Version control is critical in software development, providing a systematic way to manage changes in your codebase. Without version control, working on a project with multiple contributors—or even managing your own work across different devices—can quickly become chaotic. Two indispensable tools in this realm are Git, a distributed version control system, and GitHub, a platform that builds on Git to provide a robust environment for collaboration, code review, and project management.
This guide explores advanced Git techniques and GitHub workflows, offering actionable insights to help developers and small businesses optimize their workflows. By mastering these tools, you can unlock their full potential, from advanced branching strategies to integrating automation with GitHub Actions. We’ll also highlight Prateeksha Web Design’s expertise in leveraging these tools to deliver seamless solutions for small businesses.
Why Master Git and GitHub?
Whether you’re a solo developer or part of a large team, Git and GitHub play a pivotal role in your workflow. Here’s why mastering these tools is essential:
1. Streamline Collaboration Among Teams
Modern development often involves teams working across various locations and time zones. Git and GitHub provide features that make collaboration intuitive and organized:
- Branching and Merging: Developers can work on features independently in branches and merge changes into the main codebase when ready.
- Pull Requests: Team members can review, comment on, and approve changes before they are integrated.
- Conflict Resolution: Git highlights conflicts when two developers modify the same part of a file, allowing them to address the issue collaboratively.
For example, a team at Prateeksha Web Design might use GitHub’s collaboration features to streamline a website design project, ensuring everyone stays on the same page.
2. Ensure Seamless Version Control
Git enables developers to maintain a complete history of every change made to their codebase. This history is invaluable for:
- Rolling Back Changes: If a bug is introduced, you can revert to a previous stable version.
- Tracking Code Evolution: Understand why and how changes were made over time by examining commit logs.
- Experimentation: Safely explore new ideas by creating feature branches without affecting the main codebase.
For example, a small business using Prateeksha Web Design’s services might benefit from version control when testing new features for their Shopify store. Any unwanted changes can easily be undone without disrupting the live website.
3. Enable Effective Troubleshooting of Issues
Debugging and troubleshooting are critical aspects of software development. Git and GitHub simplify these processes by:
- Allowing developers to pinpoint the exact commit where an issue was introduced using tools like git bisect.
- Providing issue tracking systems on GitHub that help organize and resolve bugs efficiently.
- Keeping a detailed history of changes, which makes it easier to trace the origins of a problem.
For instance, if a small business’s e-commerce site encounters a performance issue, Prateeksha Web Design can use Git’s history to identify and fix the problem quickly.
4. Manage Large-Scale Projects Efficiently
As projects grow, managing them becomes more complex. Git and GitHub offer tools to tackle these challenges:
- Monorepo Support: Git can handle large repositories with multiple subprojects.
- GitHub Projects: A project management tool that integrates seamlessly with your repository to track tasks, milestones, and progress.
- Git LFS (Large File Storage): Manage large files like images or videos without bloating your repository.
For a roofing business website designed by Prateeksha Web Design, Git and GitHub might be used to manage various assets, such as high-resolution images, scripts, and templates, ensuring the project remains organized.
Advanced Git Commands and Techniques
When you move beyond the basics of git add
, git commit
, and git push
, Git reveals its true potential. Here are some advanced commands and strategies to enhance your workflow.
1. Git Rebase vs. Git Merge
While merging is a common way to integrate branches, rebasing offers a cleaner commit history.
- Git Merge: Preserves the entire history, including merge commits, which can clutter the log.
- Git Rebase: Reapplies commits from one branch onto another, creating a linear history.
Example Usage:
git checkout feature-branch
git rebase main
However, use rebasing cautiously in shared branches to avoid conflicts. A good rule is: Rebase locally, merge publicly.
2. Stashing Work-in-Progress
Ever had to switch branches while working on incomplete changes? Git stash temporarily saves your work without committing.
- Command:
git stash
- Restore it later with:
git stash apply
For better organization, name your stashes:
git stash save "WIP: feature X"
3. Interactive Rebase for Clean Histories
Want to rewrite history? Interactive rebasing allows you to edit, squash, or reorder commits.
- Command:
git rebase -i HEAD~n
Replace n
with the number of commits you want to modify.
- Use it to squash minor commits, making your project history professional and readable.
4. Using Aliases for Efficiency
Speed up your workflow by creating shortcuts for common commands:
- Example:
git config --global alias.co checkout
git config --global alias.br branch
Instead of typing git checkout
, simply use git co
. Aliases save time and reduce errors.
Optimizing GitHub Workflows
GitHub workflows by Program Geeks are designed to enhance collaboration and automation. Let’s explore strategies to get the most out of GitHub.
1. Branching Strategies
Adopting a structured branching strategy like GitFlow, Feature Branching, or Trunk-Based Development ensures better project management.
- GitFlow: Uses feature, develop, and release branches for complex projects.
- Trunk-Based Development: Focuses on keeping the main branch updated with small, frequent changes.
2. GitHub Actions for Automation
GitHub Actions is a powerful CI/CD tool that automates tasks such as testing, deployment, and notifications.
- Example Workflow File:
name: CI Pipeline
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Run Tests
run: npm test
Benefits:
- Automate repetitive tasks.
- Ensure code quality with pre-merge checks.
- Deploy code with minimal effort.
3. Code Reviews and Pull Requests
Code reviews are critical for maintaining high-quality code. Follow these best practices:
- Use templates for pull requests to standardize reviews.
- Require approvals from specific team members.
- Automate checks with GitHub Actions.
4. GitHub Advanced Search
Finding issues, PRs, or code snippets is easy with GitHub's advanced search filters.
- Search within a repository:
repo:owner/repository "search-term"
- Filter issues by label:
is:issue label:bug
This feature is especially useful for large repositories.
Version Control Best Practices
Maintaining a robust version control system requires discipline. Here are the best practices for version control with Program Geeks:
1. Write Clear Commit Messages
A good commit message explains what and why in a concise format. Use the following structure:
<type>(scope): <description>
[optional body]
[optional footer]
For example:
fix(auth): resolve login timeout issue
2. Avoid Committing Secrets
Never commit sensitive information like API keys. Use tools like GitGuardian to scan for potential leaks.
3. Utilize Tags for Releases
Tagging specific commits helps organize your project. Use annotated tags for detailed release information:
git tag -a v1.0.0 -m "Initial release"
git push origin --tags
Recent Advancements in Git and GitHub
Both Git and GitHub are constantly updated to address the evolving needs of developers, making them more efficient, powerful, and user-friendly. These advancements not only improve productivity but also enhance collaboration, security, and project management. Below, we delve into three notable recent features that have significantly impacted how developers use these tools.
1. GitHub Copilot: The AI-Powered Coding Assistant
GitHub Copilot, powered by OpenAI's GPT technology, is a revolutionary AI tool designed to streamline coding. Acting as an intelligent code assistant, it suggests code snippets and solutions in real time, based on the context of your code.
How GitHub Copilot Works
- Context Awareness: Copilot understands the code you're working on and suggests relevant snippets. For instance, if you're writing a function in Python, it can autocomplete the function or suggest an implementation.
- Multi-Language Support: Copilot supports several programming languages, including Python, JavaScript, TypeScript, Ruby, Go, and more.
- Integration: It works seamlessly with popular IDEs like Visual Studio Code, JetBrains, and GitHub Codespaces.
Benefits of GitHub Copilot
- Improved Efficiency: By reducing the time spent writing boilerplate code, developers can focus on solving complex problems.
- Error Reduction: Copilot often suggests code that adheres to best practices, helping you avoid common pitfalls.
- Learning Tool: For newer developers, it provides examples and suggestions that can aid learning.
Example in Action
Suppose you’re working on a function to calculate factorials in Python. Copilot might suggest:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
Impact for Businesses: Small businesses, like those working with Prateeksha Web Design, can use Copilot to accelerate project timelines, reduce costs, and improve code quality.
2. Git Sparse-Checkout: Optimize Repository Management
Large repositories often contain directories and files that are irrelevant to all contributors. Git Sparse-Checkout allows developers to download and work with only specific parts of a repository, significantly reducing disk usage and speeding up operations.
How Sparse-Checkout Works
Sparse-checkout enables you to select specific directories or files to include in your working directory while excluding others.
- Command to Enable Sparse-Checkout:
git sparse-checkout init
- Selecting Specific Directories:
git sparse-checkout set path/to/directory
Benefits of Sparse-Checkout
- Efficiency: Developers working on large monorepos can avoid downloading unnecessary files, saving bandwidth and disk space.
- Faster Cloning and Pulls: By reducing the size of the working directory, operations like cloning, pulling, and fetching are faster.
- Streamlined Focus: Developers can focus only on the parts of the project they’re working on.
Real-World Application
For example, in a project with separate modules for frontend, backend, and documentation, a backend developer can use sparse-checkout to only clone the backend folder.
Impact for Businesses: By using sparse-checkout, teams can better manage large repositories, making collaboration more efficient—something that Prateeksha Web Design utilizes to handle complex projects with ease.
3. Enhanced Security Features: Dependabot and More
Security is a top priority for developers, and GitHub has introduced several features to ensure repositories are secure. One of the standout features is Dependabot, which automates dependency updates to address vulnerabilities.
Dependabot’s Role
Dependabot scans your repository for outdated or insecure dependencies and creates pull requests to update them.
- Automated Security Updates: If a vulnerability is detected in a dependency, Dependabot automatically creates a pull request to update it to a secure version.
- Customizable Alerts: Developers can configure Dependabot to notify them of vulnerabilities via GitHub notifications or email.
- Integration with Code Scanning: Dependabot integrates with GitHub’s advanced code scanning tools to provide a holistic view of repository security.
Additional Security Enhancements
- Secret Scanning: Alerts you if sensitive information (like API keys or credentials) is accidentally committed to the repository.
- Code Scanning: Runs static analysis tools to detect vulnerabilities in your codebase.
Benefits of Enhanced Security Features
- Reduced Manual Effort: Automating dependency updates saves time for developers.
- Proactive Issue Resolution: Fixing vulnerabilities before they’re exploited ensures better protection.
- Improved Trustworthiness: Secure repositories build trust with clients and end-users.
Real-World Application
Suppose a project uses an outdated version of a library with a known security flaw. Dependabot identifies the issue, proposes a solution, and provides a pull request to update the library, ensuring the project remains secure.
Impact for Businesses: By leveraging these security features, Prateeksha Web Design can ensure that client projects are robust and safe from vulnerabilities. This is especially crucial for e-commerce platforms or any project handling sensitive user data.
Why Choose Prateeksha Web Design?
At Prateeksha Web Design, we integrate advanced version control techniques into our projects. Our expertise ensures that:
- Your codebase remains clean and organized.
- Collaborative efforts are seamless and productive.
- Small businesses gain access to cutting-edge development workflows.
We leverage tools like GitHub Actions, GitHub Copilot, and advanced Git strategies to streamline your digital projects.
Call-to-Action: Let’s Build Your Future Together
If you’re a small business looking to adopt efficient version control practices, Prateeksha Web Design is your partner for success. Whether it’s web design, e-commerce solutions, or digital strategy, our team ensures your business stays ahead in the digital landscape. Contact us today and let’s discuss how we can elevate your projects.
By applying these Git tips from Program Geeks and embracing advanced GitHub workflows, you’ll master the art of version control, ensuring your projects are efficient, secure, and ready for future growth.
About Prateeksha Web Design
Prateeksha Web Design offers advanced training in mastering Git and GitHub for efficient version control in web development projects. Our services include in-depth tutorials on branching, merging, rebasing, and resolving conflicts in Git. We also provide hands-on practice in using advanced features like Git hooks, interactive rebase, and cherry-picking for better project management. Our expert trainers will guide you in optimizing your workflow and maximizing the benefits of version control with Git and GitHub.
Interested in learning more? Contact us today.
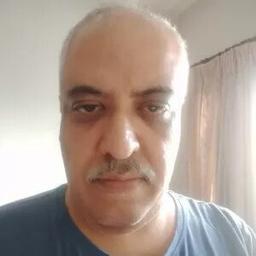