Setting Up Environment Variables in Next.js Projects: A Complete Program Geek Setup Guide
Introduction
Environment variables are a cornerstone of modern web development, offering a secure and flexible way to manage sensitive data and configuration details. In Next.js projects, they are essential for managing secrets like API keys, database credentials, and other project-specific configurations.
This blog is a Program Geek setup guide to help you understand how to effectively use Next.js environment variables. We'll dive into best practices, the latest updates in Next.js, and advanced strategies. Along the way, we’ll showcase Prateeksha Web Design’s expertise and why small businesses should consider their services for professional web development needs.
Why Use Environment Variables in Next.js?
Environment variables are essential for modern web development, especially in frameworks like Next.js. They allow developers to manage application configurations dynamically and securely without hardcoding sensitive or environment-specific data into the codebase. Let’s break this down further:
1. Secure Sensitive Data
Hardcoding sensitive data like API keys, database credentials, or third-party service tokens into your source code poses a significant security risk. If someone gains access to your code (through a public repository or a security breach), they could exploit these credentials. Using environment variables ensures sensitive information is stored in separate configuration files, inaccessible to the public.
For example:
- Instead of embedding an API key directly into your JavaScript file:
const apiKey = "12345-ABCDE";
- Store it in an environment variable:
API_KEY=12345-ABCDE
This isolates sensitive data, making your application safer and easier to maintain.
2. Simplify Configuration Management
Applications often need different configurations depending on the environment:
- Development: Uses local databases or testing APIs.
- Staging: Mimics the production environment for testing.
- Production: Runs live and uses real services.
Instead of manually switching configurations, environment variables automate this process. For example:
- A single
.env.development
file can contain:API_URL=http://localhost:3000
- While
.env.production
contains:API_URL=https://api.production.com
Next.js automatically selects the appropriate file based on the environment, making deployment seamless.
3. Enhance Scalability
When scaling an application, dynamic configuration becomes critical. Environment variables allow your application to adapt to different scenarios, such as:
- Switching databases or API endpoints for a new geographic region.
- Integrating third-party services for specific environments.
- Adjusting features dynamically without redeploying the app.
For example, in a multi-region app, you might use environment variables to route users to the nearest API endpoint:
NEXT_PUBLIC_API_URL=https://api.region1.example.com
NEXT_PUBLIC_API_URL_REGION2=https://api.region2.example.com
Your application logic can then select the appropriate endpoint dynamically, enhancing scalability and performance.
Understanding How Environment Variables Work in Next.js
Next.js makes working with environment variables straightforward by providing built-in support and specific conventions. Let’s explore these features in detail:
1. .env
Files
Next.js recognizes .env
files for defining environment variables. These files allow you to declare key-value pairs that Next.js will load automatically. The framework supports three main types of .env
files:
-
.env.local
:- Used for local development.
- Should not be committed to version control to keep sensitive data private.
- Example:
NEXT_PUBLIC_API_URL=http://localhost:3000 SECRET_KEY=local-secret
-
.env.development
:- Used for development builds when running
npm run dev
. - Example:
NEXT_PUBLIC_API_URL=http://dev.api.example.com
- Used for development builds when running
-
.env.production
:- Used during production builds (
npm run build
andnpm start
). - Example:
NEXT_PUBLIC_API_URL=https://api.example.com
- Used during production builds (
Next.js ensures that the correct .env
file is loaded based on the environment.
2. Prefix Requirement
To ensure a clear distinction between server-side and client-side variables, Next.js enforces a NEXT_PUBLIC_
prefix for any variable that needs to be accessed in the browser. This prevents accidental exposure of sensitive server-side data.
Example:
-
In your
.env
file:NEXT_PUBLIC_API_URL=https://api.example.com SECRET_KEY=super-secret-key
-
Access in your code:
// Client-side: Accessible because of NEXT_PUBLIC_ prefix const apiUrl = process.env.NEXT_PUBLIC_API_URL; // Server-side: Accessible but not exposed to the client const secretKey = process.env.SECRET_KEY;
Without the NEXT_PUBLIC_
prefix, the variable is considered private and can only be used on the server.
3. Using the Process Object
Environment variables in Next.js are accessed through the process.env
object. This is a standard way to work with environment variables in Node.js, and Next.js builds on this convention.
Example:
-
Defining Variables:
NEXT_PUBLIC_API_URL=https://api.example.com SECRET_KEY=my-secret-key
-
Accessing in Code:
const apiUrl = process.env.NEXT_PUBLIC_API_URL; // Browser-safe const secretKey = process.env.SECRET_KEY; // Server-side only
During the build process, Next.js injects these values into your application, ensuring they are available wherever needed.
Setting Up Environment Variables in a Next.js Project
Here’s a step-by-step guide to setting up and using environment variables in your Next.js application:
Step 1: Create a .env
File
- Navigate to your Next.js project directory.
- Create a
.env.local
file for local development. - Add your environment variables in the format:
NEXT_PUBLIC_API_URL=https://api.example.com SECRET_KEY=my-secret-key
Step 2: Access Variables in Code
Use process.env
to access the variables in your code:
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
const secretKey = process.env.SECRET_KEY;
Step 3: Restart the Development Server
After adding or updating environment variables, restart your Next.js development server to ensure the changes take effect:
npm run dev
Advanced Next.js Config Tips for Environment Variables
Managing environment variables in Next.js can be taken to the next level with advanced techniques that ensure safety, flexibility, and better development practices. Let’s dive into these advanced configurations and troubleshooting tips in detail.
Using TypeScript for Safer Configurations
When building a Next.js project with TypeScript, defining types for your environment variables ensures that you catch errors during development. This is particularly useful in large projects where multiple developers might interact with the same variables.
Why Use TypeScript for Environment Variables?
- Type Safety: Prevent runtime errors by ensuring the correct types are used.
- Documentation: Your environment variables become self-documented through type definitions.
- Error Detection: Catch issues like misspelled variable names or incorrect types during the development phase.
How to Set Up TypeScript Types for Environment Variables:
-
Create a
next-env.d.ts
File: This file will declare the type definitions forprocess.env
.declare namespace NodeJS { interface ProcessEnv { NEXT_PUBLIC_API_URL: string; // Must be a string SECRET_KEY: string; // Must be a string NODE_ENV: 'development' | 'production' | 'test'; // Must be one of these values } }
-
Example Usage: With TypeScript, you get autocomplete and error checking:
const apiUrl: string = process.env.NEXT_PUBLIC_API_URL; // No type mismatch const secretKey: string = process.env.SECRET_KEY;
This setup ensures that any typo or incorrect usage of the environment variables will result in a TypeScript error, giving you immediate feedback.
Loading Variables Dynamically
Dynamic loading of environment variables allows your application to adapt based on its deployment environment. This approach is especially helpful for applications deployed in multiple regions or environments.
How to Load Variables Dynamically:
-
Use the
NODE_ENV
Variable: TheNODE_ENV
variable indicates whether the app is running indevelopment
,production
, ortest
mode. -
Load Environment Variables Programmatically:
const isProd = process.env.NODE_ENV === 'production'; const apiUrl = isProd ? process.env.PROD_API_URL : process.env.DEV_API_URL; console.log(`Using API URL: ${apiUrl}`);
-
Benefit of Dynamic Loading:
- Reduces the need for multiple
.env
files. - Makes configuration flexible and environment-specific.
- Reduces the need for multiple
Example Scenario: For an app deployed in both the US and Europe, you can set region-specific API endpoints dynamically:
NEXT_PUBLIC_API_URL_US=https://api-us.example.com
NEXT_PUBLIC_API_URL_EU=https://api-eu.example.com
const region = process.env.REGION;
const apiUrl = region === 'EU'
? process.env.NEXT_PUBLIC_API_URL_EU
: process.env.NEXT_PUBLIC_API_URL_US;
Protecting Sensitive Variables
Sensitive data like database credentials, API secrets, or private keys should never be exposed to the browser. Exposing these can lead to serious security vulnerabilities.
Best Practices for Protecting Sensitive Data:
-
Avoid
NEXT_PUBLIC_
Prefix: Any variable prefixed withNEXT_PUBLIC_
is bundled into the client-side code and accessible in the browser.Example of what not to do:
NEXT_PUBLIC_SECRET_KEY=my-secret-key
-
Use Server-Side Variables Only: Sensitive variables should be kept without the
NEXT_PUBLIC_
prefix and accessed only in server-side logic.Example:
export default function handler(req, res) { const secretKey = process.env.SECRET_KEY; // Accessible only on the server res.status(200).send(`Using secret key: ${secretKey}`); }
-
Audit Your
.env
Files: Regularly review your.env
files to ensure no sensitive information is accidentally exposed. -
Exclude Sensitive Files from Version Control: Add
.env.local
to your.gitignore
file to prevent it from being committed:# .gitignore .env.local
Troubleshooting Common Issues
Even with the best configurations, you might face issues while using environment variables. Let’s explore some common problems and their solutions.
Variables Not Loading
Symptoms:
process.env.VARIABLE_NAME
isundefined
.
Possible Causes:
- The
.env
file is not in the root directory of your project. - You forgot to restart the server after adding or modifying the
.env
file.
Solution:
- Ensure the
.env
file is in the project root directory. - Restart the development server to load the updated variables:
npm run dev
Undefined Variables
Symptoms:
- You see errors like
process.env.VARIABLE_NAME is not defined
.
Possible Causes:
- Typos in variable names in either the
.env
file or the code. - Missing
NEXT_PUBLIC_
prefix for variables used in the browser.
Solution:
- Double-check the spelling of your variables in both the
.env
file and the code. - Add the
NEXT_PUBLIC_
prefix if the variable needs to be accessible on the client side.
Overwriting Defaults
Symptoms:
- Incorrect values are being loaded, even though the
.env
file is correct.
Possible Causes:
- Conflicting variables across
.env
files (e.g.,.env.local
,.env
). - Incorrect loading order.
Solution:
-
Understand the Loading Order: Next.js loads
.env
files in the following order:.env.local
.env.development
or.env.production
.env
Variables in
.env.local
override those in other.env
files. Be mindful of this hierarchy. -
Example Scenario: If both
.env.local
and.env
contain:API_URL=http://local-url.com
The
.env.local
value will take precedence during local development.
Recent Advancements in Environment Variable Handling
Next.js has evolved significantly over recent versions, introducing robust features for managing environment variables. These enhancements streamline configuration management and improve performance, security, and developer experience. Let’s explore these advancements in detail.
1. Static Environment Variables in next.config.js
One of the most notable improvements in Next.js is the ability to define static environment variables directly in the next.config.js
file. This approach simplifies build-time variable management and optimizes performance.
What Are Static Environment Variables? Static environment variables are injected into the build process and remain constant throughout the lifecycle of the application. These variables are ideal for values that do not change based on runtime conditions, such as API endpoints or feature flags.
How to Define Static Variables in next.config.js
:
You can specify environment variables within the env
property of the next.config.js
file:
module.exports = {
env: {
NEXT_PUBLIC_API_URL: 'https://api.example.com', // Publicly accessible variable
SECRET_KEY: 'my-static-secret-key', // Server-side only variable
},
};
Benefits of Using Static Variables:
- Performance Optimization:
- Since these variables are injected during the build process, the runtime does not need to process them dynamically, improving performance.
- Consistency:
- Ensures that all builds use the same environment variables, reducing inconsistencies between development and production environments.
- Ease of Access:
- Variables are accessible through
process.env
just like those defined in.env
files.
- Variables are accessible through
Example Use Case: If your application uses a third-party API, you can define the base URL as a static variable:
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
This approach is particularly beneficial for applications deployed in static environments like Vercel or Netlify.
2. Environment Variables in Middleware
Another powerful advancement in Next.js is the ability to use environment variables within middleware. Middleware functions in Next.js are used to modify requests and responses or execute logic during the routing process.
What Makes This Feature Valuable? Environment variables in middleware allow developers to implement advanced functionality based on configurations. Examples include:
- Geo-based API routing.
- Conditional authentication logic.
- Feature flag management.
How to Use Environment Variables in Middleware: Middleware can access environment variables directly, enabling flexible and secure handling of requests.
Example Middleware Using Environment Variables:
import { NextResponse } from 'next/server';
export function middleware(req) {
const apiUrl = process.env.NEXT_PUBLIC_API_URL; // Public variable
const secretKey = process.env.SECRET_KEY; // Server-side variable
// Conditional logic based on environment variables
if (!secretKey) {
return NextResponse.error();
}
return NextResponse.rewrite(`${apiUrl}/new-path`);
}
Benefits of Using Environment Variables in Middleware:
- Dynamic Routing:
- Use environment variables to route users based on their region, language, or other factors.
- Enhanced Security:
- Middleware can securely access sensitive variables without exposing them to the client.
- Improved User Experience:
- Dynamically adapt responses, such as serving localized content, without requiring additional server-side logic.
Example Use Case: For an international e-commerce site, you could use middleware and region-specific API endpoints to serve localized content:
const region = req.geo.country;
const apiUrl = region === 'US'
? process.env.NEXT_PUBLIC_API_URL_US
: process.env.NEXT_PUBLIC_API_URL_EU;
SEO Optimization Tips for Using Environment Variables
While environment variables themselves do not directly impact SEO, their effective management can enhance performance, security, and reliability, which are crucial for SEO success. Let’s explore how.
1. Faster Load Times
Dynamic Configuration for Improved Performance: Environment variables can help reduce latency by dynamically configuring API endpoints based on a user’s location. Faster load times lead to better user experience and improved SEO rankings.
Example: Use environment variables to route users to the nearest API endpoint:
const apiUrl = region === 'US'
? process.env.NEXT_PUBLIC_API_URL_US
: process.env.NEXT_PUBLIC_API_URL_EU;
This setup ensures users get faster responses, improving Core Web Vitals—a critical factor in SEO rankings.
2. Security Enhancements to Build Trust
Search engines consider the trustworthiness of your website when determining rankings. Proper handling of environment variables prevents data leaks, ensuring that sensitive information like API keys or database credentials is never exposed.
Best Practices to Enhance Trustworthiness:
- Use
NEXT_PUBLIC_
prefix only for variables that must be accessible in the browser. - Avoid embedding sensitive data in public variables.
- Regularly audit your
.env
files to ensure no unnecessary variables are exposed.
Example:
-
Incorrect:
NEXT_PUBLIC_SECRET_KEY=my-super-secret-key
This exposes the secret key to the client.
-
Correct:
SECRET_KEY=my-super-secret-key
By securing sensitive variables, you build a trustworthy application, which indirectly boosts SEO by ensuring users and search engines trust your platform.
3. Leveraging Server-Side Rendering (SSR) for SEO
Environment variables enable dynamic server-side rendering (SSR), ensuring that your pages are optimized for search engines.
Example Use Case: Dynamically fetch metadata for your pages based on environment variables:
export async function getServerSideProps() {
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
const res = await fetch(`${apiUrl}/metadata`);
const metadata = await res.json();
return {
props: { metadata },
};
}
This approach allows you to serve SEO-friendly pages with dynamic content tailored to user needs.
Why Choose Prateeksha Web Design for Your Next.js Projects
Prateeksha Web Design specializes in custom web development, including configuring secure and scalable Next.js projects. Here’s why small businesses should consider their services:
- Expertise: Years of experience in creating high-performing, SEO-friendly websites.
- Authority: Proven track record of successful projects for small businesses.
- Trustworthiness: A focus on secure coding practices and client satisfaction.
Whether you need help setting up Next.js environment variables or creating an entire e-commerce platform, Prateeksha Web Design ensures a professional and seamless experience.
Conclusion
Setting up Next.js environment variables is an essential skill for any developer looking to build secure and efficient web applications. By following this Program Geek setup guide, you can confidently manage your application’s configurations and improve your development workflow.
About Prateeksha Web Design
Prateeksha Web Design offers expert services in setting up environment variables in Next.js projects. Our team can help define and securely manage environment variables for your project, ensuring smooth deployment and operation. We specialize in configuring environment variables for various environments such as development, staging, and production. Trust us to handle all aspects of setting up environment variables to enhance the functionality of your Next.js projects. Contact us for personalized solutions tailored to your project needs.
Interested in learning more? Contact us today.
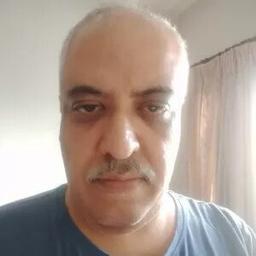