In the world of modern web development, Node.js stands tall as a go-to platform for building fast, scalable, and efficient backend systems. For 2025, the stakes are higher, with advancements in technology pushing developers to optimize every aspect of their code. If you're venturing into the world of Node.js backend development, you're in for an exciting ride. This guide breaks down the best practices for 2025, ensuring your backend systems are robust, secure, and lightning-fast.
At Prateeksha Web Design, we've mastered the art of leveraging Node.js to deliver cutting-edge solutions for businesses worldwide. Whether you're a newbie coder or a seasoned developer, these insights will set you on the path to backend development greatness.
Why Choose Node.js for Backend Development?
Node.js has gained immense popularity in recent years, and for good reason. It offers a unique blend of performance, scalability, and ease of use, making it the backbone of modern web development. Let’s dive deeper into the reasons why Node.js stands out as the go-to choice for backend development in 2025.
1. Asynchronous and Non-Blocking I/O
One of the defining features of Node.js is its asynchronous and non-blocking I/O capabilities, which are a game-changer for handling multiple tasks simultaneously.
-
How It Works:
- Traditional backend systems often operate in a blocking manner, meaning each request must complete before the next one starts.
- In contrast, Node.js uses an event-driven architecture, where tasks are queued and executed asynchronously without waiting for previous tasks to finish.
-
Real-World Benefits:
- Efficiency: Handles thousands of concurrent requests without overloading the server.
- Real-Time Applications: Ideal for apps like chat platforms, live dashboards, and online gaming that demand real-time updates.
Example:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!\n');
});
server.listen(3000, () => console.log('Server running on port 3000'));
In this example, the server can process multiple incoming requests simultaneously without waiting for one to complete before starting another.
2. Speed and Performance
Node.js is built on Google Chrome’s V8 JavaScript engine, which is renowned for its speed and efficiency. This engine compiles JavaScript code directly into machine code, bypassing the slower interpretation process.
-
Why It’s Fast:
- V8 Engine: The underlying technology optimizes execution for high-speed performance.
- Event Loop: A single-threaded event loop processes multiple requests efficiently without creating new threads for each request.
-
Practical Use Cases:
- Streaming Applications: Platforms like Netflix use Node.js to handle high-speed video streaming.
- Data-Intensive Tasks: It’s a great fit for applications processing large datasets, such as financial analytics platforms.
3. Scalability
Scalability is one of Node.js’s strongest features, making it a favorite for businesses aiming to grow their digital platforms.
-
Horizontal and Vertical Scalability:
- Node.js allows applications to scale horizontally by adding more servers or vertically by enhancing the capacity of a single server.
- Built-in tools like the Cluster module enable applications to utilize multi-core processors, improving performance.
-
Microservices Architecture:
- Node.js works seamlessly with microservices, where individual components of an application can be scaled independently.
Example of clustering:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello from Node.js!\n');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
This code utilizes all CPU cores, ensuring optimal use of system resources.
4. Single Language for Frontend and Backend
One of the most compelling reasons to choose Node.js is its ability to unify frontend and backend development under one language: JavaScript.
-
Why It Matters:
- Consistency: Developers can use the same syntax, libraries, and tools across the entire application.
- Reduced Learning Curve: Teams don’t need to learn multiple languages, which simplifies onboarding and collaboration.
- Code Reusability: Common logic (e.g., validation or data formatting) can be shared between client and server.
-
Practical Example:
- Frameworks like Next.js and Express.js streamline development by providing powerful tools for both client and server-side coding.
- A developer familiar with JavaScript can easily switch between frontend (e.g., React.js) and backend tasks.
5. Vast Ecosystem
The Node.js ecosystem, powered by the npm (Node Package Manager), is one of the richest in the development world. It offers over a million open-source packages, covering almost any functionality a developer could need.
-
Why It’s Useful:
- Time-Saving: Instead of building features from scratch, developers can rely on well-maintained packages.
- Community Support: A massive community ensures that popular packages are constantly updated and well-documented.
- Versatility: npm supports everything from simple utility libraries to advanced frameworks and tools.
-
Examples of Popular npm Packages:
- Express.js: Simplifies building web applications and APIs.
- Socket.io: Enables real-time communication for chat and collaboration apps.
- Mongoose: Makes it easy to work with MongoDB databases.
- Passport.js: Provides robust authentication solutions.
Example of integrating a package:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Welcome to Node.js with Express!');
});
app.listen(3000, () => console.log('Server is running on port 3000'));
In just a few lines of code, you can create a fully functional server using Express.js.
Best Practices for Node.js Backend Development in 2025
Node.js continues to be a favorite for backend development due to its versatility and efficiency. However, creating a robust, secure, and maintainable Node.js application requires adhering to best practices. Here’s a detailed breakdown of how to optimize your Node.js backend development in 2025.
1. Structure Your Code for Scalability
A well-structured codebase is crucial for maintaining and scaling your application. Without organization, even small changes can become tedious and error-prone.
Key Strategies:
-
Modularization:
- Break your application into manageable modules.
- Each module should handle a specific responsibility, such as routes, database queries, or business logic.
-
MVC Pattern (Model-View-Controller):
- The MVC pattern separates your application into three interconnected components:
- Model: Manages the database and data-related logic.
- View: Handles the presentation layer (optional for APIs).
- Controller: Handles the application's logic and user input.
- The MVC pattern separates your application into three interconnected components:
-
Configuration Management:
- Store sensitive data like API keys, database credentials, or environment-specific settings in dedicated configuration files or environment variables.
Example Folder Structure:
project/
├── controllers/ # Logic for processing requests
├── models/ # Database schemas and queries
├── routes/ # Application endpoints
├── services/ # Business logic or third-party integrations
├── config/ # Application configurations
├── utils/ # Helper functions
└── app.js # Application entry point
Benefits:
- Improved code readability and maintainability.
- Easier debugging and testing.
- Enhanced scalability as your application grows.
2. Leverage Modern JavaScript Features
The JavaScript ecosystem is constantly evolving. To write cleaner, more efficient code, use the latest ECMAScript features supported by Node.js.
Must-Use Features in 2025:
-
Async/Await:
- Replace callbacks with async/await for better readability and error handling.
- Avoid callback hell and make your code cleaner.
Example:
async function getUserData(userId) { try { const user = await fetchUserFromDatabase(userId); return user; } catch (error) { console.error("Error fetching user:", error); } }
-
Destructuring:
- Extract values from objects and arrays more efficiently.
Example:
const { name, email } = user; console.log(`Name: ${name}, Email: ${email}`);
-
Optional Chaining and Nullish Coalescing:
- Access deeply nested properties safely using
?.
. - Provide default values using
??
.
Example:
const userName = user?.profile?.name ?? "Guest"; console.log(userName); // Output: "Guest" if name is undefined or null.
- Access deeply nested properties safely using
-
Spread and Rest Operators:
- Simplify object and array manipulation.
Example:
const updatedUser = { ...user, email: "newemail@example.com" };
Benefits:
- Cleaner and more maintainable code.
- Fewer bugs caused by outdated syntax.
- Improved developer productivity.
3. Use Environment Variables Securely
Hardcoding sensitive information into your code is a recipe for disaster. Instead, use environment variables to securely store configuration data.
Steps to Use Environment Variables:
-
Install dotenv Package:
- Install dotenv to manage environment variables:
npm install dotenv
- Install dotenv to manage environment variables:
-
Create a
.env
File:- Place all sensitive data here:
DB_HOST=localhost DB_USER=admin DB_PASS=supersecretpassword API_KEY=your_api_key
- Place all sensitive data here:
-
Load Variables in Your Code:
require('dotenv').config(); const dbHost = process.env.DB_HOST; const dbUser = process.env.DB_USER; const apiKey = process.env.API_KEY; console.log(`Connecting to database at ${dbHost} with user ${dbUser}`);
Security Tips:
- Never commit your
.env
file to version control (e.g., Git). Add it to your.gitignore
file. - Use environment-specific
.env
files, such as.env.development
and.env.production
. - Consider using services like AWS Secrets Manager or Azure Key Vault for sensitive data in production.
Benefits:
- Keeps sensitive data out of your codebase.
- Simplifies configuration for different environments.
- Reduces the risk of accidental data exposure.
4. Secure Your Application
Security is a non-negotiable aspect of backend development in 2025. Implement these measures to safeguard your Node.js application:
-
Helmet for HTTP Headers:
- Use Helmet to add secure HTTP headers and protect against vulnerabilities like XSS and clickjacking.
const helmet = require('helmet'); app.use(helmet());
-
Input Validation:
- Use libraries like Joi or Express Validator to validate user inputs.
const Joi = require('joi'); const schema = Joi.object({ username: Joi.string().min(3).required(), password: Joi.string().min(8).required() }); const result = schema.validate(req.body); if (result.error) { res.status(400).send(result.error.details[0].message); }
-
Hash Passwords:
- Use bcrypt to hash user passwords before storing them in your database.
const bcrypt = require('bcrypt'); const saltRounds = 10; const hashedPassword = await bcrypt.hash(password, saltRounds);
-
Rate Limiting:
- Use rate-limiting middleware to prevent brute-force attacks.
const rateLimit = require('express-rate-limit'); const limiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100 // limit each IP to 100 requests per windowMs }); app.use(limiter);
5. Monitor and Log Effectively
Effective monitoring and logging ensure you can detect and resolve issues quickly.
-
Use Winston for Logging:
- Capture and manage logs with structured formats.
const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.File({ filename: 'error.log', level: 'error' }), new winston.transports.Console() ] });
-
Monitor with PM2:
- PM2 is a process manager for Node.js that helps monitor and manage application performance.
npm install pm2 -g pm2 start app.js
6. Testing and Debugging
A robust Node.js backend requires thorough testing to ensure reliability and maintainability. Here’s how you can effectively test and debug your application in 2025:
Testing Tools
-
Mocha and Chai:
- Mocha: A flexible testing framework for writing unit and integration tests.
- Chai: A popular assertion library for Node.js that works well with Mocha.
- Use Case: Ideal for testing APIs, services, and business logic.
Example:
const chai = require('chai'); const expect = chai.expect; describe('User Service', () => { it('should return user data', () => { const user = getUser(1); expect(user).to.have.property('name'); }); });
-
Jest:
- A powerful testing framework by Facebook, offering a comprehensive solution for JavaScript testing.
- Features: Snapshot testing, built-in mocking, and seamless integration with TypeScript.
Example:
test('adds 1 + 2 to equal 3', () => { expect(1 + 2).toBe(3); });
Debugging Tools
-
Node.js Inspector:
- Node.js includes an inspector that allows debugging directly via Chrome DevTools.
- Usage:
node --inspect app.js
-
VSCode Debugger:
- Visual Studio Code provides an integrated debugging experience.
- Configure the debugger in the
launch.json
file to set breakpoints, step through code, and inspect variables.
Example
launch.json
:{ "version": "0.2.0", "configurations": [ { "type": "node", "request": "launch", "name": "Debug Node.js App", "program": "${workspaceFolder}/app.js" } ] }
7. Use TypeScript for Type Safety
TypeScript is essential in 2025 for building large, scalable applications. It introduces static typing to JavaScript, reducing runtime errors and improving code quality.
Benefits of TypeScript
-
Type Safety:
- Prevents errors by catching type-related issues during development.
-
Better Tooling:
- TypeScript enhances IDE support with better autocompletion, refactoring tools, and error detection.
-
Easier Maintenance:
- Strongly typed code is more predictable and easier to refactor.
Setting Up TypeScript in Node.js
-
Install TypeScript:
npm install typescript --save-dev
-
Initialize TypeScript Configuration:
npx tsc --init
-
Basic Example:
interface User { id: number; name: string; } const getUser = (id: number): User => { return { id, name: "John Doe" }; }; console.log(getUser(1));
-
Compile TypeScript to JavaScript:
npx tsc
8. Monitor and Log
Monitoring and logging are critical for maintaining the health of your Node.js application.
Monitoring Tools
-
PM2:
- A process manager that helps monitor, restart, and manage Node.js applications.
- Usage:
npm install pm2 -g pm2 start app.js
-
Application Performance Monitoring (APM):
- Use tools like New Relic or Datadog to track performance metrics, identify bottlenecks, and debug production issues.
Logging Tools
-
Winston:
- A powerful logging library that supports multiple transports (e.g., console, files, or cloud).
- Example:
const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.Console(), new winston.transports.File({ filename: 'app.log' }) ] }); logger.info('This is an info message');
-
Log Rotation:
- Use log rotation tools to manage log file sizes and avoid disk space issues.
9. Containerize with Docker
Docker allows you to package and deploy your Node.js applications consistently across different environments.
Steps to Containerize a Node.js Application
-
Create a Dockerfile:
FROM node:14 WORKDIR /app COPY package.json . RUN npm install COPY . . CMD ["node", "app.js"]
-
Build the Docker Image:
docker build -t my-node-app .
-
Run the Docker Container:
docker run -p 3000:3000 my-node-app
-
Advantages of Docker:
- Consistency: Ensures the same environment across development, testing, and production.
- Simplified Deployment: Deploy containers with minimal effort.
10. Stay Updated
The Node.js ecosystem is constantly evolving, with regular updates and new features. Staying current ensures your application remains secure and efficient.
How to Stay Updated
-
Update Dependencies:
- Use
npm outdated
to identify outdated packages:npm outdated
- Use
-
Follow Node.js Releases:
- Subscribe to the Node.js blog or GitHub repository for release updates.
-
Security Audits:
- Regularly audit your dependencies for vulnerabilities:
npm audit npm audit fix
- Regularly audit your dependencies for vulnerabilities:
-
Participate in Communities:
- Engage in forums, GitHub discussions, and Node.js meetups to stay ahead.
Prateeksha Web Design: Your Node.js Partner
At Prateeksha Web Design, we specialize in building powerful Node.js backend solutions tailored to your needs. From scalable architectures to secure APIs, we bring expertise and innovation to the table. Whether you're launching a startup or optimizing an existing platform, we've got you covered.
Final Thoughts
Node.js backend development in 2025 is about more than just writing code—it's about crafting systems that are efficient, secure, and future-proof. By following these best practices, you'll not only build robust applications but also set yourself apart as a developer.
Ready to take your backend to the next level? Let Prateeksha Web Design help you achieve your goals with our tailored Node.js solutions.
About Prateeksha Web Design
Prateeksha Web Design offers cutting-edge Node.js backend development services focused on best practices for 2025. Their approach emphasizes modular architecture, ensuring scalability and maintainability. They prioritize security by implementing robust authentication and authorization mechanisms. With an emphasis on performance optimization, they utilize asynchronous programming to enhance responsiveness. Continuous integration and deployment workflows are integrated to streamline development and ensure high-quality releases.
Interested in learning more? Contact us today.
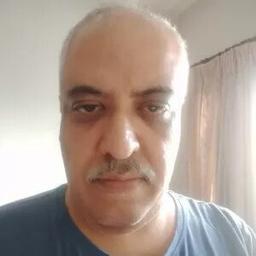