In the fast-evolving world of web development, choosing the right tools and technologies is critical for building robust, scalable, and efficient applications. Among the myriad options available, Node.js has emerged as a standout choice for backend development. Whether you're crafting a simple blog or building a large-scale real-time application, Node.js offers a combination of speed, scalability, and flexibility that’s hard to beat.
But what makes Node.js so special? Why should you choose it for your backend? Let’s break it down.
What Is Node.js?
To understand why Node.js is a game-changer, it’s essential to know what it is and how it works.
Node.js in Simple Terms
Node.js is an open-source, cross-platform runtime environment that lets you execute JavaScript code outside of a web browser. Think of it as the tool that brought JavaScript, a language traditionally used for frontend development, into the server-side world. This innovation allows developers to use one language across the entire stack, making development more efficient and seamless.
Key Features of Node.js
-
Built on Chrome’s V8 Engine:
The V8 engine compiles JavaScript directly into machine code, ensuring lightning-fast execution. -
Event-Driven Architecture:
Node.js operates on an event-driven, non-blocking I/O model, which allows it to handle multiple requests concurrently without performance degradation. -
Lightweight and Scalable:
Node.js uses a single-threaded event loop, making it highly efficient in terms of memory usage and capable of handling a large number of simultaneous connections. -
npm (Node Package Manager):
Node.js comes with npm, the largest ecosystem of open-source libraries, enabling developers to integrate a wide variety of tools and features into their applications effortlessly.
The Significance of Node.js
Before Node.js, JavaScript was primarily a client-side scripting language. Backend development required other languages like PHP, Python, or Ruby. Node.js bridged this gap by enabling developers to use JavaScript on both the client and server sides, simplifying the development process and improving productivity.
When Should You Use Node.js for Your Backend?
Node.js is versatile, but it isn’t the best choice for every project. Understanding when to use Node.js can help you maximize its strengths while avoiding potential pitfalls.
1. Real-Time Applications
If your project involves real-time communication—such as chat applications, video conferencing, or collaborative tools—Node.js is your best friend. Its event-driven architecture can handle multiple connections efficiently without lag. With libraries like Socket.IO, implementing real-time features becomes seamless.
2. Applications with High Traffic
For applications that expect a high volume of concurrent users or requests, Node.js is ideal. Its asynchronous nature ensures that the server remains responsive even under heavy loads.
3. APIs and Microservices
Node.js shines when building lightweight and fast APIs or microservices. Its ability to handle asynchronous requests makes it perfect for modern web architectures where microservices interact with various endpoints simultaneously.
4. Single-Page Applications (SPAs)
Node.js works exceptionally well with SPAs, where much of the logic happens in the frontend, and the backend serves as a lightweight data provider. Frameworks like Express.js simplify building backend services for SPAs.
5. Streaming Applications
Whether you’re building a video streaming platform like Netflix or a music streaming service like Spotify, Node.js can handle the streaming of large amounts of data efficiently. Its built-in streaming modules allow developers to process data in chunks, reducing memory usage.
6. IoT Applications
For Internet of Things (IoT) projects, where devices communicate with servers frequently, Node.js’s non-blocking I/O makes it an excellent choice. Its scalability allows it to manage data from numerous devices without breaking a sweat.
How to Use Node.js for Backend Development?
Now that you know when to use Node.js, let’s explore how to implement it effectively in backend development.
1. Set Up Node.js
- Download Node.js from Node.js official website.
- Use npm, which comes bundled with Node.js, to install packages and manage dependencies.
2. Write Your First Server
Start with a basic HTTP server:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, Node.js!');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
3. Use Frameworks Like Express.js
For more complex applications, Express.js provides a simplified interface for building APIs and web servers:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Welcome to Express.js with Node.js!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
4. Connect to a Database
Node.js works with both relational and non-relational databases:
- MongoDB: Use the Mongoose library for seamless integration.
- MySQL/PostgreSQL: Use libraries like
sequelize
orknex.js
.
5. Implement Real-Time Communication
Integrate Socket.IO for real-time communication:
const io = require('socket.io')(3000);
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('message', (msg) => {
io.emit('message', msg);
});
});
6. Optimize for Deployment
- Use PM2 for process management and monitoring.
- Deploy your application on platforms like AWS, Heroku, or DigitalOcean.
Why Should You Use Node.js for Backend Development?
Let’s talk about the "why" in detail:
1. Speed and Performance
Node.js is built on the V8 engine, ensuring that JavaScript code executes quickly. Its non-blocking I/O operations enable the server to process multiple requests simultaneously.
2. Cost Efficiency
Using JavaScript across the stack means you don’t need separate teams for frontend and backend, saving development time and cost.
3. Scalability
Node.js’s event-driven architecture makes it highly scalable, allowing it to handle thousands of concurrent connections efficiently.
4. Flexibility
Node.js adapts to various application types, from simple websites to real-time applications and microservices. The vast ecosystem of npm packages makes it easier to add features without starting from scratch.
5. Community Support
With a large and active developer community, Node.js offers plenty of resources, tutorials, and support.
Why Should You Care About Node.js?
If you’re a young, ambitious developer or a business owner planning your next project, understanding why Node.js matters is crucial. Here’s why:
-
Speed and Performance:
Node.js uses an event-driven, non-blocking I/O model, making it extremely efficient. It’s perfect for building fast and scalable apps like chat applications or real-time collaboration tools. -
JavaScript Everywhere:
With Node.js, you can use JavaScript both on the client and server sides. This eliminates the need for learning two separate languages, streamlining the development process. -
Massive Ecosystem:
Node.js has an extensive package ecosystem, npm, which boasts over a million libraries. Whether you need authentication, database interaction, or utility tools, there’s likely an npm package for it. -
Community Support:
With an active and vibrant developer community, Node.js ensures you’ll never feel stuck. From Stack Overflow to GitHub repositories, help is just a few clicks away.
When Should You Use Node.js for Backend?
Knowing when to use Node.js can make or break your project. While it’s a versatile tool, there are scenarios where it truly shines:
1. Real-Time Applications
- Examples: Chat apps, video conferencing tools, live collaboration software.
Node.js’s non-blocking architecture is ideal for handling real-time data and multiple simultaneous connections. Tools like Socket.io make implementing WebSockets (for real-time communication) seamless.
2. APIs and Microservices
- Examples: RESTful APIs, GraphQL APIs.
Node.js excels at handling lightweight APIs and microservices due to its asynchronous nature. It processes multiple requests efficiently, making it perfect for applications with high traffic.
3. Single-Page Applications (SPAs)
- Examples: Gmail, Trello.
SPAs rely heavily on server-side logic to provide a seamless user experience. Node.js ensures a fast backend to complement the dynamic frontend.
4. Streaming Applications
- Examples: Netflix, Spotify.
Thanks to its streaming capabilities, Node.js handles file uploads and streaming applications with ease. Its ability to process parts of a file as it downloads minimizes latency.
5. IoT Applications
- Examples: Smart home systems, wearable devices.
Node.js’s lightweight nature and ability to handle many requests simultaneously make it a natural choice for Internet of Things (IoT) applications.
How to Use Node.js for Backend Development?
Implementing Node.js effectively requires a solid understanding of its ecosystem and tools. Here’s a roadmap to get started:
1. Setting Up Node.js
To begin:
- Download and install Node.js from Node.js official website.
- Use npm (Node Package Manager) to install libraries and tools.
2. Creating a Basic Server
A simple "Hello, World!" server can be created in a few lines of code:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
3. Using Frameworks
While Node.js is powerful on its own, frameworks like Express.js simplify backend development:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
4. Database Integration
Node.js supports various databases, both SQL and NoSQL:
- MySQL or PostgreSQL: Use libraries like
sequelize
orknex
. - MongoDB: Use
mongoose
for seamless integration.
5. Real-Time Functionality
Incorporate Socket.io for real-time communication:
const io = require('socket.io')(3000);
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('message', (msg) => {
io.emit('message', msg);
##
});
});
6. Deploying Your Application
Tools like PM2 ensure smooth deployment and monitoring:
pm2 start app.js
For hosting, platforms like AWS, Heroku, and DigitalOcean are popular choices.
Why Use Node.js for Your Backend?
1. Scalability
Node.js uses an event-driven model, which is highly scalable compared to traditional thread-based servers.
2. High Performance
Built on the V8 engine, Node.js converts JavaScript into machine code, ensuring fast execution.
3. Cost-Effective
Using JavaScript across the stack reduces development time and costs. Plus, its lightweight nature requires fewer server resources.
4. Flexibility
With its modular design and huge npm library, Node.js adapts to various application requirements.
5. Practical for Small Businesses
If you’re running a small business, Node.js offers the perfect balance of performance and affordability. Platforms like Shopify leverage Node.js for scalability, proving its effectiveness for e-commerce.
Node.js in Action: Real-Life Applications
When it comes to proving the reliability and effectiveness of a technology, real-world applications speak louder than words. Node.js has become a cornerstone in the backend architecture of some of the world’s biggest and most demanding platforms. Companies like Netflix, LinkedIn, and Uber have adopted Node.js to power their backend systems, taking full advantage of its performance, scalability, and developer-friendly nature.
Let’s take an in-depth look at how these global giants use Node.js to solve complex problems and deliver exceptional user experiences.
1. Netflix: Fast Startup Time and Efficient Performance
Background:
Netflix, the world’s leading streaming platform with over 200 million subscribers globally, demands a highly responsive and efficient backend to provide seamless streaming experiences. With users consuming millions of hours of content daily, performance and scalability are critical for Netflix’s success.
Why Netflix Chose Node.js:
Netflix transitioned from a Java-based backend to Node.js for several key reasons:
- Startup Time Reduction: Java applications were slowing down the startup time for Netflix’s user interface. Node.js helped reduce this delay by offering faster execution and asynchronous processing.
- Unified Development: Node.js allowed Netflix engineers to use a single language (JavaScript) for both server-side and client-side development, streamlining workflows and improving efficiency.
- Lightweight and Scalable: The lightweight nature of Node.js made it easier to manage microservices, which are central to Netflix’s backend architecture.
How Netflix Uses Node.js:
Netflix uses Node.js for its server-side rendering and real-time application monitoring. The result is:
- Faster loading times for user interfaces.
- Improved developer productivity through unified codebases.
- Real-time insights into system performance and user interactions.
Outcomes:
By leveraging Node.js, Netflix achieved a 70% reduction in startup time for their application, significantly enhancing the user experience. Additionally, the switch to Node.js allowed for better handling of high traffic volumes during peak hours, such as when a popular new show or movie is released.
2. LinkedIn: High-Speed Processing for Millions of Users
Background:
LinkedIn, the largest professional networking platform with over 900 million users worldwide, is built on a backend system designed to handle millions of concurrent requests. From delivering real-time notifications to processing complex queries, LinkedIn’s backend must operate with precision and speed.
Why LinkedIn Chose Node.js:
LinkedIn migrated from Ruby on Rails to Node.js for several reasons:
- Improved Performance: Node.js’s non-blocking I/O model allowed LinkedIn to process multiple requests concurrently without latency issues.
- Resource Efficiency: Node.js enabled LinkedIn to handle the same workload with fewer servers, reducing infrastructure costs.
- Mobile Optimization: With the rise of mobile usage, LinkedIn needed a backend solution that could deliver faster and more efficient APIs to mobile devices.
How LinkedIn Uses Node.js:
LinkedIn uses Node.js to power its backend API that serves data to both its web and mobile applications. The asynchronous nature of Node.js ensures:
- Quick delivery of real-time notifications (e.g., connection requests, message alerts).
- Efficient data synchronization between devices and LinkedIn’s servers.
- Faster page loads and smoother transitions in the app.
Outcomes:
By adopting Node.js, LinkedIn reported:
- A 10x reduction in server count while maintaining the same performance levels.
- A 50% faster page load speed for its mobile app, directly improving user satisfaction and engagement.
3. Uber: Real-Time Request Handling at Scale
Background:
Uber, the global ride-hailing giant, operates a platform that connects millions of drivers and riders in real time. With a massive scale of operations and the need to process ride requests, updates, and GPS data within milliseconds, Uber required a backend that could handle high-speed, high-volume tasks.
Why Uber Chose Node.js:
Uber chose Node.js because:
- Real-Time Capabilities: Uber’s operations depend heavily on real-time data processing, and Node.js’s event-driven architecture made it a natural fit.
- Scalability: Node.js is designed to handle a large number of concurrent connections, making it ideal for Uber’s global user base.
- Rapid Development: Node.js enabled Uber’s developers to iterate quickly and deploy new features or updates without disrupting services.
How Uber Uses Node.js:
Node.js powers Uber’s dispatch system, ensuring that:
- Ride requests are matched with the nearest drivers in real time.
- GPS data is continuously updated and shared between drivers and riders.
- Notifications, such as ride statuses and fare updates, are delivered instantly.
Outcomes:
Node.js has been pivotal in Uber’s ability to scale globally while maintaining high performance. Benefits include:
- Real-Time Matching: Node.js processes thousands of requests per second, ensuring minimal wait times for riders.
- Seamless Scalability: Uber has scaled its Node.js-based backend to support millions of daily transactions without compromising performance.
Prateeksha Web Design: Your Node.js Partner
At Prateeksha Web Design, we understand the nuances of backend development. Whether you’re a startup or an established business, we can help you:
- Build robust Node.js backend systems.
- Create scalable, real-time applications.
- Optimize your web development workflow with cutting-edge technologies.
Our team specializes in leveraging the full potential of Node.js, ensuring your projects are fast, reliable, and future-proof.
Conclusion
Node.js is more than just a tool; it’s a revolution in web development. From its speed and performance to its flexibility and scalability, Node.js stands out as a top choice for server-side programming. Whether you’re building a chat app, an API, or a streaming service, Node.js has you covered.
At Prateeksha Web Design, we’ve mastered Node.js to deliver exceptional backend solutions. Ready to bring your idea to life? Let’s talk!
About Prateeksha Web Design
Prateeksha Web Design offers specialized services for integrating Node.js as a backend solution, focusing on its advantages for real-time applications and scalability. Their approach includes assessing project timelines (When) for optimal Node.js implementation, detailing implementation strategies (How) for seamless integration, and explaining the benefits (Why) of using Node.js for efficient, event-driven architecture. With expert guidance, they ensure projects harness the full power of Node.js, enhancing performance and user experience. Their services cater to businesses looking to modernize their web applications with robust backend frameworks.
Interested in learning more? Contact us today.
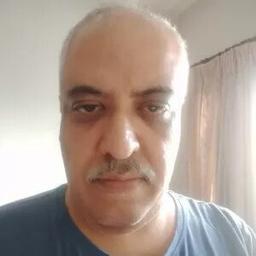