How Program Geeks Implement Internationalization (i18n) in Next.js Apps
So, you’ve decided to embark on the thrilling journey of Next.js i18n—and trust us, it’s like discovering a treasure chest overflowing with endless possibilities! Whether you’re an aspiring web developer or a seasoned tech aficionado, enabling multilingual capabilities in your application can transform your site into a global phenomenon, much like a pizza at a kid's birthday party—who doesn’t want that extra topping of accessibility? 🍕
In this blog post, we're going to thoroughly explore how you can implement internationalization (i18n) within your Next.js applications, expanding your audience reach and enhancing user experience. We’ll keep things informative yet playful, mixing in some technical goodness as we dive deep into the vast ocean of Next.js internationalization. Get ready to make your web applications as renowned as the latest pop sensation!
The Basics: Understanding Internationalization (i18n)
Internationalization (often abbreviated to i18n for a dash of flair) is the design process that allows your application to support various languages and regional peculiarities. Think of it as preparing your website for a grand world tour! When executed effectively, it allows you to create multilingual websites that resonate with diverse audiences.
Consider your website as an exquisite dish that everyone loves; however, only a handful can understand the recipe (content). With Next.js localization, you’ll make that recipe available in every conceivable language. Excited to learn more? Let’s roll up our sleeves and get cooking!
Why Choose Next.js for Internationalization?
You might wonder, "Why is Next.js the ideal choice for i18n?” Great question! Here’s why:
-
Server-Side Rendering (SSR): Next.js utilizes server-side rendering to enhance SEO significantly. Users from different parts of the globe can discover your site with ease!
-
Dynamic Routing: Next.js empowers seamless delivery of localized content based on users' preferences or geographic locations, making navigation effortless.
-
Comprehensive Documentation: A developer’s journey is best paved with great documentation, and Next.js offers plenty of resources to guide you through the intricacies of i18n.
-
Performance Optimized: This framework is engineered for speed and efficiency right from the start. After all, who doesn’t appreciate a fast-loading website?
Are you ready to take the plunge into the realm of Next.js i18n? Let’s outline the steps you need to implement internationalization in your applications!
Key Takeaways to Get Started with Next.js i18n
1. Setting Up Next.js for Multilingual Websites
Before you start whipping up multilingual content, you’ll need to install Next.js, if you haven’t done so already. Here’s how to spin up a new Next.js application:
npx create-next-app@latest your-project-name
Now that you’re in, let’s configure the Next.js localization feature. Open your next.config.js
file and add the i18n settings:
module.exports = {
i18n: {
locales: ['en-US', 'es', 'fr', 'de'],
defaultLocale: 'en-US',
},
};
This configuration informs Next.js about the languages your application will support, akin to setting up directional signposts on a road trip that guide travelers (users) to various linguistic destinations!
2. Managing Translations
Next.js does not come pre-packaged with a translation library, so you’ll need to select one that fits your needs! Some popular choices include:
- i18next: A powerful internationalization framework that provides extensive features for all your i18n needs.
- react-i18next: A seamless binding for React that leverages i18next's capabilities.
Suppose you choose to go with react-i18next. The first step is to install the library:
npm install react-i18next i18next
Next, you'll want to establish a clear structure for managing translations. Here’s how to set it up:
-
Create a folder for your translations: Inside your project directory, create a folder called
locales
. -
Organize subfolders for each language: Within the
locales
folder, create subfolders for every language you wish to support:locales/en/translation.json
locales/es/translation.json
locales/fr/translation.json
locales/de/translation.json
-
Add translations: Populate each JSON file with key-value pairs representing text in your application:
- Example for
locales/en/translation.json
:
{ "welcome": "Welcome to our website!", "description": "This is an internationalized application using Next.js." }
- Example for
locales/es/translation.json
:
{ "welcome": "¡Bienvenido a nuestro sitio web!", "description": "Esta es una aplicación internacionalizada usando Next.js." }
- Example for
3. Initialize i18next
Now that your translation files are set up, you need to initialize i18next in your application. Create a new file named i18n.js
in your root directory:
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
// Importing language files
import enTranslation from './locales/en/translation.json';
import esTranslation from './locales/es/translation.json';
import frTranslation from './locales/fr/translation.json';
import deTranslation from './locales/de/translation.json';
// Initialize i18next
i18n
.use(initReactI18next)
.init({
resources: {
en: { translation: enTranslation },
es: { translation: esTranslation },
fr: { translation: frTranslation },
de: { translation: deTranslation },
},
lng: 'en', // Default language
fallbackLng: 'en',
interpolation: {
escapeValue: false, // React already does escaping
},
});
export default i18n;
4. Integrate i18next with your Next.js app
With i18next initialized, you can now incorporate it into your Next.js application. Import the i18n.js
file in your _app.js
:
import '../styles/globals.css';
import '../i18n'; // Importing i18next
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default MyApp;
5. Use Translation in Your Components
Now you can start utilizing translations within your components. Here’s a quick example of using the useTranslation
hook:
import React from 'react';
import { useTranslation } from 'react-i18next';
const Home = () => {
const { t } = useTranslation(); // The t function will be used for translations
return (
<div>
<h1>{t('welcome')}</h1>
<p>{t('description')}</p>
</div>
);
};
export default Home;
Conclusion
Congratulations! You’ve now laid the foundation for internationalizing your Next.js application. By following these steps, you'll be well on your way to crafting an inclusive and user-friendly online presence that caters to audiences from all corners of the globe.
As you continue to refine your skills, remember that internationalization is not just about translation; it's about providing a personalized experience for all users, regardless of their language or background. So, roll up your sleeves, dive into the world of multilingual web development, and start building that incredible Next.js app that everyone will want to visit! 🌍✨
Certainly! Let's expand and refine your content on implementing internationalization (i18n) in a web application, focusing specifically on the structure of translation files, the setup of the translation provider, and utilizing translations in components.
Internationalization Setup
To effectively manage multiple languages in your web application, you can structure your translation files in a dedicated locales
directory. Here’s a hierarchical representation of how to organize your translations:
Directory Structure
/locales
/en
common.json
/es
common.json
In the above structure, the locales
folder contains subdirectories for each language you want to support. Each language directory holds a common.json
file which stores the key-value pairs for the translations.
Example of Translation Files
English (en/common.json)
{
"welcome": "Welcome to our website!",
"description": "We're glad to have you here."
}
Spanish (es/common.json)
{
"welcome": "¡Bienvenido a nuestro sitio web!",
"description": "Estamos contentos de tenerte aquí."
}
These JSON files contain key-value pairs where the keys represent the text identifiers, and the values are the corresponding translations in each language. This separation of language files allows for easy expansion and management of translations as your application grows and you add more languages.
Implementation Steps
Now that we have the translation files set up, let’s implement the translations in your application.
1. Create a Translation Provider
To manage the translation context, you need to create a provider. This can be done in your main application file, typically _app.js
if you're using Next.js. The provider will allow your entire application to have access to the translation functionalities.
Example Implementation in _app.js
import { appWithTranslation } from 'next-i18next';
// Main application component
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
// Exporting the application wrapped with the translation provider
export default appWithTranslation(MyApp);
By wrapping your application with appWithTranslation
, you enable the translation capabilities throughout all components, ensuring that you can easily access translations based on the user's selected language.
2. Using Translations in Your Components
Integrating translations into your React components is straightforward. For instance, let’s assume you want to display a welcome message in your home component. You can achieve this by utilizing the useTranslation
hook provided by the i18n library.
Example Home Component
import { useTranslation } from 'react-i18next';
const Home = () => {
// Accessing the translation function
const { t } = useTranslation('common');
return (
<div>
<h1>{t('welcome')}</h1>
<p>{t('description')}</p>
</div>
);
};
export default Home;
In the code above, the useTranslation
hook is used to access the translation function t
. This function takes a translation key (like 'welcome'
or 'description'
) and returns the appropriate translated text depending on the user's current language.
Summary
Congratulations! You’ve set up the foundational structure for supporting multiple languages in your web application. You have created a nested folder structure for your translations, implemented a translation provider, and successfully utilized translations in your components.
Next Steps
- Expand Translations: Add additional languages and more keys to your translation files.
- Language Switcher: Implement a language switcher in your app to allow users to choose their preferred language dynamically.
- Testing: Test your translations in different languages to ensure they render correctly across all components.
- Optimization: Consider optimizing loading performance for larger translation files depending on your app's requirements.
With this setup, your app is well on its way to reaching a broader audience by catering to diverse linguistic preferences! 🎉
Certainly! Here’s a refined and expanded version of your original content, focusing on clarity, detailed explanation, and engagement:
export default Home;
5. Dynamic Routing and Language Detection
Building a multilingual website has never been easier, especially with the powerful routing features of Next.js. To enhance user experience, it's crucial to direct users to content that matches their language preferences based on their browser settings. This is where dynamic routing and language detection come into play.
To implement this feature, modify the getServerSideProps()
function to analyze the HTTP request headers. Specifically, you do this by capturing the accept-language
parameter, which allows you to infer the user's preferred language. Here’s a practical example:
export async function getServerSideProps(context) {
const { req, res } = context;
const acceptLanguage = req.headers['accept-language'];
// Basic language detection logic
const userLocale = acceptLanguage?.split(',')[0] || 'en-US';
res.setHeader('Location', `/${userLocale}`);
res.statusCode = 302;
res.end();
return { props: {} };
}
In this snippet, we check the accept-language
header, extract the user's first language preference, and set a redirection to that language's route (e.g., /en
, /fr
, /es
). The result? When users access your site, they will automatically be greeted with content that's tailored to their language—making them feel right at home!
6. Content Localization
Beyond just translations, content localization considers the cultural nuances of your audience. This means adapting not only the language but also the context to resonate more deeply with local users. For example, if your target audience has a profound appreciation for coffee culture, it would be ideal to incorporate that into your localized content.
Consider this localized content structure:
{
"paragraph": "Our coffee culture is not just about the brews; it's about the connections we foster over a steaming cup!"
}
This personalized approach enhances relatability and can significantly improve user engagement and satisfaction.
7. Testing and Improving User Experience
Thorough testing is vital once you implement your Next.js translation features. Utilize tools like Lighthouse to assess your website's performance across different localized versions. Pay close attention to metrics such as load time, accessibility, and user interactions to ensure all visitors have a seamless experience.
Feedback is another critical component—gather insights from users who speak various languages to uncover areas for improvement. Language evolves, and fresh, user-driven content adjustments can maintain relevance and foster a more inclusive environment on your site.
8. Working with a Web Design Company
If implementing these features seems daunting, seeking assistance from a seasoned web design company could be a worthwhile investment. For instance, Prateeksha Web Design, located in Mumbai, excels in crafting stunning eCommerce website designs that seamlessly incorporate Next.js localization. Their expert team can help you navigate complexities, ensuring your global web applications resonate with diverse users worldwide.
9. Keep It Simple, Keep It Fun
Throughout your Next.js internationalization journey, prioritize user enjoyment. Aim to create a welcoming atmosphere for all users, regardless of their backgrounds.
Think of it like planning a birthday party: offering a variety of cakes (or languages) will create a more enjoyable experience. Catering to different tastes and respecting dietary preferences (local customs and norms) ensure everyone leaves the party happy—and the same should apply to your web applications.
Conclusion: Let the World See Your Work!
Congratulations, superstar 👏! You now possess the knowledge to implement Next.js i18n and prepare your application for a global audience. From dynamic routing and intuitive translation management to enhancing user experience, you are equipped with the strategies to develop multilingual websites that captivate users around the globe.
Keep in mind that internationalization is not a one-time endeavor—it’s an ongoing journey. As you create engaging content that speaks to your diverse audience, embrace the ever-evolving nature of language and localization. So go ahead, launch that project, and showcase your incredible capabilities to the world!
This version offers more detail, better structure, and encourages the reader's engagement. It also highlights the importance of testing and continuous improvement in the context of web localization.
Tip
Utilize Next.js Internationalized Routing: Leverage Next.js's built-in support for internationalized routing. By defining locales in your next.config.js
, you can easily manage multiple languages and facilitate SEO through localized routes. This allows users to access content in their preferred language seamlessly.
Fact
Support for Dynamic Content: Next.js provides a dynamic rendering feature that works well with internationalization. When fetching static or dynamic data, you can implement i18n by preparing translations for different locales, ensuring that content is automatically served based on the user's selected language or region settings.
Warning
Be Cautious with Date and Number Formatting: Different locales have variations in how dates, times, and numbers are formatted. Failing to implement locale-aware formatting can lead to confusion for users. Always utilize libraries like Intl
or external packages like date-fns
and react-intl
to ensure that your application displays these elements correctly according to the user’s locale.
About Prateeksha Web Design
Prateeksha Web Design specializes in integrating internationalization (i18n) for Next.js applications, ensuring seamless language support and localization. Their services include implementing locale detection, managing translation files, and optimizing routing for multiple languages. With a focus on user experience, they help developers create apps that cater to diverse audiences globally. They also offer ongoing support for updates and enhancements to i18n features. Prateeksha’s expertise ensures your Next.js app reaches a wider audience effectively.
Interested in learning more? Contact us today.
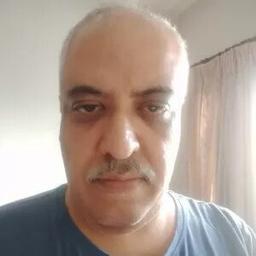