Table of Contents
- Introduction
- Understanding Image Optimization
- Image Optimization Techniques
- Next.js Image Component
- Next.js Image Optimization API
- Practical Guide to Mastering Image Optimization in Next.js
- Common Pitfalls and Best Practices
- Conclusion
- References
Introduction
1. Brief Overview of Next.js, Its Features, and Benefits
Next.js is a powerful framework built on top of React, designed to enhance the development process by offering features like server-side rendering (SSR), static site generation (SSG), and API routes. These features make it an ideal choice for building high-performance web applications that are optimized for SEO and user experience.
2. The Importance of Images in Web Development
Images are a crucial component of web development, significantly impacting user engagement, visual appeal, and content delivery. However, without proper optimization, images can become a major bottleneck, leading to slow loading times and poor performance.
3. Introduction to Image Optimization in Next.js
Image optimization is the process of reducing the file size of images without compromising their quality, ensuring faster loading times and a better user experience. In Next.js, image optimization is an essential strategy for maintaining high-performance standards, given its built-in capabilities that simplify and enhance this process.
Understanding Image Optimization
1. What is Image Optimization?
Image optimization involves a series of techniques aimed at reducing the size of image files while maintaining their visual quality. This process includes compressing images, choosing the right format, and implementing responsive images to deliver the best quality for varying screen sizes and devices.
2. Why is Image Optimization Crucial in Web Development?
Optimizing images is crucial because it directly impacts a website's loading speed, performance, and SEO rankings. Large, unoptimized images can slow down a website, leading to higher bounce rates and a negative user experience. Search engines, particularly Google, prioritize websites that load quickly, making image optimization a key factor in achieving better visibility and higher search rankings.
3. How Image Optimization Affects Loading Speed, Performance, and SEO
Optimized images reduce the amount of data that needs to be transferred over the internet, which speeds up page loading times. Faster loading pages not only improve user satisfaction but also contribute to better SEO outcomes, as search engines reward fast-loading websites with higher rankings.
Image Optimization Techniques
1. Different Image Optimization Techniques in Next.js
In Next.js, several techniques can be employed to optimize images:
- Compression: Reducing the file size of images using lossy or lossless compression algorithms.
- Responsive Images: Delivering different image sizes depending on the user's device or screen resolution.
- Lazy Loading: Loading images only when they appear in the viewport, reducing the initial load time.
- WebP Format: Using modern image formats like WebP, which provide better compression and quality.
Each of these techniques has its advantages and disadvantages, depending on the specific use case.
2. Advantages and Disadvantages of Each Technique
- Compression:
- Advantages: Reduces file size, faster loading.
- Disadvantages: Potential loss of image quality.
- Responsive Images:
- Advantages: Optimal display on all devices.
- Disadvantages: Increased complexity in implementation.
- Lazy Loading:
- Advantages: Decreases initial load time.
- Disadvantages: Can affect SEO if not implemented correctly.
- WebP Format:
- Advantages: Superior compression and quality.
- Disadvantages: Not supported by all browsers.
3. Code Snippets and Examples
import Image from "<a href="/blog/how-to-optimize-images-in-nextjs-with-the-image-component">next/image</a>";
export default function Example() {
return (
<Image
src="/path/to/image.jpg"
alt="Example Image"
width={500}
height={500}
quality={75}
placeholder="blur"
blurDataURL="data:image/png;base64,..."
/>
);
}
This code demonstrates the use of Next.js's Image
component, which automatically optimizes images by applying some of the techniques mentioned above.
Next.js Image Component
1. The Next.js Image Component and Its Role in Image Optimization
The Image
component in Next.js is a powerful tool designed to make image optimization seamless. It automatically handles resizing, compressing, and delivering responsive images, ensuring that your site loads quickly and looks great on any device.
2. How to Use the Image Component in Next.js
To use the Image
component, you simply need to import it and include it in your component tree. Here's a step-by-step guide:
- Step 1: Import the
Image
component fromnext/image
. - Step 2: Replace traditional
img
tags with theImage
component. - Step 3: Provide necessary props like
src
,alt
,width
, andheight
. - Step 4: Optionally, use additional props such as
quality
,layout
, andplaceholder
for more control over image behavior.
3. Relevant Code Samples and Screenshots
import Image from "next/image";
export default function Home() {
return (
<div>
<Image
src="/images/hero.jpg"
alt="Hero Image"
width={1200}
height={600}
layout="responsive"
/>
<h1>Welcome to My Next.js Site</h1>
</div>
);
}
This example demonstrates how to integrate the Image
component into a typical Next.js page, ensuring that the image is fully optimized and responsive.
Next.js Image Optimization API
1. The Next.js Image Optimization API: Overview
The Next.js Image Optimization API is a backend service that automatically optimizes images on the fly. It handles resizing, format conversion, and caching, delivering the most appropriate image size and format to the user.
2. Step-by-Step Guide to Using the Image Optimization API
- Step 1: Enable the API in your Next.js configuration.
- Step 2: Use the
Image
component to automatically leverage the API's features. - Step 3: Customize settings like default quality, formats, and caching strategies in the
next.config.js
file.
3. Examples of Usage and Explanation of Results
// next.config.js
module.exports = {
images: {
domains: ["example.com"],
deviceSizes: [640, 750, 1080, 1920],
imageSizes: [16, 32, 48, 64, 96],
},
};
In this configuration, the API is set up to handle images from a specific domain, with predefined device sizes for responsive image delivery. The API automatically selects the best size and format based on the user's device and network conditions.
Practical Guide to Mastering Image Optimization in Next.js
1. Combining Techniques, Image Component, and Image API
Mastering image optimization in Next.js involves combining all the discussed techniques—compression, responsive images, lazy loading—with the powerful features of the Image Component and Image Optimization API.
2. Real-World Scenario: Optimizing an E-commerce Website
Imagine you're working on an e-commerce website where high-quality images are crucial for showcasing products. Here’s how you can implement image optimization:
- Use the Image Component for product images to ensure they load quickly and look great on any device.
- Enable lazy loading for product thumbnails, so they load only when the user scrolls to them.
- Configure the Image Optimization API to deliver images in WebP format for browsers that support it.
3. Step-by-Step Implementation Guide
- Step 1: Analyze the types and sizes of images used on your site.
- Step 2: Implement the Image Component across your site.
- Step 3: Configure the Image Optimization API to handle dynamic image resizing and format conversion.
- Step 4: Monitor the performance impact using tools like Lighthouse or WebPageTest.
Common Pitfalls and Best Practices
1. Common Mistakes in Image Optimization
Some common mistakes include:
- Ignoring mobile optimization: Not delivering appropriately sized images for mobile devices.
- Overcompressing images: Reducing quality to the point where it affects the user experience.
- Not using modern formats: Failing to leverage WebP or other modern formats.
2. Best Practices to Avoid These Pitfalls
- Always test images on different devices and screen sizes to ensure they look good everywhere.
- Balance quality and file size by experimenting with different compression levels.
- Use modern formats like WebP when possible, while providing fallbacks for older browsers.
3. Tips and Tricks for Further Optimization
- Use CDNs (Content Delivery Networks) to deliver images faster by caching them closer to the user.
- Implement progressive loading techniques, so images appear gradually as they load.
- Regularly audit your site to find and fix any new image-related issues.
Conclusion
1. Summary of Key Points
Image optimization in Next.js is crucial for maintaining a fast, efficient, and SEO-friendly website. By using the Image Component and Image Optimization API, along with techniques like compression and responsive images, you can significantly enhance your site's performance.
2. Reinforcing the Importance of Image Optimization
Mastering these techniques not only improves the user experience but also boosts your site's visibility in search engines, making it a vital skill for any web developer working with Next.js.
3. Encouraging Application of Knowledge
Don’t just read about these strategies—apply them in your own projects. The benefits of optimized images are too significant to ignore, and Next.js makes it easier than ever to implement these best practices.
References
About Prateeksha Web Design
Prateeksha Web Design is a renowned web design company offering a wide array of services. They specialize in mastering image optimization in Next.js, a popular JavaScript framework. Their experts help in optimizing images to improve web performance, ensuring a seamless user experience. They prioritize responsive designs that captivate audiences while maintaining the website's speed and functionality.
Interested in learning more? Contact us today.
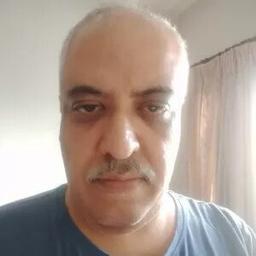