In the world of programming, terms like imperative programming and declarative programming often surface in discussions about coding paradigms. These two approaches represent fundamentally different ways of thinking about how we write code and solve problems. Whether you’re just starting your coding journey or looking to deepen your understanding of these paradigms, this guide will make it easy to grasp the core differences while highlighting why they matter in real-world programming.
At Prateeksha Web Design, we leverage both imperative and declarative programming paradigms to build powerful, efficient, and user-friendly applications for our clients. By understanding these concepts, you’ll gain insights into how professional developers think and work.
What Is Imperative Programming?
Imperative programming is one of the most fundamental programming paradigms. It is centered around the idea of providing detailed, step-by-step instructions for the computer to follow to achieve a desired outcome. This approach is akin to following a recipe or giving directions where each step is explicitly defined.
In imperative programming, you focus on the how—the exact process and sequence of operations needed to solve a problem. This means you control the program flow explicitly, managing the state and making changes as needed.
Understanding Imperative Programming with a Real-Life Example
Imagine you’re teaching someone how to cook pasta. In the imperative approach, you’d detail every step:
- Boil water in a pot.
- Add a pinch of salt to the boiling water.
- Place the pasta into the pot.
- Stir occasionally to prevent sticking.
- Wait for 10 minutes or until the pasta is cooked.
- Drain the water.
- Serve the pasta with sauce.
The person following your instructions doesn’t need to think about the goal—they simply execute the steps as provided. Similarly, in imperative programming, the computer executes commands in the precise order you define.
Key Characteristics of Imperative Programming
-
Explicit Control Flow:
- The programmer defines the exact sequence of instructions and operations.
- Constructs like loops, conditionals, and functions are used to control execution.
-
State Management:
- The state of the program (e.g., variable values) is managed and modified explicitly.
- This often involves operations like assigning values, incrementing counters, or updating flags.
-
Step-by-Step Instructions:
- Each action in the process is explicitly written out in the code.
- Example: Initializing variables, iterating over loops, and performing calculations.
-
Languages That Support Imperative Style:
- Languages like C, Java, and Python are commonly used for imperative programming.
- Even functional or declarative languages can have imperative features when used in certain ways.
Imperative Programming in Action
Example 1: Cooking Instructions
In the earlier pasta example, each step is explicitly defined. If the instructions aren't followed precisely, the desired result may not be achieved. This is similar to how a program must execute each instruction in the order it’s written.
Example 2: Summing Numbers Imperatively
In Python, summing numbers from 1 to 5 imperatively might look like this:
total = 0 # Initialize a variable to store the total
for i in range(1, 6): # Loop through numbers 1 to 5
total += i # Add each number to the total
print(total) # Print the result
Here’s what’s happening step-by-step:
- A variable
total
is initialized to 0. - A loop iterates over the numbers 1 through 5.
- Each number is added to
total
. - The result is displayed.
In this approach, you manage the loop, the variable, and the calculation explicitly. The computer doesn’t infer what you want—it executes the exact steps you specify.
Why Use Imperative Programming?
Strengths
-
Fine-Grained Control:
- You have precise control over the program's behavior and performance.
- Useful for tasks where optimization and efficiency are critical (e.g., system-level programming).
-
Closer to Machine Execution:
- Imperative code reflects how the computer’s hardware processes instructions, making it an ideal paradigm for low-level tasks.
-
Ease of Learning:
- For beginners, imperative programming is intuitive because it mirrors how humans think about problem-solving in a sequential way.
-
Flexibility:
- Allows developers to tailor the solution exactly to the requirements, especially when solving complex problems.
Challenges of Imperative Programming
-
Verbose Code:
- Writing out every detail can make the code longer and harder to read or debug, especially for complex programs.
-
State Management Complexity:
- Tracking and managing the program’s state manually increases the risk of bugs, especially in large-scale applications.
-
Tight Coupling:
- Since imperative programs often depend on specific sequences and state changes, they can be harder to modify or extend.
-
Reduced Scalability:
- As programs grow in size and complexity, imperative approaches can become unwieldy, requiring significant effort to maintain.
Where Imperative Programming Excels
-
Performance-Critical Applications:
- Imperative programming provides control over low-level details, making it ideal for applications like operating systems, embedded systems, and real-time processing.
-
Procedural Tasks:
- When the solution requires a defined sequence of steps (e.g., mathematical calculations, sorting algorithms).
-
Learning the Basics:
- Imperative programming lays the foundation for understanding more advanced paradigms like object-oriented or functional programming.
What Is Declarative Programming?
Declarative programming is all about describing what you want to achieve, not how to achieve it. Instead of focusing on step-by-step instructions, you define the desired outcome, and the underlying system figures out the details.
Key Characteristics of Declarative Programming:
- Focuses on what the task is.
- Uses declarative programming languages like SQL, HTML, and React.
- Relies on abstractions to hide implementation details.
Example of Declarative Programming:
Returning to our cooking example, a declarative approach would be:
- "Cook a vegetable curry."
You describe the result you want, and someone else figures out the steps to achieve it.
Code Example: Using Python, a declarative way to sum numbers might look like this:
total = sum(range(1, 6))
print(total)
Here, you simply declare your intention to sum a range of numbers, leaving the specifics to the sum()
function.
Strengths of Declarative Programming:
- Easier to read and maintain.
- Reduces errors by abstracting implementation details.
- Ideal for large-scale applications where simplicity and scalability matter.
Drawbacks:
- Limited control over performance optimizations.
- Steeper learning curve when first encountering concepts like declarative syntax.
Key Differences Between Imperative and Declarative Programming
Aspect | Imperative Programming | Declarative Programming |
---|---|---|
Focus | How to perform tasks | What the outcome should be |
State Management | Explicitly managed | Abstracted away |
Examples | C, Java, Python (imperative style) | SQL, HTML, React |
Complexity | More verbose, step-by-step | More abstract and concise |
Control | Fine-grained | Higher-level |
Ease of Maintenance | Harder as complexity grows | Easier due to abstraction |
Declarative Programming Paradigms: A Closer Look
1. Functional Programming
Functional programming is a subset of declarative programming. It avoids changing states and focuses on pure functions.
Example in JavaScript:
const numbers = [1, 2, 3, 4, 5];
const total = numbers.reduce((sum, num) => sum + num, 0);
console.log(total);
2. Query Languages (e.g., SQL)
SQL is a classic example of a declarative programming language.
SELECT SUM(amount) FROM transactions;
This query doesn’t specify how to calculate the sum but describes the desired outcome.
3. UI Development (e.g., React)
React uses declarative programming to build user interfaces.
function App() {
return <h1>Hello, World!</h1>;
}
You describe what the UI should look like, and React takes care of rendering it.
Choosing Between Imperative and Declarative
When deciding between imperative vs. declarative programming, the choice often depends on the problem at hand.
Use Imperative When:
- You need fine-grained control over performance.
- The problem involves a lot of state changes or low-level operations.
Use Declarative When:
- You want clean, maintainable code.
- The problem can be abstracted into higher-level constructs.
- Scalability and simplicity are priorities.
Declarative vs. Imperative APIs
APIs (Application Programming Interfaces) are at the core of modern software development, allowing developers to interact with systems and manipulate data. Just like programming paradigms, APIs can also follow either an imperative or declarative approach, each serving different purposes depending on the needs of the application.
Imperative APIs
An imperative API is designed to provide developers with functions or methods that allow explicit, step-by-step manipulation of data or system behavior. Developers must specify exactly how to achieve the desired result, controlling every detail of the process.
Characteristics of Imperative APIs:
- Explicit Instructions:
- Developers provide step-by-step commands to manipulate the system.
- State Management:
- The developer is responsible for managing the state and transitions.
- Granular Control:
- Fine-tuned control over every aspect of the API’s behavior.
Example: DOM Manipulation with JavaScript
const div = document.createElement('div'); // Create a new div element
div.textContent = 'Hello, World!'; // Set its content
document.body.appendChild(div); // Append it to the document body
In this example:
- You explicitly create a
div
, set its text, and attach it to the DOM. - Every step in the process is controlled by the developer.
Advantages of Imperative APIs:
- Provides complete control over the process.
- Useful for low-level programming or performance-critical tasks.
- Makes the flow of operations explicit, which can help in debugging.
Disadvantages of Imperative APIs:
- Verbose and harder to maintain as complexity increases.
- Higher potential for errors due to manual state management.
- Requires developers to focus on how things are done rather than on the desired outcome.
Declarative APIs
A declarative API allows developers to describe the desired outcome or state without worrying about the underlying implementation details. The API abstracts the operational steps and handles the process behind the scenes.
Characteristics of Declarative APIs:
- Abstracted Implementation:
- Developers specify what they want, and the API figures out how to achieve it.
- Simplified State Management:
- The API manages the state transitions and updates automatically.
- Higher-Level Abstraction:
- Focuses on the desired result rather than the procedural details.
Example: JSX in React
const App = () => <div>Hello, World!</div>;
In this example:
- The developer simply declares the desired outcome: a
div
with the text "Hello, World!". - React handles the underlying DOM manipulations to create and render the element.
Advantages of Declarative APIs:
- Easier to read, write, and maintain.
- Less error-prone due to abstraction of implementation details.
- Focuses on business logic and user experience rather than procedural steps.
Disadvantages of Declarative APIs:
- Limited control over low-level operations.
- Can be harder to debug when things go wrong, as the underlying implementation is abstracted.
- May introduce overhead compared to imperative approaches.
Comparing Imperative and Declarative APIs
Aspect | Imperative API | Declarative API |
---|---|---|
Focus | How to perform tasks | What the outcome should be |
Control | Developer has complete control | Control is abstracted by the API |
State Management | Managed explicitly by the developer | Automatically handled by the API |
Readability | More verbose and harder to read | Cleaner and easier to understand |
Examples | DOM Manipulation (JavaScript) | JSX in React, SQL Queries |
Declarative vs. Imperative Programming in Real-Life Scenarios
The distinction between imperative and declarative paradigms can also be observed in web development. Here are two concrete examples:
Example 1: Styling a Webpage
-
Declarative (CSS):
body { background-color: lightblue; }
- The developer declares what the webpage should look like.
- The browser handles the implementation details to achieve the desired style.
-
Imperative (JavaScript):
document.body.style.backgroundColor = 'lightblue';
- The developer explicitly sets the background color through JavaScript.
- Each step (accessing the
body
, modifyingstyle
, setting the color) is controlled by the developer.
Example 2: Rendering UI Elements
-
Declarative (React JSX):
const App = () => <button>Click Me</button>;
- The developer specifies the desired UI outcome (a button with text).
- React handles the rendering and updates the DOM as needed.
-
Imperative (Vanilla JavaScript):
const button = document.createElement('button'); // Create button button.textContent = 'Click Me'; // Set text document.body.appendChild(button); // Attach to DOM
- Every step is explicitly handled, from creating the button to adding it to the webpage.
Why Declarative APIs Are Becoming More Popular
The rise of frameworks like React, Angular, and Vue.js has brought declarative programming into the spotlight. Declarative APIs improve developer productivity and make codebases easier to maintain, especially for large-scale applications.
At Prateeksha Web Design, we embrace both imperative and declarative APIs, choosing the best approach based on the project’s needs. For example:
- We use imperative APIs for precise control over animations or custom integrations.
- We leverage declarative APIs like React for building scalable and maintainable front-end applications.
The Future of Programming: Declarative Development?
The evolution of programming has seen a clear shift towards simplicity, abstraction, and maintainability. As software becomes more complex and teams scale, developers increasingly rely on declarative paradigms to reduce boilerplate code, simplify workflows, and enhance productivity. Declarative development is emerging as a preferred approach in many areas, including cloud computing, infrastructure management, UI development, and more.
Why Declarative Development Is the Future
-
Simplifies Complexity
Declarative development abstracts away the how of execution, allowing developers to focus on the what. This makes it easier to work on complex systems without delving into low-level details.Example:
- When using Kubernetes, instead of writing scripts to manage and configure containers manually, developers can write YAML files that describe the desired state of their system. Kubernetes ensures the system matches this state.
-
Enhances Productivity
Developers spend less time writing procedural logic and debugging complex workflows. Declarative syntax improves readability and makes it easier for teams to collaborate.Example:
- React JSX allows developers to define the structure and behavior of UIs declaratively, reducing the time spent on DOM manipulation.
-
Improves Scalability and Maintenance
Declarative codebases are easier to scale and maintain. Since they focus on outcomes, they are less prone to bugs caused by intricate state management or step-by-step execution. -
Embraces Automation
Declarative tools align well with automation trends, particularly in DevOps and cloud-native environments. By defining desired states, declarative systems automate the process of ensuring those states are achieved.Example:
- Tools like Terraform enable developers to define infrastructure as code, describing resources (e.g., servers, databases) without detailing how they should be provisioned.
Trends Driving Declarative Development
-
Serverless Computing Serverless platforms like AWS Lambda and Azure Functions are inherently declarative. Developers define triggers, events, and desired outputs while the platform handles the underlying execution.
Example:
- You can write a serverless function that processes user data when uploaded to a storage bucket without worrying about provisioning or managing servers.
-
Infrastructure as Code (IaC) IaC tools like Terraform and AWS CloudFormation are becoming industry standards. They allow developers to define infrastructure configurations in a declarative way.
Terraform Example:
resource "aws_instance" "web" { ami = "ami-12345678" instance_type = "t2.micro" }
The above code describes the desired configuration, and Terraform ensures the instance is created and maintained.
-
Container Orchestration with Kubernetes Kubernetes uses YAML-based declarative configuration files to define the desired state of clusters, deployments, and services.
Kubernetes Example:
apiVersion: apps/v1 kind: Deployment metadata: name: nginx-deployment spec: replicas: 3 selector: matchLabels: app: nginx template: metadata: labels: app: nginx spec: containers: - name: nginx image: nginx:1.17
This configuration ensures that three replicas of the Nginx container are running.
-
Front-End Frameworks Front-end frameworks like React, Vue.js, and Svelte embrace declarative programming for UI development. Instead of imperatively manipulating the DOM, developers describe the UI and its behavior.
React JSX Example:
const App = () => <h1>Hello, World!</h1>;
React takes care of rendering and updating the DOM efficiently.
-
Data Querying Declarative query languages like SQL allow developers to describe what data they want rather than how to fetch it.
SQL Example:
SELECT name, age FROM users WHERE age > 21;
The database system interprets the query and retrieves the desired data.
Examples of Declarative Languages
Declarative programming is prevalent in various domains, with each language designed to describe specific outcomes:
-
SQL (Structured Query Language):
- Used to query and manipulate databases.
- Focuses on what data is needed, not how to retrieve it.
Example:
SELECT * FROM products WHERE price > 100;
-
HTML (HyperText Markup Language):
- Defines the structure of a webpage.
- Focuses on what elements should appear, leaving rendering to the browser.
Example:
<h1>Welcome to My Website</h1>
-
CSS (Cascading Style Sheets):
- Describes how elements should appear (styling) on a webpage.
- Focuses on outcomes, like background color or font size.
Example:
body { background-color: lightblue; }
-
React JSX (JavaScript Syntax Extension):
- Describes the UI structure and behavior.
- Focuses on defining components and their relationships.
Example:
const App = () => <button>Click Me!</button>;
Declarative Development at Prateeksha Web Design
At Prateeksha Web Design, we leverage declarative paradigms to build robust, scalable, and user-friendly applications. Here’s how we use declarative development:
-
Infrastructure Automation:
- Using tools like Terraform for cloud infrastructure.
- Ensuring repeatability and consistency in deployments.
-
- Crafting stunning front-end interfaces with frameworks like React and Vue.js.
- Using CSS and HTML to create responsive and accessible designs.
-
Streamlined Workflows:
- Adopting declarative tools to simplify tasks like CI/CD pipelines and container orchestration.
-
Data-Driven Applications:
- Writing efficient SQL queries for database management.
- Integrating APIs that support declarative configurations.
Conclusion
Understanding the differences between imperative vs declarative programming is essential for every developer. While imperative programming gives you granular control, declarative programming simplifies the development process and improves maintainability. At Prateeksha Web Design, we harness the best of both worlds to deliver top-notch solutions for our clients.
By choosing the right paradigm for the right task, you can write better code, solve problems efficiently, and elevate your programming skills to the next level. Whether you’re coding for a personal project or collaborating with a team, knowing when to use imperative code versus declarative syntax can make all the difference.
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services focused on educating clients about imperative vs. declarative programming. Our expert team creates engaging content and visual aids to simplify the differences between these programming paradigms. We provide tailored web solutions that highlight their applications in modern web development. Our aim is to empower businesses to make informed decisions based on their specific programming needs. Let us help you navigate the complexities of coding for optimal online performance.
Interested in learning more? Contact us today.
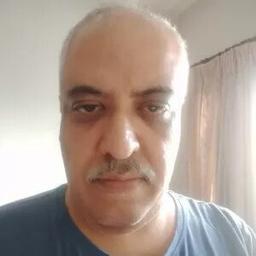