Welcome to the exciting world of Next.js logs and error tracking! If you’re stepping into this universe of debugging and sleuthing, don’t worry—you’ve come to the right place. Whether you’re a budding developer or a seasoned pro brushing up on best practices, we’ll walk you through the essentials in a friendly, jargon-free (mostly) way.
At Prateeksha Web Design, we live and breathe all things Next.js. We’ve dealt with error logs so tricky they felt like riddles from a wizard. Today, we’re here to share our knowledge with you.
Why Are Logs and Error Tracking So Important?
Let’s dig deeper into why logs and error tracking are the unsung heroes of your Next.js application.
The Dashboard Analogy: A Simple Way to Understand Logs
Imagine you’re driving a car. The dashboard is your lifeline—it provides critical information like your speed, fuel level, engine temperature, and alerts for potential issues. Without it, you’d be flying blind. You might run out of fuel in the middle of nowhere, unknowingly overheat your engine, or even miss a life-saving warning.
Now, think of your Next.js app as that car. Logs and error tracking tools are your dashboard. They give you visibility into your app’s health, behavior, and performance. Without these tools, you’re essentially driving blind in the world of code.
What Do Logs Do?
Logs are the bread and butter of application monitoring. They record significant events that occur while your app is running. Think of them as your app’s journal or diary. Whether a user signs in, an API request fails, or your app renders a page, a log can capture and document it.
Why Logs Matter
-
Monitoring Application Health Logs let you know if your app is operating as expected. For example:
- Is the homepage loading successfully?
- Are the API calls returning the correct data?
- Are there performance slowdowns during peak traffic?
-
Debugging When something goes wrong, logs are your first port of call. They help pinpoint:
- Where the issue occurred (e.g., a specific component or API call).
- What caused the problem (e.g., a missing variable or a misconfigured service).
- The impact of the issue (e.g., partial failure or complete crash).
-
Performance Optimization Logs can reveal bottlenecks, like slow database queries or heavy image files. By analyzing logs, you can fine-tune your app to run faster and smoother.
What is Error Tracking?
Error tracking is like having a detective on call for your app. While logs give you raw information, error tracking tools provide structured insights and help you take action. They not only capture errors but also:
- Group similar issues: Preventing clutter by grouping identical errors.
- Provide context: Including stack traces, user activity, and environment details.
- Send alerts: Notifying you instantly when something goes wrong.
Why Error Tracking is Essential
-
Early Detection Catch issues before they become user complaints. For example:
- If your payment gateway integration breaks, error tracking tools alert you before customers flood support with “Why can’t I pay?” tickets.
-
Increased Reliability A reliable app builds trust with users. By resolving errors proactively, you ensure users experience fewer disruptions.
-
Saves Time and Resources With detailed stack traces and context, you can debug faster. This reduces the time spent hunting for issues and allows your team to focus on feature development.
Benefits of Robust Error Tracking and Logging
1. Detect Bugs Before Users Do
Users expect smooth, seamless interactions. The dreaded “Oops, something went wrong!” message can frustrate them and lead to churn. Logs and error tracking tools act as your early warning system. They flag issues so you can fix them before they impact users.
Example: Let’s say an API request fails due to a timeout.
- Without logs: You’d hear about the issue only when users complain.
- With logs: You’d see the failed API request immediately in your logs and could resolve it before users even notice.
2. Debug with Confidence
Debugging without logs is like solving a murder mystery without clues—it’s pure guesswork. Logs and error tracking tools give you the evidence you need to solve problems effectively.
Example: Imagine your app crashes when users visit the product page. Logs could reveal:
- The error: A missing
productId
parameter in the API request. - The source: A typo in your code causing the parameter to be undefined.
3. Optimize Performance
Performance bottlenecks are silent killers—they don’t crash your app but degrade user experience. By analyzing logs, you can identify:
- Slow database queries.
- Long API response times.
- Components with high rendering times.
Example: If logs show a database query consistently takes 5 seconds, you can investigate and optimize it to improve overall performance.
Key Takeaways for Next.js Logging and Error Tracking
Let’s break it down step-by-step, like building a LEGO set (but less likely to end with a tiny brick embedded in your foot).
1. Set Up Basic Logging in Next.js
Next.js comes with a built-in logging system—console.log. Yes, the humble console.log
is still your best friend. But to take things up a notch, you’ll need a structured logging library.
Best Practices for Console Logs
- Use clear, descriptive messages:
console.log("Fetching user data for userId:", userId);
- Avoid overloading your logs. Spamming logs is like shouting in a library—no one’s going to appreciate it.
Advanced Logging Libraries
For more power, use libraries like Winston or Pino. They provide structured logs, log levels, and integration with log management tools.
Example with Winston:
import winston from "winston";
const logger = winston.createLogger({
level: "info",
format: winston.format.json(),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: "error.log", level: "error" }),
],
});
logger.info("Application started successfully.");
logger.error("An error occurred while fetching data.");
2. Leverage Error Tracking Tools
Error tracking is like having a detective on speed dial. These tools capture errors in real-time and give you the clues you need to fix them.
Popular Error Tracking Tools for Next.js
- Sentry: Tracks errors and performance issues with detailed stack traces.
- LogRocket: Replays user sessions, so you can see what went wrong.
- Bugsnag: Provides real-time error monitoring and actionable insights.
How to Integrate Sentry with Next.js
Sentry is a favorite in the Next.js community. Here’s how to set it up:
-
Install the Sentry SDK:
npm install @sentry/nextjs
-
Add Sentry Configuration: Create a
sentry.server.config.js
andsentry.client.config.js
in your project’s root directory. Add your Sentry DSN (from your Sentry project settings):import * as Sentry from "@sentry/nextjs"; Sentry.init({ dsn: "https://examplePublicKey@o0.ingest.sentry.io/0", tracesSampleRate: 1.0, });
-
Test It: Throw an intentional error in your app and check the Sentry dashboard to see it captured.
3. Debugging in Next.js: Tools and Techniques
Debugging is where the real fun begins. Here are some essential tools and techniques to make debugging less daunting.
1. Use the Next.js Dev Tools
Next.js provides built-in tools that help you debug effortlessly:
- Error overlay: Shows helpful error messages in the browser.
- Fast Refresh: Automatically reloads changes without losing state.
2. Enable Source Maps
Source maps link your compiled code to the original code, making stack traces easier to understand.
In your next.config.js
:
module.exports = {
productionBrowserSourceMaps: true,
};
3. Debugging with VS Code
Set breakpoints in your code and use the Debugging Console in VS Code. This method is perfect for inspecting variables and stepping through code.
4. Monitor Performance Logs
Performance issues are sneaky. They don’t crash your app outright but slowly drag it down. Use these tools to stay ahead:
- Lighthouse: Analyze your app’s performance, accessibility, and SEO.
- Next.js Analytics: A built-in feature that tracks page load speed and other metrics.
Example:
import { reportWebVitals } from 'next/app'
export function reportWebVitals(metric) {
console.log(metric);
}
This will log metrics like TTFB (Time to First Byte) and CLS (Cumulative Layout Shift).
5. Log Management: Centralize and Analyze
Logs are incredibly useful, but only if you can make sense of them. If your logs are scattered across different files, systems, or servers, they quickly become overwhelming—like trying to find matching socks in a giant pile of laundry. To stay organized and make your logs actionable, you need a centralized log management system.
Centralizing your logs means collecting them in a single platform, where they can be searched, filtered, and analyzed efficiently. This not only simplifies debugging but also provides valuable insights into your application’s performance, reliability, and user behavior.
Why Centralize Logs?
-
Unified View of Application Health Centralized logs allow you to see all your app’s events, errors, and performance metrics in one place. This holistic view ensures you don’t miss critical issues.
-
Faster Debugging When an issue arises, instead of hunting through multiple servers or systems, you can search for specific events or errors in a single platform.
-
Improved Collaboration Centralized platforms make it easy for teams to share insights, troubleshoot issues together, and maintain a consistent logging strategy.
-
Proactive Monitoring Many platforms offer alerting features, notifying you when specific thresholds or anomalies are detected.
How to Centralize Logs in a Next.js Application
Centralizing logs typically involves sending them to a log management tool via integrations or APIs. Here’s an example workflow:
-
Generate Logs in Next.js Use a library like Winston or Pino to create structured logs.
-
Send Logs to a Centralized Platform Use APIs or pre-built integrations provided by platforms like Elastic Stack, Datadog, or Papertrail.
-
Analyze and Act Use the platform’s features (like search, filtering, and visualization) to identify trends, diagnose issues, and optimize performance.
Best Log Management Platforms
Here are some of the top tools for managing logs effectively:
1. Elastic Stack (ELK)
- What It Is: A powerful open-source solution consisting of:
- Elasticsearch: Stores and indexes logs.
- Logstash: Collects and processes log data.
- Kibana: Visualizes logs with customizable dashboards.
- Why Use It: Ideal for real-time log monitoring, analytics, and creating custom dashboards.
- Best For: Teams that need a highly customizable and scalable solution.
Example Setup: You can ship logs to ELK using Logstash or directly from your Next.js app via the Elasticsearch API.
2. Datadog
- What It Is: A comprehensive platform combining log management, metrics, and tracing.
- Why Use It: Offers seamless integration with Next.js, powerful search and filtering, and built-in monitoring for performance and security.
- Best For: Teams looking for an all-in-one solution to monitor application health.
How It Works: Install the Datadog agent in your environment and use their logging API to stream logs from your Next.js app:
npm install --save datadog-metrics
With minimal setup, Datadog collects logs and provides out-of-the-box alerts for critical errors.
3. Papertrail
- What It Is: A lightweight, user-friendly log management tool.
- Why Use It: Simple setup, affordable pricing, and an intuitive interface.
- Best For: Small teams or projects that don’t need advanced analytics.
Example Integration: You can send logs from your Next.js app to Papertrail using their syslog or API integration.
Additional Recommendations
- Loggly: Cloud-based logging with powerful search and graphing capabilities.
- Fluentd: An open-source tool for log collection and aggregation.
- Graylog: Another open-source platform known for its scalability and customization.
Pro Tips for Log Management
-
Use Log Levels: Categorize logs (e.g., info, warn, error) so you can filter based on urgency.
logger.error("Critical database failure."); logger.info("User logged in successfully.");
-
Structure Your Logs: Use JSON formatting to ensure logs are machine-readable and easy to parse.
{ "timestamp": "2025-01-19T12:00:00Z", "level": "error", "message": "API timeout", "service": "user-service", "details": { "userId": "12345", "requestId": "abc-123" } }
-
Set Alerts: Configure alerts for critical issues like high error rates or performance degradation.
-
Monitor Trends: Use your centralized platform to analyze trends over time, such as increasing API errors or slow-loading pages.
6. Test Your Error Handling
Testing your error-handling mechanisms ensures your app stays robust. Simulate different error scenarios:
- Network failures.
- API errors.
- Server crashes.
Example: Custom Error Page
Create a pages/_error.js
file for a custom error page:
function Error({ statusCode }) {
return (
<p>
{statusCode
? `An error ${statusCode} occurred on server`
: "An error occurred on client"}
</p>
);
}
Error.getInitialProps = ({ res, err }) => {
const statusCode = res ? res.statusCode : err ? err.statusCode : 404;
return { statusCode };
};
export default Error;
7. Security and Privacy in Logs
Don’t log sensitive data like user passwords or credit card information. Mask or encrypt any sensitive details before logging.
Example:
const user = { name: "John Doe", password: "123456" };
// BAD
console.log(user);
// GOOD
console.log({ ...user, password: "*****" });
8. Stay Updated with Next.js Releases
The world of web development is constantly evolving, and Next.js is no exception. With every release, new features, optimizations, and bug fixes are introduced to make your applications faster, more secure, and easier to manage. Staying on top of these updates isn’t just a good idea—it’s essential for keeping your logging and error-handling practices current.
Here’s why and how to stay updated with Next.js releases:
Why Staying Updated Matters
-
Access New Features
Next.js frequently rolls out powerful features that can simplify your development process or improve your app’s performance. For instance:- Middleware introduced in Next.js 12 added flexible request/response handling.
- React Server Components in Next.js 13 brought improved rendering capabilities.
By staying updated, you ensure your app benefits from these advancements.
-
Fix Bugs and Security Issues
Each release includes patches that fix known bugs and address security vulnerabilities. Running an outdated version increases the risk of encountering issues that have already been resolved. -
Optimize Performance
Updates often include performance improvements, whether through better build times, faster rendering, or smaller bundle sizes. Staying current ensures your app performs at its peak. -
Stay Compatible with Logging and Error Tools
Logging and error-handling tools like Sentry or Datadog often update their integrations to support new Next.js features. Running an older version of Next.js might mean losing compatibility with these tools.
How to Stay Updated
-
Follow the Next.js GitHub Repository
The GitHub repo is your go-to source for:- Release notes
- Upcoming changes (via pull requests and issues)
- Contributions from the community
-
Subscribe to the Release Notes
The release notes summarize what’s new, including feature highlights, bug fixes, and breaking changes. You can find them in the repository’s releases section. -
Use the
next
orcanary
Tags
For experimental features or the latest updates, install the canary version of Next.js:npm install next@canary
This allows you to test new features before they’re officially released.
-
Follow Next.js Blog and Social Media
Keep an eye on the Vercel Blog and Next.js’s official Twitter account for announcements, tutorials, and best practices. -
Join the Community
The Next.js community is active on platforms like:- Next.js Discord
- Reddit’s r/nextjs
- Stack Overflow
These forums are great for discussing updates, troubleshooting, and sharing insights.
Tips for Updating Your Next.js Project
-
Review the Changelog First
Before updating, read the changelog to understand:- What’s new
- Deprecated features
- Breaking changes
-
Test in a Development Environment
Never update directly on a production app. Test the update in a staging or development environment to ensure everything works as expected. -
Update Dependencies
Next.js updates often require updates to related dependencies, like React or your logging/error-handling tools. -
Automate Updates Where Possible
Use tools like Dependabot or Renovate to automate dependency updates and get notified about new Next.js versions.
Conclusion: Debug Like a Pro!
Mastering Next.js logs and error tracking isn’t just about preventing errors; it’s about crafting a smoother, more reliable user experience. Logs tell you what’s happening; error tracking tools help you act fast, and debugging techniques make solving problems a breeze.
At Prateeksha Web Design, we specialize in building robust, error-free Next.js applications that scale. If you need expert guidance or want to hand off the heavy lifting, we’ve got your back.
Your Call to Action: Take the Next Step!
Are you ready to level up your Next.js skills? Start by setting up basic logs, integrating an error-tracking tool like Sentry, and experimenting with debugging techniques. Small steps today can save you from big headaches tomorrow.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services in Next.js, focusing on effective logs and error tracking to enhance web application performance. Their expertise includes integrating advanced monitoring tools, facilitating real-time error reporting, and implementing best practices for efficient debugging. By leveraging comprehensive analytics, they help clients identify and resolve issues swiftly, ensuring seamless user experiences. Their tailored solutions aim to optimize application reliability and streamline development workflows. Trust Prateeksha for a robust and error-free Next.js implementation.
Interested in learning more? Contact us today.
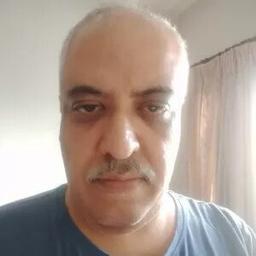