How to Automate Your Workflow with Python Scripts
In today’s fast-paced world, workflow automation has become essential. Whether you’re a small business owner, a developer, or a student, automating repetitive tasks can save you time, reduce errors, and increase productivity. This blog dives deep into Python scripting, showcasing how you can use it to simplify your tasks. Python is celebrated for its versatility and is a go-to tool for workflow automation.
At Prateeksha Web Design, we emphasize empowering businesses with tools and strategies that enhance efficiency, including Python automation. Let’s explore how Python automation tips by Program Geeks can revolutionize your daily tasks.
Why Choose Python for Workflow Automation?
Python’s simplicity, readability, and vast library support make it an ideal choice for automation. Here's why you should consider Python:
- User-Friendly: Python’s syntax is easy to learn, making it accessible even if you’re new to programming.
- Extensive Libraries: With libraries like
os
,shutil
,pandas
, andselenium
, you can automate almost any task. - Community Support: Python has an active community offering tutorials, solutions, and libraries.
- Scalability: Python scripts can handle both simple tasks and complex workflows.
By using workflow scripting with Program Geeks, you can achieve robust automation.
Setting Up Python for Automation
Before diving into scripting, ensure your system is set up properly:
-
Install Python: Download Python from the official website and install it on your system. Ensure you add Python to your system PATH during installation.
-
Set Up a Virtual Environment: A virtual environment isolates your projects, avoiding conflicts between libraries. Create one using:
python -m venv env source env/bin/activate # On Mac/<a href="/blog/how-to-install-shopifycli-on-linux">Linux</a> .\env\Scripts\activate # On Windows
-
Install Libraries: Use
pip
, Python’s package manager, to install necessary libraries:pip install pandas requests selenium
-
Choose an Editor: Use an editor like Visual Studio Code or PyCharm, which supports Python development with features like linting and debugging.
Essential Python Libraries for Automation
1. os and shutil
These libraries allow you to interact with the operating system. You can automate file and directory management tasks.
-
Example: Organize files in a directory based on their types.
<a href="/blog/difference-between-javascript-require-vs-import">import</a> os import shutil def organize_files(directory): for file in os.listdir(directory): if os.path.isfile(os.path.join(directory, file)): ext = file.split('.')[-1] folder = os.path.join(directory, ext) os.makedirs(folder, exist_ok=True) shutil.move(os.path.join(directory, file), folder) organize_files('/path/to/your/folder')
2. pandas
Pandas is essential for handling and analyzing data. It’s great for tasks like processing CSV files or generating reports.
-
Example: Automate sales report generation.
import pandas as pd def generate_report(file): data = pd.read_csv(file) summary = data.groupby('Product')['Sales'].sum() summary.to_csv('sales_summary.csv') generate_report('sales_data.csv')
3. requests
Use the requests
library to interact with APIs. Automating data retrieval from web services is a breeze with this library.
-
Example: Fetch the latest cryptocurrency prices.
import requests def fetch_crypto_prices(): response = requests.get('https://api.coingecko.com/api/v3/simple/price?ids=bitcoin,ethereum&vs_currencies=usd') print(response.json()) fetch_crypto_prices()
4. selenium
For web automation, selenium
is the go-to library. It helps with tasks like form filling, web scraping, and testing.
-
Example: Automate login to a website.
from selenium import webdriver driver = webdriver.Chrome() driver.get('https://<a href="/blog/data-validation-made-easy-with-pydantic-a-complete-guide">example</a>.com/login') username = driver.find_element_by_name('username') password = driver.find_element_by_name('password') username.send_keys('your_username') password.send_keys('your_password') driver.find_element_by_name('submit').click()
Practical Use Cases for Python Automation: Expanded with Detailed Examples
Python offers unparalleled versatility, enabling automation across diverse tasks. Below, I’ve expanded on the use cases you mentioned, providing in-depth explanations and more examples for each.
1. Email Automation
Email communication is vital for businesses, whether for customer outreach, internal updates, or promotional campaigns. Python’s smtplib
and email
libraries allow you to automate these processes effectively.
-
Basic Email Sending:
import smtplib from email.mime.text import MIMEText def send_email(subject, body, to_email): sender_email = "your_email@example.com" password = "your_password" msg = MIMEText(body) msg['Subject'] = subject msg['From'] = sender_email msg['To'] = to_email with smtplib.SMTP_SSL('smtp.gmail.com', 465) as server: server.login(sender_email, password) server.send_message(msg) send_email("Monthly <a href="/blog/laravel-9-crud-application-tutorial-with-example">Update</a>", "Here is your update...", "recipient@example.com")
-
Bulk Emailing: Use a CSV file containing recipient details and iterate through it to send emails in bulk.
import csv def send_bulk_emails(csv_file, subject, body_template): with open(csv_file, 'r') as file: reader = csv.reader(file) for name, email in reader: body = body_template.format(name=name) send_email(subject, body, email) send_bulk_emails('contacts.csv', "Special Offer", "Hello {name}, check out our latest offers!")
-
Advanced Automation: Integrate with APIs like Gmail API or SendGrid for advanced features like tracking, scheduling, or handling attachments.
2. Data Entry
Manual data entry can be tedious and error-prone. Python can automate such tasks, whether you’re handling spreadsheets, databases, or online forms.
-
Automating Spreadsheet Updates: Use Python’s
openpyxl
orpandas
to manipulate Excel files.import pandas as pd def update_sales_data(file, new_data): df = pd.read_excel(file) updated_df = pd.concat([df, pd.DataFrame(new_data)]) updated_df.to_excel(file, index=False) sales_data = [{'Product': 'Widget', 'Sales': 150}, {'Product': 'Gadget', 'Sales': 200}] update_sales_data('sales.xlsx', sales_data)
-
Filling Online Forms: Automate filling and submitting online forms using
selenium
.from selenium import webdriver from selenium.webdriver.common.keys import Keys driver = webdriver.Chrome() driver.get("https://example.com/form") name_field = driver.find_element_by_name("name") email_field = driver.find_element_by_name("email") name_field.send_keys("John Doe") email_field.send_keys("johndoe@example.com") driver.find_element_by_name("submit").click()
-
Database Entry Automation: Populate databases using Python and SQL integration with libraries like
sqlite3
.import sqlite3 def insert_data(data): conn = sqlite3.connect('data.db') cursor = conn.cursor() cursor.execute("CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT, email TEXT)") cursor.executemany("INSERT INTO users (name, email) VALUES (?, ?)", data) conn.commit() conn.close() user_data = [("Alice", "alice@example.com"), ("Bob", "bob@example.com")] insert_data(user_data)
3. Social Media Posting
Managing social media platforms manually is time-consuming. Python can help schedule posts, analyze trends, or manage interactions.
-
Scheduling Tweets: Automate Twitter posting using the
tweepy
library.import tweepy def post_tweet(content): api_key = "your_api_key" api_secret = "your_api_secret" access_token = "your_access_token" access_token_secret = "your_access_token_secret" auth = tweepy.OAuth1UserHandler(api_key, api_secret, access_token, access_token_secret) api = tweepy.API(auth) api.update_status(content) post_tweet("Hello, world! This is an automated tweet.")
-
Facebook API for Scheduled Posts: Use Facebook Graph API to automate post scheduling, requiring token-based authentication.
import requests def post_to_facebook(page_id, message, token): url = f"https://graph.facebook.com/{page_id}/feed" payload = {'message': message, 'access_token': token} response = requests.post(url, data=payload) print(response.json()) post_to_facebook('your_page_id', 'Automated <a href="/blog/infographic-meta-shares-live-video-tips-from-creators-for-facebook-and-instagram">Facebook</a> post!', 'your_access_token')
-
Analytics Reporting: Automate social media analytics by retrieving data from APIs like Twitter’s or Instagram’s.
4. SEO and Web Analytics
Python can streamline SEO efforts and provide detailed insights into your website’s performance.
-
Keyword Research Automation: Scrape search volume and keyword data using APIs like Google Ads API or tools like
BeautifulSoup
.from bs4 import BeautifulSoup import requests def get_keywords(search_query): response = requests.get(f"https://example.com/search?q={search_query}") soup = BeautifulSoup(response.text, 'html.parser') keywords = [tag.text for tag in soup.find_all('keyword_tag')] return keywords print(get_keywords("best <a href="/blog/choosing-the-best-python-gui-library-for-your-project">Python tools</a>"))
-
Backlink Analysis: Automate backlink monitoring using tools like Ahrefs API or SEMRush API.
-
Google Analytics Reporting: Use Google Analytics API to extract performance metrics.
from googleapiclient.discovery import build def get_analytics_report(view_id, start_date, end_date): <a href="/blog/what-shopware-can-do-for-you-an-overview">analytics</a> = build('analyticsreporting', 'v4', credentials=your_credentials) report = analytics.reports().batchGet(body={ 'reportRequests': [{ 'viewId': view_id, 'dateRanges': [{'startDate': start_date, 'endDate': end_date}], 'metrics': [{'expression': 'ga:sessions'}] }] }).execute() return report print(get_analytics_report('123456789', '<a href="/blog/is-gatsbyjs-dead-a-comprehensive-look-into-the-state-of-gatsby-in-2024">2024</a>-01-01', '2024-01-31'))
-
Competitor Analysis: Scrape competitor websites to analyze their content, keywords, or backlinks using
BeautifulSoup
. -
Site Health Monitoring: Check website performance (e.g., broken links, page speed) using libraries like
requests
andselenium
.
Why Python Automation is a Game-Changer
Each of these use cases demonstrates how Python can reduce manual labor, minimize errors, and save time. Whether you’re sending newsletters, updating databases, posting on social media, or analyzing your website’s SEO, Python offers tools and libraries to make the process seamless.
By applying Python automation tips by Program Geeks and leveraging workflow scripting with Program Geeks, businesses can focus on strategy while Python handles the repetitive tasks. For small businesses, this is a powerful way to scale efficiently.
If you’re ready to elevate your workflows, consider consulting with Prateeksha Web Design. We specialize in using technology to drive efficiency and growth for businesses of all sizes.
Tips for Efficient Automation
- Plan Your Workflow: Before scripting, outline the steps you want to automate.
- Break Tasks Into Modules: Create small, reusable functions.
- Test Thoroughly: Ensure your script handles exceptions and errors gracefully.
- Document Your Code: Add comments to explain your logic.
Building Authority with Workflow Automation
At Prateeksha Web Design, we specialize in delivering tailored solutions to streamline your business processes. By integrating automation into your workflows, you can focus on what matters most—growing your business. We use insights from Python tools explained by Program Geeks to enhance your efficiency.
SEO Optimization with Python Scripts
Python can also help improve your website’s SEO. Use it to:
- Automate keyword research.
- Analyze competitor strategies.
- Track performance metrics.
Final Thoughts
Mastering Python automation tips by Program Geeks can transform how you work. By automating mundane tasks, you free up time for creativity and strategic thinking. At Prateeksha Web Design, we encourage businesses to embrace such technologies. Whether you’re running a small business or managing a team, Python’s power is undeniable.
Ready to streamline your workflows? Contact Prateeksha Web Design today, and let us help you unlock the full potential of automation!
About Prateeksha Web Design
Prateeksha Web Design offers custom Python scripting services to automate workflow processes for businesses. Our team of experienced developers can create efficient scripts to streamline tasks such as data processing, file management, and system monitoring. By automating repetitive tasks with Python scripts, businesses can save time, reduce errors, and improve overall productivity. Contact us today to discuss how we can help automate your workflow with Python.
Interested in learning more? Contact us today.
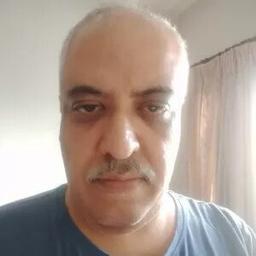