- Payload: This is where all the juicy data is stored, including user information and any permissions they have (think of it as the attractions you can access based on your ticket type).
- Signature: This is like a wax seal on your letter—ensuring that the token wasn’t tampered with during its journey.
By using JWT, your Laravel API can ensure that requests are authenticated and that users can be granted or denied access based on the information encoded within those tokens.
4. OAuth Authentication: The VIP Pass
Now, let’s step up our game with OAuth. This is more like getting a VIP pass at the amusement park, allowing you to enter exclusive attractions without waiting in line. OAuth isn’t just about logging in; it’s about delegation.
Here’s how it works:
- Users Authentication: OAuth allows third-party services to exchange credentials safely. Picture it as a bouncer who confirms your identity (authentication) but doesn’t reveal your personal details to others when you enter.
- Token Exchange: Once you’ve provided your credentials, OAuth will issue a token that represents your access level without exposing your actual credentials. This way, you can enjoy the perks of the exclusive rides while maintaining privacy.
Using OAuth with Laravel not only enhances the API’s security but also improves user experience by allowing them to utilize existing credentials from other services, like Google or Facebook, to log in swiftly.
5. Laravel Passport vs. Laravel Sanctum
In your quest for secure API development, you’ll undoubtedly stumble upon Laravel Passport and Laravel Sanctum. Both have unique roles, kind of like different types of armor:
-
Laravel Passport: This is your heavy-duty knight's armor, perfect for applications requiring robust OAuth2 authentication. It comes with all the bells and whistles necessary for complex, secure API interactions.
-
Laravel Sanctum: If your project is more about single-page applications (SPA) and simpler token-based authentication, then Sanctum is your lightweight armor—secure yet easy to manage.
Choosing between the two depends on your project’s needs, so weigh your options carefully!
6. Implementing Middleware for Enhanced Security
To further fortify your API, you’ll want to harness the power of middleware. Think of middleware as a magical barrier that catches any unwanted creatures before they reach your castle gates.
Laravel makes it easy to create custom middleware to handle various tasks, like:
- User Authentication: Ensuring that every request has a valid token.
- Rate Limiting: Preventing abuse by limiting the number of requests a user can make in a certain time frame.
- Input Validation: Making sure that incoming data is clean and follows your defined rules.
By strategically placing middleware throughout your API, you can effectively guard against common vulnerabilities.
7. Best Practices for Laravel API Security
In addition to the tools and techniques we’ve discussed, adhering to best practices will go a long way in securing your Laravel APIs:
- Use HTTPS: Always transmit your data securely using HTTPS to prevent man-in-the-middle attacks.
- Error Handling: Customize error responses to avoid leaking sensitive information. Only provide necessary details to the user while logging complete details for debugging.
- Keep Your Libraries Up-to-Date: Regularly check and update Laravel and any third-party packages to address security vulnerabilities.
Staying one step ahead of potential threats is key—like a vigilant knight preparing for battle.
8. Local Developer Scenes: Web Design in Mumbai
While embarking on this technical journey, it’s helpful to know that you’re not alone! The web development community in Mumbai is thriving, with many local agencies like Prateeksha Web Design at the forefront. These companies are equipped with talented developers who specialize in building and securing web applications. They can serve as an excellent resource for collaboration or inspiration on your projects.
9. Call to Action: Your Next Steps
So, are you ready to level up? Start implementing what you’ve learned! Here’s a simple action plan:
- Set Up a New Laravel Project: Create a new API project and install the necessary packages (like Passport or Sanctum).
- Implement JWT and OAuth: Follow documentation and tutorials to integrate authentication into your API.
- Develop with Security in Mind: Continuously test and refine your API’s security measures as you add new features.
Remember, becoming a Laravel wizard in API security doesn't happen overnight—it’s a journey filled with learning and practice. So grab your wand (or keyboard) and start coding!
By following this guide, you’re well on your way to not only securing your Laravel APIs but also becoming a part of a community that values security and excellence in software development. Happy coding! 🧙♂️✨
Here’s an expanded and refined version of your content, providing greater detail and clarity around each topic:
2. Payload: The Content of Your Ticket
In the world of authentication tokens, the payload is where the real excitement takes place. Think of it as the detailed itinerary of your amusement park adventure—the actual claims or data contained in the token that stipulates what you can access. For example, within a JSON Web Token (JWT), the payload could include information such as:
- User Identification: Unique identifiers (like user ID or username) that link the token to a specific user.
- Role Information: This could indicate what permissions the user has (e.g., admin, user, guest), dictating what areas or features they can access.
- Access List: A direct reference to the specific resources, such as the names of the rides or attractions that the user is authorized to access.
This section is crucial for the system to differentiate between users and tailor their experience according to their permissions.
3. Signature: The Validity Stamp
The signature of a JWT serves as a security measure, akin to a theme park's official stamp on your ticket. It ensures that the ticket has not been tampered with and verifies that it is indeed legitimate.
To create this signature, you take the encoded header, the encoded payload, and a secret key (a private part known only to the server) and run them through a hashing algorithm. This process results in a unique string that guarantees the integrity of the token. When a user presents their token, the server can regenerate the signature using the same secret key and compare it to the received signature. If they match, the token is considered valid; if not, access is denied.
Whenever a user logs in to your application, Laravel seamlessly generates a JWT. This token then acts as the user's identification badge for subsequent requests, ensuring that unauthorized users cannot sneak in unnoticed.
4. OAuth Authentication: The VIP Pass to Secure Access
Moving on to OAuth authentication, envision it as your exclusive VIP pass that elevates your experiences while safeguarding your credentials. OAuth is an open standard authorization protocol that allows users to grant limited access to their resources on one site, without exposing their actual password.
For example, when you opt to log in to a new web application using your Google account, OAuth functions behind the scenes, allowing you to authenticate with Google without sharing your password with the new application. This enhances both security and user experience.
In the context of Laravel, OAuth is pivotal for enabling third-party integrations with your APIs. It empowers users by allowing them to authenticate using their social media credentials—reducing the burden of yet another username and password combination that they may forget shortly thereafter. This not only streamlines the signup process but also boosts user satisfaction by using trusted authentication sources.
5. Laravel Passport vs. Laravel Sanctum: Choosing the Right Tool for Your Job
Having clarified JWT and OAuth, let’s delve deeper into two powerful Laravel authentication packages: Laravel Passport and Laravel Sanctum. Each is tailored for specific use cases, and understanding their strengths will inform your choice:
-
Laravel Passport:
- Full OAuth2 Implementation: This robust library supports the complete OAuth2 specification. It is ideal for applications requiring extensive and complex security features, such as those with multiple user types and permissions.
- Security Features: Passport includes sophisticated options like authorization codes, refresh tokens, and personal access tokens, making it suitable for applications that demand high-security standards.
-
Laravel Sanctum:
- Simplicity and Lightweight Design: Sanctum is easy to implement, making it a great option for smaller, less complicated applications.
- Best for SPAs: It is specifically designed for Single Page Applications and offers a simplified method of API token authentication, allowing users to authenticate using simple API tokens without the complexity of OAuth.
Choosing between these two frameworks is nuanced. If your application resembles a bustling metropolis with various intricate features and user roles, Passport is your best bet. Conversely, if you’re building a quaint little café (a straightforward Single Page Application), Sanctum provides all the features you need without unnecessary complexity.
6. Middleware: The Security Guards of Your Application
As we venture further into enhancing security, it’s time to discuss middleware. Think of middleware as the vigilant security guard at the entrance of your application, ensuring that only authorized users gain entry to sensitive areas.
In Laravel, middleware plays an essential role in enforcing API token authentication and ensuring users possess the appropriate permissions to access resources. Here are a few crucial types of middleware you might implement:
- Auth Middleware: This safeguards your routes by verifying that the user's authentication token is valid before granting access to specific parts of your application.
- Throttling Middleware: Just like a bouncer who monitors how many drinks a guest consumes, this middleware restricts the frequency of API requests to prevent abuse and ensure system stability.
To implement middleware in your Laravel project, you’ll create a new middleware class and register it in the Kernel.php
file. Here’s a brief snippet to illustrate how this is done:
// Create a new middleware class using artisan command
php artisan make:middleware AuthMiddleware
// In your newly created middleware class, you would define the logic:
public function handle($request, Closure $next)
{
// Check if the token is valid
if (!$this->isValidToken($request->bearerToken())) {
return response()->json(['error' => 'Unauthorized'], 401);
}
return $next($request);
}
// Finally, register your middleware in Kernel.php
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\AuthMiddleware::class,
];
With these security measures in place, your Laravel application will be fortified, allowing for safe and secure interaction with your users.
This enhanced and detailed outline should give readers a comprehensive understanding of payloads, signatures, OAuth, the differences between Passport and Sanctum, and the significance of middleware in Laravel development.
Certainly! Below is an expanded, detailed, and refined version of the content you provided, preserving the key concepts while enhancing clarity and depth.
Laravel Middleware Configuration for Enhanced API Security
In the world of Laravel, middleware serves as gatekeepers for your application, ensuring that only authorized users can access specific routes. The following configuration sets up two critical middleware components in your Laravel application:
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\Authenticate::class,
'throttle' => \App\Http\Middleware\ThrottleRequests::class,
];
With this setup, you now have established two robust guards protecting your digital domain:
-
Authentication Middleware (
auth
): This middleware is responsible for verifying user authentication. It ensures that only users who are logged in can access certain routes, preventing unauthorized access to sensitive parts of your API. -
Throttle Middleware (
throttle
): This middleware protects your application from excessive requests. By limiting the number of requests a user can make within a specific timeframe, it helps to mitigate abuse and safeguard your resources from being overwhelmed.
By implementing this structure, you create a fortress around your API, allowing only the worthy to gain entry.
7. Best Practices for Laravel API Security
As you embark on your journey to secure your Laravel APIs, it’s crucial to adopt a series of best practices. As the saying goes, "An ounce of prevention is worth a pound of cure." Here are essential practices to fortify your API security:
-
Use HTTPS: Just as a sturdy lock protects your home, employing HTTPS encrypts data in transit. It prevents man-in-the-middle attacks, ensuring that sensitive information remains confidential during transmission.
-
Validate Input: Safeguard your application against harmful data by using validation rules. This check ensures that only data meeting your defined criteria enters your system, effectively reducing the risk of SQL injection and other attacks.
-
Keep Software Updated: Stay vigilant and keep your Laravel framework and third-party libraries up to date. Regular updates patch known vulnerabilities and keep your application resilient against potential threats.
-
Implement Rate Limiting: Similar to controlling portion sizes at a party, establishing rate limits on API requests helps prevent abuse. This mechanism protects your server from overload, especially during traffic spikes, and enhances overall performance.
-
Monitor Logs Regularly: Continuous log monitoring enables you to detect and respond to malicious activities promptly. By analyzing access logs, you can identify suspicious patterns and take preventive measures before issues escalate.
Employing these strategies not only fortifies your application's defenses but also instills a culture of security awareness within your development practices.
8. Local Development Scene: Web Design in Mumbai
Before concluding, let’s explore the dynamic city of Mumbai, renowned for its rich culture and thriving digital landscape. If you’re on the lookout for website development or ecommerce website design in Mumbai, there are exceptional web design companies, such as Prateeksha Web Design, equipped to assist you in your journey.
When creating your online presence, consider the following fundamental elements:
-
User Experience (UX): Your website should be as inviting and comfortable as a warm cup of chai on a chilly evening. An intuitive, smooth design enhances user engagement and encourages repeat visits.
-
Responsive Design: In today's mobile-centric world, ensure your site delivers a consistent and visually appealing experience across all devices. The flexibility of your design will cater to users regardless of whether they are on a phone, tablet, or desktop.
-
SEO Optimization: Implement SEO best practices to enhance your website's visibility. No matter how well-designed your site is, optimizing it for search engines helps attract more visitors and boost your online presence.
Investing in a skilled web design company in Mumbai will serve as a pivotal step towards establishing a secure and visually compelling online identity.
9. Call to Action: Your Next Steps
Congratulations! 🎉 You have taken your first steps into the realm of secure Laravel APIs. Remember, consistent practice leads to growth. Dive into your development projects, explore the intricacies of JWT and OAuth, and let your curiosity drive your learning.
Should you encounter any obstacles or uncertainties along the way, don’t hesitate to seek help from professionals. Organizations like Prateeksha Web Design are ready to support you with their expertise in both development and design.
Go forth and secure your APIs! Enjoy the journey of backend development, laden with challenges and innovation. With the right strategies and tools at your disposal, you can confidently navigate this landscape. Keep learning, keep coding, and ensure your APIs are as robust and secure as your favorite spot on the couch!
This version of the content is designed to provide a thorough understanding while maintaining a friendly, informative tone. If you need further refinement or specific adjustments, feel free to ask!
Tip: Always use HTTPS for your Laravel APIs when implementing JWT and OAuth to ensure that sensitive information, like tokens and credentials, is encrypted during transmission. This protects against man-in-the-middle attacks.
Fact: JSON Web Tokens (JWT) are stateless, meaning that all the necessary information is contained within the token itself. This allows for easier scalability and reduces the server load, as there’s no need to store session data on the server.
Warning: Be cautious with token expiration settings. Setting the expiration time too long can increase the risk of token abuse if a token is compromised. Conversely, setting it too short may lead to frequent re-authentication, which can frustrate users. Aim for a balanced expiration strategy.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services in building secure Laravel APIs, focusing on JWT and OAuth authentication methods. Their expertise includes developing robust, scalable API architectures tailored to client needs. They provide comprehensive guidance on best practices for API security and integration. The team ensures seamless functionality and performance through rigorous testing and debugging. Additionally, they offer ongoing support and maintenance for sustained security and efficiency.
Interested in learning more? Contact us today.
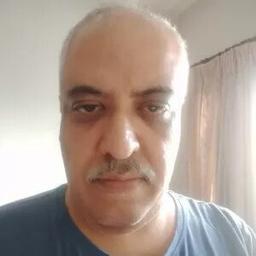