Simplified Data Fetching in React Query and Next.js for Better Performance
Welcome to the world of React Query and Next.js! If you’re someone who’s looking to level up your web development game, specifically when it comes to fetching data in a performant way, then you’ve come to the right place. I’m here to break down some key concepts, show you how React Query can supercharge your data fetching in Next.js, and help you understand how all of this translates to better performance for your app.
Whether you’re building your first React app or you’ve already dipped your toes into the world of Next.js, understanding how to fetch data efficiently is crucial. Trust me—when you start optimizing your app’s data flow, you'll notice a big difference in how fast and smooth it runs. So let’s dive in!
Table of Contents:
- What is React Query?
- Why React Query is a Game-Changer for Data Fetching
- How React Query Works with Next.js
- Improving Next.js Performance with React Query
- The Magic of React Query's Caching
- Handling Errors and Loading States Like a Pro
- React Query Best Practices for Performance Optimization
- Prateeksha Web Design’s Expertise: Building Fast and Efficient Web Apps
- Conclusion: Your Next Steps
What is React Query?
Let’s dive into the details of React Query and see why it’s such a crucial library for modern React applications, especially when combined with Next.js.
At its core, React Query is a library for managing data fetching, caching, synchronization, and background data updates in React applications. Sounds like a lot, right? Let me break it down for you, and we’ll see how it helps developers tackle some of the trickiest parts of building dynamic applications.
The Problem with Data Fetching in React
When building modern web apps, you're often working with remote data—whether it's coming from a REST API, a GraphQL endpoint, or any other external source. Managing that data properly can be a bit of a headache. Here’s a simple rundown of the common challenges:
- Fetching data asynchronously: React components need to wait for data to load from external sources, and this asynchronous process can make it tricky to manage loading states, error states, and rendering the UI efficiently.
- Caching data: Often, you’ll fetch the same data multiple times—perhaps on different pages or after a user action. Without proper caching, this can lead to unnecessary network requests, slow performance, and poor user experience.
- Error and Loading States: Manually handling loading and error states for every API call can clutter your code and lead to inconsistent UX.
- Background updates: Data may change after it's been fetched, but how do you make sure your app reflects the most up-to-date data without overwhelming your users with constant re-renders?
Enter React Query
React Query solves these challenges by abstracting the data-fetching logic into an easy-to-use and declarative API. Essentially, it takes care of all the heavy lifting, allowing you to focus on what really matters—building great user experiences and optimizing performance.
Key Features of React Query:
- Data Fetching: React Query allows you to fetch data from external sources with just a few lines of code. You tell it what data you need, and it handles fetching, caching, and managing the lifecycle of the data.
- Caching: Once data is fetched, React Query automatically caches it. If you try to fetch the same data again, it doesn’t go back to the server. Instead, it uses the cached data, which improves performance by reducing the number of network requests.
- Synchronization: React Query helps sync data between the server and the client. This is especially useful for when the data on the server changes and you need to update the client-side state.
- Background Data Updates: With React Query, you can fetch data in the background, ensuring that the user always sees the most up-to-date information without interrupting their experience. This feature is great for things like news feeds, stock tickers, or live data dashboards.
- Automatic Refetching: You can configure React Query to automatically refetch data in certain scenarios (e.g., when the window gains focus or when the user reconnects to the internet).
How Does React Query Work?
React Query operates through hooks that you use inside your React components. These hooks abstract away most of the complicated parts of handling remote data. Let’s take a look at a simple example of how React Query works:
import { useQuery } from 'react-query';
function Posts() {
const { data, error, isLoading } = useQuery('posts', fetchPosts);
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return (
<div>
<h1>Posts</h1>
<ul>
{data.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
In this example:
useQuery
is a React Query hook that automatically fetches the data (fetchPosts
is a function that returns the data from an API).- Loading state: If the data is still being fetched, it will display a "Loading..." message.
- Error state: If there’s an error while fetching the data, it will show an error message.
- Data rendering: Once the data is loaded, it will be displayed in a list.
React Query automatically handles all of the following:
- Fetching the data.
- Caching the data once it’s fetched.
- Returning the appropriate loading and error states.
- Updating the component once the data is ready.
Why Is React Query Perfect for Next.js?
Next.js is a React framework that’s optimized for server-side rendering (SSR), static site generation (SSG), and client-side rendering (CSR). When building a Next.js app, performance is key, and React Query helps you leverage Next.js’s powerful features to get the best of both worlds.
Here’s how React Query works seamlessly with Next.js:
-
Server-Side Rendering (SSR) with React Query: With SSR in Next.js, you can fetch the data for a page before rendering it on the server, and then send the fully populated page to the client. React Query fits in perfectly with this by allowing you to fetch data during the SSR process. This means the user gets a fully-rendered page with the data already loaded, which improves Time-to-First-Byte (TTFB) and overall page load times. Here’s an example of SSR with React Query in Next.js:
import { useQuery } from 'react-query'; import { fetchPosts } from '../api'; export async function getServerSideProps() { const posts = await fetchPosts(); return { props: { posts } }; } function PostsPage({ posts }) { const { data } = useQuery('posts', fetchPosts, { initialData: posts }); return ( <div> <h1>Posts</h1> <ul> {data.map(post => ( <li key={post.id}>{post.title}</li> ))} </ul> </div> ); } export default PostsPage;
-
Static Site Generation (SSG) with React Query: For static pages, React Query can fetch data during the build process, allowing you to pre-render pages with all their data included. This is great for blogs, e-commerce stores, and any site with content that doesn’t change too frequently. Using React Query with SSG helps you deliver fast-loading pages without relying on client-side fetching.
-
Client-Side Rendering (CSR) with React Query: For pages that need to load data dynamically or don’t need to be pre-rendered, React Query works wonders with CSR in Next.js. It automatically handles fetching data after the page loads, updating the UI as soon as the data is available. Plus, React Query’s caching ensures that subsequent page visits or interactions don’t trigger unnecessary network requests.
The Dynamic Duo: React Query + Next.js = Better Performance
When you combine React Query with Next.js, you’re unlocking a powerful duo that can drastically improve the performance of your application. Here’s why:
-
Fast Initial Loads: Thanks to SSR and SSG in Next.js, your pages can be rendered with all data ready to go. React Query ensures that the data is fetched efficiently, meaning your app is ready to go as soon as the user lands on the page.
-
Efficient Data Handling: React Query manages all your data-fetching logic, caching, and background updates, allowing you to focus on building great UI and UX. You don’t need to worry about re-fetching data every time a user visits your page or clicks around—it’s cached and ready when needed.
-
Automatic Updates: React Query can automatically update data in the background, ensuring that your app stays up-to-date without interrupting the user’s experience. This is perfect for real-time apps or apps where data changes frequently.
-
Reduced Server Load: Because React Query caches your data, you won’t overwhelm your API or server with unnecessary requests. This is especially helpful in large-scale applications where performance is key.
In short, React Query helps you fetch data in a way that is optimized for speed, efficiency, and scalability—all of which are crucial for Next.js apps.
Why React Query is a Game-Changer for Data Fetching
Alright, let’s get to the real talk—why should you care about React Query? Well, let’s put it this way: data fetching in modern web apps can get messy real quick. You have to deal with loading states, error handling, re-fetching data, and keeping everything in sync. Without something like React Query, you’ll likely end up managing all of this manually, which is both time-consuming and prone to errors.
React Query swoops in to save the day by automating many of these processes and offering a declarative API for your data needs. What does that mean? Well, instead of manually writing logic for fetching data every time a component needs it, you can simply declare what data your component needs, and React Query handles the rest. Here’s a quick rundown of why it’s such a game-changer:
-
Automatic Caching: React Query caches your data for you. If you’ve already fetched some data once, it won’t re-fetch it unless necessary. This reduces unnecessary network calls, which ultimately boosts performance.
-
Automatic Background Updates: React Query allows you to fetch fresh data in the background, so your users always get the latest version without interrupting their experience.
-
Built-in Pagination and Infinite Scrolling: Whether you’re dealing with a small dataset or infinite data, React Query makes handling pagination a breeze.
-
Error and Loading States: Gone are the days of manually managing loading and error states. React Query does it for you, making your components cleaner and easier to maintain.
Now, when you pair React Query with Next.js, you're not only optimizing your frontend but also leveraging Next.js’s strengths, like server-side rendering and static site generation.
How React Query Works with Next.js
Next.js is all about building optimized React applications with features like server-side rendering (SSR), static site generation (SSG), and client-side rendering (CSR). These features are designed to help your application load faster, rank better on search engines, and provide an overall smoother user experience.
But how does React Query fit into this? Let’s break it down:
-
Server-Side Rendering (SSR) with React Query: One of the main benefits of Next.js is SSR, where data is fetched on the server before the page is rendered. React Query can be used to fetch data during SSR, allowing you to send a fully hydrated page to the client, improving the time-to-first-byte (TTFB) and making your app feel faster.
-
Static Site Generation (SSG) with React Query: With Next.js, you can generate static pages at build time. Using React Query, you can fetch the data during the build process, allowing you to pre-render pages with all their data ready to go. This is especially useful for blogs, e-commerce stores, or any content-heavy site.
-
Client-Side Rendering (CSR) with React Query: React Query excels at client-side rendering, where the data is fetched after the page loads. This is particularly useful for pages that don’t need to be pre-rendered, such as dashboards or user-specific pages.
In essence, React Query seamlessly integrates with all the rendering strategies Next.js offers, enhancing the performance and user experience. The magic happens when you combine React Query’s powerful data-fetching capabilities with Next.js’s robust rendering features.
Improving Next.js Performance with React Query
Now let’s talk about performance. As a developer, improving performance should always be one of your top priorities. With Next.js and React Query, performance is baked right into the way both tools work, but there are still some strategies you can employ to make things even faster.
1. Optimizing API Requests
React Query allows you to control how often and when data is fetched. For instance, you can specify when to fetch new data or when to skip fetching altogether. Here’s a simple example:
const { data, error, isLoading } = useQuery('posts', fetchPosts, {
staleTime: 60000, // data will be considered fresh for 1 minute
cacheTime: 300000, // data will stay in the cache for 5 minutes
});
By adjusting the staleTime
and cacheTime
options, you can prevent unnecessary requests, and React Query will ensure that you're not wasting time re-fetching data that hasn’t changed.
2. Reducing Render Times with Suspense
React Query has built-in support for React’s Suspense feature, which helps delay the rendering of a component until its data is ready. This can drastically reduce rendering times and improve the perceived performance of your app.
const { data } = useQuery('posts', fetchPosts);
return (
<Suspense fallback={<div>Loading...</div>}>
<PostList data={data} />
</Suspense>
);
With Suspense, you can ensure your app only renders once the data is available, improving both perceived and actual performance.
3. Parallel and Dependent Queries
React Query makes it easy to manage multiple data-fetching operations in parallel or in sequence, depending on your needs. For example, if you need to fetch user data and their posts simultaneously, you can use useQueries
:
const [userData, postsData] = useQueries([
{ queryKey: 'user', queryFn: fetchUser },
{ queryKey: 'posts', queryFn: fetchPosts },
]);
By fetching multiple pieces of data in parallel, you reduce the total wait time and make your app feel faster.
The Magic of React Query's Caching
One of the most powerful features of React Query is its caching system. Let me paint you a picture. Imagine you’re running a coffee shop. Every time a customer asks for a coffee, you go to the back and make a new one. But eventually, you realize, most of your customers just want a plain black coffee. Instead of making it fresh every time, you decide to have a pot of coffee on hand, and you only brew a new one when the pot runs out. Boom—efficiency!
React Query does something similar. It caches your data, so when a component needs data again, it doesn’t make another API request. Instead, it just grabs the cached data. This reduces network calls, saves time, and makes your app faster.
Handling Errors and Loading States Like a Pro
Handling loading states and errors is an essential part of user experience. You don’t want your users staring at a blank page while waiting for data to load (that’s a recipe for frustration). React Query makes this process easier with built-in hooks for managing loading and error states.
Here’s an example:
const { data, error, isLoading } = useQuery('posts', fetchPosts);
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error fetching data</div>;
return <PostList data={data} />;
React Query automatically manages these states for you, so you can focus on what really matters—delivering a smooth user experience.
React Query Best Practices for Performance Optimization
Now that you’re familiar with the basics, let’s talk about some best practices to maximize performance:
-
Use Query Keys Wisely: Query keys in React Query help identify and cache your data. Use descriptive and unique keys to prevent collisions and optimize your queries.
-
Limit Background Refetching: React Query allows you to refetch data in the background. While this is super useful for keeping data fresh, too much background refetching can hurt performance. Use the
refetchOnWindowFocus
andrefetchOnReconnect
options wisely. -
Paginate Large Datasets: If your app deals with large datasets, make use of React Query’s built-in pagination methods, like
useInfiniteQuery
. This will allow you to load data in chunks and improve performance. -
Pre-Fetch Data for Faster Interactions: Use the
prefetchQuery
method to load data before it’s needed. This can make interactions feel snappy, as the data is ready when the user needs it.
Prateeksha Web Design’s Expertise: Building Fast and Efficient Web Apps
At Prateeksha Web Design, we understand the importance of building web apps that are both fast and user-friendly. We know that the key to great performance lies in optimizing how we fetch and manage data. That's why we use React Query and Next.js to help our clients build websites that not only look great but also perform at their best.
From caching strategies to error handling and background updates, we ensure that every app we develop runs as smoothly as possible. Whether you're building an e-commerce store, a content-driven website, or an interactive web app, we’ve got the expertise to make it fast, reliable, and efficient.
Conclusion: Your Next Steps
Congrats! You’ve now got the basics of React Query and Next.js down, and you're ready to start building lightning-fast, data-driven applications. The world of optimized data fetching is yours to explore, and with React Query and Next.js, you’re well on your way to creating high-performance web apps.
So, whether you're a seasoned developer or just starting, dive into React Query and Next.js, experiment with caching, background fetching, and data management techniques, and watch your app’s performance soar. Need help building your next awesome web app? At Prateeksha Web Design, we’re here to help you take your website to the next level.
About Prateeksha Web Design
Prateeksha Web Design specializes in leveraging React Query and Next.js to streamline data fetching processes. Their services enhance application performance by optimizing server state management and reducing loading times. With a focus on user experience, they offer tailored solutions that simplify API interactions. The team ensures seamless integration, enabling scalable and efficient web applications. Stay ahead in the digital landscape with efficient data handling and dynamic rendering.
Interested in learning more? Contact us today.
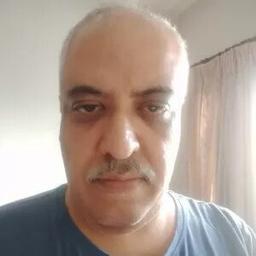