Speed Up Your Laravel Application: Tips, Tricks, and a Dash of Humor
Welcome to the bustling world of Laravel Performance Optimization! If you're reading this, you're likely a developer or a curious soul looking to enhance the speed and responsiveness of your Laravel applications. Whether you’re maintaining a vibrant ecommerce website design in Mumbai or a sleek portfolio site, the speed and performance of your app can be the defining factor between a delighted user and a frustrated one ready to leave your site faster than a cheetah on roller skates. So, let’s dive right into this fun, quirky, yet professional journey of Laravel optimization!
Key Takeaway #1: Embrace Laravel Caching
First up on our list is the magic of Laravel caching. Think of caching as your overachieving friend who’s always five steps ahead in a race. When you cache data, you’re essentially storing a copy of it in a faster-access location. This means that instead of frequently hitting your database (which can be as slow as a snail in a marathon), your application fetches a ready-made copy. It's like having your dessert ready before dinner—incredible, right?
How to Implement Caching
Laravel provides various caching drivers, including file, database, Memcached, and Redis. If you’re feeling fancy and want that high-speed experience, go for Redis; it’s like the Ferrari of caching systems!
To get started with caching, you'll want to familiarize yourself with the following methods:
// Storing data in cache
Cache::put('key', 'value', $minutes);
// Retrieving data from cache
$value = Cache::get('key');
Example in Action
Let's say you're pulling product information for an ecommerce website. Instead of querying the database every single time a user looks for a product, you can cache it like so:
$products = Cache::remember('products', 60, function () {
return DB::table('products')->get();
});
Why Does It Matter?
Every time a user requests data, hitting the database for every little thing can lead to performance shakes, rattles, and rolls. Consider this: What’s faster? A rabbit in a sprint, or a rabbit that has to stop and ask for directions every time it goes in circles? Exactly—your application should be that sprinting rabbit.
Key Takeaway #2: Master the Laravel Query Builder
Next on our journey is the Laravel query builder. This isn’t just another tool; it’s practically a magic wand that helps you retrieve data efficiently, allowing you to optimize queries without losing that sweet readability Laravel is known for.
Simplicity is Key
Using Eloquent is fantastic for many scenarios, but sometimes you need the chameleonic speed of a gazelle. The query builder allows you to write raw SQL expressions to get exactly what you need without the added overhead that comes with using Eloquent’s ORM.
Performance Improvement
For instance, instead of doing the somewhat sluggish:
$result = DB::table('users')->where('active', 1)->get();
You can improve performance with a more direct call using:
$result = DB::select('SELECT * FROM users WHERE active = 1');
Inner joins, grouping, and limiting results become easier and faster through these Laravel database optimization techniques. Think of query optimization like being frugal with your snacks—never waste them!
Best Practices for Query Optimization
- Limit Your Data: Only fetch the columns you need. If you only need the user's email, don’t fetch the whole user record.
$emails = DB::table('users')->pluck('email');
- Use Pagination: Instead of loading a whole dataset, paginate your results to improve load time.
$users = DB::table('users')->paginate(15);
- Use Indexes: Make sure your database indexes are set up correctly to improve query speed significantly.
Summary: Small Changes, Big Impact
The performance of your Laravel application hinges not just on speed, but on efficiency and responsiveness. With caching and optimized queries, you can ensure that even during peak traffic, your application handles user requests smoothly. Achieving this may require some initial effort, but the payoff in user satisfaction is absolutely worth it.
So, buckle up, implement these optimizations, and watch your Laravel application zoom past the competition—perhaps even faster than that speedy cheetah! Keep at it, sprinkle in a bit of humor, and remember: optimization is a journey, not a destination. Happy coding! 🐾
Key Takeaway #3: Database Indexing
Let’s delve into the crucial concept of Laravel database indexing. Think of it like a well-organized index in a book that allows you to quickly find pivotal chapters on building effective APIs—similarly, a database index accelerates search processes in your application.
Understanding Database Indexing
When you imagine searching for a needle in a haystack, you've got two approaches: you might sift through every single straw of hay, or you could utilize a magnet to quickly pinpoint the needle. In this analogy, database indexes serve as the magnet, turning lengthy, laborious queries into swift, efficient operations.
In Laravel, adding an index can significantly enhance the performance of your database by allowing the system to retrieve data without sifting through every row. Here’s how you do it:
Schema::table('users', function (Blueprint $table) {
$table->index('email');
});
This example illustrates how to create an index for the 'email' column in the 'users' table. By doing this, any query that searches through the 'email' field can execute much faster, as the database can now directly access the indexed entries.
Pro Tip for Database Indexing
It's crucial to regularly audit your database indexing strategy. Over time, your application and its data requirements may evolve, making some indexes redundant or inefficient. A table overloaded with indexes can resemble a traffic jam in Mumbai—impeding performance instead of enhancing it. Therefore, aim for a balance; while indexes are essential for speeding up queries, too many can degrade performance. Make sure your database operates smoothly, like a streamlined wind tunnel rather than a congested road.
Key Takeaway #4: Harnessing the Power of Laravel Queuing
Do you have tasks that don’t need to be completed immediately, like sending out emails or generating reports? Don’t hold up your users while you’re busy processing these tasks in real-time. Instead, leverage the Laravel queue system to streamline your operations!
The Functionality of Queues
Think of Laravel queues as a way to handle tasks asynchronously. When you send tasks to a queue, it's like dispatching your responsibilities to a supporting team while you attend to your guests (or your users). Tasks can be queued up, processed at your convenience, and your users can continue enjoying a seamless experience without delay.
Here’s a simple example of how to dispatch a job in Laravel:
// Dispatching a job
dispatch(new SendEmailJob($user));
In this example, the SendEmailJob
is added to the queue, allowing your application to continue functioning smoothly while the email is sent in the background.
Choosing the Right Queue System
Laravel supports various queue backends, including Redis, Beanstalkd, and even your own database. Each backend comes with its own set of features and performance characteristics, allowing you to choose one that best fits your application's needs. Just remember, utilizing queues is like inviting your tasks to a party; don't skip out on this vital strategy!
Key Takeaway #5: Mastering Laravel Debugging
Debugging is a critical part of software development, and when managed properly, it can be as delightful as indulging in a delicious donut. However, the joy of debugging can quickly vanish if you approach it haphazardly.
The Importance of Effective Debugging
Addressing errors without a structured strategy is akin to swimming with sharks without a protective cage—you’re opening yourself up to unnecessary risks. Instead, harness tools like Laravel Telescope or Debugbar to help keep your errors in check. These tools offer insight into requests in real-time, allowing you to identify and resolve issues efficiently.
Best Practices for Debugging
Maintaining clean and organized error logs is paramount. A well-structured logging system allows you to track issues effectively, saving you substantial time and preventing future headaches. Much like a top-notch web design company in Mumbai organizes multiple web designers to craft an effective digital strategy, managing your debugging process with due diligence will help you maintain a robust application. Always keep your debugging tools and strategies up to date, ensuring your development process remains smooth and efficient.
Key Takeaway #6: Optimize Your API Speed
In today’s digital landscape, APIs (Application Programming Interfaces) serve as the vital connectors between different software components, enabling seamless communication and functionality. For developers creating APIs using the Laravel framework, investing time and resources into optimizing API performance is essential. A well-optimized API not only enhances user experience but also improves resource utilization and system efficiency.
Tips for Enhancing API Speed
-
Implement Pagination: APIs often return large datasets, which can significantly slow down response times and increase server load. By implementing pagination, you can limit the number of records returned in each API call. This ensures that the user receives only a manageable subset of data, which speeds up the response time and reduces the strain on server resources. For instance, you could retrieve results in chunks of 10, 20, or any number that fits your application’s design.
-
Use Selective Fields: Instead of sending a full record with all its fields, analyze the specific information that the client actually needs. By employing selective fields, you can minimize the size of the payload, allowing for faster transmission over the network. This selective approach not only conserves bandwidth but also decreases processing time on both the client and server sides. For example, when a client requests user data, only send fields like
name
,email
, andphone
, instead of all details, includingpassword
,created_at
, andupdated_at
. -
Implement API Rate Limiting and Throttling: Just as exclusive clubs employ bouncers to manage entry, you can use rate-limiting mechanisms to manage the number of requests a user can make to the server within a given timeframe. This not only protects your server from being overwhelmed by excessive requests but also prioritizes resource allocation for critical users. Laravel provides built-in middleware for rate limiting:
// Rate limiting example Route::middleware('throttle:10,1')->group(function () { Route::get('/api/resource', 'ApiController@index'); });
This example allows a maximum of 10 requests per minute per user, ensuring smooth access during peak times.
By applying these strategies, your APIs can operate as seamlessly as a Ferrari on an open stretch of highway rather than a scooter navigating through congested traffic.
Key Takeaway #7: Leverage Laravel Configuration Caching
One of the simplest yet most impactful optimization techniques is caching your configuration files in Laravel. Each time the application is initialized, it reads configuration files, which can introduce unnecessary latency. By caching these configurations, you significantly reduce the overhead during the application’s bootstrapping process.
To cache your configuration settings, simply execute the following command:
php artisan config:cache
Benefits of Configuration Caching
Implementing configuration caching results in a marked improvement in application performance, particularly in production environments. This process is analogous to pre-packaging your lunch to avoid the midday rush; it streamlines operations and enhances efficiency.
Key Takeaway #8: Use the Right Tools: Profiling and Monitoring
Effective performance optimization is difficult without proper visibility. To identify potential bottlenecks and areas for improvement, employ profiling tools that monitor your application’s performance. Laravel provides a built-in Profiler along with integrated metrics, and also allows for integration with third-party monitoring services. These tools can offer invaluable insights, such as memory usage, request processing time, and overall application health.
Wrap It Up
In the rapidly evolving field of web development, the need for speed is paramount. By implementing the strategies outlined above, not only will your application function more effectively, but it will also provide a superior user experience. If expertise is required, consider collaborating with a reputable web design agency in Mumbai, such as Prateeksha Web Design. Their proficiency encompasses various domains, including eCommerce website development in Mumbai and tailored solutions from skilled website developers.
Are you ready to refine and elevate your Laravel skills? Make performance optimization a priority, and you will be amazed at how much faster and smoother your application can become.
Lasting Encouragement
In the world of technology, every small enhancement can lead to meaningful improvement. Keep exploring, experimenting, and pushing the limits of your knowledge in Laravel development. If you find yourself facing challenges, don’t hesitate to reach out to experts in the field. Happy coding, and may your development journey be swift and rewarding! 🚀
Tip:
Utilize Caching Effectively: Leverage Laravel's built-in caching mechanisms by utilizing drivers like Redis or Memcached for your configuration, routes, views, and query results. This reduces database load and speeds up your application by serving frequently requested data from memory instead of hitting the database each time.
Fact:
Eager Loading vs. Lazy Loading: When working with relationships in Laravel using Eloquent, remember that eager loading can significantly improve performance by minimizing the "N+1 query problem." By loading all necessary data in a single query instead of querying the database multiple times, your application can execute faster and handle more requests efficiently.
Warning:
Avoid Overusing Middleware: While middleware provides a powerful way to filter HTTP requests entering your application, overusing them can lead to performance bottlenecks. Each middleware adds to the processing time of requests, so it’s important to use them judiciously and only when necessary to avoid slowing down your application.
About Prateeksha Web Design
Prateeksha Web Design offers expert Laravel performance optimization services aimed at enhancing application speed and efficiency. Our team analyzes code structure, implements caching strategies, and optimizes database queries to reduce load times. We focus on improving asset management and leveraging Laravel's built-in features for maximum performance. With tailored solutions, we ensure your application runs smoothly under heavy traffic. Trust us to boost your Laravel application's responsiveness and user experience.
Interested in learning more? Contact us today.
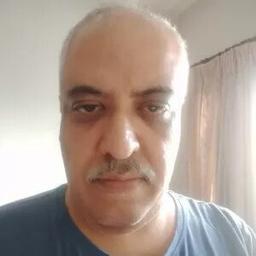