How to Build a Custom CMS System with Next.js: A Step-by-Step Guide
Table of Contents:
- 1. Introduction to Custom CMS and Next.js
- 2. Setting Up Your Development Environment
- 3. Why Choose Next.js for Building a CMS
- 4. Creating the Basic Structure of Your Next.js App
- 5. Designing the Data Architecture for Your CMS
- 6. Building the Admin Dashboard for CMS Management
- 7. Implementing Dynamic Routes for CMS Pages
- 8. Adding Content Management Features
- 9. Integrating a Database for Content Storage
- 10. Deploying Your Custom CMS with Next.js
- 11. Optimizing Performance and SEO for CMS
- 12. Conclusion
1. Introduction to Custom CMS and Next.js
In today’s fast-paced web development environment, content management systems (CMS) have become essential for companies and individuals to manage their digital content effortlessly. A CMS allows non-developers to update websites without diving into code. However, off-the-shelf solutions like WordPress or Joomla may not always meet specific needs, leading to the desire to build custom CMS systems.
Next.js, a powerful React framework, is the ideal choice for building a fast, scalable, and flexible custom CMS. With its server-side rendering (SSR) and static site generation (SSG) capabilities, Next.js provides unparalleled flexibility and performance. This step-by-step guide will walk you through building a CMS tailored to your specific needs, offering both in-depth explanations and practical code snippets.
2. Setting Up Your Development Environment
Before diving into code, you need to ensure your development environment is properly configured. Here’s a step-by-step process to set up your environment:
-
Install Node.js
To build a Next.js app, you need Node.js installed on your machine. Download it from Node.js official website. -
Install Next.js and React
Open your terminal and use the following commands:npx create-next-app@latest custom-cms cd custom-cms npm install
This creates a Next.js app with the necessary files and dependencies.
-
Set Up a Git Repository
To track your progress and collaborate with other developers, initialize a Git repository.git init git add . git commit -m "Initial commit for custom CMS"
-
VS Code and Extensions
Use Visual Studio Code as your text editor. Install extensions such as ESLint, Prettier, and Babel for code formatting and linting to ensure consistent code quality.
By setting up the development environment properly, you create a strong foundation for building your custom CMS.
3. Why Choose Next.js for Building a CMS
When building a custom CMS, selecting the right framework is crucial. Here's why Next.js stands out:
-
Performance with Server-Side Rendering (SSR)
Next.js allows you to render pages on the server, making your site faster and more responsive. This is especially useful for a CMS where page content constantly changes. -
Static Site Generation (SSG)
Next.js supports static page generation, which pre-renders pages at build time. For content that doesn’t change frequently, this results in blazing-fast loading times. -
API Routes for Flexibility
With Next.js API routes, you can create your custom backend logic without needing a separate server. You can add, edit, and manage content through API endpoints directly within your Next.js app. -
SEO Optimized
Next.js has built-in SEO features such as meta tags management, structured data, and clean URLs, ensuring that your CMS content ranks well on search engines.
Choosing Next.js equips you with a powerful toolset, blending both performance and scalability for your custom CMS.
4. Creating the Basic Structure of Your Next.js App
Once your development environment is set up, it’s time to create the basic structure of your Next.js CMS application.
-
File Structure
Next.js organizes your app with the following important folders:pages/
: Every file inside this folder is automatically routed to a URL.public/
: This folder contains static assets such as images, fonts, and other files that do not need to be processed by the server.components/
: Store your React components here for reusability.
-
Routing with Next.js
Create a homepage for your CMS by adding anindex.js
file inside thepages/
directory. Inside the file:const Home = () => { return <h1>Welcome to Your Custom CMS!</h1>; }; export default Home;
This simple homepage sets the foundation for your CMS.
-
Navigation and Layouts
To provide a smooth user experience, create a basic layout component in thecomponents/
folder:const Layout = ({ children }) => ( <div> <header>Your CMS</header> <main>{children}</main> <footer>© 2024 Your CMS</footer> </div> ); export default Layout;
-
Page Templates
CMS pages need specific templates to handle dynamic content. Start by creating page templates that can pull data and render it. For example:const BlogPost = ({ post }) => { return ( <Layout> <h1>{post.title}</h1> <p>{post.content}</p> </Layout> ); };
Having this basic structure in place gives you the building blocks to start implementing more advanced CMS features.
5. Designing the Data Architecture for Your CMS
A well-structured data architecture is critical for CMS development. You need to define how data will be created, stored, and fetched.
-
Data Models
CMS content, such as blog posts or articles, can be represented as a model. A simple blog post model might look like this:{ "title": "How to Build a CMS", "author": "John Doe", "content": "Lorem ipsum...", "createdAt": "2024-09-21" }
-
Content Types
You can define different content types (e.g., blog posts, landing pages, product descriptions) depending on the CMS you are building. -
Headless CMS vs. Traditional CMS
Headless CMS separates the content management backend from the front-end presentation. This means your CMS will deliver content as APIs rather than rendering it on the server, offering more flexibility in how and where the content is displayed. -
GraphQL for Querying Data
Many modern CMS systems use GraphQL to query data efficiently. By setting up GraphQL with Next.js, you can define precisely what content you need, reducing over-fetching of data.
By carefully designing your data architecture, you ensure your CMS remains scalable and adaptable.
6. Building the Admin Dashboard for CMS Management
The admin dashboard is where content managers will interact with your CMS. Here’s how you can build an intuitive and feature-rich admin panel:
-
Admin Login and Authentication
Implement user authentication to ensure that only authorized users can manage the content. You can use NextAuth.js for easy integration with authentication providers (e.g., Google, GitHub). -
Admin Dashboard UI
Use a popular UI library like Material UI or Ant Design to create a sleek and responsive admin interface. Example:import { Button } from "antd"; const AdminDashboard = () => { return ( <div> <h1>Admin Dashboard</h1> <Button type="primary">Create New Post</Button> </div> ); };
-
Content Management Features
Inside the dashboard, create a content management panel where users can create, edit, and delete content. This will involve building forms to handle input and API routes to store or update data in the database. -
Preview and Publishing Options
Add features such as content preview and scheduled publishing so content managers can see how content will look before going live.
The admin dashboard should be user-friendly, intuitive, and allow for seamless content management.
[7. Implementing Dynamic Routes for CMS Pages](#implementing-dynamic-routes-for-c
ms-pages)
A custom CMS often requires dynamic pages that render unique content based on user interactions. Next.js provides a robust system for managing dynamic routes.
-
File-based Routing System
To create dynamic pages in Next.js, simply add square brackets around the file name inside thepages/
folder:pages/ └── [slug].js
-
Fetching Content Dynamically
In a blog-based CMS, you can use Next.js’sgetStaticPaths
andgetStaticProps
functions to fetch content dynamically based on the URL parameters. Here’s an example for a blog post:export async function getStaticPaths() { const posts = await fetchPosts(); const paths = posts.map((post) => ({ params: { slug: post.slug }, })); return { paths, fallback: false }; } export async function getStaticProps({ params }) { const post = await fetchPostBySlug(params.slug); return { props: { post } }; }
-
Dynamic Navigation
Implement dynamic navigation in the frontend to allow users to navigate between different CMS pages. This can be done by linking the dynamic routes with the content structure in the database.
Dynamic routing is the backbone of any CMS system, ensuring that content is rendered based on user input and interactions.
8. Adding Content Management Features
To transform your CMS into a fully functional system, you need to add core content management features. Here's how:
-
CRUD Operations (Create, Read, Update, Delete)
Set up API routes to handle basic CRUD operations for your content:POST
request to create new contentGET
request to read contentPUT
request to update contentDELETE
request to delete content
-
Rich Text Editors
Integrate a rich text editor like Slate.js or Quill for content managers to create and format their posts with ease. -
Image and File Uploads
Use libraries like Cloudinary or AWS S3 to store and serve images or media files. Add a file upload feature in the admin dashboard, and ensure that the files are stored and retrieved efficiently. -
Custom Content Blocks
Let users create custom content blocks (e.g., image galleries, quote sections). These blocks can be predefined templates that users can drag and drop into their pages.
By adding these features, your CMS becomes not only flexible but also powerful, allowing content creators to manage their content with ease.
9. Integrating a Database for Content Storage
To store CMS content, you need a reliable database system. Next.js supports multiple databases, but here are some popular choices:
-
PostgreSQL
PostgreSQL is a powerful, open-source relational database that works well with large-scale CMS projects. You can use Prisma as an ORM to interact with the database. -
MongoDB
MongoDB is a NoSQL database that's great for handling unstructured content like blog posts and pages. -
Setting Up Database with Prisma
Install Prisma and configure it to connect with your database:npm install @prisma/client npx prisma init
Once Prisma is set up, you can define your data models inside the
schema.prisma
file and generate database migrations:model Post { id Int @id @default(autoincrement()) title String content String createdAt DateTime @default(now()) }
-
Performing Queries
Use Prisma’s client API to perform queries inside your Next.js app:const posts = await prisma.post.findMany();
Integrating a database with your CMS ensures that your content is stored securely and can be retrieved efficiently.
10. Deploying Your Custom CMS with Next.js
Once the CMS is ready, it’s time to deploy your project for the world to see.
-
Choosing a Hosting Platform
You can host your Next.js CMS on platforms like Vercel, Netlify, or AWS Amplify. Vercel is the best option as it is optimized for Next.js apps. -
Environment Variables
Ensure that your database credentials, API keys, and other sensitive information are stored in environment variables. Add them to the.env.local
file and reference them in your code:const databaseUrl = process.env.DATABASE_URL;
-
Deploying to Vercel
To deploy your Next.js app to Vercel, follow these steps:- Link your GitHub repository to Vercel.
- Select the project, and Vercel will automatically build and deploy it.
-
Continuous Deployment
Set up continuous deployment so every change in your GitHub repo triggers an automatic build and deploy process on Vercel.
By deploying your CMS, you make it accessible to users and can start managing content in real-time.
11. Optimizing Performance and SEO for CMS
Optimizing your CMS for both performance and SEO is essential for ensuring that your site ranks well and provides a great user experience.
-
Server-Side Rendering and Static Generation
Use SSR and SSG to optimize the loading speed of your CMS pages. For frequently updated pages, SSR is ideal, while SSG works great for static content. -
Lazy Loading and Code Splitting
Implement lazy loading for images and code splitting for large JavaScript bundles to reduce initial load times. Next.js does this automatically, but you can enhance it with libraries like React Lazy. -
Meta Tags and Structured Data
Use Next.js Head component to manage meta tags and structured data for SEO. Add the following to every page:import Head from "next/head"; const BlogPost = ({ post }) => ( <> <Head> <title>{post.title} | My CMS</title> <meta name="description" content={post.excerpt} /> </Head> <h1>{post.title}</h1> <p>{post.content}</p> </> );
-
Caching Strategies
Implement caching strategies to store frequently accessed content on the client’s side. You can use Next.js's Image Optimization to serve images in web-friendly formats like WebP.
By fine-tuning your CMS for performance and SEO, you ensure that your content reaches the maximum audience and delivers a seamless experience.
12. Conclusion
Building a custom CMS system with Next.js provides a flexible, high-performance solution for managing digital content. This step-by-step guide walked through everything from setting up your development environment to deploying a fully functional CMS with dynamic content management features. With Next.js, you leverage cutting-edge technologies like SSR, SSG, and powerful API routes, enabling you to build a scalable CMS tailored to your unique needs.
This detailed Next.js CMS system guide is designed to empower developers, CMS builders, and web designers to confidently build and deploy custom content management systems. Now that you have a solid understanding of how to build custom CMS with Next.js, you can start exploring the possibilities and extend it with features such as user roles, custom plugins, and multilingual support.
About Prateeksha Web Design
Prateeksha Web Design Company offers a step-by-step guide on building a custom CMS system using Next.js. Their service includes complete assistance from design to development, ensuring an efficient and user-friendly CMS system.
Interested in learning more? Contact us today.
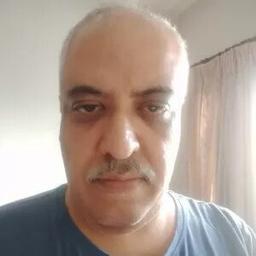