If you’ve dipped your toes into the programming world, chances are you’ve heard the terms Ruby and Ruby on Rails floating around. While these two are closely related, they are not the same thing, and understanding their key differences can open up new possibilities for your web development journey. Whether you’re just starting out or looking to build your next project, this blog will help you get a clear understanding of Ruby vs Ruby on Rails.
Let’s dive in and break it all down in a casual, engaging, and practical way.
What Is Ruby?
Ruby is a dynamic, high-level, and interpreted programming language designed with a single goal: to make programming enjoyable and efficient for developers. Yukihiro “Matz” Matsumoto, Ruby’s creator, introduced it in the mid-1990s, blending the best features of existing languages like Perl, Python, and Smalltalk.
The result? A programming language that balances simplicity with power, emphasizing human readability and productivity. Ruby empowers developers to focus on solving problems instead of wrestling with the complexity of code. It’s often compared to art—allowing programmers to craft elegant solutions effortlessly.
Breaking Down Ruby's Key Characteristics
1. Dynamic and Interpreted Language
Ruby is dynamic, meaning you don’t need to specify variable types during declaration—Ruby handles that for you at runtime. It’s also interpreted, which means code runs directly without requiring compilation into machine code. This makes development faster and debugging more accessible.
Example:
name = "Ruby"
puts "Hello, #{name}!"
The above code outputs Hello, Ruby!
without you needing to define name
as a string explicitly.
2. Object-Oriented to the Core
In Ruby, everything is an object. Whether you’re working with numbers, strings, arrays, or custom-defined types, they all inherit from Ruby’s base object class.
Example:
5.times { puts "Ruby is fun!" }
Here, the number 5
is treated as an object, and the method .times
is called on it to execute the block of code.
This object-oriented foundation makes Ruby exceptionally flexible. It allows you to:
- Extend classes on the fly.
- Override existing methods.
- Define custom behaviors for nearly anything.
3. Human-Friendly Syntax
Ruby’s syntax is one of its most celebrated features. It’s designed to be readable and intuitive, often resembling plain English. This lowers the learning curve for beginners while maintaining the depth needed for advanced programmers.
Example:
if age > 18
puts "You are eligible to vote."
else
puts "You are too young to vote."
end
This code is easy to read and understand, even if you’re new to programming.
4. Rich Ecosystem of Libraries (Gems)
Ruby comes with a treasure trove of libraries, commonly referred to as gems. These are pre-written code packages that you can integrate into your projects to save time and effort. Gems cover a wide range of functionalities, from web scraping to data analysis.
Example: Popular Gems
- Devise: Handles user authentication.
- Puma: High-performance server for web applications.
- Nokogiri: Parses and manipulates HTML and XML.
- Rails: The most famous gem, which powers the Ruby on Rails framework.
Installing and using gems is simple with Ruby’s package manager, Bundler.
Example:
gem install nokogiri
Once installed, you can include and use the gem in your Ruby project.
5. Versatility
Ruby isn’t confined to just web development. While Rails is its most popular use case, Ruby’s versatility allows it to shine in several domains:
- Scripting and Automation: Ruby scripts can automate repetitive tasks, from server maintenance to data processing.
- Game Development: Frameworks like Gosu enable Ruby developers to create 2D games.
- Data Analysis: Gems like
narray
andruby-daru
make data manipulation straightforward. - Command-Line Tools: Ruby is often used to create custom CLI tools for developers.
Why Ruby Stands Out
- Developer-Focused Design: Ruby prioritizes the needs of developers, aiming to reduce frustration and enhance creativity.
- Flexible and Open: You can modify nearly every part of Ruby to fit your needs.
- Community-Driven Growth: Ruby boasts a vibrant community that continuously contributes gems, tutorials, and tools to its ecosystem.
Example Use Case: Automating File Renaming
Let’s say you want to rename a batch of files. Here’s how Ruby makes it simple:
Dir.glob("*.txt").each_with_index do |file, index|
File.rename(file, "file_#{index + 1}.txt")
end
This script renames all .txt
files in a folder to file_1.txt
, file_2.txt
, and so on. A task that might take hours manually is completed in seconds.
Ruby in the Real World
Ruby has been adopted by companies and projects that value creativity and rapid development. Here are a few notable examples:
- Shopify: E-commerce platforms powered by Ruby and Rails.
- GitHub: The go-to repository hosting service.
- Basecamp: The project management tool that inspired the creation of Rails.
By choosing Ruby, you’re joining a community of developers dedicated to innovation and problem-solving.
Ruby vs Ruby on Rails: Breaking It Down
While Ruby and Ruby on Rails are closely linked, they serve different purposes. Let’s break down the key differences:
Aspect | Ruby | Ruby on Rails |
---|---|---|
Type | Programming Language | Web Application Framework |
Purpose | General-purpose programming | Focused on web development |
Usage | Can be used for scripting, automation, and more | Primarily used for building web apps |
Syntax | Elegant and expressive | Built on Ruby's syntax |
Learning Curve | Beginner-friendly | Slightly steeper, requires knowledge of Ruby first |
How Ruby and Rails Work Together
Ruby and Rails share a symbiotic relationship that makes web development faster, more efficient, and enjoyable. To fully appreciate how they complement each other, let’s break it down.
Ruby: The Foundation
Ruby is the programming language that powers Ruby on Rails. It provides the syntax, structure, and functionality that Rails uses to deliver its powerful features. Ruby’s elegant and dynamic nature is why Rails can offer so much flexibility and developer-friendly functionality.
Here’s how Ruby serves as the foundation:
-
Core Language: Rails applications are written entirely in Ruby. All the logic, classes, and methods you write in Rails are just Ruby code, executed using Ruby’s interpreter.
-
Customization: Ruby’s flexibility allows developers to extend or override methods easily, giving Rails its highly customizable nature.
-
DRY and Clean Code: Ruby’s principles, such as Don’t Repeat Yourself (DRY) and clean syntax, align perfectly with Rails’ design philosophy, ensuring minimal repetition and maximum efficiency.
Rails: The Framework
Rails is a web application framework built on Ruby. It adds layers of abstraction, predefined conventions, and tools that simplify the process of building complex web applications. While Ruby provides the raw material, Rails shapes it into a cohesive structure for web development.
Here’s how Rails enhances Ruby:
-
Pre-Built Components: Rails offers tools for routing, database interaction, form handling, and more, which are built using Ruby. This saves developers from reinventing the wheel.
-
Convention over Configuration: Rails minimizes the need for boilerplate code by following conventions. For example:
- Folder structures (e.g.,
models
,views
,controllers
) are predefined. - Naming conventions (e.g., a
Product
class automatically maps to aproducts
database table).
- Folder structures (e.g.,
-
Rapid Development Tools:
- Scaffolding: Auto-generates code for CRUD (Create, Read, Update, Delete) functionality.
- Active Record: Rails’ ORM (Object-Relational Mapping) system allows seamless database interactions.
How They Complement Each Other
Think of Ruby and Rails like a writer and a typewriter. Ruby provides the language and creative flexibility, while Rails supplies the tools to organize and produce the final product efficiently.
Example: Creating a Blog Post
Let’s say you’re building a blog post feature. Here’s how Ruby and Rails work together:
-
Model: Ruby handles the structure and logic. For example, the
Post
model defines attributes (title, content) and methods for business logic.class Post < ApplicationRecord validates :title, presence: true validates :content, length: { minimum: 10 } end
-
Controller: Rails defines how to handle user requests for posts (e.g., displaying, creating, or deleting them).
class PostsController < ApplicationController def create @post = Post.new(post_params) if @post.save redirect_to @post else render 'new' end end end
-
View: Ruby is used to write embedded Ruby templates (
.erb
) for dynamic HTML rendering.<h1><%= @post.title %></h1> <p><%= @post.content %></p>
This seamless combination of Ruby’s language capabilities and Rails’ framework conventions makes development faster, simpler, and more enjoyable.
Why Choose Ruby and Rails?
Ruby and Rails together form a dynamic duo for developers and businesses. Here are compelling reasons to choose this combination for your next project:
1. Developer Happiness
Ruby and Rails were designed with developers in mind, offering a smooth and enjoyable coding experience.
- Readable Code: Ruby’s syntax is clean and easy to understand.
- Effortless Debugging: Rails’ descriptive error messages make debugging a breeze.
- Tooling: Rails’ built-in generators and debugging tools minimize hassle, letting developers focus on creativity.
2. Rapid Prototyping
For startups or businesses in their early stages, speed is often critical. Ruby and Rails allow for the rapid development of MVPs (Minimum Viable Products).
- Scaffolding: Rails generates working code for basic CRUD operations in minutes.
- Convention over Configuration: Developers can skip tedious configurations and dive straight into building features.
- Ready-to-Use Gems: Gems like Devise (for authentication) and Active Admin (for admin panels) further speed up development.
Example: Companies like Shopify and Airbnb used Rails to build their early-stage products quickly.
3. Strong Community Support
Both Ruby and Rails boast thriving communities. This means you’ll never feel stuck, as there are endless tutorials, documentation, forums, and gems at your disposal.
- Ruby Gems: Over 150,000 gems are available, covering almost every imaginable functionality.
- Conferences: Events like RailsConf and RubyKaigi bring developers together to share knowledge and best practices.
4. Scalability
There’s a misconception that Ruby and Rails can’t scale, but this isn’t true. With proper optimization and tools, they can handle massive traffic loads.
- Concurrency: Tools like Puma and Unicorn ensure multiple requests are handled efficiently.
- Background Jobs: Sidekiq manages background tasks, improving performance for resource-intensive processes.
- Caching: Fragment and action caching in Rails boost page load times.
Example: GitHub and Shopify use Rails to manage millions of users and transactions daily.
Key Applications of Ruby and Rails
Ruby and Rails shine in several domains, thanks to their versatility, speed, and developer-friendly nature. Let’s explore their most prominent applications in detail.
1. E-Commerce
E-commerce platforms thrive on flexibility, scalability, and the ability to deliver personalized experiences to users—all of which Ruby and Rails excel at. Rails provides a robust framework for building dynamic online stores, while Ruby’s clean syntax simplifies customization and feature development.
How Ruby on Rails Powers E-Commerce:
- Rapid Development: Rails enables quick implementation of features like product catalogs, shopping carts, and payment gateways.
- Customizable Frontends: Ruby's flexibility ensures that the frontend experience can be tailored to match the brand’s identity.
- Scalability: Tools like Sidekiq for background processing and caching mechanisms like Redis ensure that stores can handle high traffic.
Example: Shopify
Shopify, one of the world’s leading e-commerce platforms, is built on Rails. It empowers businesses of all sizes to create and manage online stores efficiently, offering features like:
- Inventory management
- Payment processing
- Marketing tools
- Scalable hosting
With Ruby and Rails, Shopify supports millions of businesses globally, proving the framework’s capability in the e-commerce sector.
2. Social Media
Social media platforms require robust frameworks that support fast prototyping and the ability to handle millions of interactions in real-time. Ruby on Rails delivers on both counts, making it a popular choice for building early-stage social networks.
Why Rails Suits Social Media Development:
- Real-Time Features: Rails supports features like live notifications and chat, enhancing user engagement.
- Quick Iteration: The framework’s convention-over-configuration philosophy enables developers to iterate and release updates rapidly.
- Rich Community and Gems: Pre-built gems like Devise (authentication) and ActiveCable (real-time features) simplify development.
Example: Twitter (Early Days)
Twitter originally used Ruby on Rails, leveraging its rapid development capabilities to bring the platform to life. While Twitter eventually moved to other technologies to handle its massive scale, Rails was instrumental in its early success.
3. SaaS (Software as a Service)
Ruby and Rails are particularly popular among SaaS startups due to their ability to streamline the development of multi-tenant applications, subscription models, and APIs.
Key Advantages for SaaS Development:
- Built-in Tools for APIs: Rails includes native support for building RESTful APIs, a crucial requirement for SaaS products.
- Scalability and Maintainability: Modular architecture and support for background jobs allow SaaS applications to scale efficiently.
- Focus on User Experience: Ruby’s clean code and Rails’ rapid development tools enable teams to focus on enhancing user experience.
Examples:
- Basecamp: A project management tool developed by the creators of Rails, demonstrating the framework’s potential in creating collaborative SaaS solutions.
- GitHub: A code hosting platform built on Rails, serving millions of developers with features like repositories, pull requests, and issue tracking.
How Prateeksha Web Design Leverages Ruby and Rails
At Prateeksha Web Design, we harness the full potential of Ruby and Rails to craft high-quality web applications tailored to our clients' needs. Whether it’s an e-commerce store, a SaaS application, or a social media platform, our team ensures your vision becomes a reality.
Why Work with Us?
1. Custom Solutions
We don’t believe in one-size-fits-all. Every project we undertake is uniquely designed to meet the client’s goals, leveraging Rails’ flexibility to build features that matter most.
2. SEO Optimization
Our expertise in SEO ensures that every Rails-based web application we create is primed to rank well in search engines, helping businesses attract more traffic.
3. Mobile-Friendly Designs
In today’s mobile-first world, we ensure that your web app is responsive, delivering a seamless experience across devices.
4. Ongoing Support
We provide long-term support to keep your application updated, secure, and performing at its best.
Learning Ruby and Rails: A Beginner’s Guide
If you’re inspired by the capabilities of Ruby and Rails, learning them can open doors to exciting opportunities in web development. Here’s a step-by-step guide to get started:
Step 1: Learn Ruby
Ruby is the foundation of Rails, so start by mastering its basics. Platforms like Codecademy, RubyMonk, and The Odin Project offer beginner-friendly courses.
What to Focus On:
- Data types, variables, and operators
- Object-oriented programming concepts (classes, objects, inheritance)
- Control structures (loops, conditionals)
- File handling and scripting
Example: A Simple Ruby Script
puts "Enter your name:"
name = gets.chomp
puts "Hello, #{name}! Welcome to Ruby!"
Step 2: Explore Rails
Once you’re comfortable with Ruby, move on to Rails. The framework’s official guides and Michael Hartl’s Ruby on Rails Tutorial are excellent resources.
What to Focus On:
- Understanding the MVC (Model-View-Controller) architecture
- Working with routes, controllers, and views
- Using Active Record for database interactions
- Implementing RESTful APIs
Example: Generating a Rails App
rails new my_app
cd my_app
rails generate scaffold Post title:string content:text
rails db:migrate
rails server
This simple example sets up a blog application with posts that have titles and content.
Step 3: Build Projects
Hands-on practice is the best way to solidify your knowledge. Start small and gradually increase complexity.
Beginner Project Ideas:
- To-Do List App: Create an app to manage daily tasks.
- Blog Platform: Build a platform to write, edit, and share articles.
- Recipe Organizer: Design an app to store and categorize recipes.
Step 4: Join the Community
Ruby and Rails have vibrant communities. Participate in forums, attend meetups, and contribute to open-source projects to accelerate your learning.
Recommended Resources:
- Railscasts: Video tutorials on Rails topics.
- Ruby Weekly: A newsletter with updates and tips.
- GitHub: Explore and contribute to Rails projects.
Step 5: Work with Experts
If you’re looking for guidance or want to see how professionals use Ruby and Rails, partnering with a team like Prateeksha Web Design can give you valuable insights and hands-on experience.
Conclusion
Understanding the difference between Ruby and Ruby on Rails is crucial for any aspiring web developer. Ruby is the versatile language that forms the foundation, while Rails is the powerful framework that brings your web development ideas to life. Together, they’ve empowered countless developers and businesses to create amazing digital experiences.
If you’re looking to build your next web project or just exploring these technologies, Prateeksha Web Design is here to guide you every step of the way. Let’s transform your ideas into reality with Ruby and Rails!
About Prateeksha Web Design
Prateeksha Web Design specializes in both Ruby and Ruby on Rails development, focusing on creating efficient, dynamic web applications. Ruby, a versatile programming language, offers flexibility in coding, while Ruby on Rails, a powerful framework, streamlines development with conventions and built-in tools. Their services include custom web applications, e-commerce solutions, and API development, leveraging the strengths of both technologies to enhance performance and scalability. Additionally, Prateeksha emphasizes best practices in code quality and maintainability, ensuring robust solutions for clients. With a dedicated team, they aim to deliver innovative digital experiences tailored to business needs.
Interested in learning more? Contact us today.
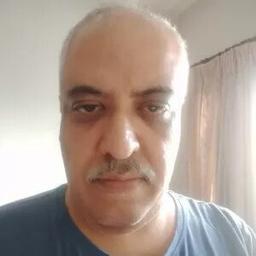