Welcome to the world of Next.js! If you're a coding enthusiast, a budding developer, or simply someone curious about building blazing-fast websites, you're in the right place. Today, we’ll dive deep into one of the most exciting features of Next.js — the app directory. Don’t worry, we’ll keep things light, fun, and easy to follow, so you’re not drowning in technical jargon. Let’s get started!
🚪 What Is the Next.js App Directory?
The Next.js app directory, introduced in Next.js 13, is like upgrading from a simple toolkit to a high-tech workshop. It's the centerpiece for structuring your Next.js application, designed to simplify the development process while leveraging modern advancements in React, particularly Server Components and the React Server Components architecture.
Breaking It Down:
-
What Does It Do?
- The app directory organizes your project files in a way that reflects how your application is structured. Think of it as a blueprint for your website where every folder and file has a purpose, representing a specific route or functionality.
-
Why Is It Important?
- It introduces React Server Components, which let you combine the efficiency of server-side rendering with the flexibility of React components. This means your app can fetch data, render pages, and deliver optimized user experiences more efficiently.
-
How Does It Work?
- Each folder in the app directory can represent a route or a logical group of functionality. For example:
app/ about/ page.js // Accessible at /about blog/ [slug]/ page.js // Dynamic route for /blog/some-post layout.js // Shared layout for multiple routes
- Inside these folders, files like
page.js
define what content is rendered when the route is accessed.
- Each folder in the app directory can represent a route or a logical group of functionality. For example:
An Analogy:
Imagine walking into a perfectly organized library. The app directory is that library:
- Sections: Each folder is a section of the library, such as Fiction or Science.
- Bookshelves: Inside each section (folder), you find specific books (files) related to that topic.
- Labels: Everything is clearly labeled (logical structure), so you can find what you need without getting lost.
🗂️ Why Does the App Directory Matter?
Before Next.js 13, developers used the pages directory to define routes and organize components. While this worked well for smaller projects, it had limitations when scaling up to larger, more complex applications.
The app directory was introduced to solve these problems, making it easier to build scalable and maintainable projects. Here's why it matters:
1. Better Organization
The app directory promotes logical file structures. Each route is its own folder, and files like layout.js
or page.js
clearly indicate their purpose. This modular approach reduces clutter and makes your project easier to navigate.
Example:
Instead of having all routes jumbled together in one folder (pages/
), you can structure your app like this:
app/
dashboard/
layout.js
page.js
settings/
page.js
Here, each feature (like Dashboard or Settings) is neatly separated.
2. Server Components
The app directory brings React Server Components into the spotlight. With Server Components:
- You can fetch data on the server, reducing the amount of JavaScript sent to the client.
- This improves performance and keeps the client-side app lightweight.
Why This Matters: Previously, you’d need to use APIs or data-fetching libraries to pass data to your React components, often duplicating work on the server and client. Now, you can handle most of this logic server-side, seamlessly.
3. Dynamic and Static Routing
Routing becomes more intuitive with the app directory. Dynamic and static routes can be created effortlessly by naming folders and files appropriately.
Dynamic Routing Example:
To create a route for individual blog posts, like /blog/123
, simply use:
app/blog/[id]/page.js
This file automatically handles the dynamic parameter id
.
Static Routing Example:
For a simple About page (/about
), you create:
app/about/page.js
No configuration needed!
4. Improved Scalability
The app directory shines when working on large-scale applications. By separating logic into smaller, manageable pieces, it avoids the chaos of having everything bundled into one massive folder (as was common with the pages directory).
- Shared layouts, like headers or sidebars, can be defined once and reused across multiple pages.
- API routes can be placed alongside their corresponding pages for better context and maintainability.
Example Structure for a Scalable App:
app/
layout.js // Shared layout
dashboard/
page.js
analytics/
page.js // Nested route for /dashboard/analytics
api/
products/
route.js // API endpoint for /api/products
🔑 Key Features of the Next.js App Directory
The Next.js app directory is packed with powerful features that make building modern web applications a breeze. Let’s break them down step by step to see why this new approach is a game-changer.
1. File-Based Routing
Gone are the days of manually configuring routes in a separate file. With the app directory, you simply create folders and files, and Next.js automatically handles the routing for you. It’s as straightforward as naming a folder and dropping in a file.
How It Works:
- Each folder represents a route.
- Files like
page.js
define the content for that route. - Dynamic routes can be created by using square brackets
[ ]
.
Examples:
-
A static route:
app/about/page.js
corresponds toyoursite.com/about
. -
A dynamic route:
app/products/[id]/page.js
dynamically generates pages likeyoursite.com/products/123
oryoursite.com/products/456
.
💡 Analogy: Imagine running a pizza shop where every room serves a specific dish. Each room has a signboard showing what’s available (e.g., Pizza, Pasta, Drinks). File-based routing is like these signboards—clear, organized, and easy to follow.
2. Server Components
One of the standout features of the app directory is Server Components. These components are rendered on the server by default, which brings several advantages for performance and development simplicity.
Why Server Components Are Awesome:
- Faster Initial Page Loads: Since the server handles rendering, the browser gets only what it needs, reducing the load on the client.
- Reduced JavaScript Overhead: Less JavaScript is sent to the browser, improving performance.
- Simplified Data Fetching: You can fetch data directly on the server without extra configuration or client-side state management libraries.
Example Use Case:
Let’s say you’re building a product page. With Server Components, you can fetch the product details directly from the server and render them efficiently.
// app/products/[id]/page.js
import { fetchProductDetails } from '../../lib/api';
export default async function ProductPage({ params }) {
const product = await fetchProductDetails(params.id);
return (
<div>
<h1>{product.name}</h1>
<p>{product.description}</p>
</div>
);
}
How It Works:
- The
fetchProductDetails
function retrieves data from an API or database on the server. - The component renders the data before sending the fully formed HTML to the client.
- The result? A fast-loading, SEO-friendly product page.
3. Layouts and Nested Routes
One of the coolest features of the Next.js app directory is the ability to use layouts. A layout acts like a reusable wrapper for your pages, ensuring a consistent design across multiple routes without duplicating code.
How Layouts Work:
- Layouts wrap pages and share common UI components such as headers, footers, and sidebars.
- Nested layouts allow you to create different designs for specific sections of your application.
File Structure Example:
Here’s a simple structure with a shared layout:
app/
layout.js // Shared layout
about/
page.js // Content for /about
contact/
page.js // Content for /contact
layout.js
wraps bothabout/page.js
andcontact/page.js
.- For example, your
layout.js
might include a header and a footer:
export default function Layout({ children }) {
return (
<div>
<header>My Site Header</header>
<main>{children}</main>
<footer>My Site Footer</footer>
</div>
);
}
When you visit /about
or /contact
, both pages will have the same header and footer, but their unique content is displayed in the <main>
tag.
💡 Pro Tip: Think of layouts as parent containers that save you from the pain of copy-pasting headers or footers into every page. It’s efficiency with a sprinkle of elegance.
4. API Routes Inside App Directory
In Next.js, you can define API routes right inside the app directory. This co-location of front-end and back-end logic makes your codebase much easier to manage, especially for projects where the two are tightly coupled.
How to Define API Routes:
- Create an
api
folder inside the app directory. - Add files named
route.js
to define endpoints.
Example:
To create an endpoint for fetching product data, you can set up the following file structure:
app/
api/
products/
route.js
Here’s what route.js
might look like:
export async function GET(request) {
const products = [
{ id: 1, name: 'Laptop', price: 1000 },
{ id: 2, name: 'Phone', price: 500 },
];
return new Response(JSON.stringify(products), { status: 200 });
}
- Access this endpoint at
/api/products
. - This setup allows you to keep your API logic close to the relevant front-end components.
💡 Why It’s Great: Instead of juggling separate back-end repositories or folders, everything stays together. It’s like keeping your utensils in the same drawer as your stove for easy access while cooking.
5. Client Components
While Server Components are the default in the app directory, there are times when you need interactivity on the client side—for example, handling button clicks, animations, or real-time updates. That’s where Client Components come in.
How to Define a Client Component:
To explicitly define a component as client-side, add 'use client';
at the top of the file. This tells Next.js to load the component on the client.
Example:
Here’s a simple Client Component that handles a button click:
'use client';
export default function InteractiveComponent() {
return <button onClick={() => alert('Clicked!')}>Click Me</button>;
}
- Without
'use client';
, this component would default to being a Server Component, which cannot handle browser-specific interactions likeonClick
.
📚 Structuring Your Next.js App Directory
A well-organized app directory is the backbone of any scalable Next.js application. The app directory in Next.js 13 introduces a structure that’s both intuitive and powerful, making it easier to maintain and scale your application.
Here’s a suggested structure to get you started:
File Structure Example:
app/
layout.js // Shared layout for all routes
page.js // Homepage at /
about/
page.js // About page at /about
blog/
[slug]/
page.js // Dynamic blog post at /blog/[slug]
api/
products/
route.js // API endpoint at /api/products
What This Structure Does:
-
layout.js
:- Acts as a global wrapper for your application.
- Contains shared components like headers, footers, or navigation.
-
page.js
:- Defines individual pages. For example,
page.js
in the root is your homepage. - Use one
page.js
per route to define its content.
- Defines individual pages. For example,
-
Dynamic Routes (
[slug]
):- The
[slug]
folder underblog/
handles dynamic URLs like/blog/my-first-post
or/blog/nextjs-guide
.
- The
-
API Routes:
- The
api/
folder contains server-side API logic. - For instance,
route.js
inproducts/
defines an API endpoint for/api/products
.
- The
💡 Pro Tip: Start small. If your app grows, add folders for features or sections like dashboard/
or settings/
to keep everything tidy.
🚀 Advantages of Using the Next.js App Directory
The Next.js app directory isn’t just a shiny new feature—it’s a reimagining of how we structure modern web applications. Here are its key benefits:
1. Improved Performance
- Server Components: The app directory uses server rendering by default, ensuring that only the essential HTML is sent to the client.
- This reduces the amount of JavaScript needed, leading to faster load times and better user experiences.
2. SEO-Friendly
- Dynamic routing and server-side rendering make your content easily crawlable by search engines.
- Faster load times and optimized metadata contribute to higher rankings.
3. Ease of Maintenance
- The modular structure of the app directory means you can work on individual parts of the application without affecting others.
- Debugging becomes simpler because related logic (routes, components, and API endpoints) is colocated.
4. Scalability
- Whether you’re building a small personal site or a large enterprise application, the app directory scales effortlessly.
- You can add nested routes, dynamic pages, and API endpoints without cluttering your codebase.
😄 Let’s Get Playful: “The App Directory Cookout”
Imagine you’re hosting a cookout for your friends. You’ve got a bustling kitchen where everything is organized perfectly to whip up a fantastic meal. This kitchen is your Next.js app directory.
Here’s how the analogy works:
-
The Fridge (Server-Side Components):
The fridge stores ingredients that need to stay cool—like your server-rendered components. These components handle “cold data,” such as fetching information from a database or API, ensuring that your dishes (pages) are fresh and fast to serve. -
The Pantry (Client-Side Components):
The pantry holds dry goods—your client-side components that bring “hot interactivity.” These are the clickable buttons, animations, and dynamic content that spice up your app, just like condiments spice up your food. -
The Recipe Book (Layouts and Routes):
The recipe book is your blueprint. It defines the steps (routes) and ensures each dish (page) is served with the right presentation (layout). Once you follow the instructions, every dish comes out consistently delicious.
Why the App Directory Makes Life Easier:
Just like a well-organized kitchen helps you cook effortlessly, the app directory helps developers manage their projects with ease. No more searching through messy cabinets or files—everything has its place, making the development process smooth and enjoyable.
🌟 Why Choose Prateeksha Web Design?
At Prateeksha Web Design, we’re like seasoned chefs in the world of web development. Whether you need a simple portfolio site, an advanced e-commerce store, or a complex application built with Next.js, we’ve got the expertise to bring your vision to life.
Here’s What Sets Us Apart:
-
Deep Expertise in Next.js:
We stay ahead of the curve by mastering the latest features, including the app directory, Server Components, and dynamic routing. -
Customized Solutions:
Just like every cookout has its unique menu, we tailor your website to meet your specific needs, whether it’s for a small business or a large enterprise. -
Focus on Performance and Scalability:
We optimize every line of code to ensure your site is fast, scalable, and ready to grow with your business. -
User-Centric Design:
We prioritize creating beautiful, intuitive designs that leave a lasting impression on your audience.
Ready to Cook Up Something Amazing?
Whether you’re new to web development or an experienced techie, the Next.js app directory is a tool you’ll love. And with Prateeksha Web Design by your side, you’ll not only learn the ropes but also build an incredible web experience.
💡 Practical Tips for Using the App Directory
- Start Small: Experiment with a single page and layout to understand the basics.
- Organize Early: Plan your file structure before diving into coding.
- Use Documentation: The official Next.js guide is your best friend.
- Ask for Help: Whether it’s the Next.js community or professionals like Prateeksha Web Design, don’t hesitate to reach out.
🏁 Wrapping It Up
The Next.js app directory is a game-changer. With features like file-based routing, Server Components, and layouts, it simplifies complex processes and enhances performance. Whether you’re building a personal portfolio, a blog, or a full-fledged application, mastering the app directory is a skill worth investing in.
So, what are you waiting for? Open up your text editor, start a new Next.js project, and explore the app directory. Need guidance? Reach out to Prateeksha Web Design — we’re here to make your web development journey seamless and fun.
About Prateeksha Web Design
Prateeksha Web Design offers expert services in Next.js app directory management, providing a thorough understanding of its structure and functionality. Our team customizes web solutions that leverage Next.js features for enhanced performance and scalability. We conduct in-depth training sessions to empower clients with the knowledge to navigate and utilize the app directory effectively. Additionally, we provide ongoing support to ensure seamless integration and optimization of Next.js applications. Experience a streamlined approach to web development with Prateeksha's specialized Next.js services.
Interested in learning more? Contact us today.
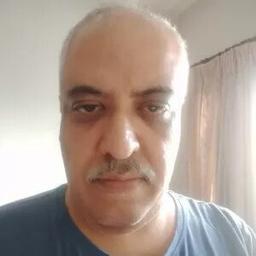