In the ever-evolving world of web development, React.js stands out as a game-changer. It's not just another front-end framework; it’s your ticket to building sleek, interactive, and dynamic web apps that captivate users. Whether you’re a coding newbie or someone transitioning into web development, this guide will unravel the magic of React.js, equipping you with the skills to create your first dynamic web app.
At Prateeksha Web Design, we’ve been at the forefront of creating stunning web solutions using React.js. Our expertise lies in turning concepts into engaging digital experiences. But enough about us—let's dive into the world of React.js!
What Is React.js and Why Should You Care?
React.js, commonly referred to as React, is a JavaScript library developed by Facebook. It’s specifically designed for building dynamic and interactive user interfaces (UIs) for web applications. Unlike traditional methods where the entire page reloads to reflect changes, React enables developers to create single-page applications (SPAs) where updates happen seamlessly without disrupting the user experience.
React is all about making development efficient, scalable, and fun, especially with its focus on reusable components. These components simplify the process of building complex applications by breaking the UI into small, independent, and reusable blocks of code. Let’s explore why React is such a revolutionary tool in modern web development.
Why Should You Care About React.js?
React.js isn’t just another buzzword in the tech world—it’s a practical and powerful solution for creating dynamic web apps. Here’s why React should be your go-to tool:
1. Component-Based Architecture
At the heart of React is its component-based architecture, a design philosophy that simplifies the development of large applications.
-
What Does It Mean?
Instead of managing a monolithic codebase, you split the UI into smaller, reusable pieces called components. Each component manages its logic, state, and rendering, making your app easier to build and maintain. -
Example:
Imagine building a blog. Instead of coding the header, footer, and content repeatedly, you create each as a reusable component. This approach ensures consistency and reduces redundant code.function Header() { return <header>Welcome to My Blog</header>; } function Footer() { return <footer>All Rights Reserved</footer>; }
2. Virtual DOM for Lightning-Fast Performance
React uses a Virtual DOM to make your web app incredibly fast and efficient.
-
How It Works:
The Virtual DOM is a lightweight copy of the actual DOM. Whenever you make changes to your app (e.g., a user clicks a button), React updates the Virtual DOM first. It then calculates the minimal changes needed to update the actual DOM. -
Why It Matters:
This process minimizes heavy operations, leading to faster performance and a smoother user experience, even in complex apps.
3. Rich Ecosystem and Strong Community Support
React isn’t just a tool—it’s a thriving ecosystem supported by an extensive community of developers.
-
Why This Is Beneficial:
- Access to numerous libraries and tools like React Router, Redux, and Material-UI.
- Tons of tutorials, forums, and open-source contributions to guide you.
- Regular updates and innovations backed by Facebook ensure its longevity.
-
Example:
If you want to add routing to your app, React Router is just an npm install away:npm install react-router-dom
4. SEO-Friendly
Search Engine Optimization (SEO) is crucial for any web app, and traditional JavaScript frameworks often struggle here. React solves this problem with server-side rendering (SSR).
- Why It’s Important:
By rendering React components on the server before sending them to the browser, search engines can index your content more effectively, improving visibility in search results.
5. Versatility Across Platforms
React isn’t limited to web development. With tools like React Native, you can use the same React principles to build mobile apps for iOS and Android.
- Bonus: You write once and deploy everywhere.
6. Learning Curve: Ideal for Beginners
React is designed to be intuitive and beginner-friendly:
- Familiar syntax: JSX feels like writing HTML within JavaScript.
- Plenty of resources for learning, such as the official React documentation, YouTube tutorials, and community blogs.
- A large library of pre-built components to kickstart your projects.
Why React.js Rocks for Beginners
- Component-Based Architecture: Start with simple components and gradually build complex apps.
- Virtual DOM: Worry less about performance and more about functionality.
- Rich Ecosystem: Everything you need is just a library away.
- SEO-Friendly: Even your first React app can rank well on Google.
React.js is your ultimate playground for mastering modern web development and building apps that feel smooth, dynamic, and professional.
Getting Started with React.js
Now that you know why React is awesome, let’s set up your environment and start coding.
Step 1: Setting Up Your Environment
-
Install Node.js and npm
React relies on Node.js for running JavaScript outside the browser and npm (Node Package Manager) for managing dependencies.- Download Node.js from nodejs.org.
- Check installation:
node -v npm -v
-
Set up a Code Editor
- Use Visual Studio Code (VS Code) for its React-friendly features like IntelliSense, debugging tools, and extensions.
-
Create Your First React App
Use thecreate-react-app
tool to set up a boilerplate project.npx create-react-app my-app cd my-app npm start
Once you run these commands, you’ll see a default React app running at
http://localhost:3000
.
Step 2: Understanding the File Structure
A typical React app has the following structure:
-
src/
This is your main workspace. It contains:App.js
: The root component where everything comes together.index.js
: The entry point for rendering your React app.
-
public/
Houses static files like images andindex.html
. React injects your app into thediv
with the IDroot
inindex.html
. -
node_modules/
Stores all your app’s dependencies.
Breaking Down React Basics
1. Components: The Building Blocks
In React, everything is a component. Think of components as LEGO blocks—you assemble them to create a complete app.
Here’s a simple example of a React component:
import React from 'react';
function Greeting() {
return <h1>Hello, React!</h1>;
}
export default Greeting;
Save this as Greeting.js
in your src
folder, and you’ve just created your first React component!
2. JSX: The Syntax Sugar
React uses JSX (JavaScript XML), a syntax extension that allows you to write HTML-like code inside JavaScript.
Example:
const element = <h1>Welcome to React.js!</h1>;
Under the hood, this gets converted to React.createElement()
calls.
3. Props: Passing Data
Props (short for "properties") allow you to pass data between components.
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
Use it like this:
<Welcome name="John" />
4. State: Managing Data Dynamically
State is like a component’s memory. It stores data that changes over time.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
Building Your First Dynamic Web App
Let’s create a simple To-Do List app. It’s the perfect project to grasp React basics.
Step 1: Set Up Your Components
- Header Component: Displays the title.
- Input Component: Takes user input.
- List Component: Displays the to-dos.
Header Component
function Header() {
return <h1>My To-Do List</h1>;
}
Input Component
import React, { useState } from 'react';
function Input({ addItem }) {
const [task, setTask] = useState('');
const handleAdd = () => {
addItem(task);
setTask('');
};
return (
<div>
<input
type="text"
value={task}
onChange={(e) => setTask(e.target.value)}
/>
<button onClick={handleAdd}>Add</button>
</div>
);
}
export default Input;
List Component
function List({ items }) {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
export default List;
Bringing It All Together
In App.js
:
import React, { useState } from 'react';
import Header from './Header';
import Input from './Input';
import List from './List';
function App() {
const [tasks, setTasks] = useState([]);
const addItem = (task) => {
setTasks([...tasks, task]);
};
return (
<div>
<Header />
<Input addItem={addItem} />
<List items={tasks} />
</div>
);
}
export default App;
React Advanced: The Next Steps
After mastering the basics of React.js, it’s time to explore some advanced concepts and tools that will make your web applications more powerful, efficient, and scalable. Two major aspects to delve into are routing and state management. Together, they allow you to create feature-rich applications that handle navigation and data flows seamlessly.
1. Routing with React Router
When building web applications, you often need multiple pages or views, such as a homepage, an "About" page, or a "Contact Us" page. While React is designed for single-page applications (SPAs), it provides tools to handle navigation between these views dynamically without reloading the page.
This is where React Router comes into play.
What is React Router?
React Router is a popular library for handling routing in React applications. It allows you to:
- Create multi-page apps while staying within the SPA paradigm.
- Dynamically switch between views based on the URL.
- Pass data and parameters between pages (using query strings or route parameters).
Installing React Router
To use React Router, you’ll need to install it first:
npm install react-router-dom
Setting Up Routes
Here’s how to create a basic routing setup in your app:
-
Import necessary components from
react-router-dom
:import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
- Router: Wraps your app to enable routing functionality.
- Route: Defines a route and the component to render when the URL matches.
- Switch: Ensures only one route is rendered at a time.
-
Set up your routes in
App.js
:function App() { return ( <Router> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> </Switch> </Router> ); }
path
: Defines the URL for the route.component
: Specifies the component to render for the given path.exact
: Ensures that the route matches the exact path.
Dynamic Routing
React Router also supports dynamic routing, where you can pass parameters through the URL. For instance, if you have a blog and want to navigate to a specific post using an ID, you can do something like this:
-
Define a dynamic route:
<Route path="/post/:id" component={Post} />
-
Access the parameter in the component:
import { useParams } from 'react-router-dom'; function Post() { const { id } = useParams(); return <h1>Post ID: {id}</h1>; }
With React Router, navigating between pages becomes seamless, and you can add robust navigation features to your app effortlessly.
2. State Management with Redux
As your app grows, managing its state can become challenging. State refers to data that changes over time, such as user inputs, API responses, or UI elements like modals. React’s built-in state management using useState
and useReducer
works well for smaller apps, but for more complex apps, Redux becomes indispensable.
What is Redux?
Redux is a state management library that helps you:
- Store all your application’s state in a central location called the store.
- Share data across components without passing props through multiple layers.
- Debug state changes with powerful tools like the Redux DevTools.
Installing Redux
To start using Redux, install the following packages:
npm install redux react-redux
Key Concepts in Redux
- Store: The single source of truth that holds the state of your app.
- Actions: Plain JavaScript objects that describe what you want to do (e.g., ADD_ITEM or REMOVE_ITEM).
- Reducers: Pure functions that determine how the state changes in response to actions.
- Dispatch: A method to send actions to the store.
Basic Redux Example
-
Define Actions:
const increment = () => ({ type: 'INCREMENT' }); const decrement = () => ({ type: 'DECREMENT' });
-
Create a Reducer:
const counterReducer = (state = 0, action) => { switch (action.type) { case 'INCREMENT': return state + 1; case 'DECREMENT': return state - 1; default: return state; } };
-
Create a Store:
import { createStore } from 'redux'; const store = createStore(counterReducer);
-
Connect Redux to React: Use the
react-redux
library to connect your React app to the Redux store.import { Provider, useSelector, useDispatch } from 'react-redux'; function App() { return ( <Provider store={store}> <Counter /> </Provider> ); } function Counter() { const count = useSelector((state) => state); const dispatch = useDispatch(); return ( <div> <h1>{count}</h1> <button onClick={() => dispatch({ type: 'INCREMENT' })}>+</button> <button onClick={() => dispatch({ type: 'DECREMENT' })}>-</button> </div> ); }
Redux is especially useful for apps with complex workflows or when multiple components need access to the same data.
Why Choose React.js for Dynamic Web Apps?
Now that you’ve explored advanced features like routing and state management, let’s revisit why React.js is ideal for building dynamic web apps.
1. Scalability
React’s component-based architecture ensures that your app can grow without becoming unwieldy. Whether you’re building a simple blog or a full-fledged e-commerce platform, React adapts to your needs.
2. Community Support
With an enormous developer community and extensive resources, React makes troubleshooting, learning, and implementing new features easier. Libraries like React Router, Redux, and Material-UI are just a few examples of how the ecosystem supports your growth.
3. SEO and Performance
React apps deliver lightning-fast performance thanks to its Virtual DOM and the ability to implement server-side rendering (SSR) with tools like Next.js. This combination ensures that your app not only performs well but also ranks better on search engines.
Why Prateeksha Web Design Loves React.js
At Prateeksha Web Design, we specialize in creating dynamic web apps using React.js. Whether it’s a portfolio, an e-commerce store, or a custom dashboard, we harness React's power to deliver exceptional results.
Our expertise includes:
- Building responsive, performance-optimized apps.
- Implementing advanced features like state management and APIs.
- Creating reusable components for modular, clean code.
If you’re looking to bring your ideas to life, trust Prateeksha Web Design to make it happen!
Final Thoughts: React.js Is Just the Beginning
React.js is more than just a front-end framework; it’s a stepping stone to building dynamic web apps that leave a lasting impression. Whether you're a student, a budding developer, or a small business owner, React offers endless opportunities to innovate.
At Prateeksha Web Design, we believe in empowering you with tools and insights to succeed. If you’re ready to take the plunge, start your React journey today—and remember, we’re here to help every step of the way!
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services for beginners learning React.js, focusing on building dynamic web applications. Their offerings include interactive tutorials, hands-on project guidance, and customized training sessions to enhance coding skills. They provide essential resources and tools to help developers grasp core concepts effectively. Additionally, Prateeksha emphasizes best practices in web design and development for a seamless user experience. Beginners can benefit from ongoing support and community engagement to foster confidence and creativity in their projects.
Interested in learning more? Contact us today.
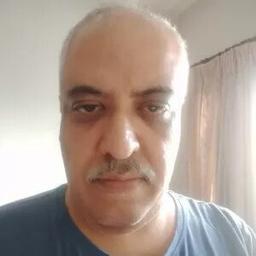