Using React Query in Next.js for Data Fetching Made Simple
React Query has revolutionized data fetching and state management in React-based projects. When combined with Next.js, it becomes a powerful tool for building scalable, fast, and user-friendly applications. This blog will take you through a detailed guide on using React Query in Next.js, making complex concepts easy to understand, even if you’re new to these tools. Let's dive in, explore how React Query works, and how to leverage its capabilities to create dynamic applications effortlessly.
Why Choose React Query in Next.js for Data Fetching?
Modern applications require efficient data fetching strategies to keep users engaged with fast and responsive interfaces. React Query simplifies this by providing an out-of-the-box solution for server-state management. Unlike traditional approaches (like useEffect
for fetching), React Query:
- Automates data caching, background updates, and request deduplication.
- Enhances performance with automatic data refetching and stale-while-revalidate strategies.
- Provides better error handling and state management tools.
By integrating React Query with Next.js, you gain access to features like server-side rendering (SSR), static site generation (SSG), and seamless routing, which are crucial for modern web apps.
Getting Started with React Query in Next.js
Here’s how you can integrate React Query into a Next.js application:
Step 1: Install Dependencies
To start, you need to install the required packages:
npm install react-query @tanstack/react-query react-query-devtools
These include the core React Query library and the devtools for debugging.
Step 2: Set Up a Query Client
React Query uses a QueryClient to manage cache and queries. Create a queryClient
instance and wrap your application with the QueryClientProvider.
// pages/_app.js
import { QueryClient, QueryClientProvider } from "@tanstack/react-query";
const queryClient = new QueryClient();
function MyApp({ Component, pageProps }) {
return (
<QueryClientProvider client={queryClient}>
<Component {...pageProps} />
</QueryClientProvider>
);
}
export default MyApp;
This setup ensures that all components within your app can use React Query's hooks.
Fetching Data with React Query
React Query provides hooks like useQuery
and useMutation
for fetching and modifying data.
Using useQuery
for Data Fetching
The useQuery
hook is the heart of React Query. It simplifies data fetching while handling caching, retries, and background updates.
Here’s an example of fetching data from an API:
import { useQuery } from "@tanstack/react-query";
const fetchPosts = async () => {
const res = await fetch("https://jsonplaceholder.typicode.com/posts");
return res.json();
};
const Posts = () => {
const { data, error, isLoading } = useQuery(["posts"], fetchPosts);
if (isLoading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<ul>
{data.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
);
};
export default Posts;
In this example:
useQuery(['posts'], fetchPosts)
takes a unique query key and a fetcher function.- React Query caches the data, so subsequent requests for
['posts']
are fetched from cache unless stale.
Advanced Data Fetching Strategies with React Query
React Query is more than just fetching. It helps you manage pagination, infinite scrolling, and dependent queries.
Pagination
Fetching paginated data can be challenging without proper tools. React Query handles pagination seamlessly.
const fetchPaginatedPosts = async ({ pageParam = 1 }) => {
const res = await fetch(`https://api.example.com/posts?page=${pageParam}`);
return res.json();
};
const PaginatedPosts = () => {
const { data, fetchNextPage, hasNextPage, isFetchingNextPage } =
useInfiniteQuery(["paginatedPosts"], fetchPaginatedPosts, {
getNextPageParam: (lastPage, allPages) => lastPage.nextPage ?? false,
});
return (
<div>
{data.pages.map((page, i) => (
<React.Fragment key={i}>
{page.results.map((post) => (
<p key={post.id}>{post.title}</p>
))}
</React.Fragment>
))}
<button
onClick={() => fetchNextPage()}
disabled={!hasNextPage || isFetchingNextPage}
>
{isFetchingNextPage ? "Loading more..." : "Load More"}
</button>
</div>
);
};
Dependent Queries
Use dependent queries when data from one query determines another's execution.
const PostDetail = ({ postId }) => {
const { data: post } = useQuery(["post", postId], () =>
fetch(`https://api.example.com/posts/${postId}`).then((res) => res.json())
);
const { data: comments } = useQuery(
["comments", postId],
() =>
fetch(`https://api.example.com/posts/${postId}/comments`).then((res) =>
res.json()
),
{ enabled: !!postId }
);
if (!post) return null;
return (
<div>
<h1>{post.title}</h1>
<p>{post.body}</p>
<ul>
{comments.map((comment) => (
<li key={comment.id}>{comment.body}</li>
))}
</ul>
</div>
);
};
SEO and Server-Side Rendering with React Query and Next.js
React Query supports server-side rendering (SSR) out of the box, which is crucial for improving SEO and performance in Next.js applications.
Implementing SSR with React Query
In Next.js, you can prefetch queries during server-side rendering.
import { dehydrate, QueryClient, useQuery } from "@tanstack/react-query";
const fetchPosts = async () => {
const res = await fetch("https://api.example.com/posts");
return res.json();
};
export async function getServerSideProps() {
const queryClient = new QueryClient();
await queryClient.prefetchQuery(["posts"], fetchPosts);
return {
props: {
dehydratedState: dehydrate(queryClient),
},
};
}
const PostsPage = () => {
const { data } = useQuery(["posts"], fetchPosts);
return (
<div>
{data.map((post) => (
<p key={post.id}>{post.title}</p>
))}
</div>
);
};
export default PostsPage;
Conclusion
Integrating React Query in Next.js transforms how you handle data fetching. By automating complex tasks, it allows developers to focus on creating engaging user experiences. With its caching, server-side rendering capabilities, and advanced querying features, it’s a must-have tool for modern web development.
If you’re a programming geek or a small business owner, React Query can simplify your development process while improving your app's performance. Partner with experts like Prateeksha Web Design to unlock the full potential of these technologies.
For program geeks, aspiring developers, and businesses, adopting React Query is the first step toward creating future-ready applications. Stay ahead of the curve with Prateeksha Web Design!
About Prateeksha Web Design
Prateeksha Web Design offers expert services in using React Query in Next.js for simplified data fetching. Our team utilizes React Query to efficiently manage data fetching, caching, and updating in Next.js applications. We ensure seamless integration of React Query to enhance the performance and user experience of your website. With our expertise, we empower clients to easily fetch and manage data while optimizing speed and reliability. Trust Prateeksha Web Design for a streamlined data fetching process using React Query in Next.js.
Interested in learning more? Contact us today.
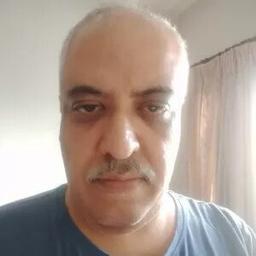