Mastering Next.js Authentication With Secure Practices: A Beginner’s Guide to Safe and Scalable Login Systems
Welcome to the world of Next.js authentication, where login buttons do more than just open doors—they secure your castle. Whether you're building a social app, an e-commerce platform, or the next big thing in tech, mastering secure login in Next.js is your golden ticket to keeping user data safe and your app reputable. Buckle up, because we're diving deep into the why, what, and how of authentication practices—served with a sprinkle of humor to make this technical feast more digestible.
Key Takeaways for This Guide
In this guide, we’re laying out the foundation for mastering authentication in Next.js. Each takeaway serves as a pillar for understanding and implementing secure login systems effectively. Here’s what we’re diving into:
1. Why Authentication Matters in Modern Web Apps
Authentication isn’t just a technical checkbox—it’s the backbone of user security. In today’s world, data breaches, phishing attacks, and unauthorized access are rampant. A poorly secured authentication system puts not just your app but also your users at risk.
Here’s Why You Should Care:
- Protects User Data: Login credentials, personal information, and financial details are often the most targeted by hackers.
- Builds Trust: Users are more likely to engage with apps that prioritize their security.
- Legal Compliance: Regulations like GDPR and CCPA mandate secure data handling, making authentication non-negotiable.
🔑 Think of your app as a digital vault. Authentication is the lock and key that keeps it secure.
2. Core Concepts Behind Secure Login in Next.js
Before diving into code, understanding the basics is crucial. Authentication in Next.js revolves around these key concepts:
-
Authentication vs. Authorization:
- Authentication: Verifies a user’s identity (e.g., logging in).
- Authorization: Determines what a user can access (e.g., admin privileges).
-
Session Management:
Sessions keep users logged in after authentication. They can be managed with:- Cookies: Stored on the client’s browser, often encrypted.
- Tokens (JWTs): A stateless method of session storage.
-
Third-Party Providers:
Services like Google, Facebook, or GitHub simplify authentication by handling the heavy lifting (e.g., password storage and verification).
3. Best Practices to Avoid Common Pitfalls
The internet can be a dangerous place, and secure authentication practices shield your app from vulnerabilities. These are the golden rules:
- Encrypt Everything: Always use HTTPS and encrypt sensitive data like passwords.
- Prevent Brute-Force Attacks: Implement rate limiting to block malicious repeated login attempts.
- Leverage 2FA (Two-Factor Authentication): Even if passwords are stolen, 2FA adds an extra security layer.
- Validate Inputs: Prevent SQL injection and XSS attacks by sanitizing and validating user inputs.
🔒 Remember: Security isn’t a one-time effort. It’s an ongoing commitment to your app and users.
4. Implementation With Examples (Yes, We’ll Code!)
Enough theory—let’s get practical! We’ll walk through implementing a secure login system using NextAuth.js, a library tailor-made for Next.js authentication.
- Step 1: Install the library and set up your API route.
- Step 2: Use Google OAuth for authentication.
- Step 3: Secure session management with cookies or tokens.
- Step 4: Add a user-friendly login/logout interface.
📚 By the end, you’ll have a working example of a secure and scalable login system.
5. Bonus: Pro Tips From Prateeksha Web Design to Level Up Your Next.js Game
At Prateeksha Web Design, we’ve seen it all—from common authentication blunders to cutting-edge solutions. Here’s how we ensure our apps are secure and user-friendly:
- Monitor for Vulnerabilities: Regularly test and audit your app using tools like OWASP ZAP.
- Optimize for Scalability: Use serverless authentication (e.g., NextAuth.js) to handle growing user bases effortlessly.
- Enhance User Experience: Simplify login processes with options like social logins or passwordless authentication.
🎯 When you combine security with usability, you’re not just building an app—you’re building trust.
Why Should You Care About Authentication?
Picture this: You leave your front door unlocked and invite trouble in. That’s exactly what an insecure authentication system does to your app. With hackers lurking around, secure login practices are your first line of defense.
Authentication Is More Than Security
- Earns User Trust: A secure system shows users you value their privacy.
- Strengthens Credibility: Apps with robust authentication systems appear more professional and reliable.
- Meets Legal Standards: Many regulations require apps to implement secure authentication to protect user data.
🔐 Think about it: Would you use an app that doesn’t prioritize security? Neither will your users.
Hands-On: Setting Up Secure Login in Next.js
Let’s get practical and build a secure login flow using NextAuth.js, a flexible and easy-to-use library for authentication in Next.js. Follow these steps to set up a working example of secure login with Google OAuth.
Step 1: Install Dependencies
First, you need to install NextAuth.js:
npm install next-auth
This library handles the heavy lifting of authentication, including session management, secure cookies, and integration with third-party providers like Google.
Step 2: Configure API Routes
NextAuth.js works by defining an API route that acts as the authentication backend.
Create a new file at pages/api/auth/[...nextauth].js
and configure it like so:
import NextAuth from 'next-auth';
import GoogleProvider from 'next-auth/providers/google';
export default NextAuth({
providers: [
GoogleProvider({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
],
secret: process.env.NEXTAUTH_SECRET,
callbacks: {
async session({ session, token }) {
session.user.id = token.sub;
return session;
},
async jwt({ token, user }) {
if (user) {
token.sub = user.id;
}
return token;
},
},
});
Key Points:
- GoogleProvider: Handles OAuth login via Google.
- Callbacks: Allow you to customize JWT and session objects, like adding a user ID to the session.
- NEXTAUTH_SECRET: Adds an extra layer of security, ensuring your authentication tokens are encrypted.
Step 3: Add Login UI
Your users need a way to log in and out. Let’s create a simple UI for this in your pages/index.js
:
import { signIn, signOut, useSession } from 'next-auth/react';
export default function Home() {
const { data: session } = useSession();
return (
<div style={{ padding: '20px', fontFamily: 'Arial, sans-serif' }}>
<h1>Welcome to Next.js Authentication</h1>
{!session ? (
<div>
<p>You are not logged in.</p>
<button onClick={() => signIn('google')}>Login with Google</button>
</div>
) : (
<div>
<p>Hi, {session.user.name}! 🎉</p>
<img src={session.user.image} alt={session.user.name} width={50} style={{ borderRadius: '50%' }} />
<p>Email: {session.user.email}</p>
<button onClick={() => signOut()}>Logout</button>
</div>
)}
</div>
);
}
Key Functions:
signIn
: Redirects the user to the Google login page.signOut
: Logs the user out of their session.useSession
: Fetches the current user’s session data, such as their name, email, and profile picture.
Step 4: Secure Your Environment Variables
To keep sensitive credentials safe, store them in a .env.local
file at the root of your project. Add the following:
GOOGLE_CLIENT_ID=your-google-client-id
GOOGLE_CLIENT_SECRET=your-google-client-secret
NEXTAUTH_SECRET=your-random-secret-key
Generating NEXTAUTH_SECRET
:
You can generate a secure random key using Node.js or any online generator:
node -e "console.log(require('crypto').randomBytes(32).toString('hex'))"
Step 5: Run Your App
Start your development server:
npm run dev
Visit http://localhost:3000 in your browser. You should see your login page. Try logging in with Google and observe how session data appears.
Step 6: Deploy Securely
When deploying your app (e.g., to Vercel), make sure to set the same environment variables in your hosting platform. Without these, authentication won’t work.
Optional Enhancements
- Custom Styling: Use Tailwind CSS or styled-components to enhance the UI.
- Role-Based Access: Extend your session data with roles (e.g., admin, user) and restrict pages accordingly.
- Persistent Sessions: Adjust session expiration to meet your app’s requirements.
Pro Tips From Prateeksha Web Design
At Prateeksha Web Design, we understand that building a secure authentication system is as much about planning as it is about execution. Over the years, we’ve tackled a variety of authentication challenges, and here’s our advice to keep your systems bulletproof and your users happy:
1. Test Like a Hacker
Think like an intruder and test your app for vulnerabilities.
- Tools to Use:
- Postman: Simulate API requests and test edge cases for authentication endpoints.
- OWASP ZAP (Zed Attack Proxy): Scan your app for common vulnerabilities like XSS, SQL injection, and misconfigurations.
- What to Look For:
- Can a user bypass login screens?
- Are tokens or session cookies easily hijacked?
- Are rate limits in place to prevent brute-force attacks?
🔍 Tip: Set up automated security testing in your CI/CD pipeline to catch issues early.
2. Monitor Logs for Suspicious Activity
Authentication isn’t a set-it-and-forget-it feature. Continuous monitoring is crucial.
- Why? Logs can help identify suspicious patterns, such as repeated failed login attempts or access from unusual locations.
- Tools to Consider:
- LogRocket: Tracks user sessions, including login activities, and surfaces anomalies.
- Graylog or ELK Stack: Centralize and analyze logs for deeper insights.
📈 Example: If you see hundreds of failed logins from a single IP address, you might be facing a brute-force attack.
3. Educate Your Users
Even the most secure systems can be compromised by uninformed users.
- Teach Password Hygiene: Encourage strong passwords and discourage reuse across platforms.
- Spot Phishing Attempts: Share tips on identifying fake emails or URLs masquerading as your app.
- Promote Two-Factor Authentication (2FA): Educate users on why enabling 2FA is their best bet against account breaches.
🧠 Remember: An informed user is your first line of defense.
Need Help?
Authentication is a complex field, but you don’t have to tackle it alone. At Prateeksha Web Design, we specialize in building secure, scalable apps with Next.js authentication systems that meet both technical and user-friendly requirements. Whether you’re setting up from scratch or improving an existing setup, we’ve got you covered.
Common Pitfalls to Avoid
Even experienced developers sometimes fall into these traps when implementing authentication. Avoiding them can save you a ton of headaches (and frantic debugging sessions).
1. Skipping Input Validation
Always sanitize and validate user inputs to prevent malicious activities like:
- SQL Injection: Hackers can manipulate database queries through poorly validated inputs.
- XSS Attacks: Injecting malicious scripts into input fields to execute in the user’s browser.
🛠 Solution: Use libraries like validator.js or built-in validation frameworks in your stack.
2. Storing Passwords in Plain Text
This is a rookie mistake with catastrophic consequences. If your database is compromised, plain-text passwords will be an easy target.
- Best Practice: Always hash passwords before storing them.
- Use algorithms like bcrypt or Argon2.
- Add a salt (random string) to make each password hash unique, even for identical passwords.
3. Ignoring Error Handling
Errors are inevitable, but how you handle them can make or break your app’s security.
- Common Mistake: Showing detailed error messages like “Invalid username” or “Incorrect password” gives attackers clues.
- Best Practice: Use generic error messages like “Invalid credentials” to keep attackers guessing while guiding legitimate users.
Wrapping It Up
By now, you should have a solid understanding of secure login in Next.js and how to implement it confidently. Remember, authentication isn’t just a feature—it’s a foundation. Get it right, and you’ll build trust with your users and protect your app from unwanted intruders.
So, what’s your next step? Implement these tips, test your login system, and share your results! And if you need help crafting a secure and scalable Next.js app, reach out to us at Prateeksha Web Design. We’ve got your back!
About Prateeksha Web Design
Prateeksha Web Design specializes in Next.js authentication solutions that prioritize security and efficiency. Their services include implementing robust authentication mechanisms, such as JWT and OAuth, tailored to client needs. They focus on best practices for secure user data handling and access management. Additionally, Prateeksha offers comprehensive training on integrating these secure practices within Next.js applications. Clients benefit from enhanced user trust and streamlined development processes.
Interested in learning more? Contact us today.
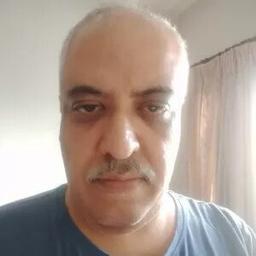