Hey there, tech adventurer! Thinking about learning Next.js but not quite sure if it's worth the hype? You're in the right place. Let's unravel the mystery of Next.js, one of the most buzzworthy frameworks in web development, and explore why developers (yes, even those just starting) can’t stop raving about it.
TL;DR: It’s fast, powerful, and makes building modern web apps a dream.
If you’ve ever dabbled with React.js, you’re already halfway there. But Next.js? It’s like React’s cool older sibling who knows all the shortcuts and has connections to make your developer life way easier. Whether you're building a portfolio, an e-commerce platform, or the next big social network, Next.js has got your back.
What is Next.js Anyway?
To truly understand Next.js, let’s break it down step by step.
React Framework: The Core of Next.js
React is a popular JavaScript library for building user interfaces. It’s loved by developers for its component-based structure, flexibility, and ability to create dynamic, interactive web applications. But React focuses solely on the "view" in the MVC (Model-View-Controller) architecture. This means developers must handle additional tasks, such as routing, API integration, and server-side rendering, by integrating external libraries and tools.
Enter Next.js, a React-based framework developed by Vercel. It takes React's power and adds pre-configured capabilities like server-side rendering (SSR), static site generation (SSG), image optimization, API routes, and more. Think of it as React with superpowers—it simplifies complex tasks and provides out-of-the-box solutions for common web development challenges.
Why Next.js is a Game-Changer
-
Pre-configured Goodness With React, you spend time setting up webpack, Babel, and routing systems. Next.js eliminates this overhead by providing:
- Built-in routing (based on the folder structure)
- Automatic code splitting for faster page loads
- Configurations that just work, so you can focus on coding instead of debugging tools.
-
Versatility Next.js is suitable for almost any type of web project:
- Static websites (blogs, portfolios)
- Dynamic applications (dashboards, social media platforms)
- E-commerce stores (with advanced product pages and dynamic filtering)
-
Production-Ready Unlike some tools that require extensive tweaking to go live, Next.js is designed for production from the get-go. Features like serverless deployment, CDN integration, and server-side rendering make it an ideal choice for professional-grade projects.
The Lego Analogy
Imagine building a massive Lego model:
- React gives you all the individual pieces, and you have the creative freedom to assemble them any way you like. But figuring out the right structure and finding missing pieces can be overwhelming.
- Next.js hands you pre-built sections, like walls and towers, along with a clear guide. You still have creative freedom, but you’re not starting from scratch or fumbling with tiny pieces.
In essence, Next.js is React, but better organized, faster, and with a developer-friendly workflow.
Key Takeaways: Why You Should Learn Next.js
1. SEO Like a Pro
Ever wondered why some websites rank higher on Google? It often boils down to SEO-friendly practices like fast loading times and well-structured content. Next.js excels in this area with two core features:
-
Server-Side Rendering (SSR): Pages are generated on the server with content already present, which search engines love. Instead of waiting for JavaScript to load content dynamically, crawlers get a fully rendered page.
-
Static Site Generation (SSG): Pre-built HTML files for each page are generated at build time, offering lightning-fast load times and better indexing by search engines.
Example in Action:
Imagine you’re launching an online store for customized T-shirts. Using Next.js with SSR, every product page can dynamically fetch and render details (like price and size options) from your backend, ensuring the freshest content for both users and search engines.
2. Blazing Fast Performance
Next.js isn’t just fast—it’s ridiculously fast. Here’s why:
- Automatic Code Splitting: Only the JavaScript required for the current page loads, keeping things lean.
- Image Optimization: The built-in
<Image>
component resizes and compresses images for faster delivery. - Pre-Fetching: Links between pages are pre-loaded, so when users click, the new page appears instantly.
Analogy: Think of performance like driving. A basic website might be like a sedan—reliable but not particularly speedy. Next.js, on the other hand, is a Tesla—sleek, efficient, and designed to get you there faster. It’s not just about speed; it’s also about how smoothly the journey goes.
Real-World Use Case:
Prateeksha Web Design recently worked on an e-commerce store built with Next.js. By optimizing image loading and using SSG for product pages, the store saw a 40% reduction in page load times, leading to higher user engagement and improved SEO rankings.
3. All About Flexibility
One of Next.js’s standout features is its ability to adapt to different types of web applications, whether it’s a simple static site, a complex dynamic app, or a hybrid of both. Here’s how it pulls this off:
Data-Fetching Capabilities
Next.js gives you the tools to decide how and when your data should be fetched and rendered. This level of control ensures your app is as fast and efficient as possible, no matter the complexity. Here’s a closer look at the key methods:
-
getStaticProps
(Static Site Generation)- What It Does: Fetches data at build time. This is ideal for pages that don’t need frequent updates, such as blogs or product catalogs.
- How It Works: The data is pre-fetched and baked into the HTML when you build the app, making these pages load lightning-fast.
- Example Use Case: You’re creating a blog. The content of each blog post is pulled from a CMS like WordPress or a markdown file during the build process, ensuring the page is ready to serve immediately.
export async function getStaticProps() { const res = await fetch('https://api.example.com/posts'); const posts = await res.json(); return { props: { posts }, }; }
-
getServerSideProps
(Server-Side Rendering)- What It Does: Fetches data at runtime on the server. This ensures users get the latest content with every request.
- How It Works: The page is rendered dynamically on the server and sent to the browser, making it great for real-time data or pages with personalized content.
- Example Use Case: Building a dashboard for users that displays their real-time data, like stock prices or social media analytics.
export async function getServerSideProps(context) { const res = await fetch(`https://api.example.com/data/${context.params.id}`); const data = await res.json(); return { props: { data }, }; }
-
API Routes for Backend Logic
- What It Does: Lets you create custom API endpoints directly within your Next.js app.
- How It Works: Add a file under the
/pages/api/
directory, and it becomes a serverless function. No need for a separate backend server. - Example Use Case: Creating a contact form that submits data to
/api/contact
and sends an email response using Node.js libraries like Nodemailer.
export default function handler(req, res) { if (req.method === 'POST') { const { name, email, message } = req.body; // Handle form submission logic res.status(200).json({ message: 'Form submitted successfully!' }); } else { res.status(405).json({ error: 'Method not allowed' }); } }
4. Built-In Routing
Routing is where Next.js shines with its file-based system. Forget about installing external libraries or writing long routing logic; just name your files, and Next.js takes care of the rest.
How It Works
- Each file in the
/pages
directory automatically becomes a route. - Subfolders in
/pages
create nested routes. - No configuration or additional dependencies are required.
Examples of File-Based Routing:
-
Basic Route
- Add
about.js
in/pages
, and it maps to/about
. - Add
contact.js
, and it maps to/contact
.
export default function About() { return <h1>About Us</h1>; }
- Add
-
Dynamic Routing
- Create dynamic routes by wrapping a file name in square brackets. For example,
[id].js
maps to/post/:id
.
export async function getServerSideProps(context) { const { id } = context.params; const res = await fetch(`https://api.example.com/posts/${id}`); const post = await res.json(); return { props: { post } }; } export default function Post({ post }) { return <h1>{post.title}</h1>; }
- Create dynamic routes by wrapping a file name in square brackets. For example,
-
Nested Routing
- Use folders for more structured routes.
Example:/pages/blog/post.js
maps to/blog/post
.
- Use folders for more structured routes.
Advantages of File-Based Routing
- Zero Setup: Start creating routes immediately without worrying about configuring a routing system.
- Dynamic Pages Made Easy: Whether it’s a blog post, user profile, or product detail, dynamic routing makes it straightforward.
- Cleaner Code: Your project structure directly mirrors the app’s routing structure, making it easier to maintain.
5. Image Optimization
Let’s be honest—managing images can feel like a chore. Resizing, compressing, and making sure they don’t slow down your website? Not exactly the fun part of development. But Next.js takes the headache out of image handling with its built-in Image component.
What is the Next.js Image Component?
The <Image>
component is a powerful tool that handles image optimization automatically. It resizes images, compresses them, and serves them in the best possible format (e.g., WebP when supported).
Here’s what makes it so awesome:
- Responsive Sizing: Automatically adjusts images to fit various screen sizes.
- Lazy Loading: Loads images only when they come into view, speeding up initial page load times.
- Placeholder Support: Adds a blurry placeholder while the image is loading, creating a smooth user experience.
How It Works
Using the <Image>
component is as simple as writing a few lines of code:
import Image from '<a href="/blog/how-to-optimize-images-in-nextjs-with-the-image-component">next/image</a>';
export default function Home() {
return (
<Image
src="/example.jpg"
alt="An example image"
width={500}
height={300}
quality={80}
/>
);
}
Why It Matters
- Improves Performance: Faster websites lead to better user experiences and higher search engine rankings.
- Saves Time: No need for third-party tools or manual image adjustments.
- SEO-Friendly: Optimized images help reduce page size, which directly benefits your SEO efforts.
👉 Humor Alert: Think of the <Image>
component as your personal photo editor. It doesn’t just resize your images—it gives them a glow-up, ready to impress your visitors without screaming, “#NoFilter.”
6. Scalability for Big Dreams
Whether you’re coding in your dorm room or leading a team for a Fortune 500 company, scalability is a big deal. No one wants their app to crash just because it got a sudden spike in traffic. Next.js has scalability baked into its DNA, making it a go-to choice for projects of all sizes.
What Makes Next.js Scalable?
-
Serverless Architecture
- What It Is: Next.js allows you to create serverless functions within the app. Each API route runs as an independent, scalable function in the cloud.
- Why It’s Cool: It’s cost-effective and can handle traffic spikes without breaking a sweat.
Example: Imagine launching a campaign that goes viral overnight. Your serverless API route can automatically scale up to handle thousands of concurrent requests, and scale back down when traffic subsides—all without any manual intervention.
-
Hybrid Rendering
- Next.js allows you to mix and match static and dynamic content. This flexibility means you can serve blazing-fast static pages while still offering dynamic features like user dashboards or real-time notifications.
-
Vercel Integration
- Vercel, the creators of Next.js, provide seamless hosting with features like automatic scaling, edge caching, and instant deployments.
- Why It’s a Game-Changer: Your Next.js app is optimized to run on Vercel’s platform, ensuring top-notch performance and reliability.
👉 Analogy: Think of Vercel as the VIP lounge for your Next.js app. It makes sure your app gets first-class treatment, no matter how crowded the airport (or the internet) is.
-
CDN Support
- Next.js uses content delivery networks (CDNs) to serve your content closer to your users, reducing latency and improving load times globally.
Why It’s Perfect for Big Projects
- E-Commerce: Handle thousands of product pages and user requests without a hitch.
- Social Media Apps: Serve dynamic, user-generated content efficiently.
- Enterprise-Level Applications: Manage high traffic and complex functionality with ease.
Real-World Example
At Prateeksha Web Design, we helped an enterprise client scale their website using Next.js. With dynamic routing, serverless API routes, and Vercel’s hosting, the client could serve millions of users seamlessly while keeping costs under control.
How Prateeksha Web Design Leverages Next.js
At Prateeksha Web Design, we don’t just build websites; we create solutions tailored to our clients’ unique needs. Next.js plays a pivotal role in helping us achieve this, offering the perfect blend of performance, scalability, and user experience. Here’s how we use Next.js to deliver exceptional results:
1. Crafting SEO-Friendly E-Commerce Stores
E-commerce success hinges on two critical factors: visibility and speed. Next.js ensures both with features like server-side rendering (SSR) and static site generation (SSG), which allow us to create stores that are:
- Easily discoverable by search engines thanks to optimized HTML content.
- Fast-loading, ensuring potential customers don’t bounce due to delays.
Case Study:
We recently worked with an e-commerce store specializing in artisanal products. Using Next.js, we revamped their site to:
- Improve load times by 40% through optimized image handling and lazy loading.
- Increase organic search traffic by 30% with SEO-friendly page structures.
- Enhance the shopping experience with dynamic product filtering and fast navigation.
2. Building Lightning-Fast Landing Pages
For clients launching new products or campaigns, we leverage Next.js to create high-performing landing pages that:
- Load in milliseconds, even on slower devices or networks.
- Deliver seamless experiences with pre-fetching and automatic code splitting.
Example:
A client needed a campaign page for their fitness product launch. Using Next.js’s static site generation, we delivered a page that:
- Could handle high traffic spikes during the campaign launch.
- Achieved a near-perfect Google PageSpeed score, impressing both users and search engines.
3. Dynamic Content Management for Small Businesses
Small businesses often need websites that can scale with them as they grow. With Next.js, we integrate dynamic features like:
- API routes for custom functionality (e.g., form handling, booking systems).
- Easy integration with CMS platforms like WordPress or Shopify for content updates.
Real-World Success:
A small business in Bryan, Ohio, approached us to modernize their online store. Here’s what we achieved using Next.js:
- Faster Load Times: Leveraging server-side rendering, their product pages now load instantly, reducing bounce rates.
- SEO Boost: Improved rankings by optimizing metadata and creating clean URL structures.
- User Delight: Customers loved the revamped experience, reflected in higher engagement and repeat visits.
4. Scalable Solutions for Growing Enterprises
For larger clients, scalability is non-negotiable. Next.js’s serverless architecture and seamless integration with Vercel allow us to:
- Deploy applications capable of handling millions of users.
- Ensure uptime and reliability during high-traffic events.
Why We Choose Next.js at Prateeksha Web Design
- Efficiency: Pre-configured tools and built-in features reduce development time.
- Flexibility: From static blogs to dynamic dashboards, Next.js adapts to every project.
- Client Success: The combination of performance and SEO optimization ensures our clients see tangible results, from improved traffic to better conversions.
Let’s Break Down Next.js Capabilities
Still not convinced? Let’s dig deeper into the technical goodies Next.js offers:
1. Server-Side Rendering (SSR)
Pages are rendered on the server and sent to the browser fully formed. This makes loading super fast and SEO-friendly.
2. Static Site Generation (SSG)
Pre-render your pages at build time for even faster performance. Perfect for blogs, portfolios, or content-heavy websites.
👉 Real-Life Use Case: Building a blog about AI-powered tools? SSG ensures your posts are lightning fast and ready to dominate Google rankings.
3. API Routes
Need backend functionality without setting up a full-blown server? Use Next.js API routes.
👉 Example: Create a /api/contact
route for handling form submissions without relying on third-party tools.
4. Automatic Code Splitting
Next.js smartly splits your code, loading only what’s needed for a specific page. This reduces initial load times.
5. Dynamic Routing
For apps with thousands of pages (e.g., product catalogs), Next.js dynamically generates routes, keeping your code clean and manageable.
👉 Example: An e-commerce site selling bikes can dynamically create pages like /bikes/mountain
, /bikes/road
, etc.
Getting Started with Next.js
Step 1: Install Next.js
If you’ve got Node.js installed, you’re just one command away:
npx create-next-app@latest my-next-app
Step 2: Start Building
Explore the /pages
directory and create your first route.
Step 3: Learn by Doing
The best way to learn Next.js is by building projects. Start small—a blog, portfolio, or to-do app.
Why 20-Year-Old Developers Love Next.js
- It’s beginner-friendly but has advanced features for when you’re ready to level up.
- Tons of tutorials, a vibrant community, and excellent documentation.
- Instant gratification: The live reload feature shows changes instantly, keeping you motivated.
Ready to Dive In?
Learning Next.js is a game-changer, especially if you want to stay ahead in the fast-paced world of web development. Whether you’re a budding coder or a seasoned pro, its capabilities will save you time, impress your clients, and make you fall in love with coding all over again.
At Prateeksha Web Design, we’re here to guide you. If you’re curious about how Next.js can transform your projects, let’s chat!
Call to Action:
Start your Next.js journey today. Build something small, experiment, and see the magic unfold. And remember, when in doubt, the Next.js documentation is your best friend (seriously, it’s amazing).
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services focused on Next.js, highlighting its advantages for developers. We provide in-depth tutorials and resources that showcase Next.js's powerful features like server-side rendering, static site generation, and optimized performance. Our team creates engaging content that emphasizes scalability and SEO benefits, ensuring developers understand how to leverage Next.js effectively. We also offer personalized training sessions to enhance skill sets in modern web development. Join us to master Next.js and elevate your web design capabilities.
Interested in learning more? Contact us today.
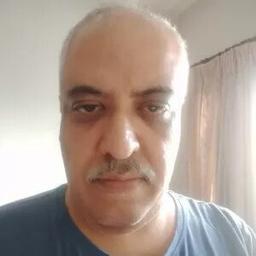