In today’s fast-evolving digital landscape, serverless app development is revolutionizing how developers create and deploy applications. This approach eliminates the need to manage servers, allowing you to focus on building features and delivering value to your users. One of the most powerful combinations for developing serverless apps is Next.js and Firebase. These technologies provide a robust framework for creating modern, scalable, and efficient web applications.
This comprehensive guide will walk you through the essentials of using Next.js with Firebase to build serverless apps. We’ll delve into the latest advancements, strategies, and best practices while showcasing Prateeksha Web Design's expertise in leveraging these tools for small businesses.
What is Next.js?
Next.js is a powerful React-based framework that simplifies the process of building modern web applications. Developed by Vercel, Next.js offers an opinionated setup that combines the flexibility of React with additional features to enhance performance, SEO, and developer productivity.
Unlike plain React, which is primarily focused on building user interfaces, Next.js takes care of the entire lifecycle of a web application—from server-side rendering to API creation. This makes it an excellent choice for developers looking to create robust, scalable, and lightning-fast applications.
Why is Next.js So Popular?
- Enhanced Performance: By supporting server-side rendering (SSR) and static site generation (SSG), Next.js ensures that pages load quickly, delivering content to users efficiently.
- SEO Optimization: SSR and SSG ensure that pages are crawlable by search engines, helping websites rank higher in search results.
- Developer Experience: Features like hot reloading, TypeScript support, and built-in tools simplify the development process.
- Flexibility: Next.js supports hybrid rendering models (combining SSR, SSG, and client-side rendering) for greater control over app performance.
Key Features of Next.js Explained in Detail
-
Server-Side Rendering (SSR):
With SSR, pages are rendered on the server for each incoming request and sent to the browser as fully rendered HTML. This improves first-page load performance and makes the app more SEO-friendly.- Example Use Case: News websites that display real-time data benefit from SSR since content can be updated on the fly before being delivered to users.
- How It Works:
export async function getServerSideProps(context) { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data } }; }
-
Static Site Generation (SSG):
SSG pre-builds HTML pages at build time, resulting in blazing-fast page loads. It’s ideal for content that doesn’t change frequently.- Example Use Case: Blogs, portfolio websites, or documentation sites, where content is updated periodically but doesn’t require dynamic rendering.
- How It Works:
export async function getStaticProps() { const res = await fetch('https://api.example.com/posts'); const posts = await res.json(); return { props: { posts } }; }
-
API Routes:
Next.js allows you to create server-side APIs within your project. These API routes eliminate the need for a separate backend server, simplifying the development stack.- Example Use Case: Building a contact form that submits user data to an email service or database directly through the API route.
- How It Works:
Create an API route in thepages/api
directory:export default function handler(req, res) { if (req.method === 'POST') { const data = req.body; res.status(200).json({ message: 'Data received', data }); } }
-
Image Optimization:
Next.js includes a built-in image optimization feature that automatically resizes and serves images in modern formats like WebP. This improves page speed and reduces bandwidth usage.- Example Use Case: E-commerce platforms, where high-quality images are essential but need to load quickly.
- How It Works: Use the
Image
component:import Image from 'next/image'; <Image src="/product.jpg" alt="Product Image" width={500} height={500} />;
-
File-Based Routing:
Navigation in Next.js is as simple as creating files in thepages
directory. Each file corresponds to a route in your application.- Example Use Case: Creating an About page by adding
about.js
in thepages
directory automatically creates the/about
route. - How It Works: No configuration is needed—just add files or folders in the
pages
directory.
- Example Use Case: Creating an About page by adding
What is Firebase?
Firebase is a powerful Backend-as-a-Service (BaaS) platform offered by Google. It provides a suite of tools and services that allow developers to build, manage, and scale applications without worrying about the complexities of server infrastructure. Firebase is especially popular among developers for its real-time capabilities, scalability, and ease of integration with modern frameworks like Next.js.
Why Firebase?
Firebase eliminates the need for setting up and maintaining servers, databases, or backend logic. Developers can focus on building features while Firebase handles the heavy lifting. With its pay-as-you-go pricing model, Firebase is cost-effective for startups and small businesses.
Core Features of Firebase Explained in Detail
-
Firebase Authentication:
Firebase makes implementing user authentication straightforward. It supports multiple providers, including email/password, Google, Facebook, and more.- Example Use Case: A social media app that needs secure user logins.
- How It Works:
import { getAuth, signInWithEmailAndPassword } from "firebase/auth"; const auth = getAuth(); signInWithEmailAndPassword(auth, email, password) .then((userCredential) => console.log(userCredential.user)) .catch((error) => console.error(error));
-
Firestore Database:
Firestore is a real-time, NoSQL database that scales automatically. It enables real-time updates to connected clients, making it perfect for collaborative or dynamic applications.- Example Use Case: A messaging app where users see new messages instantly.
- How It Works:
import { getFirestore, collection, getDocs } from "firebase/firestore"; const db = getFirestore(); const querySnapshot = await getDocs(collection(db, "messages")); querySnapshot.forEach((doc) => console.log(doc.data()));
-
Cloud Functions:
Cloud Functions allow you to run serverless backend code triggered by Firebase events (e.g., a new user signing up) or HTTP requests.- Example Use Case: Sending a welcome email to users after they sign up.
- How It Works:
Write your function and deploy it using the Firebase CLI:exports.sendWelcomeEmail = functions.auth.user().onCreate((user) => { const email = user.email; // Logic to send email });
-
Firebase Hosting:
Firebase Hosting is a fast and secure hosting solution for static and dynamic content. It integrates seamlessly with Next.js for deploying serverless applications.- Example Use Case: Hosting a Next.js blog site with pre-rendered pages.
- How It Works:
Deploy your site with a single command:firebase deploy
-
Firebase Analytics:
Firebase Analytics provides insights into user behavior, helping you make data-driven decisions.- Example Use Case: Tracking app usage to identify popular features.
- How It Works:
Add Firebase Analytics to your app, and view reports in the Firebase console.
Why Combine Next.js with Firebase?
Next.js and Firebase together form a powerful, flexible toolkit for building serverless applications. By leveraging the strengths of both technologies, developers can create highly-performant web apps that scale effortlessly and deliver exceptional user experiences. Let’s break down why this combination is so effective and why it’s a game-changer for modern web development.
Key Advantages of Combining Next.js and Firebase
-
Serverless Architecture
The term "serverless" doesn’t mean there are no servers—it means that developers don’t need to manage them. With Next.js, server-side rendering (SSR) and static site generation (SSG) occur without the hassle of provisioning servers, while Firebase provides serverless backend services like authentication, databases, and cloud functions.- How it Helps: This approach allows automatic scaling based on user demand. Whether you have 10 or 10 million users, the infrastructure adapts seamlessly.
- Example: An e-commerce platform using SSG for product pages and Firebase Firestore for real-time inventory updates.
-
Enhanced Developer Productivity
Both Next.js and Firebase are designed to streamline development. Next.js simplifies frontend tasks with built-in tools like routing and image optimization, while Firebase reduces backend complexity by offering pre-built services.- How it Helps: Developers can focus on creating features and improving user experiences rather than spending time on infrastructure.
- Example: Building a blog site with Next.js’s SSG and Firebase Hosting reduces the setup time to just hours instead of days.
-
Seamless Integration
Firebase tools integrate smoothly into Next.js projects. For example:- Authentication: Easily set up secure login systems using Firebase Authentication.
- Database: Manage and retrieve data in real-time using Firestore.
- Cloud Functions: Use serverless logic triggered by events like user sign-ups or form submissions.
- Example: A fitness app with user-generated workout plans uses Next.js for the UI, while Firebase Authentication and Firestore handle user accounts and data storage.
-
Improved SEO
Next.js’s server-side rendering (SSR) ensures that content is delivered as fully rendered HTML, making it crawlable by search engines. Firebase boosts this experience by providing fast hosting and seamless database interactions.- How it Helps: Websites rank higher on search engines, leading to increased organic traffic.
- Example: A local business directory site built with Next.js and Firebase can display fast-loading, SEO-optimized pages for each business listing.
-
Cost-Effective Development
Firebase’s pay-as-you-go pricing ensures affordability, especially for startups and small businesses. You only pay for what you use, whether it’s database reads, authentication logins, or cloud function invocations. Next.js complements this by reducing hosting costs with its static export options.- How it Helps: Minimizes costs while still delivering enterprise-level performance.
- Example: A small business selling artisanal products can build a professional-grade online store without incurring high server costs.
Getting Started with Next.js and Firebase
Here’s a detailed guide to help you set up a project combining Next.js and Firebase:
Step 1: Setting Up a Next.js Project
-
Install Node.js and Create a Next.js App:
Use the Next.js starter template to set up your project quickly.npx create-next-app my-app cd my-app
-
Run the Development Server:
Once the setup is complete, launch the development server.npm run dev
Open
http://localhost:3000
in your browser to view the starter app.
Step 2: Adding Firebase to Your Next.js Project
-
Install Firebase:
Add the Firebase SDK to your project.npm install firebase
-
Set Up Firebase in Your Project:
- Go to the Firebase Console and create a new project.
- Add a web app to your project and copy the configuration details (API key, project ID, etc.).
- Create a
firebase.js
file in your project to initialize Firebase:import { initializeApp } from "firebase/app"; const firebaseConfig = { apiKey: "your-api-key", authDomain: "your-auth-domain", projectId: "your-project-id", storageBucket: "your-storage-bucket", messagingSenderId: "your-messaging-sender-id", appId: "your-app-id", }; const app = initializeApp(firebaseConfig); export default app;
Step 3: Integrating Firebase Services into Next.js
-
Firebase Authentication:
Add user login functionality using Firebase Authentication:import { getAuth, signInWithEmailAndPassword } from "firebase/auth"; const auth = getAuth(); signInWithEmailAndPassword(auth, "email@example.com", "password123") .then((userCredential) => console.log(userCredential.user)) .catch((error) => console.error(error));
-
Firestore Database:
Fetch and display data in your app with Firestore:import { getFirestore, collection, getDocs } from "firebase/firestore"; const db = getFirestore(); const querySnapshot = await getDocs(collection(db, "posts")); querySnapshot.forEach((doc) => console.log(doc.data()));
-
Deploying Your App with Firebase Hosting:
Firebase Hosting ensures your app is served securely and efficiently.- Build your Next.js app:
npm run build
- Deploy to Firebase Hosting:
firebase deploy
- Build your Next.js app:
Real-World Example: Blogging Platform
- Frontend: Built with Next.js for SSR/SSG, ensuring fast page loads and SEO optimization.
- Backend: Firebase for user authentication, real-time database for posts and comments, and cloud functions for sending notifications.
- Hosting: Firebase Hosting for quick and secure deployment.
Building Core Features with Next.js and Firebase
Creating a robust and scalable application involves implementing key features such as authentication, real-time database management, and server-side logic. Using Next.js with Firebase, you can easily integrate these features into your app. Let’s dive into the details.
1. Authentication
Firebase Authentication simplifies user management by offering pre-built services for email/password authentication, social logins (e.g., Google, Facebook), and anonymous sign-ins. With Firebase Authentication, you can securely handle user credentials while reducing the risk of vulnerabilities.
Step-by-Step Guide to Integrate Authentication
-
Install Firebase Authentication: Begin by adding the Firebase Authentication module to your project:
npm install firebase/auth
-
Set Up Authentication: In your
firebase.js
file, initialize the authentication service:import { getAuth, signInWithEmailAndPassword } from "firebase/auth"; const auth = getAuth(app); export const login = (email, password) => { return signInWithEmailAndPassword(auth, email, password); };
-
Creating a Login Page: Add a simple login form in your Next.js app:
import { login } from "../firebase"; const LoginPage = () => { const handleLogin = async (e) => { e.preventDefault(); const email = e.target.email.value; const password = e.target.password.value; try { await login(email, password); alert("Login successful!"); } catch (error) { alert("Login failed: " + error.message); } }; return ( <form onSubmit={handleLogin}> <input type="email" name="email" placeholder="Email" required /> <input type="password" name="password" placeholder="Password" required /> <button type="submit">Login</button> </form> ); }; export default LoginPage;
2. Real-Time Database Integration
Firebase’s Firestore database enables real-time data updates across all connected clients. This feature is ideal for dynamic applications like chat apps, collaborative tools, or dashboards.
Step-by-Step Guide to Use Firestore
-
Install Firestore: Add Firestore to your project:
npm install firebase/firestore
-
Initialize Firestore: In
firebase.js
, set up Firestore:import { getFirestore, collection, addDoc } from "firebase/firestore"; const db = getFirestore(app); export const addUser = async (userData) => { const usersCollection = collection(db, "users"); await addDoc(usersCollection, userData); };
-
Using Firestore in a Component: Create a form to add user data to Firestore:
import { addUser } from "../firebase"; const AddUser = () => { const handleSubmit = async (e) => { e.preventDefault(); const name = e.target.name.value; const email = e.target.email.value; try { await addUser({ name, email }); alert("User added successfully!"); } catch (error) { alert("Failed to add user: " + error.message); } }; return ( <form onSubmit={handleSubmit}> <input type="text" name="name" placeholder="Name" required /> <input type="email" name="email" placeholder="Email" required /> <button type="submit">Add User</button> </form> ); }; export default AddUser;
3. Cloud Functions
Firebase Cloud Functions allow you to execute server-side logic without managing servers. Functions can be triggered by events such as database changes, authentication events, or HTTP requests.
Step-by-Step Guide to Use Cloud Functions
-
Initialize Cloud Functions: Run the Firebase CLI commands to set up cloud functions:
firebase init functions
-
Write a Cloud Function: Create a simple function to send a welcome email when a new user signs up:
const functions = require("firebase-functions"); exports.sendWelcomeEmail = functions.auth.user().onCreate((user) => { const email = user.email; console.log(`Welcome email sent to ${email}`); // Add logic to send email });
-
Deploy Cloud Functions: Deploy your function to Firebase:
firebase deploy --only functions
Firebase Hosting for Next.js
Firebase Hosting is a fast and secure solution for deploying Next.js apps. It supports both static and server-side rendered content, ensuring optimal performance and user experience.
Step-by-Step Guide to Deploy with Firebase Hosting
-
Install Firebase CLI: Install Firebase’s command-line tools:
npm install -g firebase-tools
-
Initialize Firebase Hosting: Set up hosting for your project:
firebase init hosting
-
Build and Deploy:
- Build your Next.js app:
npm run build
- Deploy to Firebase:
firebase deploy
- Build your Next.js app:
Best Practices for Next.js and Firebase
-
Optimize SEO:
Utilize Next.js’s SSR and SSG to deliver pre-rendered content, ensuring better search rankings and faster initial page loads. -
Secure Firebase Keys:
Store your Firebase credentials in environment variables (.env
file) to keep them secure. -
Implement Caching:
Use Firebase’s CDN and Next.jsgetStaticProps
orgetStaticPaths
to cache frequently accessed content for faster delivery. -
Monitor Performance:
Leverage Firebase Analytics for insights into user behavior and Next.js’s performance profiling tools to optimize app speed. -
Scalable Database Design:
Structure Firestore collections and documents to accommodate future app growth.
Real-World Use Cases
1. E-commerce Platforms
- Frontend: Use Next.js’s SSG for static product pages and dynamic routes for user-specific content.
- Backend: Firestore for managing inventory and Firebase Authentication for secure user logins.
2. Content Management Systems
- Frontend: Next.js provides an intuitive admin interface with SSR for live content previews.
- Backend: Firestore stores content, and Cloud Functions handle data transformations.
3. Social Media Applications
- Frontend: Next.js delivers fast-loading user interfaces.
- Backend: Firebase Authentication manages user accounts, while Firestore handles real-time updates for posts and comments.
Prateeksha Web Design’s Expertise
At Prateeksha Web Design, we specialize in crafting serverless applications using Next.js with Firebase. Our team delivers custom, scalable, and SEO-optimized solutions tailored for small businesses. Whether you’re launching an e-commerce platform, a social app, or a content-rich website, we ensure top-notch performance and user experience.
Conclusion
Next.js and Firebase are a powerful duo for creating modern, serverless applications. By leveraging the strengths of both technologies, developers can deliver high-performance, scalable, and secure apps without the hassle of managing infrastructure. For small businesses, these tools provide a cost-effective way to build robust digital solutions.
If you’re ready to take your business to the next level with a serverless app, let Prateeksha Web Design guide you through the process. Contact us today to explore how we can transform your vision into reality.
About Prateeksha Web Design
Prateeksha Web Design offers expert services in developing serverless applications using Next.js and Firebase. Our team specializes in creating dynamic and scalable web applications that utilize the power of serverless architecture. With our extensive experience in Next.js and Firebase, we can help you build efficient and secure applications that meet your business needs. Contact us today to learn more about how we can revolutionize your app development process.
Interested in learning more? Contact us today.
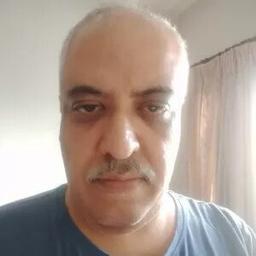