How To Identify And Fix Node.js Memory Leaks: A Complete Guide for 2025
Node.js is a game-changer for building scalable and efficient backend applications, but even the best developers occasionally face challenges like memory leaks. Memory leaks in Node.js can slow down your application, increase costs, and create a poor user experience if left unaddressed. In this blog, we’ll dive deep into identifying and fixing memory leaks in Node.js, simplifying the process so it’s easy to follow—even if you’re new to backend optimization.
At Prateeksha Web Design, we specialize in creating seamless, high-performance backend solutions for businesses of all sizes. Debugging tools, backend optimization, and memory management are part of our daily toolkit, and we’re here to help you tackle memory leaks head-on.
What Are Node.js Memory Leaks?
To grasp the concept of memory leaks in Node.js, let’s first understand how memory works in Node.js applications.
Dynamic Memory Allocation in Node.js
Node.js, like many programming environments, uses dynamic memory allocation. This means the memory required for variables, objects, and functions is allocated at runtime. Once the data is no longer needed, the garbage collector (GC) is responsible for deallocating or "freeing" the memory, making it available for reuse.
When Memory Leaks Happen
A memory leak occurs when the application allocates memory but fails to release it after it’s no longer required. This happens because the garbage collector cannot reclaim the memory. This leftover memory remains “in use” from the system's perspective, even though the application doesn’t need it.
For instance:
let cache = {};
function addData(key, value) {
cache[key] = value; // Data added to the cache
}
addData('data1', 'value1'); // Memory is allocated
// If `cache` is never cleared, memory remains occupied unnecessarily.
Impact of Memory Leaks
Over time, these unreleased chunks of memory accumulate, leading to:
- Increased memory consumption.
- Slower application performance as resources become strained.
- Potential crashes when the system runs out of memory.
Why Do Node.js Memory Leaks Matter?
Memory leaks may start small, but their effects grow over time, especially in long-running applications. Here's a closer look at why they are a critical issue:
1. Performance Drops
Every application has access to a limited amount of memory. When memory leaks occur, this limited space fills up unnecessarily. The garbage collector must work harder and more frequently to clean up memory, causing additional CPU load and latency. This results in slower response times and diminished performance for users.
For example:
- A real-time chat application may experience delayed message delivery as the server struggles with memory pressure.
- An e-commerce app may take longer to process transactions, frustrating users.
2. Higher Costs
In cloud-hosted environments like AWS, Google Cloud, or Azure, your application’s resource consumption directly affects your costs. Memory leaks inflate resource usage, leading to increased charges for memory allocation. This inefficiency not only drains system resources but also drains your budget.
3. Poor User Experience
A sluggish or unresponsive application is a surefire way to frustrate users. Memory leaks can lead to crashes, forcing users to reload pages, restart applications, or, worse, abandon your app altogether. In an age where users expect seamless experiences, even minor disruptions can hurt your brand’s reputation.
4. Scalability Issues
A memory-leaking application becomes challenging to scale. With each new user or feature, the app consumes additional resources, compounding the problem. This creates a bottleneck that can prevent your application from serving a growing user base effectively.
Why Understanding Memory Leaks Is Essential
Understanding memory leaks isn’t just about fixing bugs—it’s about ensuring your application runs smoothly, efficiently, and at scale. Whether you’re building a startup app or managing a large enterprise application, proactive memory management is critical.
At Prateeksha Web Design, we prioritize backend optimization in all our projects. By identifying and resolving memory leaks early, we help businesses deliver faster, more reliable applications while keeping costs in check.
Common Causes of Memory Leaks in Node.js
Memory leaks in Node.js often stem from subtle coding mistakes that go unnoticed during development. Let’s break down the most common culprits so you can spot and address them before they escalate.
1. Global Variables
Global variables are variables accessible throughout the application’s lifecycle. While they’re convenient, they pose a significant risk if mismanaged.
When variables are declared without let
, const
, or var
, they default to global scope. This makes them persist in memory for the duration of the application, even if they’re no longer needed.
Example:
function addData() {
globalData = 'This is a global variable'; // Declared without let/const/var
}
addData();
// globalData remains in memory indefinitely
How It Causes a Memory Leak: Global variables cannot be garbage-collected because they are always referenced in the global scope. This leads to memory accumulation over time.
Solution:
Use let
or const
to define variables within a specific scope:
function addData() {
let localData = 'This is scoped to the function';
}
2. Uncleared Timers
Timers like setInterval
and setTimeout
are often used for repeated tasks or delayed execution. If these timers aren’t cleared when no longer needed, they hold references to the callback functions, preventing the memory they occupy from being released.
Example:
const timer = setInterval(() => {
console.log('Running...');
}, 1000);
// If this timer is not cleared, it will persist indefinitely
How It Causes a Memory Leak: The timer continues to hold references to the function and its variables, even if the function is no longer needed.
Solution: Clear timers when they’re no longer required:
clearInterval(timer);
3. Closures
Closures are a powerful feature in JavaScript, but they can lead to memory leaks if not used carefully. A closure occurs when an inner function remembers variables from its outer function, even after the outer function has finished execution.
Example:
function createClosure() {
const largeObject = { key: 'value' };
return function () {
console.log(largeObject);
};
}
const closure = createClosure();
// The largeObject remains in memory because the closure references it
How It Causes a Memory Leak: The closure retains a reference to the outer function’s variables, preventing them from being garbage-collected.
Solution: Avoid unnecessary closures or ensure variables are not retained unnecessarily:
function createClosure() {
const largeObject = { key: 'value' };
return function () {
console.log('Closure executed without retaining largeObject');
};
}
4. Event Listeners
Event listeners are crucial for handling user interactions or system events. However, failing to remove listeners when they’re no longer needed keeps references alive, leading to memory leaks.
Example:
const EventEmitter = require('events');
const emitter = new EventEmitter();
function handleEvent(data) {
console.log('Event received:', data);
}
emitter.on('event', handleEvent);
// If this listener is not removed, it persists indefinitely
How It Causes a Memory Leak: The event emitter retains references to all active listeners, preventing them from being garbage-collected.
Solution:
Remove unused listeners using removeListener
or removeAllListeners
:
emitter.removeListener('event', handleEvent);
5. Caching Issues
Caching can improve performance by storing frequently accessed data in memory. However, excessive or poorly managed caching can cause memory leaks if old or unnecessary data is not removed.
Example:
const cache = {};
function addToCache(key, value) {
cache[key] = value;
}
// Old cache entries may remain indefinitely if not removed
How It Causes a Memory Leak: Over time, the cache grows uncontrollably, consuming more and more memory.
Solution: Use caching libraries like lru-cache to limit the size of the cache and automatically evict old entries:
const LRU = require('lru-cache');
const cache = new LRU({ max: 100 }); // Maximum of 100 items
cache.set('key', 'value');
How To Identify Memory Leaks in Node.js
Detecting memory leaks is the first step toward resolving them. Here’s a step-by-step guide:
1. Use Monitoring Tools
Monitoring your application’s memory usage is essential. Tools like PM2 and New Relic provide real-time insights into your app’s resource consumption.
2. Profile the Memory
Node.js has built-in profiling tools to track memory usage:
- Use the
--inspect
flag to open the Chrome DevTools debugger. - In Chrome, navigate to
chrome://inspect
and connect to your Node.js app. - Use the Heap Snapshot feature to analyze memory usage over time.
3. Analyze Garbage Collection
Garbage collection (GC) in Node.js helps reclaim unused memory. Use the --trace-gc
flag to observe how often GC runs and how much memory it’s recovering.
4. Identify Retained Objects
Retained objects are the primary cause of memory leaks. Use tools like Heapdump or v8-profiler-next to capture memory snapshots and pinpoint objects that persist longer than expected.
5. Log Memory Usage
Log memory usage periodically using process.memoryUsage()
. For example:
setInterval(() => {
console.log(process.memoryUsage());
}, 10000);
How To Fix Node.js Memory Leaks
Once you’ve identified memory leaks, it’s time to fix them. Follow these practical steps:
1. Eliminate Unnecessary Global Variables
Use strict mode ('use strict'
) to catch undeclared variables:
'use strict';
function example() {
undeclaredVar = 'This will throw an error in strict mode';
}
example();
2. Clear Timers and Intervals
Always clear timers when they’re no longer needed:
const timer = setInterval(() => {
console.log('Running...');
}, 1000);
setTimeout(() => {
clearInterval(timer);
}, 5000);
3. Remove Unused Event Listeners
Use removeListener
or removeAllListeners
to prevent listeners from piling up:
const EventEmitter = require('events');
const emitter = new EventEmitter();
function onEvent() {
console.log('Event occurred');
}
emitter.on('event', onEvent);
// Remove listener
emitter.removeListener('event', onEvent);
4. Optimize Caching
Use libraries like lru-cache to manage memory limits in your caching strategy:
const LRU = require('lru-cache');
const cache = new LRU({ max: 100 });
cache.set('key', 'value');
console.log(cache.get('key'));
5. Fix Retained Objects
Analyze heap snapshots and refactor code to avoid unnecessary object retention:
let cachedObject = null;
function createObject() {
cachedObject = { data: 'Heavy data' }; // Retained in memory
}
createObject();
cachedObject = null; // Release memory
Best Practices for Preventing Node.js Memory Leaks
Preventing memory leaks in Node.js starts with writing efficient and mindful code. Implementing the following best practices will keep your application leak-free and ensure it runs smoothly.
1. Limit Scope
Minimizing the scope of variables helps prevent unintended references from persisting in memory. Variables declared in the global scope or within closures can remain accessible longer than needed, leading to memory leaks.
Example of Poor Practice:
let globalVar = 'I persist forever!';
function setGlobalVar(value) {
globalVar = value;
}
Best Practice:
Keep variables localized and use block-scoped declarations (let
and const
) wherever possible:
function processRequest(input) {
const localVar = input;
console.log(localVar); // Released after the function ends
}
2. Use WeakMap and WeakSet
WeakMap and WeakSet are memory-efficient data structures because they allow garbage collection for objects that are no longer referenced elsewhere. Unlike regular maps or sets, they do not prevent objects from being reclaimed.
Example:
const weakMap = new WeakMap();
let key = {};
weakMap.set(key, 'value');
key = null; // Memory associated with `key` is released
When to Use:
- When you need to associate metadata with objects.
- When you don’t want to affect the lifecycle of the object.
3. Audit Dependencies
Dependencies can introduce memory leaks, especially if you’re using outdated or poorly maintained packages. Third-party libraries might have bugs or retain references unnecessarily.
Best Practices:
- Regularly update dependencies to their latest stable versions.
- Audit packages using tools like npm audit or Snyk.
- Replace inefficient libraries with well-maintained alternatives.
4. Use a Monitoring Dashboard
Monitoring tools like Kibana, Grafana, and New Relic provide real-time insights into memory usage, CPU load, and performance trends. These dashboards help you identify abnormal memory growth before it impacts users.
Why It Helps:
- Tracks memory over time.
- Provides visualizations of garbage collection behavior.
- Alerts you when memory thresholds are exceeded.
5. Test in Staging
Staging environments mimic production conditions and allow you to catch memory leaks before deployment. Stress-testing your app under load ensures you identify leaks that might not occur during development.
How to Test:
- Use load-testing tools like Apache JMeter or k6.
- Simulate high-traffic scenarios to observe memory patterns.
- Monitor resource usage over extended periods.
Debugging Tools for Node.js Memory Leaks
When memory leaks occur, debugging tools can help you identify and resolve the issue efficiently. Here’s a look at some of the most effective tools:
1. Chrome DevTools
Chrome DevTools includes a Node.js debugger that supports heap profiling, allocation tracking, and memory analysis.
How to Use:
- Start your Node.js application with the
--inspect
flag:node --inspect app.js
- Open Chrome and navigate to
chrome://inspect
. - Attach the debugger to your application.
- Use the Heap Snapshot feature to analyze memory usage.
2. Heapdump
Heapdump generates memory snapshots that you can analyze to identify retained objects and memory usage patterns.
How to Use:
- Install Heapdump:
npm install heapdump
- Trigger a snapshot programmatically:
const heapdump = require('heapdump'); heapdump.writeSnapshot('./heapdump.heapsnapshot');
- Load the snapshot in Chrome DevTools for analysis.
3. clinic.js
Clinic.js is a suite of tools designed to diagnose performance and memory issues in Node.js applications.
Features:
- Clinic Doctor: Diagnoses common performance problems.
- Clinic Flame: Visualizes CPU bottlenecks using flame graphs.
- Clinic Heap: Identifies memory leaks and analyzes heap snapshots.
How to Use:
- Install clinic.js:
npm install -g clinic
- Run your application through Clinic:
clinic doctor -- node app.js
4. PM2
PM2 is a process manager that includes built-in monitoring and memory tracking for Node.js applications.
Key Features:
- Tracks memory usage over time.
- Provides automated restarts if memory thresholds are exceeded.
- Integrates with monitoring dashboards for detailed insights.
How to Use:
- Install PM2:
npm install pm2 -g
- Start your application with monitoring:
pm2 start app.js --watch
5. N|Solid
N|Solid is an enterprise-grade performance monitoring tool for Node.js. It offers advanced insights into memory leaks, CPU usage, and application performance.
Key Benefits:
- Identifies memory leaks and performance bottlenecks in real time.
- Provides actionable diagnostics for Node.js applications.
- Designed for production environments.
How to Use:
- Sign up for N|Solid and integrate it into your application.
- Use its dashboard to track memory and CPU usage trends.
Real-World Example: Fixing a Memory Leak
Let’s walk through an example. Suppose you have a chat application where users report performance issues.
Problem
Memory usage grows over time, even when users leave the chat.
Diagnosis
After profiling, you discover:
- Event listeners for
message
aren’t removed when users disconnect. - Cached user objects aren’t cleared.
Solution
- Remove Listeners
io.on('connection', (socket) => {
const onMessage = (msg) => console.log(msg);
socket.on('message', onMessage);
socket.on('disconnect', () => {
socket.removeListener('message', onMessage);
});
});
- Clear Cache
const userCache = new Map();
function addUser(userId, userData) {
userCache.set(userId, userData);
}
function removeUser(userId) {
userCache.delete(userId);
}
The Role of Backend Optimization in Node.js
Efficient memory management is a crucial part of backend optimization. By proactively monitoring and managing memory, you can:
- Improve application performance.
- Enhance user satisfaction.
- Reduce operational costs.
At Prateeksha Web Design, our expertise in backend optimization ensures your Node.js applications are robust, scalable, and future-ready.
Final Thoughts
Node.js memory leaks can feel daunting, but with the right tools and techniques, you can identify and resolve them efficiently. By adopting best practices, leveraging debugging tools, and optimizing your code, you’ll ensure your app performs smoothly—even under heavy loads.
If you need expert help optimizing your Node.js backend, Prateeksha Web Design is here for you. We combine years of experience with cutting-edge tools to deliver high-performance applications tailored to your business needs.
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services for identifying and fixing Node.js memory leaks. Our expert team utilizes advanced profiling tools to detect memory consumption patterns and pinpoint leaks. We implement effective strategies to optimize memory usage and enhance application performance. Additionally, we provide detailed reporting and recommendations for long-term prevention. Trust us to ensure your Node.js applications run smoothly and efficiently.
Interested in learning more? Contact us today.
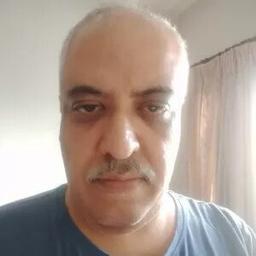