Hey there, future web wizards! 🌟 So, you’ve heard about Next.js, and you're eager to dive into the world of Next.js middleware and API security? Buckle up, because we’re about to embark on a journey that’s not just informative, but fun too! Picture this as a treasure hunt where your map is lined with insights you need to protect your web applications like a pro.
What Exactly Is This Middleware Thing?
Imagine you’re at a carnival – the popcorn smell is enticing, the rides are thrilling, and everywhere you look, there’s something happening. But here’s the catch: the carnival has its way of keeping the fun safe and organized. That’s where middleware comes in. In Next.js, middleware acts as the friendly bouncer at the entrance – checking IDs, ensuring everyone’s of age, and making sure the party stays safe.
In technical terms, middleware is a function that sits between the request and response cycle. It’s like that magical filler in burritos that keeps all the delicious ingredients in place! It processes incoming requests before they reach your API routes, allowing you to implement essential security measures like authentication, rate limiting, and access control.
Why You Should Care About Next.js Middleware
In case you’re still on the fence about the importance of middleware, let’s break it down:
-
API Security: With the rise of cyber threats, solidifying your API security has never been more crucial. Middleware lets you add security measures seamlessly, acting as a first line of defense against malicious activities.
-
Flexible Authentication: Want to verify user identity effortlessly? Middleware does just that. It checks whether users are who they claim to be before granting access to resources. This means users can feel secure, knowing that their data is protected.
-
Rate Limiting Magic: Are bot attacks keeping you awake at night? Middleware can limit the number of requests from a single IP address, preventing those pesky bots from overwhelming your site. This ensures a smooth and stable experience for your legitimate users.
-
Control Access: Middleware allows you to restrict access to certain routes based on user roles. Think of it like a VIP section at a concert – only certain fans get in! This reinforces the principle of least privilege, ensuring users can only access the data and functions they need.
Now that we’re all on the same page, let’s delve deeper into how you can implement these features to elevate your app’s security!
Next.js Middleware: Implementing API Security Step-by-Step
Step 1: Setting Up Your Next.js Project
Before we get into the nitty-gritty details of Next.js middleware, let’s set the stage for our adventure.
npx create-next-app your-next-app
cd your-next-app
Once your app is up and running, you’re ready to start utilizing middleware to enhance your application's security.
Step 2: Introducing Middleware
Creating middleware in Next.js is as easy as pie (or should I say, easier than pie?). You simply need to create a new file named _middleware.js
in your pages directory. This special file is where the magic happens. Let’s say you want to add a layer of authentication. Your middleware might look something like this:
// pages/_middleware.js
import { NextResponse } from 'next/server';
import { verifyJWT } from './utils/auth'; // Your JWT verification function
export function middleware(req) {
const authHeader = req.headers.get('authorization');
if (!authHeader) {
return NextResponse.redirect('/login'); // Redirect if no token present
}
const token = authHeader.split(' ')[1];
try {
const user = verifyJWT(token); // Verify the token
req.user = user; // Attach the user to the request
return NextResponse.next(); // Allow the request to continue
} catch (err) {
return NextResponse.redirect('/login'); // Redirect if verification fails
}
}
Step 3: Implementing Rate Limiting
Rate limiting is another essential feature to mitigate abuse or overuse of your API. You can integrate a simple rate-limiting strategy using a middleware function that tracks requests.
// pages/api/_middleware.js
const rateLimit = (req, res, next) => {
// Define a basic in-memory store for limits (could be replaced with a database)
const ip = req.headers['x-forwarded-for'] || req.connection.remoteAddress;
// Your rate-limiting logic here
// Suppose you have a limit set for requests per minute:
const limit = 100; // Max requests
const timeWindow = 60 * 1000; // 1 minute
// Your implementation for tracking requests from 'ip' and managing counts
return next(); // Proceed to the next middleware/route handler
};
// Usage
export function middleware(req, res) {
rateLimit(req, res, () => {
// your authentication logic or further middleware
});
}
Step 4: Setting Access Control
Access control is your toolset for enforcing user permissions. Here’s how you can implement a robust access control system:
// pages/_middleware.js
export function middleware(req) {
const { role } = req.user; // Assume you've attached user info in the previous middleware
if (req.nextUrl.pathname.startsWith('/admin') && role !== 'admin') {
return NextResponse.redirect('/403'); // Redirect to a 403 Forbidden page
}
return NextResponse.next(); // Allow the request to continue
}
Conclusion: Your Secure Digital Domain Awaits!
And there you have it, aspiring web wizards! With Next.js middleware under your belt, you’re now armed with tools to enhance API security, manage authentication, limit requests, and control access to various resources.
As you continue to explore and experiment, remember that the world of web development is always evolving. Stay curious, and don’t hesitate to continuously refine your approach to security. Your digital domain deserves the best protection possible!
So, get coding, have fun, and may your Next.js projects shine as bright as the stars! 🌟
Detailed Explanation of JWT Verification and Redirection Logic
In a web application, managing user authentication and security is crucial. The following snippet demonstrates how developers can implement a basic JWT verification system typically within a Next.js framework.
try {
const user = verifyJWT(token); // Verify the JWT
req.user = user; // Attach user info to the request object
return NextResponse.next(); // Proceed to the next middleware or handler
} catch (error) {
return NextResponse.redirect('/login'); // Redirect if the token is invalid
}
Explanation of the Code
-
JWT Verification: The
verifyJWT(token)
function is responsible for validating the JSON Web Token. It checks whether the token is well-formed and has not expired. If the verification fails (e.g., the token is missing, malformed, or expired), it throws an error which will be caught by thecatch
block. -
Attaching User Information: If the token is valid, the decoded user information is extracted and stored in
req.user
. This makes the user's identity available throughout the request lifecycle, facilitating access to user-specific data and permissions in subsequent middleware and handlers. -
Proceeding with Request Handling: The
NextResponse.next()
function call signals to the Next.js framework that the middleware has completed its task successfully, allowing the request to proceed to the next middleware function or the final route handler. -
Redirecting to Login: If an error occurs during JWT verification, such as an invalid or absent token, the user is redirected to the login page. This ensures that only authenticated users can access protected routes, maintaining the security of the application.
Analogy: The Bouncer
To visualize this process, think of the middleware as a bouncer at a nightclub. The bouncer checks each guest’s ID (the JWT) before letting them enter. If someone cannot produce valid ID (i.e., if the token fails verification), the bouncer will ensure they do not enter the club, directing them instead to a designated area (the login page).
Step 3: Enhancing Authentication in Next.js Applications
As your application scales and attracts more users, ensuring each individual's identity is essential. Implementing a robust authentication mechanism not only enhances security but also improves user experience.
Popular solutions like Auth0 provide an out-of-the-box authentication service, simplifying the process significantly. They handle everything from token issuance to user management. Alternatively, you can build a custom authentication middleware using libraries like jsonwebtoken for token handling and bcrypt for password hashing.
Security Best Practices
-
Password Storage: Always store user passwords securely. Hashing passwords before storage is a non-negotiable practice; consider using libraries like bcrypt for this purpose. Never store plain-text passwords.
-
Token Expiry: Ensure your JWTs have an appropriate expiration interval to minimize risk. Most JWT libraries allow you to set expiration times upon token creation.
-
Issue Tokens Securely: Tokens should be issued securely and transmitted via HTTPS to reduce the chances of interception.
Step 4: Rate Limiting in Web Applications
Just as personal trainers regulate workout intensity to optimize results, rate limiting is a mechanism that controls the number of requests a user can make to a server in a specified timeframe. This prevents server overload and protects against abuse, such as denial-of-service attacks.
Here’s a basic implementation of rate limiting through middleware:
// pages/_middleware.js
let requestCount = {};
export function middleware(req) {
const ip = req.ip; // Capture user IP address
// Initialize request count for the IP address
if (!requestCount[ip]) {
requestCount[ip] = 1; // Initialize request count
} else {
requestCount[ip]++; // Increment request count
}
// Check if the request count exceeds the limit
if (requestCount[ip] > 100) {
return new Response('Too many requests', { status: 429 }); // Send 429 status response if limit exceeded
}
// Reset count after 1 minute
setTimeout(() => {
requestCount[ip]--; // Decrement request count
}, 60 * 1000);
return NextResponse.next(); // Allow the request to proceed
}
How This Rate Limiting Function Works
-
Tracking Requests: The code uses an object (
requestCount
) to maintain a tally of requests made from distinct IP addresses. When a request comes in, it checks if an entry for the requesting IP exists, initializing it if not. -
Limit Enforcement: If the request count for an IP exceeds 100 within a minute, the middleware responds with a 429 status code, indicating "Too many requests." This mechanism prevents abuse and ensures fair use of server resources.
-
Count Resets: The
setTimeout
function resets the count after one minute, allowing legitimate users to make requests again once the limit period lapses.
Step 5: Mastering Access Control
Access control is a vital part of securing your applications. It involves ensuring that users can only perform actions or access resources that they are authorized for.
Techniques for Access Control
-
Role-Based Access Control (RBAC): Users are assigned roles, and these roles dictate what resources or actions users can access. For instance, admin users may have access to manage settings while regular users can only view content.
-
Attribute-Based Access Control (ABAC): Access permissions are granted based on attributes (user attributes, resource attributes, environment attributes) rather than fixed roles. This method provides more granular control over access.
-
OAuth Scopes: When integrating third-party services (like Google or Facebook for sign-in), you may use OAuth scopes to limit the access tokens to specific actions on behalf of the user.
-
Security Reviews and Continuous Testing: Regularly review your access control logic, particularly after making changes to your user roles or permissions model. Automated tests can help in identifying potential vulnerabilities arising from new features or changes.
By implementing these strategies, you can enhance your Next.js application's security, ensuring a robust, user-friendly experience while protecting sensitive user information.
Not Everyone Should Have the Same Access!
In today’s digital landscape, security and privacy are paramount. Just as exclusive clubs only allow certain individuals to enter, applications too must implement controlled access to ensure that sensitive areas are only accessible to those who belong. As a developer, it is your responsibility to ensure that only authorized personnel can access specific sections of your application.
Think of it like a private members-only club: the entrance is monitored, and access is restricted to a select group of members who meet specific criteria. This approach safeguards your application from unauthorized users and potential security breaches.
Role-Based Access Control: A Practical Example
One effective way to manage access control in your application is through role-based access control (RBAC). This strategy assigns permissions based on user roles, allowing you to define what each user can or cannot do within the application.
Below is a simplified example using JavaScript middleware in a Next.js application:
// pages/_middleware.js
import { NextResponse } from 'next/server';
export function middleware(req) {
const userRole = req.user?.role; // Assumes user information comes from authentication
// Check if the user is trying to access an admin route
if (req.nextUrl.pathname.startsWith('/admin') && userRole !== 'admin') {
return new Response('Access Denied', { status: 403 }); // Respond with a forbidden status
}
// If access is granted, continue with the request
return NextResponse.next();
}
In this code snippet, we are utilizing a middleware function that captures incoming requests. It checks if the user attempting to access any route starting with /admin
is indeed an administrator. If they are not, the system returns a 403 Forbidden response, effectively blocking access. This is a fundamental yet powerful way to ensure that only users with the designated 'admin' role can enter the administrative section of your application.
BONUS: Organizing Middleware for Maximum Efficiency
Feeling adventurous? You can combine multiple access control rules into a single middleware file, thereby creating a robust, centralized access control system. However, it's essential to keep your code organized – think of it as a blueprint for maintaining clarity within your codebase. Structure is your secret weapon in avoiding confusion and ensuring long-term sustainability.
Next.js Data Protection: Best Practices
While middleware significantly bolsters your API security, there are additional strategies that you should implement to further safeguard your application environment:
-
Use HTTPS: Always serve your application over HTTPS. This acts as an invisible cloak that encrypts data in transit, protecting it from potential eavesdroppers.
-
Validate Input: Treat user inputs with caution. Always validate and sanitize to guard against common vulnerabilities such as SQL injection and cross-site scripting (XSS).
-
Keep Dependencies Updated: Maintaining up-to-date libraries and frameworks is akin to keeping your body’s vaccinations current. An outdated application is susceptible to security gaps and vulnerabilities.
-
Implement CORS: Cross-Origin Resource Sharing (CORS) is an important control mechanism that dictates which domains are permitted to interact with your API. It’s like maintaining a guest list – only verified origins should be allowed in.
-
Use Environment Variables: Store sensitive information, such as API keys and database connection strings, in environment variables. This practice keeps your secrets out of your source code, reducing the risk of exposure.
Crafting a Stunning Next.js Application
While functionality is crucial, aesthetics matter too. A visually appealing application can significantly enhance user engagement rates. Collaborating with professionals such as Prateeksha Web Design can help elevate your project's design quality. Based in Mumbai, Prateeksha Web Design specializes in ecommerce website design in Mumbai and provides premium web design services that bolster your brand's presence online.
Why Choose Prateeksha Web Design?
- Expertise: With a proficient team of Mumbai website designers, they ensure that your website doesn’t just function well, but also captivates users visually.
- Tailored Solutions: Whether you need to develop a website in Mumbai or focus on ecommerce website development, they tailor solutions to align with your specific business goals.
- Support: Should you require assistance, the dedicated support team at Prateeksha is always ready to lend a helping hand!
Wrapping It Up with a Bow 🎁
Congratulations! Armed with the essential knowledge of Next.js middleware for securing APIs, you are now ready to embark on your web development journey. Remember, middleware is like a loyal partner in safeguarding your application. Focus on implementing robust authentication measures and providing your users with a seamless experience.
So, what's next? Begin building your application! Put these insights into practice, experiment with middleware features, and remember to prioritize design. In a competitive digital world, delivering an application that balances functionality with aesthetic appeal will make all the difference in engaging and retaining users. Happy coding!
Tip: Utilize built-in Next.js middleware to handle authentication and authorization for your API routes efficiently. Implementing middleware at the edge allows you to run code before a request is completed, enabling you to verify tokens or session data before proceeding with API logic.
Fact: Next.js middleware runs on the Edge Network, which means it can respond to requests much faster than traditional server-side logic. This leads to lower latency and improved performance for secure API calls since the middleware is executed before reaching the application server.
Warning: Be cautious when implementing custom middleware; improperly configured middleware can inadvertently expose your API to security vulnerabilities. Ensure that you validate all inputs and securely manage sensitive data (like credentials or tokens) to prevent unauthorized access or data leaks. Always test your middleware thoroughly in a staging environment before deploying to production.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services in Next.js middleware implementation to enhance API security for developers. Their expertise includes creating optimized middleware solutions that protect against vulnerabilities while maintaining performance. Clients receive tailored guidance on best practices for secure API interactions. Additionally, Prateeksha provides comprehensive support and training to empower teams in leveraging Next.js effectively. This ensures a robust framework for building secure web applications.
Interested in learning more? Contact us today.
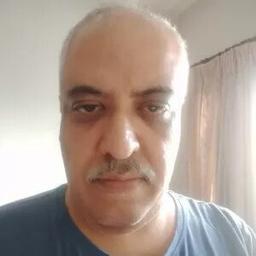