability: Handle large or unpredictable datasets without bloating the build process.
- User Experience: Dynamically serve pages while ensuring seamless caching for future visits.
3. Cache Invalidation
Cache invalidation is the process of replacing old versions of a page with updated ones after the revalidation time has passed. This ensures users always see fresh content while maintaining the performance of static pages.
How It Works:
- After a page is revalidated, Next.js discards the outdated static version and replaces it with the new one in the cache.
- Future visitors automatically receive the updated page.
Example:
Imagine a blog site using ISR:
- A new post is published, and the API data changes.
- Once the revalidation time elapses, the first visitor triggers a background regeneration.
- The newly generated page replaces the old one, ensuring all subsequent visitors see the latest content.
Benefits of Cache Invalidation:
- Fresh Content: Users always see the most recent updates after a revalidation cycle.
- Reduced Deployment Overhead: No need to manually rebuild and deploy the entire site for small updates.
Benefits of ISR for Modern Web Applications
ISR isn’t just a technical marvel; it delivers practical advantages that make it an essential tool for modern web development. Let’s explore how it benefits developers, businesses, and users:
1. Improved Performance
ISR leverages the power of static files to ensure fast load times:
- Pre-rendered pages are served directly from Content Delivery Networks (CDNs), minimizing latency.
- Static files reduce server dependency, allowing the site to handle higher traffic without additional infrastructure.
Why It Matters:
- E-commerce Stores: Faster product page loads can lead to higher conversions.
- Content-Heavy Sites: Blogs and news platforms can scale efficiently while keeping their content fresh.
2. SEO Optimization
Search engines prioritize sites with fast load times and easily crawlable content. ISR optimizes these factors:
- Static pages are indexed quickly by search engines, improving visibility.
- Dynamic updates ensure that search engines always find the latest content, enhancing rankings.
Why It Matters:
- Higher Rankings: Fresh, indexed content helps your site rank above competitors.
- Better User Experience: Fast-loading pages reduce bounce rates and increase time on site.
3. Dynamic Content Updates
With ISR, you no longer need to redeploy your entire website to update static pages. Updates happen automatically:
- ISR is perfect for scenarios where content changes frequently but doesn’t need real-time updates.
- For example, updating pricing, blog posts, or product details can happen without disrupting other site pages.
Why It Matters:
- Time-Saving: Developers can focus on creating new features instead of rebuilding the site.
- User Satisfaction: Visitors always see relevant and updated information.
4. Cost Efficiency
ISR reduces the need for continuous server-side rendering (SSR), which can be resource-intensive and costly:
- Static files are inexpensive to store and serve, even at scale.
- By caching pages and revalidating them in intervals, ISR minimizes server usage, lowering hosting costs.
Why It Matters:
- Startups and Small Businesses: ISR offers enterprise-level performance without enterprise-level costs.
- E-commerce: Handle sales surges or promotional events without additional infrastructure.
The Key Concepts Behind ISR—Revalidation Time, Fallback Pages, and Cache Invalidation—are the building blocks of a feature that combines the best of static site generation and server-side rendering. The benefits of ISR, including improved performance, SEO optimization, dynamic updates, and cost efficiency, make it a transformative tool for any modern web application.
At Prateeksha Web Design, we leverage ISR to build high-performance websites tailored to your business needs. Whether you're running a blog, e-commerce platform, or corporate site, we ensure your website is fast, dynamic, and scalable. Contact us today to learn how ISR can elevate your web presence!
Setting Up ISR in Your Next.js Application (App Router)
The App Router in Next.js (introduced with Next.js 13) offers a new approach to setting up ISR by leveraging React’s Server Components and simplifying dynamic rendering with better separation of concerns. Below is a detailed guide to implementing ISR effectively using the App Router, along with sample code for each step.
1. Define Revalidation in generateStaticParams
and fetch
In the App Router, revalidate
is used in the page component itself or in the generateStaticParams
function for dynamic routes. Here’s how it works:
Static Page with ISR (Revalidation)
You can specify the revalidate
property directly when fetching data for your pages. The revalidation interval determines how often the data is refetched in the background.
// app/page.js
export const revalidate = 60; // Revalidate every 60 seconds
async function getData() {
const res = await fetch("https://api.example.com/data", {
next: { revalidate: 60 },
});
if (!res.ok) {
throw new Error("Failed to fetch data");
}
return res.json();
}
export default async function HomePage() {
const data = await getData();
return (
<main>
<h1>Welcome to ISR with Next.js</h1>
<p>Data fetched: {data.message}</p>
</main>
);
}
2. Handling Dynamic Routes with Fallback Pages
For dynamic routes, ISR is handled by specifying a generateStaticParams
function and enabling fallback behavior with revalidate
.
Dynamic Routes with ISR
- Use
generateStaticParams
to predefine paths during the build process. - Use
fetch
with{ next: { revalidate: 60 } }
to enable ISR for dynamic pages.
// app/products/[id]/page.js
export const revalidate = 60; // Revalidate every 60 seconds
async function getProduct(id) {
const res = await fetch(`https://api.example.com/products/${id}`, {
next: { revalidate: 60 },
});
if (!res.ok) {
throw new Error("Failed to fetch product");
}
return res.json();
}
export async function generateStaticParams() {
const res = await fetch("https://api.example.com/products");
const products = await res.json();
// Predefine paths during the build
return products.map((product) => ({
id: product.id.toString(),
}));
}
export default async function ProductPage({ params }) {
const { id } = params;
const product = await getProduct(id);
return (
<main>
<h1>Product: {product.name}</h1>
<p>Price: ${product.price}</p>
<p>Description: {product.description}</p>
</main>
);
}
Key Notes:
generateStaticParams
ensures that some pages are pre-built.- Pages not pre-built will be dynamically rendered the first time they’re accessed, thanks to the revalidation mechanism.
3. Monitor ISR with Logs and Tools
To ensure ISR is functioning correctly, monitor its performance using:
-
Vercel Analytics:
- Log into your Vercel dashboard to track revalidation, cache hits/misses, and regeneration times.
-
Custom Logs:
- Add logs in your API or server to monitor data fetching and page generation times.
// Example logging in API route
export default async function handler(req, res) {
console.log("Fetching data for ISR at:", new Date().toISOString());
const data = await fetchData();
res.status(200).json(data);
}
How This Works in the App Router
Step-by-Step Breakdown:
-
Define Revalidation:
- The
revalidate
property is either defined in the page or within thefetch
options using{ next: { revalidate: N } }
.
- The
-
Dynamic Route Handling:
- Use
generateStaticParams
to specify the paths to pre-build and fallback handling for others. - Dynamic pages not pre-built are generated on the first request and cached for subsequent requests.
- Use
-
Background Revalidation:
- Once the revalidation time elapses, Next.js fetches fresh data in the background.
- Users continue to see the old page until the new one is ready.
Full Example for a Blog with ISR (App Router)
Here’s a complete setup for a blog with dynamic routes, ISR, and App Router:
Blog Home Page
// app/blog/page.js
export const revalidate = 60; // Revalidate every 60 seconds
async function getPosts() {
const res = await fetch("https://api.example.com/posts", {
next: { revalidate: 60 },
});
if (!res.ok) {
throw new Error("Failed to fetch posts");
}
return res.json();
}
export default async function BlogHome() {
const posts = await getPosts();
return (
<main>
<h1>Our Blog</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>
<a href={`/blog/${post.id}`}>{post.title}</a>
</li>
))}
</ul>
</main>
);
}
Individual Blog Post
// app/blog/[id]/page.js
export const revalidate = 120; // Revalidate every 120 seconds
async function getPost(id) {
const res = await fetch(`https://api.example.com/posts/${id}`, {
next: { revalidate: 120 },
});
if (!res.ok) {
throw new Error("Failed to fetch post");
}
return res.json();
}
export async function generateStaticParams() {
const res = await fetch("https://api.example.com/posts");
const posts = await res.json();
return posts.map((post) => ({
id: post.id.toString(),
}));
}
export default async function BlogPost({ params }) {
const { id } = params;
const post = await getPost(id);
return (
<main>
<h1>{post.title}</h1>
<p>{post.content}</p>
</main>
);
}
Monitoring and Troubleshooting
- Log Cache Status: Add middleware to log cache hits/misses.
- Analyze Logs: Use Vercel or custom analytics for better insights.
- Test Fallbacks: Ensure fallback pages work correctly by visiting non-prebuilt paths.
This approach ensures your Next.js app is dynamic, fast, and scalable, leveraging the full potential of ISR with the App Router.
Best Practices for Using ISR
-
Optimize Revalidation Times:
- Shorter revalidation intervals provide fresher content but may increase server load.
- Balance performance and freshness based on your audience's needs.
-
Cache Efficiently:
- Use CDNs to distribute your static files globally. This ensures low latency for users across regions.
-
Monitor Data Sources:
- Ensure your APIs or databases return data consistently and quickly. ISR relies on real-time data fetching, and slow responses can delay updates.
-
Test Fallback Scenarios:
- If using fallback pages, test them to avoid unexpected delays for first-time visitors.
-
SEO Enhancements:
- Structure your ISR pages with rich metadata, alt tags, and descriptive URLs. These elements enhance search engine discoverability.
Latest Advancements in ISR and Next.js
-
Next.js 13 and App Directory:
- The introduction of the app directory in Next.js 13 simplifies ISR implementation and allows better control over revalidation strategies.
- Server components are now seamlessly integrated, enabling lighter client-side bundles.
-
Enhanced Middleware:
- Middleware in Next.js lets you perform user-specific checks (e.g., geolocation or authentication) before serving ISR-generated pages.
-
Vercel’s Edge Network:
- Vercel, the company behind Next.js, has expanded its edge infrastructure. This ensures that ISR pages are served blazingly fast across the globe.
Why ISR is Ideal for Small Businesses
Small businesses need websites that are fast, reliable, and cost-efficient. ISR enables this by:
- Keeping product pages and blogs updated in real-time.
- Offering better SEO performance due to static rendering.
- Reducing hosting costs by serving pages as static files.
At Prateeksha Web Design, we understand these needs and build customized Next.js solutions that leverage ISR for maximum impact.
Next.js Optimization Tips for ISR
-
Data Fetching Efficiency:
- Use lightweight APIs or GraphQL to reduce payload sizes.
- Avoid over-fetching data; fetch only what’s necessary.
-
Pre-render Critical Pages:
- Identify high-traffic or high-value pages (e.g., landing pages) and ensure they are pre-rendered.
-
Lazy Loading for Non-critical Content:
- Use lazy loading for images and secondary content to improve perceived load times.
-
Use Environment Variables:
- Secure API keys and sensitive configurations using environment variables. This enhances trustworthiness.
-
Performance Monitoring:
- Tools like Lighthouse or Vercel’s performance insights can help you measure and optimize ISR performance.
Prateeksha Web Design: Your Partner in Next.js Excellence
When it comes to implementing cutting-edge features like ISR, experience matters. At Prateeksha Web Design, we specialize in:
- Next.js Development: Building fast, scalable websites.
- SEO-Optimized Designs: Ensuring your website ranks high.
- Custom E-commerce Solutions: Tailored stores with Next.js ISR.
- Maintenance and Support: Keeping your website updated and secure.
With expertise in modern web development, we empower small businesses to succeed online. Whether you’re launching a blog, e-commerce site, or corporate portal, our Next.js optimization tips ensure maximum performance.
Conclusion: Mastering ISR for the Future
Mastering Incremental Static Regeneration (ISR) in Next.js is essential for creating websites that are fast, reliable, and SEO-friendly. By leveraging this feature, you can strike the perfect balance between performance and dynamic content.
Small businesses, especially, can benefit immensely from ISR. With Prateeksha Web Design’s expertise, you can stay ahead of the curve, deliver exceptional user experiences, and achieve long-term success in the digital space.
Let us help you take your website to the next level with Next.js ISR. Contact Prateeksha Web Design today to discuss your project!
About Prateeksha Web Design
Prateeksha Web Design offers services to help clients effectively utilize Next.js Incremental Static Regeneration (ISR) for faster and more efficient web development. Our team provides guidance on implementing ISR to generate static pages with dynamic data, ensuring improved performance and SEO benefits. We assist in setting up ISR with optimal revalidation intervals to keep content updated without compromising speed. Prateeksha Web Design also offers training and support to maximize the benefits of ISR for client websites.
Interested in learning more? Contact us today.
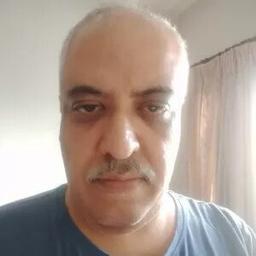