Certainly! Let's expand and refine your original content into a more detailed and engaging guide to mastering Laravel API development.
Mastering Laravel API Development: A Playful Guide to Building Secure and Efficient APIs
Welcome to the exciting and dynamic realm of Laravel API development! 🎉 If you've chosen to embark on this journey, you're in for an exhilarating ride! Think of Laravel not just as a framework but as your trusty sidekick, always ready to lend a hand while you craft robust APIs. Whether this is your first foray into building a Laravel REST API or you're simply looking to enhance the security and efficiency of your existing APIs, this guide will serve as your trusty roadmap to achieving mastery.
Key Takeaways
1. Understanding the Basics of APIs
Before we dive deeper, let’s clarify what an API actually is. API stands for Application Programming Interface, and you can think of it as the waiter in a bustling restaurant. When you place an order, the waiter takes your requests and ensures the kitchen delivers a delicious meal (in this case, data) back to you. In the world of APIs, these interactions occur via requests (like “Can I have the latest product details?”) and responses, typically structured in JSON format, which is easy for developers to read and parse.
Why Use Laravel for APIs?
Laravel shines as an API development framework due to its extensive suite of features designed to streamline the development process. Routing lets you define clean and user-friendly URLs, middleware allows you to filter incoming requests, and Eloquent ORM provides an elegant way to interact with your database. Together, these features facilitate the creation of robust, efficient, and maintainable RESTful APIs.
2. Setting Up Your Laravel Environment
Before you don your API wizard hat, ensure your development environment is ready. Here's what you need:
- PHP (version 7.3 or higher) - As the language underpinning Laravel, PHP is crucial for your application.
- Composer - This is your go-to dependency manager that will help you install Laravel and any third-party packages you'll need.
- Laravel - Always opt for the latest version to unlock the newest features and improvements.
To install Laravel quickly, you can execute the following command in your terminal:
composer create-project --prefer-dist laravel/laravel projectName
Pro Tip: If you're uncertain about which version to use, always stick with the latest one—think of it as selecting the freshest produce at the market!
3. Crafting Your First Laravel API
Congratulations! Your Laravel environment is set up! Now, it’s time to roll up your sleeves and build your very first API. Start by creating a new resource using Artisan, Laravel’s powerful command-line interface:
php artisan make:model Post -mcr
This command generates a Post model, a migration file for creating the database table, and a resource controller, which will handle your API endpoints.
4. Routing to the Rescue
Routing is essential for defining the pathways your application will respond to. In Laravel, routing is straightforward. You’ll find the routes for your web application in web.php
and the routes for your API in api.php
. Here’s how to create a route for fetching all posts:
Route::get('/posts', [PostController::class, 'index']);
This line effectively connects the HTTP GET request to the index
method in the PostController
, making it easy to retrieve all post data.
5. JSON Responses: Making Data Delicious
One of the most vital aspects of any API is how it responds to requests. In most cases, you'll return data in JSON format due to its lightweight nature and ease of use. Laravel simplifies this greatly with the response()->json()
method. Here’s an example of returning all posts in JSON format:
public function index()
{
return response()->json(Post::all());
}
This concise function fetches all posts from the database and returns them as a JSON response, which clients can easily consume.
6. Security Essentials for Your API
As you build your API, security should be at the forefront of your concerns. Here are some fundamental practices to secure your Laravel API:
- Authentication: Use Laravel's built-in authentication systems, such as Laravel Passport or Laravel Sanctum, to manage user authentication through tokens.
- Rate Limiting: Implement rate limiting using middleware to prevent abuse and ensure fair usage. Laravel provides a simple way to define rate limits in your
api.php
using thethrottle
middleware. - Input Validation: Always validate incoming requests to prevent malicious data from compromising your application. Laravel’s request validation can be done using form requests or directly in your controller methods.
public function store(Request $request)
{
$validatedData = $request->validate([
'title' => 'required|string|max:255',
'body' => 'required|string',
]);
// Create a new Post using validated data
Post::create($validatedData);
}
7. Efficient Data Handling with Eloquent ORM
Laravel’s Eloquent ORM allows for expressive database interactions. When retrieving data, consider eager loading relationships to reduce the number of queries. For example:
public function index()
{
$posts = Post::with('comments')->get();
return response()->json($posts);
}
Eager loading helps optimize performance by loading related data all at once, making your API responses faster and more efficient.
Conclusion
And there you have it! You are now armed with the foundational knowledge to start building secure and efficient APIs using Laravel. As you continue on your journey, remember that practice is vital. Experiment with advanced features like versioning your APIs, implementing different authentication methods, and using tools like Postman to test your endpoints. Happy coding, and may your Laravel APIs be both fun to build and delightful to use! 🎊
This expanded version adds more depth and detail to each section, enhancing the guide's instructional value while preserving the playful tone.
Certainly! Here's a detailed expansion and refinement of the provided content, breaking each section down for better clarity and understanding.
public function index() {
return response()->json(Post::all());
}
In this snippet, we have a simple yet powerful function, index
, that serves a dual purpose: it retrieves all posts from the database and sends them back to the frontend in a formatted JSON response. This is especially user-friendly as JSON (JavaScript Object Notation) is a lightweight data interchange format that's easy to read and write for both humans and machines. For frontend developers, this means they can easily parse the data, manipulate it, and display it within their applications without extraneous conversion processes.
6. Securing Your API
Let's talk about the importance of API security. If our API were depicted as a superhero in the digital realm, then API security would undoubtedly be its cape, providing essential protection against vulnerabilities. Without this layer of security, confidential data risks exposure to unauthorized users, thus jeopardizing both user privacy and application integrity. To bolster your API's defenses, consider leveraging two formidable tools: Laravel Passport and Laravel Sanctum.
Laravel Passport
Imagine Laravel Passport as your full-powered jetpack, capable of navigating the complex skies of OAuth2 authentication with ease. It is particularly well-suited for larger applications that demand robust API authentication mechanisms. To implement Passport in your Laravel project, start by installing the package:
composer require laravel/passport
After installation, you will need to run migrations to prepare your database:
php artisan migrate
Next, you can install Passport by executing:
php artisan passport:install
With these steps completed, you'll be armed with the ability to authenticate users via access tokens securely. Implementing API security through Passport ensures that only authorized users can access sensitive data, providing layers of trust and protection for your application.
Laravel Sanctum
Conversely, when simplicity and ease of implementation are what you need, think of Laravel Sanctum as the trusty bicycle in your security toolbox. Sanctum is designed for Single Page Applications (SPAs) and provides a straightforward approach to token-based authentication without the complexities of OAuth2. To get started with Sanctum, install it using Composer:
composer require laravel/sanctum
Once it’s installed, you need to add the Sanctum middleware to your Laravel API by modifying the api.php
route file. This middleware helps ensure that all routes you want to protect are indeed secured with token authentication.
7. Middleware: The Bodyguards of Your API
Every superhero needs a team, and that’s where Laravel middleware comes into play! Middleware acts as the gatekeepers of your API, ensuring that each request adheres to specific rules before it reaches its intended route. By creating custom middleware, you have the power to enforce various protocols, such as request throttling, which limits the number of requests a single user can make in a specified time frame, thus protecting your server from potential overload.
Creating a custom middleware is straightforward. For instance, if you want to create middleware that checks the user's age, use the command:
php artisan make:middleware CheckAge
Once generated, you can refine its functionality in the newly created file within the app/Http/Middleware
directory. By implementing middleware effectively, you ensure that your API remains secure and functions smoothly according to your designed rules.
8. Handling Errors Gracefully
Finally, even the best APIs can run into troubled waters, which is why handling errors gracefully is paramount. A well-designed API should respond to errors in a structured manner that helps users (developers and clients) understand what went wrong without exposing sensitive system information.
For instance, instead of returning a generic error message, your API can provide specific error codes and messages that offer clarity. Here's an example of how you might structure a response for a not-found error:
public function show($id) {
$post = Post::find($id);
if (!$post) {
return response()->json(['error' => 'Post not found.'], 404);
}
return response()->json($post);
}
By utilizing structured error responses like this, you improve the user experience and make your API more robust. Incorporating thoughtful error handling not only helps in debugging but also reassures clients that the API is well-maintained and user-focused.
Conclusion
Overall, by carefully implementing these features — from serving data in accessible formats to securing your API and handling errors elegantly — you can create a well-functioning, secure, and user-friendly environment for both developers and users. Embracing these best practices will ensure that your API stands as a reliable & efficient service in the software ecosystem.
Understanding Error Handling in API Development
Errors are an inevitable part of any development process. Imagine the delight of ordering a cheeseburger only to receive a salad instead—definitely not the intended outcome! In the same way, when users interact with your API, they expect seamless interactions, and encountering errors can disrupt their experience. Implementing effective error handling is crucial for maintaining user satisfaction and ensuring a smooth workflow.
Why Implement Error Handling?
Incorporating error handling into your API allows you to manage unexpected situations gracefully. Instead of leaving users confused with a blank screen or a cryptic error message, you can provide meaningful feedback that helps them understand what went wrong.
For instance, using Laravel’s built-in error handling capabilities means you can create user-friendly responses. Here’s a basic example of how to handle errors when fetching a post:
public function show($id) {
$post = Post::find($id);
if (!$post) {
return response()->json(['error' => 'Post not found.'], 404);
}
return response()->json($post);
}
In this code snippet, if the post with the specified ID doesn't exist, the user receives a clear JSON response indicating that the post was not found along with the appropriate HTTP status code (404). This allows developers and users to debug and interact with the API more effectively.
The Importance of Testing Your API
Just like a well-rounded diet includes a mix of healthy and indulgent foods, robust API development necessitates thorough testing. Laravel’s PHPUnit framework provides developers with the tools to conduct automated tests, ensuring that every endpoint behaves as expected before it goes into production.
To get started on writing tests for your API, you can create a new test file with the following command:
php artisan make:test PostTest
This command will generate a skeleton test class where you can add your test cases. For example, you can assert that your API correctly returns a post when a valid ID is provided, or that it gracefully handles requests for non-existent posts. This practice ensures that your API remains reliable and doesn’t turn into a chaotic mess of broken functionalities.
Documentation: The Key to a Successful API
Creating documentation for your API is akin to writing a recipe for a beloved dish—essential for guiding others through its use. Comprehensive documentation allows other developers, or even yourself in the future, to understand how to interact with your API seamlessly.
Tools like Swagger and Postman are invaluable for generating clear and visually appealing documentation. They provide interactive features that allow users to test API calls, making the integration process smoother and more efficient. Consistently updated documentation helps foster collaboration and enhances the API’s usability.
Best Practices for API Development
To ensure that your API stands out for its quality and reliability, consider implementing some best practices:
-
Version Your API: This is crucial for maintaining compatibility as your API evolves. For example, use URLs like
api/v1/posts
to indicate the version in use. -
Follow RESTful Naming Conventions: Resourceful naming—using nouns instead of verbs—makes your API endpoints intuitive and easy to understand.
-
Document Endpoints and Payloads: Clear documentation helps users understand what each endpoint does, what parameters are required, and the structure of the expected payloads.
-
Enhance Error Handling: Don’t just handle expected errors. Consider edge cases and craft responses that help users troubleshoot issues.
Deploying Your API
After putting in all the hard work into developing and testing your API, it’s time for the grand unveiling! Deploying your Laravel API can be done through various popular platforms like Heroku, DigitalOcean, or AWS. Each platform has its own unique features and pricing models, so choose one that best fits your project needs.
It’s important to consider ongoing maintenance after deployment. Just like a carefully prepared cake can go stale if forgotten, your API requires regular updates and monitoring to ensure it runs smoothly and securely.
Partnering with Professionals
As you embark on your API development journey, consider collaborating with specialists like Prateeksha Web Design. This web design company, based in Mumbai, focuses on ecommerce website design and backend development, including creating robust APIs. Whether you're developing a project from scratch or need to integrate your Laravel API with a captivating frontend, their expertise can guide you to success.
Conclusion
In conclusion, you've now explored the vibrant landscape of Laravel API development. From the fundamentals of crafting your API and implementing error handling to thorough testing and documentation, you’re equipped with vital tools for success.
If you encounter challenges along the way, remember the importance of maintaining a sense of humor and seeking assistance from web design professionals when needed. The ultimate takeaway? APIs can be a fun and rewarding aspect of web development, empowering you to turn your ideas into tangible applications.
So, what are you waiting for? Jump in, start building that API, and elevate your development skills to new heights. Your Laravel adventure is just getting started—let’s get coding! 💻✨
Tip
Utilize Laravel's Built-in Tools: Leverage Laravel’s built-in features such as Eloquent ORM for database interactions, Laravel Passport for API authentication, and resource controllers for organizing your API routes. This will not only speed up your development process but also help you adhere to best practices.
Fact
RESTful Architecture: Laravel APIs are inherently designed to follow RESTful principles, allowing for stateless operations and standardized responses. Understanding these principles can improve the efficiency of your API and enhance its usability for front-end developers.
Warning
Beware of Data Validation: Failing to implement thorough data validation can lead to security vulnerabilities and data consistency issues. Always use Laravel’s validation features to ensure that incoming data meets your expectations and maintain the integrity of your API.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services in mastering Laravel API development, focusing on creating secure and efficient APIs tailored to client needs. Their expertise includes best practices for authentication, data validation, and performance optimization. Clients benefit from comprehensive guidance on API design principles and implementation strategies. With a commitment to quality, Prateeksha ensures scalable solutions that drive user engagement and satisfaction. Enhance your web applications with robust APIs that streamline functionality and improve overall user experience.
Interested in learning more? Contact us today.
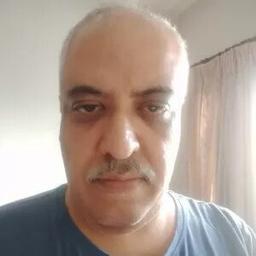