Table of Contents:
- Introduction to WordPress Plugin Development
- Understanding the Core of a WordPress Plugin
- Setting Up Your Development Environment
- Creating Your First WordPress Plugin
- Understanding Hooks and Filters
- Security Best Practices in WordPress Plugin Development
- How to Handle Updates for Your Custom Plugin
- Testing and Debugging Your Plugin
- Advanced Features and Customization
- Deploying Your Custom WordPress Plugin
- Conclusion and Best Practices
1. Introduction to WordPress Plugin Development
WordPress plugin development is an exciting journey that allows you to add unique functionality to your website. A plugin in WordPress is a piece of software that you can add to your site to extend its capabilities. There are thousands of plugins available in the WordPress plugin directory, but sometimes, you need something custom to suit your specific needs.
In this tutorial, we'll walk you through step-by-step how to create a custom WordPress plugin from scratch. Whether you're new to coding or familiar with PHP (the language WordPress is built on), we'll cover everything in detail, so you understand not only how but also why we perform each step.
With recent advancements in WordPress development, it's easier than ever to integrate modern functionalities like REST API, custom post types, or even connect your plugin with external services. Let’s dive deep into understanding the basics, so by the end of this blog, you’ll be equipped to create a powerful plugin tailored to your needs.
2. Understanding the Core of a WordPress Plugin
At the heart of every WordPress plugin is a simple PHP file that contains a small header comment. This header allows WordPress to recognize the file as a plugin and make it available in the dashboard. Even though this sounds simple, a plugin can range from a few lines of code to thousands, depending on what you want it to do.
What Makes a Plugin?
A WordPress plugin is primarily composed of:
- PHP code: This handles the core logic of the plugin.
- HTML/CSS/JavaScript: To create user interfaces, if required.
- Database operations: Some plugins need to interact with the WordPress database, and for that, we use MySQL queries.
- Hooks and filters: These are essential for interacting with WordPress's core functionalities without altering the core files. We'll explain this in greater detail in the later sections.
Plugin Anatomy
- Plugin header: A specific comment block that tells WordPress about the plugin (name, version, author, etc.).
- Main plugin file: Where most of the core logic resides.
- Other resources: If your plugin is more complex, it may contain additional files like CSS, JavaScript, and language files.
This section will set up the foundation and knowledge needed to move on to the more complex aspects of creating a custom WordPress plugin.
3. Setting Up Your Development Environment
Before we can create a plugin, it’s essential to set up a proper development environment. Having a good setup helps you efficiently code, debug, and test your plugin without affecting a live website.
Tools You Will Need
- Local development environment: Software like XAMPP, MAMP, or Local by Flywheel allows you to run WordPress on your computer without needing a live server.
- Code editor: Visual Studio Code, Sublime Text, or PHPStorm are some popular editors.
- Version control: It’s highly recommended to use Git or another version control system to manage changes to your plugin. Platforms like GitHub or Bitbucket make this easy.
Setting Up WordPress Locally
- Install XAMPP or MAMP to get a local server running.
- Download WordPress from wordpress.org and extract it into your server’s root folder.
- Create a MySQL database using phpMyAdmin.
- Run the WordPress installation wizard to set up your local site.
Once the local site is running, you're ready to start coding your plugin! This local setup allows you to experiment without any consequences, and you can easily test changes to your plugin instantly.
4. Creating Your First WordPress Plugin
Now that our environment is ready, let’s dive into actually creating the plugin. A custom WordPress plugin begins with a simple PHP file placed in the wp-content/plugins/
directory.
Step-by-Step Guide to Creating the Plugin:
- Navigate to the
wp-content/plugins/
directory in your WordPress installation. - Create a new folder for your plugin, for example,
my-first-plugin
. - Inside that folder, create a PHP file. Let’s name it
my-first-plugin.php
. - In that PHP file, begin by adding the following comment block at the top: `php
- To see if your plugin is recognized by WordPress, go to your dashboard, and under "Plugins," you should see "My First Plugin." Activate it!
At this point, you have created your very first custom WordPress plugin. It doesn't do anything yet, but the groundwork is laid for building upon this.
5. Understanding Hooks and Filters
Hooks and filters are the backbone of any WordPress plugin development. They allow you to "hook into" existing WordPress functionality or modify how WordPress behaves without altering core files.
Hooks: These are specific points in WordPress where you can attach your custom code.
- Action hooks: Execute custom code at specific points. For example, adding code to the footer of a website.
- Filter hooks: Modify content or data before it's rendered. For example, changing the content of a post before it’s displayed.
How to Use Hooks in a Plugin
// Add code to the footer
function my_footer_message() {
echo '<p>This is my custom footer message!</p>';
}
add_action('wp_footer', 'my_footer_message');
The add_action
function hooks our custom my_footer_message()
function to WordPress’s wp_footer
action, which runs just before the closing </body>
tag.
Understanding how to use hooks and filters will allow you to make your plugin powerful by integrating deeply with WordPress's core functions.
6. Security Best Practices in WordPress Plugin Development
When building a custom WordPress plugin, security should be a top priority. A plugin that doesn't follow best practices can expose a website to a variety of vulnerabilities, from SQL injections to XSS attacks.
Key Security Considerations
- Sanitize input: Always sanitize any input coming from users.
- Escape output: When displaying data, ensure it’s escaped to prevent malicious scripts.
- Nonces: Use nonces to verify actions on forms or URL parameters.
- Database security: Use WordPress’s database functions like
$wpdb->prepare()
to prevent SQL injection attacks.
We’ll explore more in detail how you can secure your plugin and avoid common pitfalls in this section.
7. How to Handle Updates for Your Custom Plugin
Once your WordPress plugin is live, you'll likely need to update it to add new features, fix bugs, or address security vulnerabilities. But how do you ensure your users can smoothly update your plugin without any issues?
In this section, we'll look at how WordPress handles updates for plugins and provide tips on:
- Versioning your plugin properly.
- Providing update notifications.
- Handling backward compatibility.
- Safely updating database structures or plugin options.
With the right strategy, you'll ensure that your users always have the latest, secure version of your plugin without breaking their sites.
8. Testing and Debugging Your Plugin
Before releasing your plugin or using it on a live site, thorough testing and debugging is crucial. In this section, we'll explore:
-
Setting up WordPress debugging modes.
-
Using PHP error logs to capture issues.
-
Testing in multiple browsers and environments.
-
Utilizing tools like WP-CLI and Unit Tests to automate some of the testing.
Testing will ensure that your plugin is stable, performs well, and doesn't introduce any issues to the broader WordPress ecosystem.
9. Advanced Features and Customization
Once you've mastered the basics, there are endless possibilities for making your plugin even more feature-rich. This section will cover:
- Adding custom settings pages to allow users to customize the plugin.
- Integrating with the WordPress REST API for complex data interactions.
- Creating custom post types or shortcodes.
- Localizing your plugin for different languages.
By diving into these advanced topics, you’ll be able to create truly custom and unique plugins that can cater to a wide range of use cases.
10. Deploying Your Custom WordPress Plugin
Once you’re ready to share your plugin with the world, it's time to deploy it. Whether you want to keep it for private use or share it with the WordPress community, we’ll explore:
- How to package your plugin correctly.
- How to submit your plugin to the WordPress plugin directory.
- Best practices for versioning, documentation, and updates.
Deploying is the final step in your WordPress plugin development journey, but it requires careful attention to ensure everything works smoothly for the end user.
11. Conclusion and Best Practices
Creating a custom WordPress plugin can seem daunting, but by breaking it down into manageable steps, anyone can do it. From understanding the basics of PHP, to exploring advanced features like custom post types, you now have the tools you need to bring your ideas to life.
About Prateeksha Web Design
Prateeksha Web Design Company is a leader in providing digital solutions, specializing in WordPress plugin development. They offer a detailed step-by-step tutorial on creating effective custom WordPress plugins, guiding users from the initial concept to final implementation. Their service is designed to help clients enhance website functionality while ensuring a seamless user experience.
Interested in learning more? Contact us today.
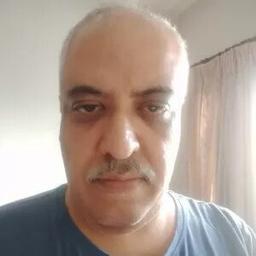