Welcome to the World of Environment Variables in Next.js!
Environment variables might sound like a scary technical term, but they’re one of the most practical and indispensable tools in a developer's toolkit. Think of them as your app’s secret code or backstage pass—crucial information that makes your app work but doesn’t need to be shared with the audience (aka your users). Now, let’s break it down in detail.
What Are Environment Variables?
Environment variables are small pieces of configuration data that you can use to control how your application behaves across different environments. Instead of hardcoding sensitive or changeable data (like API keys, database credentials, or service URLs) into your codebase, you store them in separate files. This approach keeps your app secure, maintainable, and adaptable.
Why Environment Variables Matter:
-
Security:
Imagine your app integrates with a payment gateway. The API key to communicate with that service is sensitive and should never be exposed to users. Environment variables keep this key hidden and accessible only to your app. -
Flexibility:
Your app might behave differently during development versus production. For instance, during development, you might point to a test API, while in production, you’d use the live API. Environment variables let you switch between these seamlessly. -
Maintainability:
Need to update a database connection string or an external service URL? Instead of hunting through your codebase, you update a single environment variable.
Why Should You Care About Environment Variables?
Let’s revisit the café analogy:
Running a web app is like running a café. Your environment variables are the secret recipes for your best-selling drinks. They’re crucial to your operations, but you wouldn’t print them on your menu or share them with just anyone.
For example:
- Public Recipe (NEXT_PUBLIC_API_URL):
Something everyone can know, like "Our lattes use freshly brewed coffee." - Secret Ingredient (API_KEY):
Something only you know, like the precise ratio of coffee to milk that makes your lattes irresistible.
In web development terms:
- Public Variables: These are exposed to both the server and the client. In Next.js, they are prefixed with
NEXT_PUBLIC_
to signify their availability on the client side. - Private Variables: These are accessible only on the server side, keeping them hidden from users.
How Environment Variables Enhance Your Web Development Game
-
Secure Configurations:
Your app can rely on sensitive credentials (e.g., a secret API key) without risking exposure. No more accidentally pushing secrets to GitHub! -
Organized Codebase:
By abstracting configurations into environment variables, your code remains clean and easy to understand. You don’t need to dig through lines of code to find that pesky API endpoint. -
Adaptability Across Environments:
With environment variables, your app can adapt to different environments (development, staging, and production) without requiring changes to the codebase.
How Next.js Uses Environment Variables
In Next.js, environment variables are like a magic toolbox. You simply define them in .env
files, and Next.js does the heavy lifting to make them available in your app. Depending on the environment, you can use different .env
files:
.env.local
: Used for your local development setup..env.development
: Used when running your app in a development environment..env.production
: Used when your app is running live in production.
For example, your .env.local
file might look like this:
NEXT_PUBLIC_API_URL=https://api.dev.example.com
API_KEY=supersecret123
Why It’s Important to Keep Your Secrets Secret
Let’s get real for a moment: pushing sensitive data (like your API keys) to your Git repository is like leaving your café’s backdoor open with a big “Come On In” sign. It’s an open invitation for trouble.
Environment variables keep your secrets safe by:
- Storing them locally in
.env
files (which are never shared). - Excluding
.env
files from version control using.gitignore
.
Example .gitignore
Entry:
# Ignore environment files
.env.local
.env.development
.env.production
Step-by-Step Setup of Environment Variables in Next.js
Step 1: Setting Up Your Next.js Project
To dive into environment variables, we first need a solid foundation—a working Next.js app. Here’s how to get started:
-
Create a New Next.js App: Open your terminal and run:
npx create-next-app@latest my-nextjs-app
This command:
- Installs the latest Next.js framework.
- Sets up a new project folder named
my-nextjs-app
. - Includes a default project structure to kickstart your app development.
-
Navigate into Your Project Directory: Move into the newly created project folder:
cd my-nextjs-app
-
Run the Development Server: Start the local server with:
npm run dev
This launches your app at
http://localhost:3000
, where you can see your live development environment. Success! 🎉
Step 2: Creating Your Environment Files
Now that your Next.js app is live, let’s set up the environment files where you’ll store your variables. Next.js supports a structured approach using .env
files, each serving a specific purpose:
-
Types of Environment Files:
.env.local
: For your local development environment. These variables are specific to your machine and are not shared with others..env.development
: Applied during development mode (npm run dev
). These variables can be shared if working in a team..env.production
: For production builds. These variables are used when your app is deployed.
Pro Tip: You can use different environment files to define configurations for specific deployment stages, making it easier to test and deploy.
-
Creating the
.env.local
File: In the root of your project, create a file named.env.local
. Add the following key-value pairs:NEXT_PUBLIC_API_URL=https://api.example.com SECRET_API_KEY=supersecret123
NEXT_PUBLIC_API_URL
: A public variable accessible on both the client and the server.SECRET_API_KEY
: A private variable that remains on the server.
Analogy: Think of
.env.local
as your personal notebook—it contains details for your eyes only when you’re coding locally.
Step 3: Accessing Environment Variables in Next.js
With environment variables in place, let’s access them in your Next.js application.
-
Understanding Public vs Private Variables:
- Public Variables:
- Must start with the prefix
NEXT_PUBLIC_
. - Accessible both on the server and the client side.
- Example:
NEXT_PUBLIC_API_URL
.
- Must start with the prefix
- Private Variables:
- Do not use the
NEXT_PUBLIC_
prefix. - Only available on the server side, ensuring sensitive data remains secure.
- Example:
SECRET_API_KEY
.
- Do not use the
- Public Variables:
-
Using Environment Variables in Your Code: Open the file
pages/index.js
and update it as follows:export default function Home() { console.log("Public API URL:", process.env.NEXT_PUBLIC_API_URL); console.log("Secret API Key:", process.env.SECRET_API_KEY); // This won't log on the client! return <h1>Hello, Next.js!</h1>; }
NEXT_PUBLIC_API_URL
: Logs to the browser’s console because it’s public.SECRET_API_KEY
: Logs only to the server console. If you check the browser’s console, you won’t see it—Next.js protects private variables from being exposed.
-
Testing Your Setup:
- Restart your development server (
npm run dev
) to ensure the.env.local
file is loaded. - Open the browser’s developer tools console to see the logged
NEXT_PUBLIC_API_URL
. - Verify that
SECRET_API_KEY
only appears in the terminal output where the server logs are displayed.
- Restart your development server (
Step 4: Handling Environment Variables Across Environments
You’ve heard the saying, “different strokes for different folks,” right? That’s the philosophy behind managing environment variables across different stages of your app’s lifecycle. Every environment—development, testing, staging, and production—has its unique needs, and environment variables let you configure each one without breaking a sweat.
Why Separate Environment Files?
Imagine you’re a chef. You use test kitchens to experiment with recipes and a grand kitchen for serving diners. Similarly:
- Development is your test kitchen, where you tinker with features.
- Production is your grand kitchen, where everything must be polished and perfect.
Different .env
files ensure that:
- You use test services (e.g., a mock API) in development.
- You use live, production-ready services when your app goes public.
Example: Setting Up Environment-Specific Files
-
Development Environment (
.env.development
):
For when you’re running the app locally withnpm run dev
.NEXT_PUBLIC_API_URL=https://dev.api.example.com
This might point to a development server with mock data or sandbox credentials.
-
Production Environment (
.env.production
):
For when you deploy the app withnpm run build
.NEXT_PUBLIC_API_URL=https://api.example.com
This points to your live, production-ready API endpoint.
Pro Tip: If you’re working in multiple environments (e.g., staging), you can also create a
.env.staging
file.
How Next.js Knows Which File to Use
When you run specific commands, Next.js automatically picks the correct environment file:
- Running
npm run dev
loads.env.development
. - Building your app with
npm run build
or starting it withnpm start
loads.env.production
.
No Manual Switching: You don’t need to specify which
.env
file to load—Next.js takes care of it for you. 🎉
Step 5: The Gotchas (And How to Avoid Them)
Every developer has encountered “gotchas” that cause unexpected headaches. Let’s tackle them before they bite:
1. Hot Reloading Doesn’t Detect Changes
Modified your .env.local
file but don’t see the changes reflected in your app? That’s because Next.js doesn’t watch for updates in environment files during development.
Solution:
Restart your development server whenever you change .env
files:
npm run dev
2. Forgetting to Prefix Public Variables
If you try to access a variable on the client side without the NEXT_PUBLIC_
prefix, it won’t work. Next.js enforces this to prevent accidental exposure of sensitive data.
Solution:
Always prefix client-exposed variables with NEXT_PUBLIC_
. For example:
NEXT_PUBLIC_WEATHER_API=https://weather.example.com
3. Pushing .env
Files to Git
Accidentally pushing sensitive .env
files to a public repository is a recipe for disaster. It’s like leaving your house keys on your front porch with a sign that says, “Come on in.”
Solution:
Add .env
files to .gitignore
to ensure they aren’t committed:
# Add this to .gitignore
.env.local
.env.development
.env.production
If you’ve already committed a .env
file, revoke any exposed keys and replace them immediately.
Step 6: Best Practices for Managing Environment Variables
Mastering environment variables is more than just knowing how to use them; it’s about managing them efficiently, securely, and collaboratively. Let’s dive into some best practices that will keep your setup robust and your team headache-free.
1. Use Descriptive Names
Imagine opening your .env
file and seeing variables like API_KEY
or URL
. Which API? Which URL? Descriptive names save the day!
- Instead of
API_KEY
, go forSTRIPE_API_KEY
orSENDGRID_API_KEY
. - Replace vague
URL
withNEXT_PUBLIC_WEATHER_API_URL
.
Why? It’s not just about clarity for you but for anyone who might work on the project later. Be future-you-friendly!
2. Centralize Configuration
Managing environment variables manually across multiple environments can get messy, especially in a team setting. Instead, use specialized tools to centralize and manage configurations securely.
Popular Tools:
- Doppler: Centralizes your environment variables and integrates seamlessly with CI/CD pipelines.
- Vault by HashiCorp: A powerful solution for securely managing secrets and access control.
Why Centralize?
- Ensures consistency across environments.
- Reduces human error by providing a single source of truth.
- Adds a layer of security and access control.
3. Validate Variables
One of the biggest risks in using environment variables is missing or misconfigured variables. A missing API_KEY
can break your app faster than you can say “bug report.”
Use dotenv-safe
to validate your .env
files. It ensures all required variables are defined before your app starts.
Setup:
- Install
dotenv-safe
:npm install dotenv-safe
- Create a
.env.example
file listing all required variables:NEXT_PUBLIC_WEATHER_API_URL= WEATHER_API_KEY=
- Now,
dotenv-safe
will cross-check.env
against.env.example
and warn you if anything is missing.
4. Keep Secrets Out of the Client
Public variables (prefixed with NEXT_PUBLIC_
) are embedded in your client-side JavaScript bundle. This makes them accessible to anyone using your app. Be mindful of what you expose.
Golden Rule:
- Sensitive Data (e.g., database credentials, API secrets): Keep them server-side.
- Non-Sensitive Data (e.g., feature flags, public API endpoints): Use
NEXT_PUBLIC_
for these.
Step 7: Real-Life Use Case Example
Let’s make this practical! Here’s a step-by-step example where we fetch weather data using environment variables in Next.js.
1. Add Your API Key
Create a .env.local
file and add the following variables:
NEXT_PUBLIC_WEATHER_API_URL=https://api.weather.com
WEATHER_API_KEY=supersecretapikey
NEXT_PUBLIC_WEATHER_API_URL
: This will be exposed to the client since it’s prefixed withNEXT_PUBLIC_
.WEATHER_API_KEY
: This stays private and is only accessible on the server.
2. Fetch Weather Data in a Server-Side Function
Use Next.js’s getServerSideProps
to fetch weather data securely on the server:
export async function getServerSideProps() {
const response = await fetch(`${process.env.NEXT_PUBLIC_WEATHER_API_URL}/forecast`, {
headers: {
Authorization: `Bearer ${process.env.WEATHER_API_KEY}`,
},
});
if (!response.ok) {
console.error("Failed to fetch weather data");
return { props: { forecast: null } };
}
const data = await response.json();
return { props: { forecast: data } };
}
- Why Server-Side?
TheWEATHER_API_KEY
is kept on the server, ensuring it’s not exposed to the client.
3. Display the Weather Data
In your component, render the weather forecast:
export default function Weather({ forecast }) {
if (!forecast) {
return <h1>Unable to fetch weather data. Please try again later.</h1>;
}
return (
<div>
<h1>Weather Forecast</h1>
<pre>{JSON.stringify(forecast, null, 2)}</pre>
</div>
);
}
4. Test It Locally
- Start your dev server:
npm run dev
- Open your app at
http://localhost:3000
. - See your weather forecast data displayed, fetched securely with environment variables.
Key Benefits of This Setup
- Security:
TheWEATHER_API_KEY
remains private, safe from prying eyes. - Flexibility:
You can switch to a different API endpoint for production by simply updating the.env.production
file. - Scalability:
Need to add more variables? Just update your.env
files—no code changes required.
Common Questions (And Their Answers)
Q: Can I use environment variables in custom scripts?
A: Absolutely! You can load them using libraries like dotenv
in Node.js scripts.
Q: Are environment variables secure in Next.js?
A: Yes, as long as private variables are not prefixed with NEXT_PUBLIC_
and .env
files are excluded from version control.
Q: How do I debug environment variables?
A: Use console.log(process.env)
on the server or process.env.NEXT_PUBLIC_VAR
on the client to see what’s available.
Why Prateeksha Web Design?
At Prateeksha Web Design, we make complex topics like configuring Next.js environment variables feel as easy as sipping your morning coffee. Our expertise in web development and practical solutions ensures your project not only runs smoothly but also stands out. Whether it’s Next.js, Shopify, or custom designs, we’re here to help you shine.
The Final Lap
Congratulations! You’ve mastered the basics of environment variables in Next.js. Now it’s your turn to set up a project, experiment with .env
files, and build something amazing.
💡 Pro Tip: Bookmark this guide—it’ll come in handy for future projects.
Your Next Step?
Explore more about environment variables, secure your configurations, and start building smarter apps today. If you ever feel stuck, the team at Prateeksha Web Design is just a click away. Let’s create something extraordinary together!
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services for managing environment variables in Next.js applications, ensuring seamless configuration and deployment. Their complete setup guide covers essential practices for defining and utilizing environment variables effectively. They provide step-by-step instructions, best practices for security, and insights on optimizing performance. Additionally, their expertise helps developers navigate common pitfalls and streamline their development workflow. Trust Prateeksha Web Design for a robust and efficient Next.js environment setup.
Interested in learning more? Contact us today.
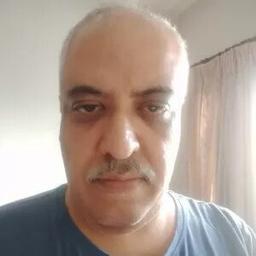