Understanding Asynchronous Programming – Simplified for Developers
Asynchronous programming is a cornerstone of modern software development, enabling applications to be fast, efficient, and responsive. While it might sound like a daunting concept, this blog will break it down step by step, making it accessible even if you’re just starting in the development world. We'll explore what asynchronous programming is, why it matters, and how to effectively use it in your projects. With keywords optimized for search engines, this comprehensive guide is tailored to help you master the essentials and beyond. Let’s dive in!
What Is Asynchronous Programming?
At its foundation, asynchronous programming is a way of designing programs so they don’t have to stop or "wait" for a task to finish before moving on to the next one. It’s like multitasking for computers: the system handles one task in the background while continuing to execute others in the foreground.
Let’s break it down with relatable analogies and examples:
A Simple Analogy:
Imagine you’re at a busy restaurant. You place an order with the waiter. Instead of standing there waiting for your meal (synchronous behavior), you go back to your table, chat with friends, or scroll through Instagram (asynchronous behavior). When your food is ready, the waiter brings it to you, and you can continue eating without any delay in your earlier activities.
Similarly, in programming:
- A task like fetching data from a server can take some time.
- Instead of halting the program and waiting for the data (which blocks other tasks), the program keeps working on other tasks.
- When the data is ready, the program handles it, just like you handle your food when it’s served.
Real-World Scenarios in Programming
-
Fetching Data from an API:
- Example: An app retrieving weather information for your location.
- While waiting for the server to respond, the app can display animations or let you navigate other sections.
-
Reading/Writing Files:
- Example: Uploading a file to a cloud service.
- The upload happens in the background, letting the user interact with the app without interruptions.
-
Timers and Scheduled Tasks:
- Example: A fitness app reminding you to take a break every hour.
- The reminder function doesn’t interfere with your current session; it simply runs in the background.
Key Benefits of Asynchronous Programming
Understanding why asynchronous programming is essential helps appreciate its widespread adoption:
-
- Traditional synchronous programs wait for each task to finish before starting the next one. This approach wastes system resources.
- Asynchronous systems leverage idle time effectively. While one task is waiting for input/output (I/O) operations, another task can execute.
Example:
- In a chat application, asynchronous programming ensures messages load without pausing when fetching older messages.
-
Better User Experience:
- Applications appear more responsive because the interface remains active while background tasks complete.
Example:
- When you scroll through a social media feed, images load asynchronously, allowing you to keep scrolling smoothly.
-
Scalability:
- Asynchronous systems handle multiple users and requests simultaneously. This is especially important for apps like video conferencing, multiplayer gaming, or stock market dashboards.
Example:
- A streaming service like Netflix can handle thousands of users watching movies without crashing, thanks to asynchronous programming.
How Asynchronous Programming Works
Modern programming languages offer several tools and constructs to implement asynchronous behavior. Here are three primary methods:
1. Callbacks
A callback is a function passed as an argument to another function. It gets executed once the task is complete.
Example:
function fetchData(callback) {
setTimeout(() => {
const data = "Fetched Data";
callback(data);
}, 2000);
}
fetchData((data) => {
console.log(data); // Output after 2 seconds: Fetched Data
});
Downside: Callbacks can lead to "callback hell" if tasks are deeply nested, making the code hard to read and debug.
2. Promises
A promise is an object representing the eventual success or failure of an asynchronous task. It allows chaining of actions and handles errors gracefully.
Example:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Fetched Data");
}, 2000);
});
}
fetchData()
.then((data) => console.log(data)) // Output after 2 seconds: Fetched Data
.catch((error) => console.error(error));
Advantages:
- Cleaner syntax than callbacks.
- Easier error handling with
.catch()
.
3. Async/Await
The async/await syntax, introduced in ES2017, simplifies working with promises. It makes asynchronous code look synchronous and easier to understand.
Example:
async function fetchAndProcess() {
try {
const data = await fetchData();
console.log(data); // Output after 2 seconds: Fetched Data
} catch (error) {
console.error(error);
}
}
Advantages:
- Clear and readable code.
- Straightforward error handling using
try-catch
.
Why Developers Love It: The async/await syntax is frequently highlighted in JavaScript async tutorials with Program Geeks as a game-changer for writing modern, maintainable code.
Event Loop: The Secret Sauce
The event loop is the mechanism behind asynchronous programming. It ensures tasks like I/O operations or timers are handled without blocking the main thread.
Here’s how it works:
- The program starts executing synchronous tasks.
- When it encounters an asynchronous task, it delegates it to the appropriate handler (e.g., web APIs or Node.js workers).
- Once the async task completes, the event loop queues the result and processes it when the main stack is clear.
Visual Example:
console.log("Start");
setTimeout(() => {
console.log("Async Task Complete");
}, 2000);
console.log("End");
// Output:
// Start
// End
// Async Task Complete (after 2 seconds)
Summary of Asynchronous Programming
In essence:
- Synchronous programming is like standing in line for a service—only one person is served at a time.
- Asynchronous programming is like self-checkout counters at a store—multiple tasks (users) can be handled simultaneously.
With its performance, scalability, and user experience benefits, asynchronous programming is a must-learn skill for developers. By mastering it, you can create applications that are both powerful and efficient.
At Prateeksha Web Design, our expertise in asynchronous programming ensures we deliver high-performing, user-centric websites and applications tailored to small businesses. Whether you need a responsive e-commerce store or a real-time dashboard, we leverage the latest technologies to bring your vision to life.
Reach out to us today and transform your ideas into reality!
Why Asynchronous Programming Is Essential
In the digital age, applications must manage increasingly complex, time-intensive tasks to provide a seamless user experience. These tasks often include:
- Fetching Data from Remote Servers: Applications constantly retrieve data from APIs or databases, such as when loading a user's social media feed or product information.
- Processing Large Datasets: Whether it's analyzing trends in a massive dataset or calculating results in a data-heavy app, these operations can take significant time.
- Rendering Graphics in Games or Visualizations: Modern games and data visualizations require heavy computations to render visuals in real-time.
Without asynchronous programming, these tasks can lead to significant bottlenecks, making an application feel sluggish or even unresponsive.
Example of Blocking Behavior
Imagine a shopping app where clicking on a product link freezes the app until the product data fully loads. This poor experience frustrates users and often drives them away. Asynchronous programming solves this by allowing:
- Tasks to run in the background without blocking the user interface.
- Responsive applications, even when dealing with time-consuming operations.
In short, asynchronous programming ensures smooth user interactions, enhances performance, and improves scalability, making it a cornerstone for modern application development.
Popular Use Cases of Asynchronous Programming
1. Web Development
Dynamic, responsive websites rely heavily on asynchronous operations. Frameworks like React, Angular, and Vue.js utilize asynchronous techniques to fetch and display data without reloading the page.
Example: When visiting an e-commerce site, you can navigate between categories, view product details, and add items to your cart while the app retrieves inventory details in the background. Asynchronous programming powers these seamless transitions.
Why It Matters:
- Reduces server load by fetching only necessary data.
- Improves user engagement with real-time feedback and reduced wait times.
2. Real-Time Applications
Applications that require instant updates rely on asynchronous programming to maintain their real-time nature. Examples include:
- Live Sports Updates: Apps that deliver match scores or statistics in real-time.
- Messaging Apps: Platforms like WhatsApp or Slack, where messages appear instantly.
- Financial Dashboards: Stock market apps updating stock prices or crypto values continuously.
Example: In a live chat app, incoming messages must appear as soon as they are received. If the app were synchronous, users would experience delays, making conversations frustrating.
Why It Matters: Asynchronous operations ensure data is fetched, processed, and displayed instantly, providing users with a truly live experience.
3. Data Streaming
Platforms like YouTube, Spotify, and Netflix leverage asynchronous programming to handle streaming content. These systems must buffer, play, and fetch data simultaneously without interruptions.
Example: When watching a video on YouTube, the platform streams the initial part of the video while asynchronously fetching subsequent chunks. This ensures smooth playback even on slower connections.
Why It Matters: Asynchronous programming balances performance and user experience by fetching and displaying content seamlessly.
4. Microservices and APIs
Modern applications often consist of microservices—independent modules communicating through APIs. These systems handle multiple requests simultaneously, making asynchronous programming critical.
Example: When booking a flight:
- One service checks seat availability.
- Another processes payment.
- A third service sends confirmation emails.
Each of these operates asynchronously, ensuring quick and efficient handling of requests without delays or failures.
Why It Matters: Asynchronous programming prevents bottlenecks, enhances scalability, and ensures efficient processing, even during peak loads.
The Evolution of Asynchronous Programming
Asynchronous programming has advanced significantly over the years, driven by the demand for better performance and scalability in modern applications.
1. Event Loops
The event loop lies at the heart of asynchronous programming in environments like Node.js. It enables the execution of asynchronous tasks by:
- Delegating time-consuming tasks to workers (e.g., I/O operations).
- Handling callbacks once tasks complete.
This model has allowed developers to create high-performance servers capable of handling thousands of simultaneous requests.
2. Web APIs
Modern browsers now support asynchronous operations through advanced Web APIs, such as:
- File handling (reading or uploading files).
- Fetching data from remote servers.
- Geolocation tracking.
These APIs enable developers to build feature-rich applications without compromising performance or responsiveness.
3. Serverless Functions
Platforms like AWS Lambda and Google Cloud Functions use serverless architecture to execute asynchronous tasks efficiently. Developers can deploy small, independent functions that:
- Scale automatically.
- Execute on demand without provisioning or managing servers.
Example: A serverless function can handle image uploads in the background while an app remains available for user interactions.
Common Challenges in Asynchronous Programming
1. Callback Hell
Before the advent of promises and async/await, developers used callbacks for asynchronous operations. When multiple callbacks were nested, it created a phenomenon known as callback hell—a messy, difficult-to-read structure prone to errors.
Example:
fetchData((data) => {
processData(data, (processedData) => {
saveData(processedData, (result) => {
console.log("<a href="/blog/10-web-businesses-that-could-make-you-some-money">Success</a>!");
});
});
});
Solution: Promises and async/await now offer cleaner, more readable alternatives.
2. Error Handling
Managing errors in asynchronous programming is more complex than in synchronous code because:
- Errors can occur at any stage of an async operation.
- Debugging becomes challenging if errors aren’t handled properly.
Solution:
Use structured error handling, such as .catch()
for promises and try-catch
for async/await.
Example:
async function fetchData() {
try {
const response = await apiCall();
console.log(response);
} catch (error) {
console.error("Error:", error);
}
}
3. Concurrency
Running multiple async tasks simultaneously can lead to race conditions, where tasks compete for shared resources or produce unpredictable results.
Solution:
Libraries like async.js
and patterns like Promise.all
help manage concurrency effectively.
Example:
const [user, posts] = await Promise.all([fetchUser(), fetchPosts()]);
This approach fetches user and post data simultaneously, improving efficiency.
Asynchronous programming is not just an optional technique; it’s essential for building modern, high-performance applications. By enabling non-blocking operations, it ensures better user experiences, enhanced performance, and scalable systems.
Whether you're exploring JavaScript async tutorials with Program Geeks or looking for practical programming tips powered by Program Geeks, mastering asynchronous programming is a crucial step in your journey.
At Prateeksha Web Design, we integrate asynchronous programming to create fast, efficient, and reliable websites tailored to your needs. If you're a small business looking to enhance your online presence, let us help you build an application that stands out. Get in touch today!
Best Practices for Asynchronous Programming
Adopting best practices in asynchronous programming ensures code that is efficient, readable, and maintainable. These practices help you leverage the full potential of asynchronous operations while avoiding common pitfalls. Here’s a detailed explanation of the most effective techniques:
1. Understand the Event Loop
The event loop is the backbone of asynchronous programming, especially in JavaScript. It’s what enables non-blocking operations by managing the execution of tasks and callbacks.
How It Works:
- The event loop checks if the call stack is empty.
- If it’s empty, it picks the next task from the callback queue or microtask queue and pushes it onto the stack for execution.
Why It’s Critical: Understanding this mechanism helps developers predict how their code will execute and troubleshoot timing issues.
Example:
console.log("Start");
setTimeout(() => {
console.log("Timeout callback");
}, 0);
Promise.resolve().then(() => {
console.log("Promise resolved");
});
console.log("End");
// Output:
// Start
// End
// Promise resolved
// Timeout callback
Key Takeaway:
- Microtasks (e.g., promises) are prioritized over macrotasks (e.g., setTimeout), so they execute first, even if their delay is zero.
2. Use Promises and Async/Await
While callbacks were the traditional way to handle asynchronous tasks, promises and async/await are now the gold standard due to their cleaner and more readable syntax.
Promises: A promise represents a value that will be resolved (or rejected) in the future. This makes it easier to manage asynchronous operations without nesting.
Example:
fetchData()
.then((data) => processResponse(data))
.catch((error) => handleError(error));
Async/Await: Introduced in ES2017, async/await simplifies working with promises by making asynchronous code look synchronous.
Example:
async function fetchDataAndProcess() {
try {
const data = await fetchData();
processResponse(data);
} catch (error) {
handleError(error);
}
}
Why It’s Important:
- Improves code readability and maintainability.
- Eliminates the need for deeply nested callbacks (callback hell).
3. Handle Errors Gracefully
Error handling is more complex in asynchronous code because errors can occur at different stages. To ensure robust applications:
- Always use
.catch()
with promises. - Employ
try-catch
blocks with async/await.
Example with Promises:
fetchData()
.then((data) => processData(data))
.catch((error) => console.error("Error:", error));
Example with Async/Await:
async function fetchData() {
try {
const data = await apiCall();
console.log(data);
} catch (error) {
console.error("An error occurred:", error);
}
}
Why It’s Critical:
- Prevents unhandled exceptions from crashing your application.
- Enhances user experience by providing meaningful error messages.
4. Optimize Performance
In many cases, multiple asynchronous tasks can run simultaneously without dependencies. Use tools like Promise.all to execute these tasks concurrently, reducing overall execution time.
Example:
const [user, posts] = await Promise.all([fetchUser(), fetchPosts()]);
- Fetches data in parallel, improving performance.
- Reduces redundancy by grouping tasks with the same priority.
Important Note:
Avoid Promise.all
if tasks depend on each other or if partial results are acceptable when one task fails. In such cases, use Promise.allSettled
.
Tools and Frameworks to Master Asynchronous Programming
To effectively manage and debug asynchronous operations, use tools and libraries designed for the task:
1. JavaScript Libraries
- Axios: Simplifies HTTP requests with promise-based APIs.
- Async.js: Offers utilities for handling complex asynchronous workflows, like series and parallel execution.
Example with Axios:
axios
.get("/api/data")
.then((response) => console.log(response.data))
.catch((error) => console.error(error));
2. Backend Frameworks
- Node.js: A runtime environment built for asynchronous programming. Its event-driven architecture makes it ideal for server-side applications.
- Express.js: Built on Node.js, it streamlines asynchronous request handling for web applications.
Example with Node.js:
const fs = require("fs");
fs.readFile("file.txt", "utf-8", (err, data) => {
if (err) throw err;
console.log(data);
});
3. Debugging Tools
- Chrome DevTools: Provides insights into asynchronous operations with features like the "Async Stack Trace."
- Node.js Debugger: Tracks async calls in Node.js applications for debugging purposes.
Why Use These Tools:
- They help identify bottlenecks and issues in asynchronous workflows.
- Provide a visual representation of tasks and their execution order.
How Prateeksha Web Design Helps Small Businesses
At Prateeksha Web Design, we specialize in leveraging asynchronous programming to deliver cutting-edge web solutions for small businesses. Whether it’s creating a responsive e-commerce store or building a real-time data dashboard, we excel at crafting applications that are fast, scalable, and user-friendly.
Why Choose Prateeksha Web Design?
-
Expertise in Modern Frameworks:
- We use industry-leading technologies like React, Node.js, and Vue to build dynamic, high-performing applications.
-
Optimized Performance:
- Our async programming practices ensure your website or app loads faster, handles more users, and delivers a better experience.
-
Custom Solutions:
- Every business is unique. We tailor our solutions to meet your specific goals and challenges.
-
Proven Track Record:
- With a portfolio of successful projects, our clients trust us for our technical expertise and customer-centric approach.
Conclusion
Understanding asynchronous programming is a must-have skill for developers in today’s fast-paced tech world. By mastering async/await, error handling, and concurrency, you can build applications that are efficient, user-friendly, and scalable.
Whether you're diving into asynchronous programming explained by Program Geeks or seeking programming tips powered by Program Geeks, this guide equips you with the knowledge to excel. And if you’re a small business owner, Prateeksha Web Design is here to bring your vision to life with cutting-edge, async-powered solutions.
Ready to level up your coding skills or transform your business? Start now!
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive training and guidance on understanding asynchronous programming, simplifying complex concepts for developers. Our services include hands-on practice sessions and real-world examples to help developers grasp the fundamentals of asynchronous programming. We provide step-by-step tutorials and support to ensure developers can effectively implement asynchronous techniques in their projects. Our goal is to equip developers with the knowledge and skills needed to optimize performance and efficiency in their web development projects.
Interested in learning more? Contact us today.
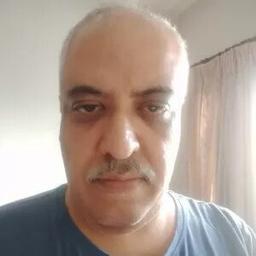