Introduction to Internationalization (i18n)
What Is Internationalization (i18n)?
The term internationalization (often abbreviated as i18n) is a clever way to condense the word. The "i18n" abbreviation represents the 18 characters between the "i" and the "n" in the word "internationalization." This concept involves designing and developing software applications, websites, or systems in a way that makes it easy to adapt them for multiple languages, regions, or cultural norms without major engineering changes.
Think of i18n as preparing a house for guests from different parts of the world. Instead of renovating the house every time a guest arrives, you set up an adaptable space where small, easy changes (like adjusting furniture or adding decorations) make each guest feel at home.
Why Is Internationalization Important in Today’s World?
The internet has brought people closer than ever. A single website can serve users in different countries, cultures, and languages. As businesses expand beyond their local markets, multilingual websites have shifted from being optional to a critical component of success. Here’s why:
-
User Expectations: Most users prefer interacting with websites in their native language. Offering content in the user’s language creates a sense of trust and comfort, making them more likely to stay on your site and convert into customers.
-
Diverse Markets: Whether you're running a blog, an e-commerce store, or a SaaS platform, having multilingual capabilities helps you connect with diverse demographics.
-
Global Accessibility: Not all users understand English or share the same cultural norms. Internationalization bridges this gap, allowing you to provide tailored content that resonates with users worldwide.
Internationalization in Web Development
For developers, i18n is a process that allows an application to:
- Support multiple languages without rewriting its core.
- Handle region-specific formats, such as dates, times, and currencies.
- Maintain flexibility for future expansions, like adding new languages or adapting to cultural preferences.
Modern tools and frameworks like Next.js simplify this process, providing built-in capabilities to implement i18n with less effort.
Why Internationalization Matters
1. Global Reach
Imagine you're running an online store that sells handmade crafts. While your primary audience might be in your country, there could be potential customers in Spain, Germany, or Japan who would love your products. By supporting their languages, you remove barriers to entry, making it easier for them to browse, understand, and buy.
2. Improved User Experience
The user experience (UX) is greatly enhanced when visitors interact with content in their native language. It makes navigation intuitive, reduces confusion, and builds trust. For example, a Japanese customer browsing a website in English might hesitate to make a purchase due to misunderstanding terms or policies. Localizing the website into Japanese creates a smoother, more personalized experience.
3. SEO Benefits
Search engines like Google rank content based on relevance and user intent. When your website offers localized content, you’re not just targeting keywords in one language—you’re optimizing for multiple regional searches. Using multilingual SEO practices like hreflang
tags helps search engines understand which version of your content is intended for specific regions, boosting visibility in those areas.
For example:
- A page targeting “handmade gifts” in English might rank for U.S. searches.
- A Spanish version optimized for “regalos hechos a mano” would appear for users in Spain or Latin America.
4. Competitive Advantage
Offering localized content isn’t just about serving users better—it’s a strategic advantage. If your competitors are only catering to English-speaking audiences, supporting multiple languages allows you to capture markets they’ve ignored. It positions your brand as more inclusive, professional, and globally aware.
How Next.js Helps with Internationalization
Next.js, one of the most popular React-based frameworks, provides native support for i18n, making it easier than ever for developers to create multilingual websites. This is especially important for businesses that want to focus on scaling their international reach quickly and efficiently.
Highlighting Prateeksha Web Design’s Expertise
At Prateeksha Web Design, we understand the nuances of building i18n-ready websites. Our team ensures that your website isn’t just translated but thoughtfully localized to meet cultural and linguistic needs. From small businesses looking to expand globally to larger enterprises optimizing their online presence, we bring expertise and attention to detail to every project.
Why Choose Us?
- We integrate Next.js i18n features seamlessly into your website for robust multilingual support.
- We provide SEO-optimized localization, ensuring your website ranks well in regional markets.
- We offer customized solutions tailored to small business needs, making global expansion accessible and affordable.
How Next.js Supports i18n Natively
The Next.js framework has simplified the process of creating multilingual websites by introducing native support for internationalization (i18n). This means you no longer have to rely on heavy third-party libraries to handle multiple languages and regional configurations in your web applications. Next.js integrates this functionality seamlessly into its ecosystem, making it a go-to solution for developers who want to build multilingual websites with minimal hassle.
Let’s break down how Next.js handles i18n and why its native support is a game-changer.
Key Features of i18n in Next.js
1. Routing Based on Locale
One of the standout features of Next.js is its ability to manage routing automatically based on the user's preferred locale. It achieves this through:
- Accept-Language Headers: Next.js can detect the language preferences set in the user’s browser and automatically redirect them to the appropriate language version of the site.
- Locale Subpaths: URLs can include locale-specific subpaths, such as
/en
,/es
, or/fr
, making it clear to both users and search engines that your site supports multiple languages.
For example:
- A user visiting
example.com
with a browser language set to Spanish (es
) can be redirected toexample.com/es
. - Similarly, a French user (
fr
) would seeexample.com/fr
.
This automatic redirection enhances user experience (UX) and helps maintain SEO best practices by using clear URL structures.
2. Dynamic Localization
With dynamic localization, content adjusts in real-time based on the user’s language preference. Next.js provides tools to dynamically render components or pages in the appropriate language without requiring page reloads. This ensures:
- A smooth, interactive experience for users.
- Reduced complexity in managing multiple versions of the same page.
3. Fallback Support
Not all translations are created equal, especially when expanding to new markets. Sometimes, certain content might not be available in a specific language. Next.js i18n provides a fallback mechanism that displays content in the default language (e.g., English) when translations are missing. This prevents the user from encountering empty or broken sections on your website.
For instance:
- If a French user accesses a page where the French translation is incomplete, they will see the English version as a fallback.
4. Integration with External Translation Libraries
While Next.js offers basic i18n features, it seamlessly integrates with powerful external libraries like i18next, react-intl, or LinguiJS. These tools add advanced functionalities such as:
- Context-aware translations.
- Pluralization.
- Date, time, and currency formatting specific to a locale.
This hybrid approach—combining native support with external libraries—makes Next.js a flexible and scalable solution for multilingual websites.
Step-by-Step Guide to Implementing i18n in Next.js
Let’s dive into the practical steps to enable and configure i18n in your Next.js project.
1. Setting Up Your Next.js Project
Start by creating a new Next.js application:
npx create-next-app my-multilingual-site
cd my-multilingual-site
This command sets up a basic Next.js project structure.
Next, install libraries that will help manage translations (optional but recommended):
npm install next-i18next react-i18next
2. Configuring next.config.js
The next.config.js
file is the main configuration point for enabling i18n in your Next.js project. Here’s how you can set it up:
module.exports = {
i18n: {
locales: ['en', 'es', 'fr'], // Languages your app will support
defaultLocale: 'en', // Default fallback language
},
};
locales
: This array specifies all the languages your app will support. For example,'en'
for English,'es'
for Spanish, and'fr'
for French.defaultLocale
: The default language used when no locale is specified.
Next.js uses this configuration to automatically handle locale routing and other i18n-related tasks.
3. Creating Locale Files
Now, you need to create translation files for each supported language. Follow this structure:
/public
/locales
/en
common.json
/es
common.json
/fr
common.json
Each JSON file stores key-value pairs for your translations. For example, common.json
in English might look like this:
{
"welcomeMessage": "Welcome to our website!",
"aboutUs": "Learn more about us"
}
The corresponding Spanish file (es/common.json
) would look like this:
{
"welcomeMessage": "¡Bienvenido a nuestro sitio web!",
"aboutUs": "Aprenda más sobre nosotros"
}
By using this structure, you can centralize all your translations in one place.
4. Using the next-i18next
Library
While Next.js provides basic i18n support, the next-i18next
library simplifies managing translations, especially for large applications. To use it, follow these steps:
-
Create an
i18n.js
file:import NextI18Next from 'next-i18next'; const NextI18NextInstance = new NextI18Next({ defaultLanguage: 'en', otherLanguages: ['es', 'fr'], }); export default NextI18NextInstance;
-
Update your
_app.js
file to wrap the application with theappWithTranslation
provider:import App from 'next/app'; import { appWithTranslation } from '../i18n'; class MyApp extends App { render() { const { Component, pageProps } = this.props; return <Component {...pageProps} />; } } export default appWithTranslation(MyApp);
This configuration ensures that translations are available across all pages in your application.
Benefits of Native i18n Support in Next.js
- Simplicity: You don’t need extensive external libraries or custom logic for basic i18n features.
- Performance: Native integration ensures optimal performance, with minimal impact on page load times.
- Scalability: As your website grows, adding new languages or locales is straightforward.
Advanced Techniques for i18n in Next.js
Once you've implemented the basics of internationalization (i18n) in Next.js, you can take it a step further by incorporating advanced techniques. These approaches enhance the functionality, usability, and performance of your multilingual website, while also ensuring a seamless user experience and improved search engine rankings.
1. Dynamic Language Detection
Dynamic language detection allows your website to automatically adapt to a user’s preferred language based on their browser settings or geographical location. This technique ensures visitors see content in their preferred language without manually selecting it, providing a personalized and intuitive experience.
How It Works:
-
Browser Language Detection: Web browsers send an
Accept-Language
header with every request, which specifies the user’s language preferences. You can use this header to determine the most appropriate language for the user. -
IP-Based Geolocation: Services like MaxMind or GeoIP allow you to infer the user’s location and suggest the corresponding language. While this approach is more resource-intensive, it’s highly effective for regional language preferences.
Implementing Dynamic Language Detection with Middleware:
Next.js middleware is a powerful tool to implement dynamic language detection. Here’s an example:
import { NextResponse } from 'next/server';
export async function middleware(req) {
const { nextUrl } = req;
// Detect language from browser's Accept-Language header
const locale = req.headers.get('accept-language')?.split(',')[0] || 'en';
// Update the URL to include the detected locale
nextUrl.locale = locale;
return NextResponse.redirect(nextUrl);
}
Explanation:
req.headers.get('accept-language')
: Extracts the user’s language preference from the browser header.- Fallback Locale: If the header is unavailable, the code defaults to English (
'en'
). - Redirect: Users are automatically redirected to their preferred language version of the site.
Benefits:
- Improves user experience by reducing friction.
- Ensures users interact with your content in their native language.
Best Practice: Always allow users to manually switch languages, as automated detection might not always align with their preferences.
2. SEO for Multilingual Websites
Search engine optimization (SEO) is crucial for multilingual websites. Properly configured i18n ensures that search engines can index and rank your content accurately for different regions and languages.
Key SEO Practices:
a. Use hreflang Tags
The hreflang
attribute helps search engines identify language variations of your content. This prevents duplicate content issues and ensures that users are directed to the correct version of your website.
Here’s an example of how to implement hreflang
tags in a Next.js project:
<link rel="alternate" hreflang="en" href="https://example.com/en/" />
<link rel="alternate" hreflang="es" href="https://example.com/es/" />
<link rel="alternate" hreflang="fr" href="https://example.com/fr/" />
You can dynamically add these tags to your pages using the next/head
component in Next.js:
import Head from 'next/head';
export default function Page() {
return (
<Head>
<link rel="alternate" hreflang="en" href="https://example.com/en/" />
<link rel="alternate" hreflang="es" href="https://example.com/es/" />
<link rel="alternate" hreflang="fr" href="https://example.com/fr/" />
</Head>
);
}
b. Generate Separate Sitemaps
A multilingual website should have separate sitemaps for each language version to improve search engine crawling and indexing. You can use dynamic sitemap generation tools or write custom scripts.
For example, using next-sitemap
:
-
Install the package:
npm install next-sitemap
-
Configure
next-sitemap.config.js
:module.exports = { siteUrl: 'https://example.com', generateRobotsTxt: true, i18n: { locales: ['en', 'es', 'fr'], defaultLocale: 'en', }, };
-
Generate the sitemaps:
npm run next-sitemap
This creates a sitemap file for each locale, improving your site’s visibility in regional searches.
3. Integrating with External Translation Services
When managing large-scale translations, external services and tools can simplify the process by providing automation, consistency, and scalability.
Popular Services:
-
Google Translate API
- Automates translations using Google’s machine learning models.
- Ideal for generating quick, draft translations.
How to integrate:
- Install the Google Translate client library.
- Use the API to dynamically fetch translations for text strings.
Example:
const { Translate } = require('@google-cloud/translate').v2; const translate = new Translate(); const text = 'Hello, world!'; const targetLanguage = 'es'; async function translateText() { const [translation] = await translate.translate(text, targetLanguage); console.log(`Translation: ${translation}`); } translateText();
-
Crowdin
- A professional platform for managing translation workflows.
- Offers collaboration features for translators and project managers.
-
Transifex
- Focuses on localization for web and mobile apps.
- Provides APIs and tools for continuous integration with your project.
Advantages of Using External Services:
- Consistency: Centralized translation management ensures uniform terminology.
- Scalability: Adding new languages becomes a seamless process.
- Efficiency: Automating repetitive tasks reduces manual effort.
Advanced Techniques for i18n in Next.js
Once you’ve set up the basic i18n functionality in Next.js, you can go further by implementing advanced techniques to enhance user experience, optimize SEO, and simplify translation management. Here’s a detailed breakdown of these techniques.
1. Dynamic Language Detection
Dynamic language detection automatically identifies a user's preferred language and serves them content in that language. This feature creates a smoother experience for users by eliminating the need for manual language selection.
How Dynamic Language Detection Works:
- Browser Preferences: Browsers send an
Accept-Language
header with every HTTP request, listing the user's preferred languages in order of priority. For example,Accept-Language: en-US,en;q=0.9,es;q=0.8
indicates that the user prefers U.S. English first, followed by general English and then Spanish. - IP-Based Geolocation: Services like GeoIP can detect the user’s location based on their IP address and infer the appropriate language.
Implementing Dynamic Language Detection in Next.js
Next.js middleware allows you to intercept and modify requests before they reach your application. You can use this feature to detect the user's preferred language and redirect them to the appropriate localized version of your site.
Here’s how to implement it:
import { NextResponse } from 'next/server';
export async function middleware(req) {
const { nextUrl } = req;
// Detect language from browser's Accept-Language header
const locale = req.headers.get('accept-language')?.split(',')[0] || 'en';
// Redirect to the appropriate locale
const urlWithLocale = new URL(nextUrl);
urlWithLocale.pathname = `/${locale}${nextUrl.pathname}`;
return NextResponse.redirect(urlWithLocale);
}
- Detect Locale: Extract the preferred language from the
Accept-Language
header. - Set Fallback: Default to English (
'en'
) if no preference is detected. - Redirect: Adjust the URL to include the locale subpath (e.g.,
/en
,/es
).
Advantages:
- Provides a personalized user experience.
- Reduces friction for first-time visitors.
Best Practices:
- Always allow users to manually override the detected language.
- Use a lightweight fallback mechanism for performance optimization.
2. SEO for Multilingual Websites
Implementing effective SEO for multilingual websites ensures that your content ranks well in different regions and languages. Poorly configured multilingual sites can confuse search engines, leading to issues like duplicate content or incorrect indexing.
Key SEO Strategies:
a. Using hreflang Tags
The hreflang
attribute signals to search engines which version of your page to show to users based on their language and region. This helps prevent duplicate content penalties and ensures users land on the correct localized page.
Example of hreflang Tags:
<link rel="alternate" hreflang="en" href="https://example.com/en/" />
<link rel="alternate" hreflang="es" href="https://example.com/es/" />
<link rel="alternate" hreflang="fr" href="https://example.com/fr/" />
Implementation in Next.js:
You can dynamically generate these tags for each page using the next/head
component:
import Head from 'next/head';
export default function Page() {
return (
<Head>
<link rel="alternate" hreflang="en" href="https://example.com/en/" />
<link rel="alternate" hreflang="es" href="https://example.com/es/" />
<link rel="alternate" hreflang="fr" href="https://example.com/fr/" />
</Head>
);
}
b. Generating Separate Sitemaps
A multilingual website should generate separate sitemaps for each language version. These sitemaps help search engines efficiently crawl and index all localized pages.
Using next-sitemap
:
- Install the package:
npm install next-sitemap
- Configure it in
next-sitemap.config.js
:module.exports = { siteUrl: 'https://example.com', generateRobotsTxt: true, i18n: { locales: ['en', 'es', 'fr'], defaultLocale: 'en', }, };
- Generate the sitemaps:
npm run next-sitemap
Benefits:
- Improves search engine visibility for all language versions.
- Ensures proper indexing of regional content.
3. Integrating with External Translation Services
Managing translations manually can be tedious, especially for large-scale projects. Integrating external translation services simplifies the process, ensures consistency, and allows for automated workflows.
Popular Translation Services:
-
Google Translate API:
- Provides machine translations for over 100 languages.
- Suitable for quick and basic translations but may require manual refinement for accuracy.
Integration Example:
const { Translate } = require('@google-cloud/translate').v2; const translate = new Translate(); const text = 'Hello, world!'; const targetLanguage = 'es'; async function translateText() { const [translation] = await translate.translate(text, targetLanguage); console.log(`Translation: ${translation}`); } translateText();
-
Crowdin:
- A professional translation management platform for collaboration between translators and developers.
- Integrates with Git repositories for continuous localization workflows.
-
Transifex:
- Focused on streamlining localization for web and mobile applications.
- Provides APIs for seamless integration and automated updates.
Advantages of Using External Services:
- Scalability: Easily add or update translations as your website grows.
- Consistency: Centralized translation management ensures uniform terminology across pages.
- Efficiency: Automates repetitive tasks, saving time and reducing errors.
Best Practices:
- Use machine translations for initial drafts but have human translators refine the output for accuracy.
- Regularly update and review translations to reflect changes in content.
Testing and Debugging i18n in Next.js
Thorough testing is essential to ensure that your i18n setup works as intended across all supported languages and regions. Here’s how to approach it:
1. Jest for Unit Testing
Use Jest to test individual components and ensure that translations load correctly:
import { render } from '@testing-library/react';
import { useTranslation } from 'react-i18next';
test('renders translated text', () => {
const { t } = useTranslation();
const { getByText } = render(<div>{t('welcomeMessage')}</div>);
expect(getByText('Welcome to our website!')).toBeInTheDocument();
});
2. Cypress for End-to-End Testing
Cypress is great for simulating real-world user interactions and verifying that all language versions behave as expected:
describe('Language switching', () => {
it('should navigate to the Spanish version', () => {
cy.visit('/en');
cy.get('button[data-cy="switch-to-es"]').click();
cy.url().should('include', '/es');
});
});
3. Lighthouse for Performance and Accessibility
Run Lighthouse audits to ensure your multilingual pages are fast, accessible, and SEO-friendly. Focus on metrics like:
- Page load speed for different locales.
- Accessibility compliance for non-English languages.
Real-World Applications of i18n in Next.js
Case Study: Prateeksha Web Design’s Multilingual Projects
At Prateeksha Web Design, we’ve implemented i18n for several small businesses, helping them expand their reach. For example, we recently developed a multilingual website for a startup catering to both English and Spanish-speaking audiences. The result? A 30% increase in international traffic and a noticeable uptick in conversions.
Challenges and Best Practices
Common Challenges:
- Handling right-to-left (RTL) languages like Arabic.
- Keeping translations in sync with content updates.
- Managing fallback languages.
Best Practices:
- Use a centralized translation management tool.
- Optimize performance by lazy-loading locale files.
- Regularly test for accuracy and UX consistency.
Conclusion
Implementing i18n in Next.js can significantly enhance the reach and impact of your website, making it accessible to global audiences. By following this guide, you’ll create a scalable, user-friendly multilingual site optimized for both users and search engines.
At Prateeksha Web Design, we pride ourselves on our expertise in creating stunning, i18n-ready websites tailored to small businesses. Whether you’re building a simple multilingual blog or a complex e-commerce platform, our team is here to help.
About Prateeksha Web Design
Prateeksha Web Design offers expert services in implementing internationalization (i18n) in Next.js projects. Our team can help you set up multi-language support, localize content, and optimize user experiences for different regions. We ensure seamless integration of i18n features, including language detection, translation management, and dynamic content rendering. With our expertise, your Next.js website can cater to a global audience effectively. Contact us today to enhance your website's international reach.
Interested in learning more? Contact us today.
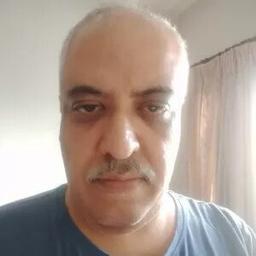