Dive into the Whimsical World of Laravel Queues and Job Handling!
Greetings, aspiring Laravel magician! 👋 Prepare yourself for an exhilarating journey into the delightful realm of Laravel queues, job handling, and the fascinating world of asynchronous processing. Whether you're an enthusiastic beginner or a seasoned veteran seeking a refresher, this comprehensive guide is designed to ensure you're not just skimming the surface but diving headfirst into the vast ocean of Laravel's powerful capabilities.
Understanding Laravel Queues: The Basics
To fully appreciate what Laravel queues bring to the table, let's take a moment to grasp the underlying concept. Picture yourself in a bustling restaurant. Every dish must be meticulously prepared, and if each order requires immediate attention from the kitchen, you can bet the chaos would ensue. This is the essence of queueing: it allows you to offload time-consuming tasks—such as sending emails, processing file uploads, or any operation that doesn’t yield instant results—into the background, allowing your application to run smoothly without keeping users waiting.
The Magic of Laravel Queues
Here are some compelling reasons to incorporate queues into your Laravel application:
-
Performance Boost: By moving long-running tasks to the background, you significantly reduce wait times for your users, enhancing their experience.
-
Efficient Task Management: Laravel's queue system helps you organize jobs systematically, avoiding the headaches of a cluttered workflow.
-
Automatic Retries: If a job fails for any reason, Laravel intelligently handles retries, ensuring resilience within your system.
Through Laravel’s queueing mechanism, you can manage a lineup of tasks waiting to be accomplished, transforming your application from a frantic, disorganized restaurant into a well-coordinated, efficient culinary operation.
Setting Up Laravel Queues: A Step-by-Step Guide
Let's get rolling with the setup! Starting with a properly installed Laravel project, you're just a few steps away from integrating queues into your application.
Step 1: Choose Your Queue Driver
Laravel supports several queue drivers, each tailored to different needs. However, for this guide's simplicity, we will use the database driver. First, configure your .env
file:
QUEUE_CONNECTION=database
This line tells Laravel to use the database driver to manage and execute queued jobs.
Step 2: Create the Jobs Table
Since you're likely well-versed in web development (shoutout to the skillful Prateeksha Web Design!), you'll appreciate that databases form the backbone of job management in Laravel. To set up a structure for storing queue jobs, create a migration with the following commands:
php artisan queue:table
php artisan migrate
This process creates a new table in your database specifically for tracking queued jobs, ensuring they hang tight until their time to shine arrives.
Step 3: Crafting a Job
Next, it's time to create a job that encapsulates the logic for the asynchronous task you wish to perform. To generate a new job, run:
php artisan make:job ProcessPodcast
This command generates a new job class located in app/Jobs/
. Let’s bring some magic into play! Imagine your goal is to send a thoughtful thank-you email to users after they complete a purchase. Your ProcessPodcast
job is the perfect tool for this task. The job structure might look something like this:
namespace App\Jobs;
use Mail;
class ProcessPodcast implements ShouldQueue
{
public function handle()
{
// Here's where the email-sending logic kicks in
Mail::to('user@example.com')->send(new ThankYouEmail());
}
}
In this example, the handle
method contains the code that will be executed when this job is dispatched. It's where you define what happens—like sending an email to users, ensuring they feel special and valued.
Step 4: Dispatching the Job
Once you have your job ready, the next step is to dispatch it, which means queueing it for execution. This process is akin to placing an item in the oven instead of cooking it right away—allowing other processes to run in the meantime. You can dispatch your job whenever a specific event occurs in your application, such as user registration or order placement, using the following command:
ProcessPodcast::dispatch();
This command puts the job into the queue, and it will run based on your queue worker configurations.
Step 5: Running the Queue Worker
To ensure your queued jobs are processed, you need to run a queue worker. This worker will continuously listen for new jobs and execute them as they arrive. Start the queue worker with the following command:
php artisan queue:work
The worker pulls jobs from the queue and executes them in the background, all while you focus on enhancing other areas of your application.
Wrapping Up
Congratulations, you've taken your first steps into the joyful and magical world of Laravel queues and job handling! Asynchronous processing can significantly enhance your application's performance and user experience, allowing you to manage tasks efficiently.
So, whether you’re sending thank-you emails or processing files, remember that with Laravel queues, you’re empowered to create a seamless and responsive application experience. As you continue exploring this whimsical realm, keep experimenting, learning, and, most importantly, have fun! Happy coding! 🎉
Certainly! Let's expand, explain, and refine the content related to Laravel queues, job scheduling, queue workers, and Laravel Horizon for a clearer understanding.
Setting Up Laravel Queues
Using Laravel queues allows you to defer the execution of a time-consuming task, such as sending an email or processing video uploads. By dispatching a job to a queue, you can improve the performance and speed of your application, as it can continue processing requests without waiting for these tasks to complete. For instance, when checking off a podcast for processing, you execute the following command:
ProcessPodcast::dispatch($podcast);
This line of code creates a new job instance and dispatches it to the queue. The $podcast
variable represents the specific podcast instance you want to process, and the queued job will handle the processing of that podcast in the background.
And voilà! You have successfully set up the Laravel queue system.
Laravel Job Scheduling
Once you're familiar with queues, it’s time to inject some job scheduling functionality. Job scheduling allows you to automate tasks at specified intervals, making it an invaluable tool for any application.
How to Schedule Jobs
Laravel simplifies job scheduling with a clean syntax. You can define your scheduled tasks in the app/Console/Kernel.php
file. Here's an example of how to schedule a job to run every hour:
protected function schedule(Schedule $schedule)
{
$schedule->job(new ProcessPodcast)->hourly();
}
In this code snippet, the schedule
method accepts an instance of the Illuminate\Console\Scheduling\Schedule class, allowing you to define various schedules for your jobs. The hourly()
method indicates that the job will execute every hour.
When Would You Use This?
Consider a scenario where you're operating an e-commerce site—possibly the next big thing in ecommerce website development in Mumbai. Scheduling tasks can significantly enhance operational efficiency. For instance, you might want to generate sales reports every hour to keep track of your performance metrics effortlessly. The automation of such tasks through scheduling can save you time and ensure critical operations run seamlessly.
Laravel Queue Workers
Queue workers are the backbone of your queue system, akin to dedicated staff in a restaurant working to fulfill customer orders. Each worker listens for jobs on the queue and processes them accordingly.
Starting a Queue Worker
To initiate a queue worker, use the following artisan command:
php artisan queue:work
This command launches the worker, and it begins to process jobs as they are dispatched. If you want to specify the queue connection you’re using (for example, if you have multiple queues), you can do so with:
php artisan queue:work database
This tells Laravel to listen to jobs specifically on the database
queue.
Monitoring Workers
Just as a skillful chef monitors the kitchen's activity, ensuring everything is running smoothly, it’s essential to supervise your queue workers. Tools such as Laravel Horizon can assist in effectively managing your workers. Horizon provides a comprehensive dashboard for monitoring queue performance, allowing you to track job completion times, retry failed jobs, and more.
Laravel Horizon: Your Queue Companion
Now let’s spotlight Laravel Horizon, an exceptional tool that enhances your queue management experience.
Why Use Laravel Horizon?
-
Dashboard: Horizon offers a beautiful, real-time dashboard that presents an overview of your jobs and queues, allowing you to visualize performance metrics.
-
Cool Metrics: It provides insights into job throughput, execution time, and other valuable metrics to help you understand how efficiently your queues function.
-
Retry Jobs: Horizon allows for easy retries of failed jobs with a single click, minimizing hassle and manual intervention.
How to Install Laravel Horizon
To use Laravel Horizon, you first need to install it via Composer:
composer require laravel/horizon
Once installed, you can launch Horizon with the following command:
php artisan horizon
This command initializes the Horizon dashboard, which you can access via your web browser. From there, you'll be able to monitor your queue jobs in real-time, offering a clear view of your application's queued tasks.
With the combination of Laravel queues, job scheduling, workers, and Horizon, you can streamline your application’s backend operations, ensuring that your tasks are executed efficiently and effectively, and freeing up resources for other important tasks. This leads to a more responsive and robust application overall!
php artisan horizon
When you run this powerful command in your terminal, it's like the lights coming on at a concert—the show is about to begin! In this context, Horizon refers to Laravel Horizon, a powerful dashboard for managing and monitoring your Laravel queues. With this command, you launch a beautiful, user-friendly interface that displays the status of your queued jobs, the performance metrics of your workers, and all the insights necessary to ensure your application runs smoothly. It’s an invaluable tool for any Laravel developer eager to optimize their job processing.
Elevating Your Workflow: Laravel Task Automation
In today's fast-paced digital landscape, where every second counts, task automation is the unsung hero of productivity. Picture this: you’d much rather kick back with a binge-worthy series than be tied down to repetitive, mundane tasks. This is where automation steps in as your reliable ally, streamlining processes and freeing your time for more creative endeavors.
Automating Tasks with Laravel Jobs
Laravel's job system enables you to automate virtually any repetitive task efficiently. From sending automated reminders to clients to scraping social media for trends to keep your web design services in Mumbai ahead of the competitive curve, job automation is a game changer.
Consider this light-hearted scenario: you want to effortlessly send out a weekly newsletter to keep your clients informed and engaged. By creating a job to execute this task, scheduling it, and letting Laravel handle the rest, you’re set for a seamless experience. Your users will thank you for the timely information, and you’ll get to put your feet up, potentially with your favorite snack in hand.
Optimizing Performance: Laravel Async Processing
Now, let's shift gears and explore the concept of asynchronous processing in Laravel. To illustrate, think of placing a takeout order—you make the call, place your order, and while you wait for your food to be prepared, you’re free to do as you please. That’s the beauty of async processing!
Benefits of Async Processing
- Efficiency: Async processing allows you to execute multiple tasks simultaneously, drastically improving how well your application performs without blocking other operations.
- Faster User Experience: Users love quick responses. By implementing async processing, you ensure that they remain engaged with your application, even during complex background tasks.
Implementing Async Processing
In Laravel, tasks pushed onto the queue are processed asynchronously. It’s like multitasking at its finest—while you wait for a large order to finish, the rest of your application stays responsive. Your users can continue exploring your sleek eCommerce platform without interruption, leading to an improved overall experience!
The Art of Communication: Laravel Event Handling
Let’s enliven the discussion with event handling in Laravel. Think of this as the dance floor for your application where different components interact smoothly, creating a harmonious user experience.
Understanding Event Handling
Events are like triggers within your application. Whenever a significant action occurs—such as a new purchase being made—an event can be fired off. This allows you to listen for specific events and execute designated jobs in a structured and efficient manner.
Setting Up Events and Listeners
To create an event in your Laravel application, you simply use Artisan commands:
php artisan make:event OrderShipped
Next, you’ll want a listener to take action when the event is triggered:
php artisan make:listener SendShipmentNotification
Once you wire these components together in your EventServiceProvider
, you transform your application into a finely tuned notification system, responding to events almost as if it has a mind of its own!
The Whole Package: Integrating Everything Together
Throughout this journey, we have explored Laravel queues, job scheduling, and the magic of asynchronous processing. You’ve learned to monitor everything with Laravel Horizon and automate tasks like a seasoned professional. 🌟
At Prateeksha Web Design, we firmly believe in harnessing the capabilities of Laravel to create extraordinary user experiences. The top web design company in Mumbai is already leveraging these techniques to enhance their clients’ websites, making them faster, more efficient, and ultimately more enjoyable for users. Imagine the wonders when you apply this knowledge to your own projects in eCommerce website design in Mumbai!
Final Thoughts and Call to Action
As you embark on your Laravel journey, remember this: Failure is a stepping stone to success! Bugs and glitches are merely part of the development process, and embracing them will help you evolve into a more formidable web developer. Each minor setback is a learning opportunity, making the path to mastery all the more enriching.
So dive deeper into the limitless possibilities that Laravel offers! Break through those queues, schedule those tasks, and automate like it’s second nature. Your audience will appreciate the enhanced functionality, and you will find joy in the creativity of development! 🎉
And if you need support or a talented design team to elevate your projects, reach out to Prateeksha Web Design. We're dedicated to transforming your web aspirations into reality, whether through a personal website or your next big e-commerce initiative! Let’s create something extraordinary together!
Tip: Make sure to use Laravel's built-in queue drivers that best fit your application’s needs. For local development, consider using the "sync" driver to process jobs immediately, which can simplify debugging. For production, drivers like Redis or Amazon SQS provide better performance and scalability.
Fact: Laravel queues provide a powerful way to defer the processing of a time-consuming task, such as sending emails or processing images. By offloading these tasks to the queue, you can greatly improve your application’s responsiveness and user experience.
Warning: Always handle job failures gracefully by implementing retries and proper logging. Failing to do so can lead to lost jobs and hidden errors that could significantly impact your application's functionality and user trust. Make sure to monitor your queue and configure your job timeouts appropriately to avoid hanging jobs.
About Prateeksha Web Design
Prateeksha Web Design offers expert services in optimizing Laravel applications through their comprehensive approach to queues and job handling. They provide tailored solutions to streamline background processing, enhance application performance, and improve user experience. Their team guides clients through best practices, ensuring efficient job management and error handling. Additionally, they offer ongoing support and customization to meet unique project needs. Elevate your web applications with Prateeksha's specialized Laravel expertise.
Interested in learning more? Contact us today.
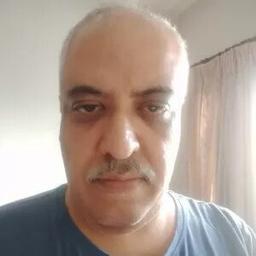