Hello there! 🎉 Are you prepared to explore the exhilarating world of server-side authentication with Next.js and NextAuth.js? You’re in for an incredible adventure! Fear not; I am here to assist you every step of the way. In this guide, we will delve deep into the architecture of a comprehensive authentication system that not only secures your application but also enhances the user experience significantly. So, grab your gear as we transform your Next.js project into a robust fortress! 🏰
Why Next.js Authentication Matters
Before we plunge into the practical coding aspects, let's clarify why Next.js authentication is essential for any application. Picture stepping into a nightclub where a bouncer meticulously checks IDs at the door—only those who pass the identification test can revel in the festive atmosphere. In a similar fashion, user authentication serves as the gatekeeper of your application, ensuring that only authorized users gain access to sensitive areas. This creates a sense of security and trustworthiness for your users, safeguarding their valuable data.
Next.js empowers developers to build ultra-fast, server-rendered web applications that provide an exceptional performance and user experience. When you combine that with NextAuth.js, you acquire an essential toolkit that efficiently manages all your API authentication needs. 🪄✨
Key Takeaways
- Enhances Security: Effective authentication mechanisms shield your application from unauthorized access, diminishing potential security threats.
- Improves User Experience: A seamless login workflow contributes to user satisfaction, helping retain and engage users effectively.
- Scalable Solutions: With NextAuth.js, adapting to a growing user base becomes a hassle-free endeavor, accommodating various providers and configurations.
Setting Up Your Next.js Project
Let’s dive into the practical setup! First and foremost, ensure that you have Node.js and Next.js installed on your machine. If you haven't completed this step yet, open your terminal and execute the following commands:
npx create-next-app your-app-name
cd your-app-name
npm install next-auth
Congratulations! You now have your Next.js project initialized and ready to work with NextAuth.js. But hang tight, we’re just getting started! 🔥
Understanding NextAuth.js
NextAuth.js is a strikingly powerful library specifically designed for Next.js applications. It adds a layer of authentication that simplifies the process of signing in users through various OAuth providers such as Google, Facebook, GitHub, and more! You can think of it as an all-you-can-eat buffet when it comes to login options—users can choose their preferred method to access your application, significantly boosting its appeal.
Quick Overview of OAuth
If you’re unfamiliar with OAuth, allow me to simplify it for you. Imagine it as a VIP pass that gives users the ability to grant limited access to third-party applications without revealing their sensitive credentials. This means users can effortlessly log in to your app using credentials from familiar and trusted services such as Google or GitHub, enhancing their overall experience.
Integrating NextAuth.js into Your App
Now for the exciting part—let's integrate NextAuth.js into your application with some code! To kick off, first create a directory under your project named pages/api/auth. Inside that folder, add a file titled [...nextauth].js—this is where the magic unfolds. 🪄
Here’s a simplified configuration to get you started:
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_ID,
clientSecret: process.env.GOOGLE_SECRET,
}),
// Additional providers can be added here!
],
database: process.env.DATABASE_URL,
});
Environment Variables
Wait a moment! It’s imperative to set up some environment variables for this configuration to operate correctly. Create a .env file in the root of your project directory and insert your Google credentials:
GOOGLE_ID=your-google-client-id
GOOGLE_SECRET=your-google-client-secret
DATABASE_URL=your-database-url
Why Use Environment Variables?
Think of environment variables as the secret spices in your grandmother's famous recipe. They keep sensitive information—like API keys and credentials—away from prying eyes. Moreover, they allow for easier management of different environments (development, staging, production), making your application more adaptable and secure.
Implementing Secure Login with NextAuth.js
With NextAuth.js now configured, let’s embark on implementing a secure login feature for your application.
Building the Login Page
You’ll want to create a simple login page where users can initiate the authentication process. The following is a basic example of a login button that leverages NextAuth.js:
import { signIn } from 'next-auth/client';
const Login = () => {
const handleLogin = () => {
signIn('google'); // Initiates login with Google
};
return (
<div>
<h1>Welcome to My App</h1>
<button onClick={handleLogin}>Login with Google</button>
</div>
);
};
export default Login;
Handling User Session
Once a user logs in successfully, it is essential to manage their session effectively. NextAuth.js provides a way to retrieve session details anywhere in your app by utilizing the useSession
hook. Here’s an example of how to implement session management:
import { useSession } from 'next-auth/client';
const Dashboard = () => {
const [session, loading] = useSession();
if (loading) return <div>Loading...</div>;
if (!session) return <div>You must be logged in to view this page.</div>;
return (
<div>
<h1>Welcome, {session.user.name}!</h1>
<p>Your email: {session.user.email}</p>
</div>
);
};
export default Dashboard;
Conclusion
As you can see, integrating authentication into your Next.js application using NextAuth.js is both simple and powerful. By leveraging this duo, you can provide a secure gateway for your users while ensuring a seamless experience. Next, you can explore additional features and integrations that NextAuth.js offers, such as custom sign-in pages, database adapters, and more robust session handling.
Now that you're equipped with the knowledge of server-side authentication, it’s time to get coding! Empower your applications, safeguard your user data, and take your Next.js projects to the next level. Happy coding! 🚀
Creating the Login Page
To initiate your user authentication process in a Next.js application, the first step is to create a Login page. This page will serve as the gateway for users to authenticate themselves. In your project’s pages folder, create a new JavaScript file named login.js. Here’s a detailed example of how that file may be structured:
import { signIn } from 'next-auth/client';
const Login = () => {
return (
<div>
<h1>Login</h1>
<button onClick={() => signIn('google')}>Log in with Google</button>
</div>
);
};
export default Login;
Explanation of the Code
-
Import Statement: The code starts by importing the
signIn
function from thenext-auth/client
. This function is essential for handling authentication through various providers, in this case, Google. -
Login Component: A functional component named
Login
is defined. This component renders a simple UI that includes a heading and a button. -
Sign-In Logic: The button utilizes an
onClick
handler that triggers thesignIn
function when clicked. The argument passed tosignIn
indicates the OAuth provider being used—in this instance, Google. -
Export Statement: Finally, the
Login
component is exported as the default export from the file, making it accessible for routing in your Next.js application.
When users click the "Log in with Google" button, they are redirected to the Google OAuth interface to enter their credentials and authorize the application, thus handling the authentication process seamlessly. This user-friendly approach enhances user experience, as it streamlines the login process.
Sign-In Button Logic
The signIn()
function from NextAuth.js abstracts much of the complexity of managing user authentication. Upon clicking the sign-in button:
-
Redirection: The user is redirected to the Google authentication page.
-
Authentication Flow: Here, they will input their credentials, which triggers the OAuth flow managed by Google.
-
Session Management: Once authenticated, the user will be redirected back to your application with a session token, effectively logging them in.
This method not only simplifies the authentication process but also provides a secure means to allow user access to your application. It is particularly useful as it offloads the responsibility of handling sensitive credential data to the OAuth provider.
Exploring Next.js Middleware for User Authentication
In web development, middleware plays a crucial role in managing user sessions and security. Think of middleware as a checkpoint; it allows you to execute code before proceeding with the user’s request. This is especially handy for user authentication and ensuring that only authorized users can access certain routes within your application.
Implementing Middleware
To create a middleware function, you will need to set up a new file named middleware.js in your project’s root directory. The following snippet shows how to implement a middleware function that protects specific routes:
import { getSession } from 'next-auth/client';
export default async function middleware(req, res, next) {
const session = await getSession({ req });
if (!session) {
// Redirect to login if not authenticated.
return res.redirect('/api/auth/signin');
}
next();
}
Explanation of the Middleware Logic
-
Session Retrieval: The middleware function uses
getSession
to check if there is an existing user session based on the incoming request. -
Authentication Check: If there is no session (which means the user isn't logged in), the middleware will redirect the user to the login page (
/api/auth/signin
). -
Proceeding with Request: If the session is valid,
next()
is called, allowing the request to continue to the intended route.
Middleware Benefits
Implementing this middleware provides a strong layer of security by requiring users to authenticate before accessing protected routes. Furthermore, it allows for flexible route management where you can define which pages should be accessible only to logged-in users.
Next.js JWT: Extra Protection with Tokens
In addition to the session-based authentication that NextAuth.js provides, you might want to consider leveraging JSON Web Tokens (JWT). JWTs offer a stateless method for authenticating users which can enhance scalability in larger applications.
Setting Up JWT in NextAuth.js
To incorporate JWT into your application, configurations must be made within your NextAuth.js setup. Below is an example configuration:
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
import jwt from 'next-auth/jwt';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
// Additional providers can be added here
],
callbacks: {
async jwt(token, user) {
// If a user is passed, set the user ID on the token
if (user) {
token.id = user.id;
}
return token;
},
async session(session, token) {
// Attach user ID from the token to the session
session.user.id = token.id;
return session;
},
},
// Additional configuration options can be added here
});
Understanding the JWT Implementation
-
Provider Setup: In the
providers
array, you specify the authentication providers you wish to use—in this case, Google. -
JWT Callback: The
jwt
callback function is invoked whenever the JWT token is created. If a user object is available (indicating that the user has just signed in), the user’s ID is added to the token. -
Session Callback: The
session
callback is responsible for adding the user ID from the token to the session object, allowing you to access it once the user is logged in.
Benefits of Using JWT
- Stateless: JWTs do not require server-side sessions, making them scalable for large applications where server memory may be a concern.
- Flexibility: Since JWTs are self-contained, they can transmit additional information (such as user roles) in the payload.
- Cross-Domain: JWT can be shared between domains, enhancing interoperability across different applications or services.
By integrating JWT into your authentication strategy, you boost both the security and flexibility of your user management system within your Next.js application. This layering of security practices allows you to build a robust authentication mechanism that scales with your needs.
Certainly! Let's expand, explain in detail, and refine the provided content on integrating Next.js with NextAuth.js for user authentication and the importance of a well-crafted web design.
// Configuration example for NextAuth.js
const authOptions = {
providers: [
// Define your authentication providers here (e.g., Google, GitHub)
],
callbacks: {
async signIn(user, account, profile) {
// Custom sign-in logic, e.g., fetching user data from API
const res = await fetch("/your-api-endpoint", {
method: "POST",
body: JSON.stringify(user),
headers: { "Content-Type": "application/json" },
});
const userData = await res.json();
return userData; // Return true or false based on the success of sign-in
}
},
secret: process.env.SECRET,
};
Why Use JWT (JSON Web Tokens)?
Adopting JWT in conjunction with NextAuth.js revolutionizes your application's authentication mechanism. JWTs are compact, self-contained tokens that encapsulate the user's identity and any relevant permissions. Here are the main advantages of using JWTs:
-
Stateless Authentication: With JWT, each token contains all necessary information for validating user claims, which eliminates reliance on server-side sessions. This means the server doesn’t need to keep track of user sessions, leading to a significant reduction in server load and enhanced scalability.
-
Enhanced Performance: Since each authentication request carries the user information, you minimize round-trip requests to the server for session validation. This can significantly speed up responses, especially if your application scales up with a higher number of users.
-
Better User Experience: JWT’s ability to maintain user state across different sessions seamlessly enhances the user experience. Users can stay logged in across the application without the need for frequent reauthentication.
Integrating Next.js with a Backend for Enhanced User Data Management
As you explore deeper functionalities, you might want to consider integrating your Next.js application with a backend for effective user data management. This integration empowers users to manage their profiles, update information, and customize their experiences according to their preferences.
Setting Up Your Backend Environment
To keep things straightforward, you might want to opt for a local database setup or leverage cloud solutions such as Firebase. Regardless of your choice, connecting the backend to NextAuth.js is essential for storing and retrieving user information efficiently.
Database Options:
- Local Database: You can use SQLite or MongoDB for development purposes.
- Cloud Services: Platforms like Firebase or AWS DynamoDB offer scalable database solutions that manage user data in real time.
Storing User Information on Login
To efficiently save user details during login, you can enhance your NextAuth.js configuration with a secure callback function. Here’s how you can achieve this:
callbacks: {
async signIn(user, account, profile) {
const res = await fetch("/api/saveUser", {
method: "POST",
body: JSON.stringify({ email: user.email, name: user.name }), // Customize as needed
headers: { "Content-Type": "application/json" },
});
const userData = await res.json();
// Return true to continue sign-in process or false if there was an error
return userData.success;
}
}
The Complete User Experience
By leveraging backend capabilities, you can create a more dynamic and engaging app. Users can interact with personalized elements, receive notifications, manage their profiles, and enjoy tailored content.
Creating a Cohesive Authentication System: An Overview
Let’s consider the architecture of your application as a multi-level building focused on providing users a secure and efficient experience:
-
The Bouncer (Authentication): Acts as the first line of defense, verifying user credentials before allowing access into your application.
-
The VIP Lounge (Protected Routes): This exclusive area is only accessible to authenticated users, safeguarding sensitive information and functionalities.
-
Surveillance (Middleware): Middleware functions track user activity and permissions, ensuring users can only access what they are entitled to, enhancing security.
-
The Vault (Database): Your secure storage solution where user data, preferences, and application-related content are kept safe and accessible only when needed by valid authenticated sessions.
With this robust structure in place, you're well-equipped to build a secure and user-friendly web application using Next.js with NextAuth.js for authentication.
The Role of Leading Web Design Companies in Mumbai: Elevating Your App's Aesthetic
If you're aiming to elevate your application’s look and feel to match its functionality, partnering with a top web design company in Mumbai like Prateeksha Web Design can be a game-changer. They specialize in creating visually stunning and user-friendly interfaces for eCommerce websites, ensuring your application stands out.
Imagine this scenario: your application features an efficient authentication system coupled with a sleek design, impressing your users right from the start.
Why Collaborate with Prateeksha Web Design?
-
Expertise and Experience: Their years of experience in crafting user-centered designs guarantee that your application will not only function well but resonate with users aesthetically.
-
Custom Solutions for Unique Needs: They prioritize understanding your specific requirements and create tailored solutions to make your app user-friendly and visually appealing.
-
Comprehensive Service Offering: Their capabilities span from web design to backend integration, providing end-to-end solutions that cover every aspect of your application's development.
Wrapping Up Your Learning Journey
Congratulations on mastering the essentials of server-side authentication in Next.js using NextAuth.js! You’ve gathered insights into implementing secure authentication practices, enhancing user experiences, and the importance of robust web design.
Final Reflections
With this newfound knowledge, I encourage you to take the plunge and start developing your web application. Remember that every distinguished application stems from a brilliant idea paired with the right motivation to bring it to fruition. Whether crafting a simple application or a complex digital platform, establishing a strong authentication system is imperative to your success.
So, roll up your sleeves, let your creativity flow, and most importantly, enjoy the journey! Happy coding! 🚀
Tip
Ensure you regularly update your NextAuth.js library and its dependencies to leverage the latest security features and enhancements. Stay informed about changes in authentication standards and library updates to keep your application secure and efficient.
Fact
NextAuth.js supports a wide variety of authentication providers, including OAuth providers like Google and GitHub, as well as email/password authentication. This flexibility makes it easier to implement robust and user-friendly authentication flows tailored to your application's needs.
Warning
Avoid exposing sensitive information in your authentication tokens or session data. Always store tokens securely and implement proper session management to prevent vulnerabilities such as session hijacking or replay attacks. Make sure to enable HTTPS in your production environment to protect data in transit.
About Prateeksha Web Design
Prateeksha Web Design specializes in implementing robust server-side authentication solutions in Next.js applications using NextAuth.js. Their services include seamless integration of user authentication workflows, providing secure session management, and enabling various authentication providers. They ensure optimal performance and security while customizing authentication features to meet specific client needs. With a focus on user experience, they help streamline login processes and protect sensitive data. Trust Prateeksha for expert guidance and implementation of Next.js authentication best practices.
Interested in learning more? Contact us today.
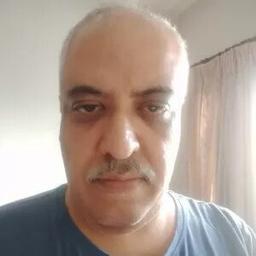