Table of Contents
- Introduction: Why Object-Oriented Design is Essential for Web-Based Application Development
- Core Principles of Object-Oriented Design and Their Importance in Web-Based Applications
- Class-Based Architecture for Web Applications: Structuring Code for Scalability
- Encapsulation in Web Design: Keeping Code Clean and Secure
- Inheritance and Reusability in Web Applications
- Polymorphism: Flexibility in Application Behavior
- Abstraction: Simplifying Complexity in Web-Based Application Design
- Design Patterns in Object-Oriented Web Development
- Applying SOLID Principles to Web-Based Applications
- Best Practices for Maintaining Object-Oriented Web Applications
- Conclusion: The Future of Object-Oriented Web Design
Introduction: Why Object-Oriented Design is Essential for Web-Based Application Development
In web development, the object-oriented approach has become the foundation for creating scalable and maintainable applications. This approach allows developers to structure their code into objects, representing different parts of the application. Objects make it easier to manage complex systems because they encapsulate both data and behaviors in a unified structure. Web-based applications are increasingly complex, involving various layers of functionality, including front-end interfaces, back-end servers, and databases. By leveraging object-oriented web design, you can create an architecture that is easy to extend, debug, and scale as the application grows.
In recent years, advancements in frameworks and technologies, such as React, Angular, and Node.js, have made object-oriented principles even more critical. These tools allow for modular development and offer a structured way to organize code. Let's dive deep into the core principles of object-oriented design and explore how they can improve web applications.
Core Principles of Object-Oriented Design and Their Importance in Web-Based Applications
Object-oriented design revolves around four major principles: Encapsulation, Inheritance, Polymorphism, and Abstraction. Each of these principles plays a crucial role in building scalable and efficient web applications. Here's how they work in practice:
- Encapsulation: Bundling data and methods together within objects ensures that the data is not directly accessible from outside, maintaining data integrity and security.
- Inheritance: This allows developers to create new classes that reuse the functionality of existing ones, promoting code reusability and saving development time.
- Polymorphism: The ability to use a single interface to represent different underlying data types enables more flexible code.
- Abstraction: Hides the complex implementation details and presents only the necessary functionality to the user.
By applying these principles in web-based application design, you create systems that are more maintainable and scalable. Each principle ensures that your application grows gracefully as you add new features, without introducing unwanted complexity.
Class-Based Architecture for Web Applications: Structuring Code for Scalability
One of the most significant advantages of using an object-oriented approach to web-based application design is the class-based structure. In a class-based architecture, each component of the application (such as users, products, or orders) is represented as a class. A class defines the blueprint for creating objects that share the same structure and behavior.
In modern web development, frameworks like Django (Python) and Laravel (PHP) use class-based architectures to promote modular and reusable code. React also promotes the use of class components, although newer versions favor functional components with hooks, they still adhere to object-oriented design principles.
Example:
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
login() {
// Logic for logging in a user
}
logout() {
// Logic for logging out a user
}
}
const user1 = new User("John Doe", "john@example.com");
user1.login();
Here, the User
class encapsulates the properties name
and email
, along with the methods login
and logout
. This design makes it easy to add new features later, such as user roles or authentication mechanisms, without modifying the existing codebase.
Class-based architectures allow developers to scale web applications without over-complicating the code structure, improving long-term maintainability.
Encapsulation in Web Design: Keeping Code Clean and Secure
Encapsulation is the practice of keeping an object's state private while exposing only necessary functions to interact with that state. This is a core concept in object-oriented web design that improves both security and code cleanliness.
For instance, in a web application that manages user accounts, you might want to ensure that certain sensitive data (like passwords) is not accessible directly from outside the object. Encapsulation ensures that only authorized parts of the application can interact with this data through well-defined methods.
In practice, this means creating getters and setters to manage how external code interacts with an object's properties. This is especially important in web applications where user input can be manipulated, exposing vulnerabilities if data isn't properly encapsulated.
Example:
class Account {
constructor(balance) {
let _balance = balance; // Private variable
this.getBalance = function () {
return _balance;
};
this.deposit = function (amount) {
_balance += amount;
};
this.withdraw = function (amount) {
if (amount <= _balance) {
_balance -= amount;
} else {
throw new Error("Insufficient balance");
}
};
}
}
const myAccount = new Account(1000);
myAccount.deposit(500); // Deposit money
console.log(myAccount.getBalance()); // Output: 1500
In this example, the balance is encapsulated in a local variable, _balance
, and can only be modified using specific methods like deposit
and withdraw
. This keeps the application secure, ensuring that outside code can’t directly alter critical data.
Encapsulation is key to keeping web-based applications secure and modular, especially as they scale and evolve.
Inheritance and Reusability in Web Applications
Inheritance is a fundamental principle of object-oriented design, allowing new classes to inherit properties and methods from existing ones. This is particularly useful in web applications where certain features are common across different components, such as user roles (e.g., admin, editor, subscriber).
By using inheritance, developers can create a base class with shared functionality and extend it for specific cases. This promotes code reusability and efficiency, as you don’t need to rewrite the same logic for every new feature or component.
For example, in an e-commerce application, you might have a base class called User
, and from this class, you can derive more specialized classes such as Customer
and Admin
.
Example:
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
login() {
// Login logic for all users
}
}
class Admin extends User {
constructor(name, email, permissions) {
super(name, email); // Call parent constructor
this.permissions = permissions;
}
manageUsers() {
// Admin-specific functionality
}
}
const admin1 = new Admin("Jane Doe", "jane@example.com", ["edit", "delete"]);
admin1.login(); // Admin can use the login method from the User class
admin1.manageUsers(); // Admin-specific method
In this example, Admin
inherits the properties and methods of User
, but also has its own functionality, such as manageUsers
. This structure allows for clean, scalable code where changes to the base class propagate through all derived classes.
Inheritance is a powerful tool for building web-based applications that require modularity and extensibility.
Polymorphism: Flexibility in Application Behavior
Polymorphism allows one interface to be used for different data types, giving flexibility to your web application’s behavior. It means that the same method can perform different tasks based on the object it’s being called on, which is invaluable in handling multiple data types in web applications.
For example, in an online payment system, you may have different types of payment methods like credit cards and PayPal. Both payment types can share a common method called processPayment()
, but each payment type will implement the method differently.
Example:
class Payment {
processPayment() {
throw new Error("Method not implemented");
}
}
class CreditCardPayment extends Payment {
processPayment() {
console.log("Processing credit card payment");
}
}
class PayPalPayment extends Payment {
processPayment() {
console.log("Processing PayPal payment");
}
}
const payments = [new CreditCardPayment(), new PayPalPayment()];
payments.forEach((payment) => payment.processPayment()); // Output: Processing credit card payment, Processing PayPal payment
In this case, processPayment()
behaves differently depending
on the type of payment being processed, even though it shares the same method signature. This flexibility allows for more dynamic and adaptable applications, where you can add new payment methods without altering the core logic.
Polymorphism is essential for building flexible and adaptable object-oriented web applications that can easily evolve with user needs.
Abstraction: Simplifying Complexity in Web-Based Application Design
Abstraction in web-based application design is about hiding the complexities of certain functionalities and exposing only the necessary parts to the user. It’s a way of managing complexity by providing a simple interface while keeping the intricate details hidden.
This concept is incredibly useful when dealing with large-scale web applications where different parts of the system interact with one another. By applying abstraction, developers can focus on building features without worrying about the underlying complexity.
For example, in a REST API, developers might abstract the details of how the API communicates with the database and instead focus on the inputs and outputs of the API. Users don’t need to know how the database is queried; they only care about the data returned by the API.
Example:
class API {
getUserData(userId) {
// Abstracting the database interaction
return database.query(`SELECT * FROM users WHERE id = ${userId}`);
}
}
const api = new API();
api.getUserData(123); // Abstraction: User doesn't need to know how the query works
By abstracting complex functionality, you create an architecture that is easier to maintain and extend as the application grows.
Abstraction is crucial for managing large-scale web applications, ensuring that the system is modular, easy to understand, and adaptable to future changes.
Design Patterns in Object-Oriented Web Development
In the context of object-oriented web development, design patterns provide reusable solutions to common problems. These patterns offer a way to structure code that is both efficient and scalable.
Some popular design patterns used in web-based applications include:
- Singleton Pattern: Ensures that a class has only one instance and provides a global point of access to it.
- Factory Pattern: Defines an interface for creating objects but allows subclasses to alter the type of objects that will be created.
- Observer Pattern: Allows one object to notify other objects about state changes, commonly used in frameworks like React.
By applying these design patterns in object-oriented web design, developers can solve architectural problems more efficiently, resulting in more robust and scalable applications.
Applying SOLID Principles to Web-Based Applications
The SOLID principles are five guidelines that help developers write more maintainable and scalable code. These principles are especially important in object-oriented web design, as they ensure the application remains flexible and easy to update.
- Single Responsibility Principle (SRP): Each class should have only one reason to change, meaning it should only have one responsibility.
- Open/Closed Principle (OCP): Classes should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subclasses should be replaceable by their base class without affecting the application’s functionality.
- Interface Segregation Principle (ISP): No class should be forced to implement methods it does not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules but should depend on abstractions.
Applying these principles to your object-oriented web design ensures that your code remains modular, testable, and easy to scale.
Best Practices for Maintaining Object-Oriented Web Applications
Maintaining a web application built using object-oriented design requires a commitment to best practices. These include:
- Consistent use of design patterns: Ensures scalability and reusability.
- Code refactoring: Regularly improve the codebase to remove any technical debt.
- Unit testing: Testing individual units of code to ensure they work as expected.
- Documentation: Properly document code to ensure other developers can easily understand and maintain it.
By following these best practices, developers can ensure that their object-oriented web applications remain maintainable over time.
Conclusion: The Future of Object-Oriented Web Design
As web applications continue to evolve, the object-oriented approach to web-based application design remains a powerful and effective way to build scalable, maintainable, and secure applications. By adhering to the principles of encapsulation, inheritance, polymorphism, and abstraction, and by leveraging design patterns and the SOLID principles, developers can create systems that are both flexible and robust.
The future of object-oriented web design is closely tied to the evolution of frameworks and technologies. As AI and machine learning become more integrated into web applications, the importance of structured, scalable, and maintainable code will only grow.
About Prateeksha Web Design
Prateeksha Web Design Company is a leading web development firm specializing in object-oriented approaches to web-based application design. They employ best practices such as encapsulation, modularity, and inheritance to create robust, scalable, and maintainable web applications.
Interested in learning more? Contact us today.
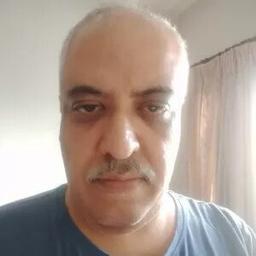