Stepping Into Next.js Development with TypeScript
When you embark on Next.js development, especially with TypeScript in your toolkit, you’re not just building web applications—you’re preparing to craft fast, modern, and scalable solutions that stand out in today’s tech-driven world. Let’s unpack what makes this such an exciting adventure.
The Spacecraft Analogy: Why Next.js + TypeScript Feels Like a Spaceship
Picture this: Next.js is your spaceship, designed for speed and precision, while TypeScript is your navigation system, ensuring you steer clear of dangers (like runtime errors). Together, they provide a robust environment where you can confidently explore and conquer the vast galaxy of web development possibilities.
But before you can fly, you need a launchpad—your development environment. This includes the tools, configurations, and mindset required to make the most of these powerful technologies.
Why TypeScript and Next.js Are the Dream Team
JavaScript: The Trusty Old Bicycle
JavaScript is the backbone of web development. It’s been around for ages, and most developers are comfortable with it. However, like an old bicycle:
- It’s reliable for basic needs but prone to wobbles (errors) if you’re not careful.
- Errors only show up during runtime, which can cause unexpected crashes and frustration.
TypeScript: The Smart, Stable Upgrade
TypeScript builds on JavaScript, adding features like:
- Type safety: It forces you to define what kind of data your code expects (e.g., strings, numbers, objects). This reduces bugs and makes your code more predictable.
- Error catching during development: Think of TypeScript as a grammar checker for your code—it flags mistakes before they cause issues.
- Improved readability and maintainability: With TypeScript, other developers (or your future self) can easily understand what your code is supposed to do.
Using TypeScript is like upgrading your bike with stabilizers, a GPS, and even an anti-theft alarm. It ensures a smooth and safe ride, no matter the terrain.
Next.js: The Turbocharged Framework
Next.js takes React, a popular library for building user interfaces, and turns it into a full-fledged framework. It adds features like:
- Server-side rendering (SSR): Pages are rendered on the server before being sent to the browser, improving load times and SEO.
- Static site generation (SSG): Content is pre-generated and served as static files, making your app lightning-fast.
- API routes: Build serverless APIs directly within your app, simplifying backend integration.
- Optimized performance: Built-in optimizations like image handling and code splitting make your app incredibly efficient.
When paired with TypeScript, these features become even more powerful because you can ensure your code is error-free and scalable from the start.
The Benefits of Combining Next.js and TypeScript
-
Catching Bugs Early TypeScript’s static type checking identifies errors during the development phase, rather than letting them cause crashes in production. For instance:
const add = (a: number, b: number) => a + b; add(5, 'hello'); // TypeScript throws an error here!
-
Improved Developer Productivity Autocomplete, intelligent code navigation, and better error messages make TypeScript a dream for developers. Combine this with Next.js’s hot reloading and fast builds, and you’ll be building apps in record time.
-
SEO Superpowers Next.js’s SSR and SSG ensure search engines can crawl your site effectively. For example, a blog post can be pre-rendered and loaded instantly, making it more likely to rank higher in search results.
-
Scalability As your application grows, managing types with TypeScript ensures the code remains clean and maintainable. This is crucial for scaling projects without losing control.
-
Enhanced Collaboration When working in teams, TypeScript’s type definitions act as documentation. They make it easier for developers to understand and work with each other’s code.
Step 1: Setting the Stage—Install Next.js with TypeScript
Breaking It Down
To kick off your journey into Next.js development with TypeScript, the very first step is setting up your project. This involves using the create-next-app
CLI tool, which makes initializing a new Next.js project as simple as pie.
Here’s the command to get started:
npx create-next-app@latest my-nextjs-typescript-app --typescript
What Does This Command Do?
-
npx
:
This utility comes with Node.js. It runs thecreate-next-app
tool directly from the npm registry, ensuring you’re using the most up-to-date version every time. No outdated dependencies here—just fresh, shiny code. -
create-next-app
:
This is Next.js’s official scaffolding tool. It sets up a boilerplate project with all the essential files, directories, and dependencies needed for a Next.js application. -
--typescript
:
This flag ensures TypeScript is included during setup. You won’t have to manually install and configure it—it’s already baked into the project. Think of it as pre-ordering your favorite pizza with every topping you love. No extra steps required!
The Output
After running the command, your terminal will generate a folder structure like this:
my-nextjs-typescript-app/
├── node_modules/
├── public/
├── styles/
├── pages/
│ ├── _app.tsx
│ ├── index.tsx
├── tsconfig.json
├── package.json
├── next.config.js
You’re now armed with a TypeScript-ready Next.js app. 🎉 Easy, right?
Step 2: Dive Into the TypeScript Setup
What is tsconfig.json
?
The tsconfig.json
file is TypeScript’s configuration file. It tells the TypeScript compiler how to process your code, enabling features like type checking, module resolution, and more.
Open it up, and you’ll see something like this:
{
"compilerOptions": {
"target": "es5",
"lib": ["dom", "dom.iterable", "esnext"],
"allowJs": true,
"skipLibCheck": true,
"strict": true,
"forceConsistentCasingInFileNames": true,
"noEmit": true,
"esModuleInterop": true,
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
"jsx": "preserve"
},
"include": ["next-env.d.ts", "**/*.ts", "**/*.tsx"],
"exclude": ["node_modules"]
}
This might look intimidating, but let’s break it down into digestible chunks.
Key Settings in tsconfig.json
:
-
"strict": true
:
This activates TypeScript's strict mode, which enforces stricter type checks. It’s like having a tough-but-fair teacher who makes sure you don’t slack off. -
"baseUrl": "."
and"paths": {}
:
These settings let you define custom import paths. Instead of using relative imports like../../../utils
, you can simplify it to@/utils
. Cleaner imports = happier developers. -
"jsx": "preserve"
:
This ensures that JSX (React’s syntax) remains unchanged during compilation, letting Next.js handle it. Think of this as letting the expert (Next.js) do the heavy lifting.
Customizing Your Setup
While the default tsconfig.json
works perfectly fine, you might want to tweak it based on your project’s requirements. Here’s an example of a more customized setup:
{
"compilerOptions": {
"target": "es6", // Target ECMAScript 2015 or higher
"module": "esnext", // Use modern ES modules
"allowJs": true, // Allow JavaScript files
"jsx": "preserve", // Keep JSX as-is for Next.js
"moduleResolution": "node", // Resolve modules like Node.js does
"strictNullChecks": true, // Prevent null/undefined mishaps
"baseUrl": ".", // Enable cleaner imports
"paths": { // Define custom aliases
"@components/*": ["components/*"],
"@utils/*": ["utils/*"]
}
}
}
What’s the Benefit?
-
Future-Proofing Your App:
By settingtarget
toes6
or higher, you ensure your app stays compatible with modern JavaScript features. -
Cleaner Codebase:
Custom paths reduce the mess of relative imports, making your code easier to read and maintain. -
Strict Type Checking:
EnablingstrictNullChecks
prevents annoying runtime errors caused by unexpected null or undefined values.
Step 3: Create Your First TypeScript Component
Creating your first TypeScript component in Next.js is an exciting milestone. It’s where the theoretical understanding starts to materialize into tangible, functional code.
Replace the Default index.js
with index.tsx
Navigate to the pages/
folder in your project, and replace the default index.js
file with a new file named index.tsx
. Here’s an example of what the updated code might look like:
import React from "react";
interface GreetingProps {
name: string;
}
const Greeting: React.FC<GreetingProps> = ({ name }) => {
return <h1>Hello, {name}! Welcome to your Next.js TypeScript app!</h1>;
};
export default function Home() {
return <Greeting name="Next.js Developer" />;
}
What’s Happening in This Code?
-
Interface Definition
interface GreetingProps { name: string; }
- This defines the structure of
props
that theGreeting
component expects. - The
name
property is strictly typed as astring
, ensuring no accidental misuse (e.g., passing a number or object).
- This defines the structure of
-
Functional Component with React.FC
const Greeting: React.FC<GreetingProps> = ({ name }) => { ... };
React.FC
explicitly tells TypeScript that this is a functional component.- The
<GreetingProps>
argument ensures the component adheres to the defined interface.
-
Type-Safe Props Usage
<Greeting name="Next.js Developer" />;
- If you forget to provide a
name
prop or pass an incorrect type, TypeScript will flag it before runtime. Think of it as a helpful backseat driver who ensures you don’t take a wrong turn.
- If you forget to provide a
-
Reusable Component
TheGreeting
component is reusable with differentname
values, making your code modular and maintainable.
Step 4: Organizing Your Project Like a Pro
A well-organized project is key to scalability and collaboration. Here’s a suggested folder structure to keep things neat:
/components
/pages
/styles
/utils
/types
What Goes Where?
-
components/
- Place reusable UI components here, such as buttons, headers, and custom widgets.
- Example: A
Button.tsx
file for a styled button.
-
pages/
- Each file in this folder corresponds to a route in your app. For example:
pages/index.tsx
→/
pages/about.tsx
→/about
- Each file in this folder corresponds to a route in your app. For example:
-
styles/
- Store your CSS files here, including global styles and CSS Modules.
- Example:
Home.module.css
for styles specific to the home page.
-
utils/
- Store utility functions like date formatters, API handlers, or helper functions.
- Example:
fetchData.ts
for making API calls.
-
types/
- Centralize all your TypeScript types and interfaces here for easier management.
- Example:
types/GreetingProps.ts
to define props used in multiple components.
This structure keeps everything organized, reducing confusion and improving maintainability.
Step 5: Styling Your App
Styling is where your app comes to life visually. Next.js offers various options for styling, including:
- CSS
- Sass
- CSS-in-JS libraries like Tailwind CSS or Emotion
For this tutorial, let’s stick with CSS Modules—a simple yet powerful way to scope styles locally.
Using CSS Modules
-
Create a CSS File
In thestyles/
folder, create a file namedHome.module.css
:.greeting { color: #0070f3; font-size: 2rem; text-align: center; }
-
Import and Use in the Component
Modify yourHome.tsx
file to include the styles:import styles from './Home.module.css'; export default function Home() { return <h1 className={styles.greeting}>Hello, Next.js with TypeScript!</h1>; }
-
What Makes CSS Modules Special?
- Styles are scoped locally by default. This means
.greeting
won’t clash with a similarly named class in another file. - Think of it as giving each piece of your wardrobe its own dedicated drawer—everything stays organized and conflict-free.
- Styles are scoped locally by default. This means
Step 6: Debugging Like a Detective
Debugging is an essential part of the development process. But don’t worry—with Next.js and TypeScript, you’ll feel like Sherlock Holmes solving mysteries with the help of your trusty magnifying glass (or in this case, your IDE and tools).
1. Next.js DevTools: Your First Clue
Running your app in development mode is the easiest way to catch issues in real time. Here’s how:
- Start your app with:
npm run dev
- Open http://localhost:3000 in your browser.
As you make changes, Next.js automatically reloads the app, letting you see updates instantly. If there’s a problem, it will often show detailed error messages directly in the browser or terminal. It’s like having a crime scene report right in front of you.
2. TypeScript Errors: The Red Squiggly Lines
Those red squiggly lines in VS Code might seem annoying at first, but they’re your biggest allies. They point out:
- Type mismatches: Passing a string when a number is expected.
- Missing props: Forgetting to provide required data to components.
- Syntax errors: Catching typos before they cause bigger issues.
For example:
interface User {
name: string;
age: number;
}
const printUser = (user: User) => {
console.log(`User: ${user.name}, Age: ${user.age}`);
};
// This will trigger a TypeScript error because 'age' is missing
printUser({ name: 'Sherlock' });
The error message will guide you to fix the issue before running the code. Think of TypeScript as your Watson—helping you avoid unnecessary pitfalls.
3. Pro Tip: ESLint and Prettier
Install these VS Code extensions to ensure your code stays clean and consistent:
- ESLint: Highlights potential issues in your code, like unused variables or improper formatting.
- Prettier: Automatically formats your code to look polished and professional.
Here’s how to set them up in your project:
- Install the dependencies:
npm install eslint prettier eslint-config-prettier eslint-plugin-react --save-dev
- Add a
.eslintrc.json
file:{ "extends": ["next/core-web-vitals", "prettier"], "plugins": ["react"] }
With ESLint and Prettier, your code will be cleaner than Sherlock’s overcoat!
Step 7: Go Big with API Routes
One of Next.js’s standout features is the ability to create API routes directly within your project. No need to set up a separate backend—it’s all built in!
Create Your First API Endpoint
Let’s add a simple API route that says hello. In your pages/api/
directory, create a new file called hello.ts
:
import { NextApiRequest, NextApiResponse } from 'next';
export default function handler(req: NextApiRequest, res: NextApiResponse) {
res.status(200).json({ message: 'Hello, API with TypeScript!' });
}
Here’s what’s happening:
- Type Imports:
NextApiRequest
andNextApiResponse
ensure your API handler has the correct types. - Handler Function: The
handler
function takes the request (req
) and response (res
) objects, sending back a JSON response.
Access Your API
Start your app (npm run dev
) and visit http://localhost:3000/api/hello. You’ll see:
{
"message": "Hello, API with TypeScript!"
}
It’s like having a secret tunnel in your spaceship for quick escapes (or data exchanges)!
Step 8: Deploy Like a Boss
Once your app is ready, it’s time to showcase your work to the world. Vercel, the creators of Next.js, make deployment effortless.
Deploying to Vercel
-
Push Your Code to GitHub
Commit and push your project to a GitHub repository. If you’re unfamiliar, use:git init git add . git commit -m "Initial commit" git branch -M main git remote add origin <your-repo-url> git push -u origin main
-
Sign Up on Vercel
- Go to Vercel.
- Log in with your GitHub account.
-
Import Your Project
- Click Import Project on the Vercel dashboard.
- Select your GitHub repository.
-
Automatic Deployment
- Vercel detects your Next.js project and deploys it automatically.
- Within minutes, your app will be live on a
.vercel.app
domain.
Why Prateeksha Web Design Loves Next.js with TypeScript
At Prateeksha Web Design, we’ve mastered the art of creating fast, scalable, and visually stunning websites using Next.js and TypeScript. Whether you’re a budding developer or a business looking to take your online presence to the next level, our team is here to help you every step of the way.
We specialize in:
- Custom Next.js development.
- TypeScript-powered scalable solutions.
- Creating seamless user experiences.
Check out our portfolio to see what we can do!
Final Thoughts: Blast Off with Confidence 🚀
By now, you’ve got everything you need to set up a robust Next.js TypeScript development environment. Remember, learning is a journey, not a race. Embrace the errors—they’re just stepping stones to greatness.
Ready to build your first app? Or maybe you’re looking for a team of experts to handle it for you? Get in touch with Prateeksha Web Design—let’s make magic together!
About Prateeksha Web Design
Prateeksha Web Design specializes in creating sophisticated web applications using Next.js and TypeScript, ensuring optimal performance and scalability. Their services include setting up a robust development environment tailored for seamless collaboration and streamlined workflow. They focus on leveraging the benefits of static site generation and server-side rendering for enhanced user experiences. Additionally, Prateeksha offers ongoing support and maintenance, ensuring projects remain up-to-date with the latest technological advancements. Their expert team provides comprehensive training to empower clients in managing their Next.js applications efficiently.
Interested in learning more? Contact us today.
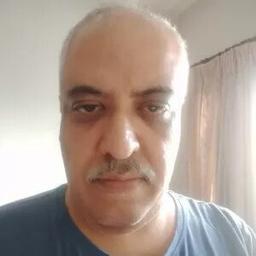