Exploring Middleware in Next.js: Enhancing Functionality and Performance Like a Pro
Stepping into the world of Next.js middleware feels like entering a secret layer of your app’s superpowers. It’s that magic middle ground where requests are intercepted, processed, and transformed before reaching their final destination. Whether you’re new to the game or sharpening your skills, understanding middleware in Next.js is your ticket to improving functionality and boosting performance in your applications.
At Prateeksha Web Design, we’ve harnessed middleware to craft solutions that aren’t just functional but downright smart. Let’s unpack the secrets of Next.js middleware and arm you with the know-how to elevate your projects.
What is Middleware? And Why Should You Care?
Let’s break this down step by step, starting with a simple analogy:
Imagine you own a coffee shop. Your customers walk in, place their orders, and expect the perfect cup of coffee. But here’s the twist: everyone has unique preferences. Some want extra foam, others want sugar-free, and a few adventurous souls ask for oat milk. Enter your barista—the middleman (or middlewoman!) who takes the order, processes the details, and customizes the drink before it reaches the customer. That’s essentially what middleware does in the world of Next.js.
Middleware is like that barista for your web application. It acts as a checkpoint, intercepting incoming requests and making adjustments before those requests reach your backend logic or rendering engine. This means you can validate, redirect, or even completely rewrite requests on the fly. Pretty cool, right?
What Middleware Does in Next.js
Middleware operates at the request-response cycle level, which is the fundamental communication process in web development. Every time someone interacts with your Next.js app—whether by clicking a link, submitting a form, or refreshing a page—a request is sent to your server, and your server responds with data.
Middleware slides into this process, sitting between the incoming request and the server's response. It’s a way to add custom logic without repeating yourself across multiple routes or components.
Key Takeaways: Why Middleware Matters
1. Middleware Runs on the Edge Runtime (Blazing Fast!)
Unlike traditional server-side logic, which executes on centralized servers, middleware in Next.js runs on the Edge Runtime. The Edge Runtime is a globally distributed environment designed for low-latency, high-performance operations. This setup ensures your middleware runs super fast, often closer to the user geographically, reducing delays.
Think of it as having a coffee shop branch in every neighborhood rather than a single shop in the city center. The closer the shop, the quicker the service!
2. Middleware Works at the Request-Response Cycle Level
Middleware operates at a core level of how the web works. It doesn’t depend on pages, components, or APIs—it works on the entire application level. Every request to your app passes through middleware, giving you a chance to inspect and modify it.
Example tasks:
- Checking user authentication (like asking, “Do you have a membership card?”).
- Redirecting users (like saying, “We’ve moved locations—head to our new store!”).
- Rewriting requests (like swapping out one ingredient for another based on preferences).
3. Perfect for Redirects, Authentication, and Logging
Middleware is a natural fit for tasks that need to happen across your app. Here’s how it shines:
- Redirects: Dynamically send users to the right place. For example, redirect
/old-blog
to/new-blog
without breaking links. - Authentication: Check if a user is logged in before granting access to certain pages, much like a bouncer checking IDs.
- Logging: Record information about every request, such as timestamps, user locations, or API usage, for debugging and analytics.
Middleware in Next.js: The Basics
Middleware in Next.js uses the middleware.ts
(or .js
) file at the root of your project. It’s as simple as this:
import { NextResponse } from 'next/server';
export function middleware(request) {
const url = request.nextUrl.clone();
if (url.pathname === '/old-route') {
url.pathname = '/new-route';
return NextResponse.redirect(url);
}
}
Here’s the rundown:
- Request: The incoming request object.
- NextResponse: A utility to respond, redirect, or rewrite requests.
- Edge Runtime: The environment where middleware operates, designed for low-latency execution.
Think of this as a bouncer at the club—checking IDs (authentication), rerouting guests (redirects), or granting VIP access (custom headers).
Where Middleware Shines: Key Use Cases
1. Authentication and Authorization
Your app’s security depends on verifying users, and middleware is your backstage hero. Instead of checking authentication in every API route, middleware can handle it globally.
export function middleware(request) {
const token = request.cookies.get('auth_token');
if (!token) {
return NextResponse.redirect('/login');
}
}
This ensures only logged-in users access protected routes, saving you the hassle of duplicating logic.
2. Redirects and Rewrites
Need to update URLs without breaking bookmarks or SEO? Middleware’s got your back.
Example: Redirecting users from /blog
to /articles
.
export function middleware(request) {
const url = request.nextUrl.clone();
if (url.pathname === '/blog') {
url.pathname = '/articles';
return NextResponse.redirect(url);
}
}
3. Localization
If your app caters to users across the globe, middleware can dynamically serve content in their preferred language.
export function middleware(request) {
const { pathname, searchParams } = request.nextUrl;
const locale = request.headers.get('accept-language')?.split(',')[0] || 'en';
request.nextUrl.pathname = `/${locale}${pathname}`;
return NextResponse.rewrite(request.nextUrl);
}
Voilà! You’ve turned your app into a polyglot without breaking a sweat.
4. Performance Monitoring
Middleware can log request details, helping you analyze performance and debug bottlenecks. Use a logging service like Datadog or Sentry to track metrics effortlessly.
export function middleware(request) {
console.log(`Request to ${request.nextUrl.pathname} at ${new Date().toISOString()}`);
return NextResponse.next();
}
Boosting Next.js Performance with Middleware
Middleware is not just a functional tool in your developer toolkit—it’s a performance optimizer that can turn your Next.js app into a lightning-fast powerhouse. Let’s explore how middleware can enhance your app’s performance in ways that make both users and developers happy.
1. Reduce Latency
One of middleware’s greatest strengths lies in its ability to execute on the Edge Runtime. Unlike traditional server-side logic that runs on a central server (which might be thousands of miles away from the user), middleware runs closer to the user geographically.
This proximity reduces the round-trip time (RTT)—the time it takes for a request to travel to the server and back. When requests are intercepted and processed on the edge, you eliminate unnecessary delays.
Example Use Case: If you have users across the globe, your middleware ensures their requests are processed at the nearest data center, making the app feel snappier regardless of their location.
2. Offload Server Logic
By handling tasks like validation, authentication, and routing in middleware, you offload work from your main server. This reduces the strain on your backend, leaving it free to focus on rendering pages or fetching data.
Here’s why this matters:
- Middleware can validate requests (e.g., checking headers or cookies) before they even hit your API routes.
- It can rewrite or redirect requests without involving your backend logic, saving precious computing cycles.
Example Use Case: For an e-commerce app, middleware can check if a user’s session is valid or if they have the required permissions before proceeding to the main server. This reduces the load on your backend, especially during high-traffic periods like Black Friday sales.
3. Streamline Caching
Efficient caching is one of the most effective ways to boost performance, and middleware can play a key role in implementing dynamic caching strategies. By adding or modifying caching headers, you can control how long certain responses are stored, reducing the need to regenerate them repeatedly.
Example: Here’s how you can use middleware to set caching headers:
export function middleware(request) {
const response = NextResponse.next();
response.headers.set('Cache-Control', 's-maxage=60, stale-while-revalidate=30');
return response;
}
What does this do?
- s-maxage=60: Cache the response on the server for 60 seconds.
- stale-while-revalidate=30: Serve stale responses for up to 30 seconds while a fresh version is revalidated in the background.
This approach ensures users always get fast responses, even during cache refreshes.
Practical Tips for Middleware Mastery
Middleware is a game-changer, but like any tool, its effectiveness depends on how you use it. Mastering middleware requires a thoughtful approach to design, testing, and integration. Here are some practical tips to ensure you get the most out of it:
1. Keep It Lightweight
Middleware runs on every request that comes to your Next.js app, so it’s critical to keep it efficient. Avoid heavy computations or complex operations that could slow down your application.
- Good Practice: Use middleware for tasks like redirects, authentication, or basic validation—things that are quick and essential.
- Bad Practice: Running database queries or performing CPU-intensive operations directly in middleware.
Think of middleware like a toll booth on a highway: it should check and let cars pass quickly, not perform an emissions test on each vehicle.
2. Test Extensively
Middleware is global in scope—it affects every incoming request. Bugs in middleware can cause cascading issues across your app, so rigorous testing is non-negotiable.
- Simulate Scenarios: Test middleware against edge cases, such as invalid URLs, missing cookies, or large payloads.
- Use Mock Requests: Create test cases that mimic real-world scenarios, including different user roles, locations, and device types.
Remember, a small oversight in middleware could mean your users are stuck at the login page or being misdirected to a 404 error.
3. Leverage Edge Functions
Middleware becomes even more powerful when paired with Vercel’s Edge Functions. These functions allow you to run serverless logic at the edge, enabling advanced customizations without sacrificing speed.
- Use Edge Functions for real-time data processing, such as fetching dynamic user data.
- Combine middleware with Edge Functions to handle region-specific content, such as displaying prices in local currencies.
Example: Middleware can rewrite the URL to an Edge Function that processes a user’s profile based on their location.
Common Pitfalls to Avoid
Middleware is a double-edged sword—it’s incredibly useful, but misusing it can cause more harm than good. Let’s look at some common mistakes and how to avoid them:
1. Overusing Middleware
Middleware is not a catch-all solution. While it’s tempting to throw everything into it, not every task belongs there. Middleware is best suited for lightweight, app-wide tasks, not for every piece of business logic.
Example of Overuse:
export function middleware(request) {
const user = fetchUserFromDatabase(request.cookies.get('id')); // Don't do this!
if (user.isAdmin) {
return NextResponse.next();
}
}
Why It’s Bad: Fetching data from a database in middleware adds unnecessary latency and should instead happen in API routes or server-side rendering logic.
2. Ignoring Edge Runtime Limits
The Edge Runtime has specific limitations. Since middleware runs at the edge, you can’t use Node.js APIs like fs
(file system) or path
. Ignoring these constraints can result in runtime errors.
Avoid These Mistakes:
- Using file system APIs to read files.
- Relying on server-specific configurations.
Instead, stick to the tools and libraries explicitly supported in the Edge Runtime.
3. Poor Debugging
Debugging middleware can be tricky because it operates on the request-response cycle. Over-reliance on console.log
is a common mistake, especially since it can clutter logs and make troubleshooting harder.
Better Debugging Practices:
- Use debugging libraries like Sentry or LogRocket to track errors and performance.
- Test middleware locally using mock requests to avoid deploying buggy code.
Pro Tip: Add meaningful error messages in your middleware to pinpoint issues faster when they occur.
Real-World Example: How Prateeksha Web Design Uses Middleware
At Prateeksha Web Design, we’ve implemented middleware to create seamless user experiences for e-commerce clients. Here’s a favorite use case:
Dynamic Pricing Middleware: For a Shopify-like app, middleware calculates discounts based on user location and membership tier. A request from Germany? Show prices in Euros, with a membership discount baked in.
export function middleware(request) {
const userTier = request.cookies.get('membership_tier');
const location = request.geo?.country || 'US';
let discount = userTier === 'gold' ? 0.2 : 0.1;
const response = NextResponse.next();
response.headers.set('X-Discount', discount);
response.headers.set('X-Currency', location === 'DE' ? 'EUR' : 'USD');
return response;
}
Ready to Level Up? Let’s Build Together!
Middleware is a game-changer in Next.js, giving you the power to improve functionality, streamline workflows, and boost performance. Whether you’re crafting a dynamic app, enhancing SEO, or ensuring airtight security, middleware is your trusty sidekick.
At Prateeksha Web Design, we specialize in bringing these technical marvels to life. Whether you’re just exploring or ready to dive in, we’re here to help you craft Next.js applications that not only work but shine.
Your Turn: Experiment and Share!
Here’s your call to action: experiment with middleware in your Next.js projects. Start small—try adding redirects or monitoring logs. Once you’ve got the hang of it, push the boundaries with dynamic rewrites or real-time personalization.
Have questions? Let us know! We’re always excited to hear how developers are using middleware to innovate.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services in exploring middleware within Next.js to elevate web application functionality and performance. Their expertise includes implementing custom middleware solutions that streamline data handling, enhance routing processes, and optimize resource loading. By leveraging Next.js's capabilities, they ensure faster page transitions and improved user experiences. Additionally, they focus on integrating third-party APIs seamlessly while maintaining robust security measures. Trust Prateeksha Web Design to unlock the full potential of your Next.js applications through innovative middleware strategies.
Interested in learning more? Contact us today.
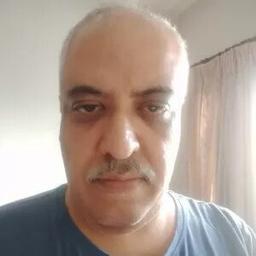