Node.js has transformed how developers build scalable and efficient web applications. Its event-driven, non-blocking I/O model makes it perfect for applications requiring high throughput. However, even the best tools have challenges. One common but critical issue in Node.js applications is memory leaks. This comprehensive guide will help you understand, detect, and prevent memory leaks while showcasing how Prateeksha Web Design can provide expert solutions to your Node.js challenges.
What is a Memory Leak?
A memory leak is a type of software bug that occurs when an application fails to release memory that is no longer needed. Memory is a finite resource, and applications must manage it efficiently. If your application allocates memory for an object but does not release it when it’s no longer in use, the memory remains occupied, even though it serves no purpose. This results in high memory usage over time, which can degrade performance and, in extreme cases, cause application crashes.
Memory Management in Node.js
Node.js uses the V8 JavaScript engine, which manages memory automatically through garbage collection (GC). The garbage collector identifies objects that are no longer reachable from the root of the application and releases the memory allocated to them.
However, some coding patterns and practices in Node.js can inadvertently keep references to unused objects, preventing the garbage collector from reclaiming their memory. These unresolved references lead to memory leaks.
Why Do Memory Leaks Matter?
Memory leaks may seem trivial initially but can significantly affect your application's performance and reliability. Here’s why they’re critical:
-
Cause High Memory Usage
As memory leaks accumulate, the application’s memory consumption grows. This can cause the process memory usage to reach the system's limit, especially in long-running Node.js applications like servers. -
Impact Performance
High memory usage forces the garbage collector to work harder and more frequently, which slows down the application. This latency can negatively affect user experience. -
Crash Applications
When memory consumption exceeds the allowable limits, Node.js applications may throw an out-of-memory (OOM) error. This disrupts services, which is particularly harmful for applications requiring high availability.
Common Causes of Memory Leaks in Node.js
Memory leaks often result from seemingly small mistakes. Here are the common causes:
-
Global Variables
Global variables persist for the application's entire lifetime because they’re always accessible. If you declare a variable withoutlet
,const
, orvar
(e.g.,x = 10
), it becomes a global variable. This can unintentionally occupy memory indefinitely.function createLeak() { leakyVariable = []; } createLeak(); // leakyVariable is now a global variable.
-
Event Listeners
Node.js heavily uses events for asynchronous programming. However, if event listeners are not removed after they’re no longer needed, they can accumulate and consume memory.const emitter = new EventEmitter(); function handler() { console.log('Event handled'); } emitter.on('event', handler); // If not removed, this listener stays in memory.
-
Closures
Closures capture variables from their enclosing scopes. If a closure inadvertently retains references to large objects, the garbage collector cannot release that memory.function createClosure() { const largeArray = new Array(1000).fill('data'); return () => console.log(largeArray.length); } const closure = createClosure(); // largeArray is retained due to the closure.
-
Caching
Over-caching or improper cache management can bloat memory. For example, using a simple in-memory cache like an object orMap
without clearing stale data can lead to memory exhaustion.const cache = new Map(); function addToCache(key, value) { cache.set(key, value); }
-
Timers
Forgetting to clearsetTimeout
orsetInterval
can result in orphaned memory, especially in applications with dynamic lifecycles.const interval = setInterval(() => { console.log('Running...'); }, 1000); // If not cleared, the interval remains in memory.
-
Third-Party Libraries
Libraries with inefficient memory management or improper cleanup mechanisms can introduce memory leaks. Always vet and monitor dependencies for known issues.
How Garbage Collection Works in Node.js
Garbage collection in Node.js uses the V8 JavaScript engine to manage memory. It divides memory into:
- JavaScript Heap: Stores objects and functions. The heap is where most memory leaks occur.
- Call Stack: Tracks execution contexts of running code.
- C++ Objects: For managing native bindings.
V8 employs a mark-and-sweep algorithm to identify unused objects. However, memory leaks can occur when references to unused objects remain, preventing their removal.
How to Detect Memory Leaks in Node.js
Detecting memory leaks requires a systematic approach and the right tools. Let’s explore some key strategies:
1. Inspect Node Memory Usage
Monitoring memory usage is crucial. Use the process.memoryUsage()
method to track memory consumption in real time.
console.log(process.memoryUsage());
2. Use Node.js Debugging Tools
Node.js provides built-in debugging tools to detect memory leaks. Use the --inspect
flag to debug your application:
node --inspect yourApp.js
Then, open chrome://inspect
in Google Chrome to access the Node.js debugging interface.
3. Profile Memory Usage
Memory profiling tools like Chrome DevTools and built-in Node.js features can help visualize memory allocation over time. Take heap snapshots to locate memory leaks in JavaScript.
4. Leverage Third-Party Monitoring Tools
Tools like NewRelic Node.js and DataDog Node.js provide advanced memory monitoring and analysis. They offer detailed insights into application performance and help pinpoint memory issues.
5. Enable Garbage Collection Logs
Run your application with the --trace-gc
flag to monitor garbage collection activity:
node --trace-gc yourApp.js
6. Use a Memory Leak Detector
A memory leak detector like leakage
or memwatch-next
can identify problematic patterns in your code. These tools help automate the detection process.
How to Check for Memory Leaks: Step-by-Step Guide
Detecting and diagnosing memory leaks is an essential part of ensuring the stability and performance of a Node.js application. Here's a detailed breakdown of the steps to identify memory leaks effectively:
1. Monitor Memory Growth
The first step is to monitor the application’s memory usage over time to identify any abnormal growth patterns.
- How to Monitor: Use the built-in
process.memoryUsage()
method in Node.js. This provides a snapshot of memory usage, including:rss
: Resident Set Size (total memory allocated by the process).heapTotal
: Total memory allocated for the JavaScript heap.heapUsed
: Memory currently in use in the heap.external
: Memory used by C++ objects bound to JavaScript objects.
setInterval(() => {
console.log(process.memoryUsage());
}, 5000);
- What to Look For: If
heapUsed
orrss
keeps growing consistently without stabilizing, it could indicate a memory leak.
2. Profile Memory with Chrome DevTools
Chrome DevTools offers robust tools for memory profiling and analysis.
-
Step 1: Run the App with
--inspect
Launch your Node.js application with the--inspect
flag to enable debugging:node --inspect app.js
-
Step 2: Open Chrome DevTools Open Chrome and navigate to
chrome://inspect
. Click on your application under “Remote Target” to open the DevTools interface. -
Step 3: Record a Heap Snapshot
- Go to the “Memory” tab in DevTools.
- Select “Heap Snapshot.”
- Click on “Take Snapshot.”
-
Step 4: Compare Snapshots Over Time Take multiple snapshots at different intervals or after significant application activity. Compare these snapshots to identify growing memory regions, indicating objects retained in memory unnecessarily.
3. Test for Retained Objects
The Retainers Panel in Chrome DevTools helps track objects that should have been garbage-collected but are still retained due to references.
-
How to Use Retainers:
- Open the snapshot you captured in the “Memory” tab.
- Look for objects with unexpectedly long lifetimes.
- Use the Retainers view to identify what is holding the reference.
-
What to Fix: Analyze why those objects are still being referenced and update the code to remove unnecessary references.
Example:
function createLeak() {
const largeArray = new Array(10000).fill('data');
return () => console.log(largeArray);
}
const closure = createLeak();
// If not handled, largeArray stays in memory due to the closure.
4. Check Event Listeners
Excessive or improperly removed event listeners are a common cause of memory leaks in Node.js.
- Monitor Event Listeners Growth:
Use the
EventEmitter
’slistenerCount()
method to check how many listeners are attached to a specific event.
const EventEmitter = require('events');
const emitter = new EventEmitter();
console.log(emitter.listenerCount('eventName'));
- Detect Excessive Listeners:
Use the
process.on('warning', ...)
handler to catch warnings related to the number of listeners exceeding the default limit (10).
process.on('warning', (warning) => {
console.warn(warning.name, warning.message, warning.stack);
});
- Fix Issues:
Remove unnecessary listeners using
emitter.removeListener()
oremitter.off()
.
5. Run Memory Leakage Tests
Simulating real-world load on your application helps identify how memory behaves under stress.
-
How to Simulate Heavy Traffic: Use tools like Apache Benchmark (
ab
), Artillery, or JMeter to send concurrent requests to your application.ab -n 1000 -c 100 http://localhost:3000/
-
Monitor Memory During Load: Use tools like
top
orhtop
to observe memory usage trends for the Node.js process. Alternatively, logprocess.memoryUsage()
at regular intervals. -
Analyze Results:
- Look for a steady increase in memory usage during the test.
- If memory does not stabilize after the load subsides, it’s a sign of a memory leak.
How to Prevent Memory Leaks
Prevention is always better than cure. Here’s how you can manage memory leaks effectively:
1. Follow Best Practices
- Avoid global variables.
- Use weak references for caches.
- Clear unused timers and intervals.
- Remove event listeners after use.
2. Use Proper Tools
- Regularly monitor memory with tools like NewRelic Node.js and DataDog Node.js.
- Incorporate memory leak detection in CI/CD pipelines.
3. Code Reviews and Testing
- Implement memory leakage testing during development.
- Conduct regular code reviews to spot potential leaks.
4. Optimize Third-Party Libraries
Be cautious when integrating third-party packages. Regularly update and review dependencies for performance issues.
Practical Example: Debug Node.js High Memory Usage
Debugging high memory usage in a Node.js application involves systematically identifying the source of the issue and implementing solutions to mitigate it. Here's a detailed walkthrough:
1. Start the App with Inspect Mode
Node.js provides an inspect mode that enables debugging tools, including heap snapshots and performance profiling.
- Command to Start Inspect Mode:
node --inspect app.js
- What It Does:
- Launches your application with the debugging interface.
- Outputs a debugging URL, such as
ws://127.0.0.1:9229/xxxx
, which can be opened in Chrome DevTools.
2. Open Chrome DevTools and Take a Heap Snapshot
Chrome DevTools is an invaluable tool for analyzing memory usage and identifying potential leaks.
-
Steps to Access DevTools:
- Open Chrome and navigate to
chrome://inspect
. - Under “Remote Target,” click “Inspect” for your Node.js application.
- Open Chrome and navigate to
-
Steps to Take a Heap Snapshot:
- Go to the “Memory” tab in DevTools.
- Select “Heap Snapshot.”
- Click on “Take Snapshot.”
-
Purpose of the Snapshot:
- Captures the state of memory allocation in the application.
- Helps identify objects retained in memory unnecessarily.
3. Simulate Load
To observe how the application handles memory under stress, simulate load using tools like Apache Benchmark (ab) or Artillery.
- Apache Benchmark Example:
ab -n 1000 -c 100 http://localhost:3000/
-
-n
: Total number of requests to make. -
-c
: Number of concurrent requests. -
What to Monitor:
- Look for unusual memory spikes in the application during load testing.
- Use
process.memoryUsage()
to log memory statistics in real time.
4. Analyze Snapshots
Once the load test is complete, take another heap snapshot and compare it with the previous one to identify changes in memory allocation.
-
Steps to Compare Snapshots:
- Open the “Memory” tab in DevTools.
- Load and compare the before and after snapshots.
- Focus on objects that persist between snapshots but should have been garbage-collected.
-
What to Look For:
- Objects with increasing counts or sizes.
- Retained objects with no apparent references in the code.
5. Fix the Leak
Once you’ve identified the root cause of the high memory usage, implement fixes in the code.
-
Common Fixes:
- Clear Unused Variables: Ensure variables no longer needed are dereferenced.
- Remove Event Listeners: Use
removeListener
oroff
to clean up listeners. - Manage Closures: Avoid unnecessary closures that retain references to large objects.
- Optimize Caches: Use time-based or size-based eviction for in-memory caches.
- Clear Timers: Use
clearTimeout
orclearInterval
to remove inactive timers.
-
Example Fix for Event Listeners:
const emitter = new EventEmitter();
function handleEvent() {
console.log('Event occurred');
}
emitter.on('event', handleEvent);
// Remove listener when it's no longer needed
emitter.removeListener('event', handleEvent);
Real-World Tools to Manage Memory Leaks
Chrome DevTools
-
Why Use It:
- Provides visual tools to analyze memory usage and performance bottlenecks.
- Allows you to capture and compare heap snapshots.
-
Key Features:
- Retainers panel to identify references keeping objects in memory.
- Real-time memory profiling during application runtime.
Node.js Profiler
-
What It Does:
- Tracks function calls and memory allocation to identify performance bottlenecks.
- Generates CPU and memory profiles for detailed analysis.
-
How to Use:
node --prof app.js
- Analyze Results:
- Use tools like
speedscope
ornode-tick-processor
to interpret profiling data.
- Use tools like
NewRelic and DataDog
For production-grade memory leak detection, NewRelic Node.js and DataDog Node.js offer powerful monitoring capabilities.
-
NewRelic Node.js:
- Tracks memory usage trends over time.
- Provides alerts for unusual memory behavior.
-
DataDog Node.js:
- Offers detailed dashboards for process memory usage.
- Allows tracking of specific functions or modules responsible for high memory consumption.
Why Choose Prateeksha Web Design?
Prateeksha Web Design specializes in creating high-performance Node.js applications while ensuring top-notch debugging and prevention strategies for issues like JavaScript memory leaks. Our team:
- Conducts thorough code reviews to check for memory leaks.
- Implements automated memory leakage testing.
- Monitors production apps for high memory usage.
- Optimizes third-party libraries to reduce Node.js garbage collection issues.
With our expertise, your application will be efficient, scalable, and reliable.
Conclusion
Memory leaks in Node.js can be daunting, but with the right tools and strategies, they’re manageable. By understanding how to find memory leaks, leveraging tools like memory leak detectors, and following best practices, you can ensure your application runs smoothly.
For expert assistance with debugging, optimizing, or developing Node.js applications, reach out to Prateeksha Web Design—your trusted partner in crafting robust and efficient software solutions.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services in identifying and resolving Node.js memory leaks, providing a comprehensive guide to debugging techniques and best practices. Their expert team leverages advanced tools to analyze memory usage, optimizing applications for performance and efficiency. They emphasize proactive prevention strategies to enhance application stability and user experience. With tailored solutions, Prateeksha ensures clients can scale their Node.js applications without memory-related bottlenecks. Trust their expertise to safeguard your web projects against costly memory leaks.
Interested in learning more? Contact us today.
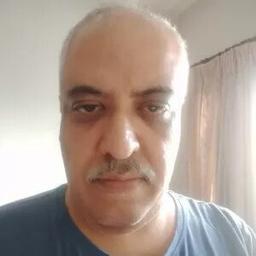