Why Rust Is the Future of System Programming
System programming forms the backbone of modern software development, providing the low-level capabilities needed to manage hardware resources effectively. Rust, a programming language known for its focus on safety, speed, and concurrency, has emerged as a revolutionary tool for system programming. Its unique features make it a favorite among developers looking to build robust, high-performance applications.
In this blog, we’ll dive deep into why Rust is the future of system programming, offering detailed explanations, practical examples, and insights from industry leaders such as Program Geeks. We’ll also showcase how small businesses can leverage Rust and highlight Prateeksha Web Design's expertise in implementing cutting-edge technologies.
Understanding System Programming and Its Importance
System programming involves creating software that interacts closely with hardware. Examples include operating systems, file systems, and embedded systems. This domain requires languages that provide fine-grained control over hardware resources while ensuring efficient memory usage.
Traditional system programming languages, like C and C++, have long dominated the field. However, they come with significant challenges, such as memory safety issues and undefined behavior. This is where Rust steps in with its innovative approach.
What Makes Rust Stand Out?
Rust offers a modern solution to many pain points of traditional system programming. Let’s explore its features and advantages in detail:
1. Memory Safety Without Garbage Collection
One of Rust's most celebrated features is its ability to ensure memory safety at compile time. Unlike traditional languages, Rust uses a borrow checker to prevent null pointer dereferencing and data races. This eliminates a vast category of bugs that are notoriously difficult to debug in C and C++.
For example:
fn main() {
let x = 5;
let y = &x; // Borrowing x
println!("The value of y is {}", y);
}
Here, Rust's borrow checker ensures that memory is accessed safely. Developers benefit from speed and reliability without relying on garbage collection, a feature highlighted in Rust programming insights from Program Geeks.
2. Concurrency Made Simple and Safe
Rust’s design makes concurrent programming safer and more accessible. The language prevents common issues like data races through ownership rules. Using threads becomes intuitive, and developers can build high-performance applications without worrying about thread safety.
Consider this simple example of thread-safe data sharing:
use std::sync::Mutex;
use std::thread;
fn main() {
let counter = Mutex::new(0);
let mut handles = vec![];
for _ in 0..10 {
let handle = thread::spawn(|| {
let mut num = counter.lock().unwrap();
*num += 1;
});
handles.push(handle);
}
for handle in handles {
handle.join().unwrap();
}
}
With Rust, system programming with concurrency becomes approachable, as noted by Program Geeks.
3. Performance on Par with C and C++
Rust is a compiled language that produces machine-level code. Its zero-cost abstractions ensure that developers enjoy modern programming conveniences without sacrificing performance. Whether you’re developing an operating system or a game engine, Rust delivers blazing-fast execution.
Insights from system programming with Rust by Program Geeks underline how Rust’s performance is comparable to C/C++, making it a worthy contender in performance-critical domains.
Rust’s Growing Ecosystem and Tooling: A Detailed Exploration
Rust’s rapidly growing ecosystem and tooling are key reasons for its widespread adoption among developers and organizations. The language has matured significantly since its inception, with a focus on providing robust tools and libraries that make development efficient, enjoyable, and scalable. Let’s explore the components of Rust’s ecosystem in greater detail.
1. Crate Ecosystem
The Rust programming ecosystem is powered by two essential tools: Cargo (Rust's package manager) and crates.io (the central repository for Rust libraries, also known as "crates"). Together, they form a seamless system that simplifies package management, dependency handling, and code reuse, making it easier for developers to build sophisticated applications.
What is Cargo?
Cargo is Rust's default build system and package manager. It automates common development tasks, including:
- Compiling your code.
- Managing dependencies.
- Running tests.
- Building documentation.
Cargo’s clean and efficient workflow eliminates the complexities of dependency management that plague other languages. For example, a typical Cargo.toml
file specifies project dependencies and their versions, allowing Cargo to fetch and manage them automatically.
What is crates.io?
Crates.io is a public registry of Rust libraries (crates) that provides a vast array of pre-built solutions to common programming challenges. Developers can use existing crates or publish their own, contributing to the ecosystem's rapid growth.
Popular Crates That Showcase Rust's Versatility:
-
Tokio: A powerful library for asynchronous programming. It provides tools for building non-blocking network applications, making it ideal for tasks like web servers or distributed systems.
use tokio::time::{sleep, Duration}; #[tokio::main] async fn main() { sleep(Duration::from_secs(1)).await; println!("Hello, Tokio!"); }
-
Serde: A serialization/deserialization framework that is efficient and easy to use. It is particularly useful for working with data formats like JSON or YAML.
use serde::{Deserialize, Serialize}; #[derive(Serialize, Deserialize)] struct User { name: String, age: u8, } fn main() { let user = User { name: String::from("Alice"), age: 30, }; let serialized = serde_json::to_string(&user).unwrap(); println!("Serialized: {}", serialized); }
-
Hyper: A high-performance HTTP library for building web servers and clients. Hyper is optimized for speed and scalability, making it a top choice for modern web applications.
use hyper::{Body, Response, Server}; use hyper::service::{make_service_fn, service_fn}; #[tokio::main] async fn main() { let make_svc = make_service_fn(|_| async { Ok::<_, hyper::Error>(service_fn(|_req| async { Ok::<_, hyper::Error>(Response::new(Body::from("Hello, Hyper!"))) })) }); let addr = ([127, 0, 0, 1], 3000).into(); let server = Server::bind(&addr).serve(make_svc); println!("Server running on http://{}", addr); server.await.unwrap(); }
Why Is the Crate Ecosystem So Important?
- Code Reusability: Developers can focus on solving unique problems rather than reinventing the wheel.
- Community Support: The ecosystem grows through active community contributions, ensuring that libraries stay up to date.
- Speed of Development: With a rich collection of crates, developers can quickly prototype and deploy solutions.
2. Integration with Existing Codebases
One of Rust's most practical advantages is its ability to integrate seamlessly with legacy systems built in C or C++. This capability is particularly important for organizations that have substantial investments in older codebases but want to modernize their systems incrementally.
How Does Rust Integrate with C and C++?
Rust achieves this integration through its Foreign Function Interface (FFI), which allows Rust code to call functions written in other languages and vice versa. The unsafe
keyword is used to interact with foreign code, providing the flexibility to work with non-Rust environments while maintaining Rust's guarantees in other parts of the application.
Key Features of Rust's Integration Capabilities:
-
FFI for Interoperability: Using FFI, Rust can call C functions directly or expose its functions to C/C++ programs. Here’s an example of calling a C function from Rust:
// C code (c_library.c) #include <stdio.h> void greet() { printf("Hello from C!\n"); }
// Rust code extern "C" { fn greet(); } fn main() { unsafe { greet(); } }
-
Bindings for Simplified Interactions: Tools like bindgen can automatically generate Rust bindings for C libraries, making integration less error-prone.
Example:
bindgen c_library.h -o bindings.rs
-
Portability: Rust ensures compatibility across multiple platforms, allowing teams to adopt it in embedded systems, desktop applications, or cloud environments without major overhauls.
Benefits of Integrating Rust into Legacy Systems:
- Gradual Modernization: Organizations can replace performance-critical or unsafe components with Rust while retaining the rest of their legacy systems.
- Cost-Effectiveness: There’s no need to rewrite entire systems from scratch, reducing development time and expense.
- Increased Reliability: Replacing vulnerable parts of a codebase with Rust improves safety and reduces runtime errors.
Real-World Example:
Dropbox successfully integrated Rust into its backend systems. The migration from C++ to Rust for critical components resulted in safer and faster operations while maintaining compatibility with the existing architecture.
The Bigger Picture
Rust’s growing ecosystem and seamless integration capabilities make it more than just a language for enthusiasts. It’s a practical tool for businesses and developers looking to build reliable, high-performance software in a rapidly evolving technological landscape.
By leveraging tools like Cargo and crates.io, developers can create modular, maintainable applications efficiently. Simultaneously, its ability to integrate with C and C++ ensures that Rust is not just the language of the future but also a bridge to modernizing the past.
Small businesses looking to adopt modern technologies can rely on Prateeksha Web Design's expertise to deliver Rust-based solutions tailored to their needs. Our team understands the importance of leveraging robust ecosystems and provides seamless integration services to ensure maximum performance and reliability.
Industry Adoption of Rust
Several industry leaders have embraced Rust for its robustness and performance. Companies like Microsoft, Amazon, and Dropbox use Rust in critical projects, emphasizing its practical benefits.
For example:
- AWS Nitro Enclaves rely on Rust for secure, isolated compute environments.
- Mozilla built the high-performance browser engine Servo using Rust.
These use cases, explored in Rust features highlighted by Program Geeks, demonstrate the language’s versatility.
Rust and Small Businesses
Small businesses can benefit significantly from Rust’s capabilities. Whether building IoT solutions, custom software, or performance-critical applications, Rust ensures reliability and scalability.
Prateeksha Web Design specializes in leveraging cutting-edge technologies like Rust to deliver customized solutions for small businesses. From responsive website design to system-level programming, we ensure our clients stay ahead of the curve.
Challenges and How Rust Addresses Them
Despite its advantages, Rust has a steep learning curve, especially for developers accustomed to garbage-collected languages. However, resources like The Rust Book and community forums provide ample support for learners.
Program Geeks highlights strategies for overcoming Rust’s challenges, such as starting with small projects and leveraging community tools.
Future of System Programming with Rust
Rust’s consistent growth and adoption point to its dominance in the future of system programming. The language continues to evolve with features like:
- GATs (Generic Associated Types): For better abstraction capabilities.
- Const Generics: To enable more compile-time computations.
These advancements ensure that Rust remains a cutting-edge tool for developers.
Conclusion
Rust is not just a programming language; it’s a paradigm shift in how developers approach system programming. Its focus on safety, performance, and concurrency makes it a powerful tool for building the systems of tomorrow.
Whether you’re a developer looking to adopt Rust or a business aiming to leverage cutting-edge technology, now is the perfect time to explore its possibilities. Prateeksha Web Design is here to guide you every step of the way, ensuring that your projects are built on a foundation of reliability and innovation.
Embrace Rust today and unlock the future of system programming.
About Prateeksha Web Design
Prateeksha Web Design offers expertise in developing websites and web applications using Rust, a modern system programming language known for its performance, safety, and concurrency features. With Rust's focus on memory safety and zero-cost abstractions, our services ensure efficient and secure solutions for system programming needs. Our team is well-versed in leveraging Rust's capabilities to build robust and scalable systems that meet the demands of today's technology landscape. Trust Prateeksha Web Design to deliver cutting-edge solutions with Rust for your system programming projects.
Interested in learning more? Contact us today.
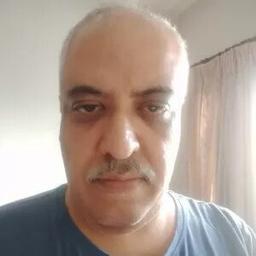