Table of Contents:
- [Introduction to Laravel 9 Authentication](#introduction-to-laravel-9-authentication)
- [Setting Up a Laravel 9 Project](#setting-up-a-laravel-9-project)
- Understanding Authentication in Laravel 9
- Creating the User Model and Migration
- Setting Up Laravel Authentication Routes
- Building the Registration Form
- Handling User Registration Logic
- Building the Login Form
- Handling Login Logic
- User Session Management
- Password Hashing and Security in Laravel
- Implementing Email Verification
- Role-Based Authentication in Laravel 9
- Best Security Practices for Authentication in Laravel
- Conclusion: Enhancing Authentication Security
1. Introduction to Laravel 9 Authentication
Laravel is one of the most popular PHP frameworks for building modern web applications due to its clean syntax and powerful tools. Laravel 9 comes with a comprehensive authentication system out-of-the-box, making it easier for developers to handle tasks like registration, login, and password management. This guide will walk you through building an authentication system using Laravel 9, following industry standards and best security practices to protect your app and users.
Why Choose Laravel 9 for Authentication?
With Laravel 9, you get access to advanced security features like password hashing, CSRF protection, and email verification built directly into the framework. This version also introduces several improvements to route handling, security updates, and performance optimizations that enhance the developer experience and ensure your authentication system is robust and future-proof.
2. Setting Up a Laravel 9 Project
Before we dive into the authentication system, let's set up a Laravel 9 project. You’ll need the following:
- PHP (>=8.0)
- Composer
- MySQL or another database
- A local development environment (e.g., XAMPP, Laravel Homestead, or Docker)
Step-by-Step Setup:
-
Install Laravel 9 using Composer. Open your terminal and run:
composer create-project --prefer-dist <a href="/blog/using-faker-in-laravel-to-generate-random-dates">laravel</a>/laravel laravel-auth
-
Navigate to the project directory:
cd <a href="/blog/mastering-subview-in-laravel-controller-a-comprehensive-guide">laravel</a>-auth
-
Configure the
.env
file for database connection:DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_auth DB_USERNAME=root DB_PASSWORD=
-
Run migrations to create necessary tables:
php artisan migrate
-
Start the development server:
php artisan serve
At this point, your Laravel 9 project should be up and running. Next, we'll explore how authentication works in Laravel.
3. Understanding Authentication in Laravel 9
Laravel provides a built-in authentication system that can handle tasks like registering new users, logging them in, and managing sessions. It includes:
- User Model: Represents the users in your application.
- Guards: Determine how users are authenticated (e.g., web or API guards).
- Providers: Define how users are retrieved from your storage (usually a database).
- Middleware: Protect routes to ensure only authenticated users can access certain pages.
Laravel’s authentication system leverages sessions to keep track of the user’s status, meaning users only have to log in once per session.
4. Creating the User Model and Migration
To enable authentication, Laravel requires a User model and a users table. Fortunately, Laravel 9 ships with a ready-made User model located in app/Models/User.php
. Let’s take a look at how this works:
User Migration
By default, Laravel includes a migration for the users table located in database/migrations
. This table contains essential fields for authentication, such as name
, email
, password
, and remember_token
.
Modifying the User Model
The User model includes essential traits that make it easy to interact with the authentication system. For example, the HasApiTokens
, Notifiable
, and HasFactory
traits are included by default.
<?php
namespace App\Models;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Laravel\Sanctum\HasApiTokens;
class User extends Authenticatable
{
use HasApiTokens, Notifiable;
protected $fillable = [
'name', 'email', 'password',
];
protected $hidden = [
'password', 'remember_token',
];
}
We will modify this model later when we implement email verification and role-based access.
5. Setting Up Laravel Authentication Routes
To enable authentication, we need to define the necessary routes for registration, login, and logout. Laravel provides these routes by default using the Auth scaffolding.
Running the Authentication Scaffolding
To generate authentication routes and views, run the following command:
php artisan make:auth
This will create the necessary views and routes for registration, login, password resets, etc. If you are using Jetstream or Breeze, you can install them to handle these tasks more elegantly:
composer require <a href="/blog/best-laravel-packages-to-build-powerful-crm-applications">laravel</a>/breeze --dev
php artisan breeze:install
npm install && npm run dev
php artisan migrate
This scaffolding generates everything needed to create a fully functional authentication system.
6. Building the Registration Form
Once the authentication routes are set up, we can customize the registration form. Laravel 9 automatically generates a view for registration located in resources/views/auth/register.blade.php
.
Form Components
Your registration form should contain fields for name, email, password, and confirm password. Here’s an example of how it looks:
<form method="POST" action="{{ route('register') }}">
@csrf
<input type="text" name="name" placeholder="Full Name" required />
<input type="email" name="email" placeholder="Email" required />
<input type="password" name="password" placeholder="Password" required />
<input
type="password"
name="password_confirmation"
placeholder="Confirm Password"
required
/>
<button type="submit">Register</button>
</form>
This form sends a POST request to the register
route, where user details are processed.
7. Handling User Registration Logic
In Laravel, registration logic is handled in the RegisterController. When a user submits the registration form, the system will validate the input and create a new user.
Registration Validation
To ensure that user input is safe and clean, Laravel uses form validation. In the RegisterController
, validation rules ensure that the email is unique, and the password meets certain security standards.
Here’s an example of how you might handle registration validation:
$request->validate([
'name' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => 'required|string|min:8|confirmed',
]);
User::create([
'name' => $request->name,
'email' => $request->email,
'password' => Hash::make($request->password),
]);
The password is hashed using Laravel’s built-in Hash
facade to ensure that it is securely stored.
8. Building the Login Form
Much like the registration form, the login form is created automatically when you scaffold the authentication views. It’s located in resources/views/auth/login.blade.php
.
Login Form Example
The login form typically asks for the user’s email and password:
<form method="POST" action="{{ route('login') }}">
@csrf
<input type="email" name="email" placeholder="Email" required />
<input type="password" name="password" placeholder="Password" required />
<button type="submit">Login</button>
</form>
Upon submission, this form sends a POST request to the login
route, where Laravel processes the login request.
9. Handling Login Logic
The LoginController manages the login logic. Laravel handles the complexity of validating user credentials and setting up a session for the authenticated user.
Login Validation and Authentication
When a user attempts to log in, Laravel validates their credentials using the Auth::attempt()
method. Here’s an example:
if (Auth::attempt(['email' => $request->email, 'password' => $request->password])) {
return redirect()->intended('dashboard');
} else {
return back()->withErrors([
'email' => 'The provided credentials do not match our records.',
]);
}
If the credentials are correct, the user is redirected to their dashboard. Otherwise, an
error message is displayed.
10. User Session Management
Laravel uses sessions to keep users logged in across requests. Once a user is authenticated, a session is created, and the user can access protected routes.
Logging Out
To log out a user, simply call the Auth::logout()
method:
Auth::logout();
return redirect('/');
This will terminate the user’s session and redirect them back to the homepage.
11. Password Hashing and Security in Laravel
Password security is crucial in any authentication system. Laravel makes this easy by using bcrypt, a secure hashing algorithm, to encrypt passwords before storing them in the database.
Hashing Passwords
When creating or updating a user’s password, Laravel uses the Hash::make()
function to securely hash it:
$password = Hash::make('your_password');
This ensures that even if the database is compromised, user passwords remain secure.
12. Implementing Email Verification
Laravel includes email verification out-of-the-box. This feature is useful for ensuring that users provide a valid email address during registration.
Setting Up Email Verification
To enable email verification, you need to implement the MustVerifyEmail
interface in your User model:
use Illuminate\Contracts\Auth\MustVerifyEmail;
class User extends Authenticatable implements MustVerifyEmail
{
// ...
}
Laravel will automatically send a verification link to the user’s email after registration.
13. Role-Based Authentication in Laravel 9
Role-based authentication is essential for controlling access to different parts of your application based on user roles (e.g., admin, editor, user).
Defining Roles
First, add a role
column to your users
table via migration, then modify the User model to include this field:
Schema::table('users', function (Blueprint $table) {
$table->string('role')->default('user');
});
Now, you can define middleware to check the user’s role before granting access to certain routes.
14. Best Security Practices for Authentication in Laravel
Security is paramount when building an authentication system. Here are some key security practices to follow in Laravel:
- Use HTTPS: Ensure all communications are encrypted using SSL.
- Rate Limiting: Protect your login routes from brute-force attacks by implementing rate limiting.
- CSRF Protection: Laravel automatically includes CSRF protection for all forms.
- Two-Factor Authentication (2FA): Enhance security by integrating 2FA using packages like Google Authenticator or Authy.
15. Conclusion: Enhancing Authentication Security
Building an authentication system in Laravel 9 is straightforward thanks to its comprehensive out-of-the-box tools. By following best security practices, you can ensure that your system is both functional and secure.
About Prateeksha Web Design
Prateeksha Web Design Company offers Laravel 9 services, specializing in creating advanced and secure authentication systems for applications. Their services ensure robust user management, protection against unauthorized access, and seamless integration with existing systems.
Interested in learning more? Contact us today.
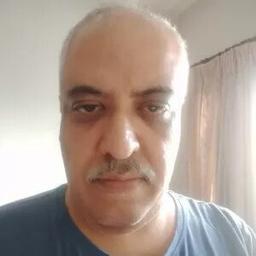