If you’re a 20-something looking to make your life easier through technology, Python is your best friend. It’s versatile, easy to learn, and has a ton of libraries and scripts designed specifically for workflow automation. Whether you’re a programming geek diving into code or just someone curious about simplifying repetitive tasks, this guide will help you automate tasks with Python while boosting your productivity.
In this blog, we’ll break down the best Python libraries for automation, offer real-world examples, and share scripts to save time. Plus, we’ll highlight how Prateeksha Web Design leverages these tools to create efficient workflows for businesses.
Why Python for Workflow Automation?
Python is often called the “Swiss Army knife” of programming because of its:
- Simplicity: Easy syntax that’s perfect for beginners.
- Versatility: Wide range of applications, from web development to data analysis.
- Extensive Libraries: Pre-built modules for almost any task you can imagine.
- Community Support: Tons of resources, tutorials, and forums for programming geeks.
Automation is all about saving time and increasing efficiency, and Python’s flexibility makes it the go-to language for task automation.
Key Benefits of Workflow Automation with Python
Before diving into the tools, let’s explore the benefits:
- Saves Time: Automating repetitive tasks frees up time for creative and strategic work.
- Reduces Errors: Manual processes are prone to mistakes, but scripts are consistent.
- Boosts Productivity: Automating processes ensures smoother workflows.
- Scales Easily: Python scripts can grow with your business needs.
- Cost-Effective: No expensive software is required—just a little coding effort.
Top Python Libraries for Workflow Automation
Here’s a curated list of the best Python libraries for automation that every program geek should know.
1. Pandas: Data Manipulation Made Easy
Pandas is the go-to library for handling data. Whether you’re cleaning spreadsheets, analyzing trends, or organizing messy datasets, Pandas makes it seamless.
- Use Case: Automating the cleaning of sales data for a Shopify store.
- Key Features:
- Handles large datasets efficiently.
- Easy to merge, filter, and pivot data.
- Built-in support for CSV, Excel, and SQL files.
Example:
import pandas as pd
# Automate cleaning sales data
data = pd.read_csv('sales_data.csv')
data['Total'] = data['Price'] * data['Quantity']
data.to_csv('cleaned_sales_data.csv', index=False)
This script can save hours of manual effort in e-commerce data management.
2. Selenium: Automate Browser Tasks
If you hate manually filling out forms or scraping data from websites, Selenium is a lifesaver. It allows you to control your web browser programmatically.
- Use Case: Automating product uploads to Shopify.
- Key Features:
- Automates browser interactions like clicking, filling forms, and navigation.
- Supports all major browsers.
Example:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://shopify.com/login')
# Automate login
driver.find_element_by_id('username').send_keys('your_email')
driver.find_element_by_id('password').send_keys('your_password')
driver.find_element_by_id('login_button').click()
Imagine automating repetitive admin tasks in minutes!
3. BeautifulSoup: Web Scraping Simplified
BeautifulSoup is a lightweight library for scraping information from websites. Combine it with Selenium for maximum impact.
- Use Case: Gathering competitor pricing data.
- Key Features:
- Parses HTML and XML documents.
- Extracts specific data points efficiently.
Example:
from bs4 import BeautifulSoup
import requests
response = requests.get('https://example.com/products')
soup = BeautifulSoup(response.text, 'html.parser')
# Extract product names
products = [item.text for item in soup.find_all('h2', class_='product-title')]
print(products)
4. Schedule: Your Personal Task Scheduler
Schedule is a simple yet powerful library for running Python tasks at specific intervals.
- Use Case: Running a daily email report at 8 AM.
- Key Features:
- Easy-to-use syntax.
- Supports interval-based task execution.
Example:
import schedule
import time
def job():
print("Automated task running...")
schedule.every().day.at("08:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
5. PyAutoGUI: Automate Mouse and Keyboard Actions
PyAutoGUI is perfect for automating GUI-based tasks like clicking buttons, typing text, or moving the mouse.
- Use Case: Automating repetitive desktop tasks.
- Key Features:
- Controls mouse and keyboard.
- Captures screen coordinates for precise actions.
Example:
import pyautogui
# Automate opening a program
pyautogui.click(100, 100) # Click at specific coordinates
pyautogui.typewrite('hello world!', interval=0.1)
pyautogui.press('enter')
Scripts to Save Time and Boost Productivity
Here are a few practical examples to inspire your automation journey.
Automating Email Reports
Using Python libraries like smtplib, you can automate email updates for teams.
import smtplib
from email.mime.text import MIMEText
def send_email():
msg = MIMEText("Daily report attached.")
msg['Subject'] = 'Daily Report'
msg['From'] = 'you@example.com'
msg['To'] = 'team@example.com'
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login('you@example.com', 'password')
server.send_message(msg)
send_email()
Automating File Management
With os and shutil, you can organize files automatically.
import os
import shutil
source_dir = '/downloads'
dest_dir = '/documents'
for file_name in os.listdir(source_dir):
if file_name.endswith('.pdf'):
shutil.move(f'{source_dir}/{file_name}', dest_dir)
Automating Social Media Posts
Using Tweepy for Twitter automation:
import tweepy
# Authenticate with Twitter API
auth = tweepy.OAuthHandler('API_KEY', 'API_SECRET')
auth.set_access_token('ACCESS_TOKEN', 'ACCESS_SECRET')
api = tweepy.API(auth)
# Post a tweet
api.update_status("Automating my Twitter feed with Python! #PythonAutomation")
How Prateeksha Web Design Excels with Python Automation
At Prateeksha Web Design, we’ve mastered the art of using Python for workflow optimization. From automating e-commerce processes to streamlining client communication, we deliver tailored solutions that save time and boost productivity for businesses.
- Custom Scripts: We develop bespoke scripts for task automation with Python.
- Efficient Workflows: Leveraging libraries like Pandas, Selenium, and Schedule, we enhance operational efficiency.
- Business Integration: Our automation solutions align perfectly with your business needs, whether it’s managing inventory or running marketing campaigns.
Final Thoughts: Unlocking Efficiency with Python
Whether you’re a beginner Python automation guide follower or a seasoned programming geek, Python offers limitless possibilities for automating repetitive tasks. By mastering libraries like Pandas, Selenium, and PyAutoGUI, you can transform your workflows and save countless hours.
Ready to boost productivity with Python? Partner with Prateeksha Web Design, and let us help you automate your way to success.
Interested in learning more? Contact us today or read more about Python Automation techniques. For comprehensive resources on Python libraries, visit Python Package Index.
FAQs
-
What is Python and why is it recommended for workflow automation? Python is a versatile and easy-to-learn programming language with a wide range of applications. It is recommended for workflow automation due to its simple syntax, extensive libraries, and strong community support, making it ideal for both beginners and experienced programmers.
-
What are some key benefits of automating tasks with Python? Automating tasks with Python can save time by freeing you from repetitive tasks, reduce errors in manual processes, boost overall productivity, allow for easy scaling as business needs grow, and be cost-effective since it requires minimal software investment.
-
Which Python libraries are best for workflow automation? Some of the best Python libraries for workflow automation include Pandas for data manipulation, Selenium for automating browser tasks, BeautifulSoup for web scraping, Schedule for task scheduling, and PyAutoGUI for automating mouse and keyboard actions.
-
Can you provide an example of how to use Python for automating email reports? Yes, using the
smtplib
library, you can automate sending email reports. An example script creates an email message and sends it using an SMTP server, allowing for daily updates or notifications to teams. -
How does Prateeksha Web Design utilize Python for automation? Prateeksha Web Design leverages Python to create efficient workflows for businesses by developing custom scripts for task automation, enhancing operational efficiency with libraries like Pandas and Selenium, and integrating automation solutions with business needs like inventory management and marketing campaigns.
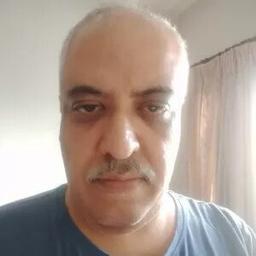