In the vibrant world of programming, one question often comes up: should you choose static typing or dynamic typing for your project? This seemingly simple choice can have far-reaching implications for your development process, team collaboration, and the final product. Let's dive deep into the differences, pros and cons, and how you can make the right choice for your next project.
Here at Prateeksha Web Design, we’ve navigated both static and dynamic typing across various projects, and we’re here to share our insights.
Understanding the Basics: Static Typing vs. Dynamic Typing
When discussing programming paradigms, two major concepts are static typing and dynamic typing. Understanding these foundational concepts is crucial because they influence how your program is written, how errors are detected, and how it performs.
What is Static Typing?
Static typing refers to a programming style where the type of every variable is explicitly defined at compile time. This means the language enforces rules about what type of data each variable can hold before the program runs. If a type mismatch is detected, the compiler throws an error and halts the process, preventing the program from running until the issue is resolved.
Languages that use static typing include Java, C++, C#, TypeScript, and others.
Key Characteristics:
-
Explicit Type Declaration
You must declare a variable's type when it’s created. For instance:let age: number = 25; let name: string = "Alice";
The compiler ensures that the value assigned to the variable matches its declared type.
-
Type Checking at Compile Time
All type-related errors are caught during the compilation phase. This ensures the code is free of type errors before it’s executed. -
Predictable and Reliable
Because variable types are locked in at compile time, static typing offers predictability. Developers know exactly what type of data a variable will hold.
Example of Static Typing in TypeScript:
let name: string = "John";
name = 42; // Error: Type 'number' is not assignable to type 'string'
In this example:
- The variable
name
is explicitly declared as astring
. - Attempting to assign a
number
toname
triggers a compile-time error.
This early error detection ensures that such type-related bugs don’t make it to runtime, reducing the chances of unexpected crashes.
Advantages:
- Fewer Runtime Errors: Errors are caught early, making the program more robust.
- Improved Code Clarity: Explicit declarations act as self-documentation, making the code easier to understand.
- Better Tool Support: Static typing languages work well with IDEs, enabling features like auto-completion, refactoring, and type inference.
- Optimized Performance: Since type checking is done at compile time, the runtime execution is faster.
What is Dynamic Typing?
Dynamic typing, in contrast, is a programming style where the type of a variable is determined at runtime. You don’t need to declare a type explicitly; the interpreter assigns a type based on the value assigned to the variable.
Languages that use dynamic typing include Python, JavaScript, Ruby, PHP, and others.
Key Characteristics:
-
No Explicit Type Declaration
Variables can hold any type, and their types can change throughout the program’s execution. For instance:name = "Alice" name = 42 # This is perfectly valid
-
Type Checking at Runtime
Errors related to variable types are only caught when the program runs. This means potential bugs might not be detected until the code is executed. -
Flexible and Agile
Dynamic typing allows for rapid prototyping and experimentation since you don’t need to worry about declaring types.
Example of Dynamic Typing in Python:
name = "John"
name = 42 # No error!
In this example:
- The variable
name
initially holds a string,"John"
. - Later, it is reassigned to a number,
42
, and Python has no problem with this because the type is evaluated dynamically.
Advantages:
- Simpler Syntax: No need to declare types explicitly, resulting in cleaner and shorter code.
- Faster Development: Perfect for prototyping and rapid iterations, as you can quickly change code without worrying about type declarations.
- Beginner-Friendly: Developers can focus on logic without worrying about type enforcement.
The Key Differences: Static Typing vs. Dynamic Typing
To summarize, let’s compare the two paradigms across critical aspects:
Aspect | Static Typing | Dynamic Typing |
---|---|---|
Type Declaration | Types must be explicitly declared. | Types are inferred dynamically based on assigned values. |
Type Checking | Performed at compile time. Errors are caught early. | Performed at runtime. Errors may not appear until the code is executed. |
Code Flexibility | Less flexible, as types cannot change after declaration. | Highly flexible, as variables can hold any type and change types dynamically. |
Error Detection | Compile-time checking prevents many runtime bugs. | Runtime errors related to type mismatches may only appear when running the code. |
Learning Curve | Steeper, due to the need to explicitly define types and understand type systems. | Easier, as developers don’t need to worry about type declarations. |
Performance | Faster, as type checks are pre-done at compile time. | Slower, due to runtime type checks. |
Use Case | Ideal for large, complex systems where stability and performance are critical. | Perfect for small projects, scripts, and rapid prototyping. |
The Pros and Cons of Static Typing: In-Depth Analysis
Static typing is a programming paradigm that enforces strict type rules at compile time, ensuring that type-related errors are caught before the program is executed. Let’s explore its advantages and disadvantages in detail.
Pros of Static Typing
1. Fewer Runtime Errors
- How It Works: In statically typed languages like Java, C++, and TypeScript, the compiler ensures all variables are assigned values that match their declared types before the program runs. For example:
let age: number = 25; age = "twenty-five"; // Error: Type 'string' is not assignable to type 'number'
- Why It’s Important: This early detection prevents many runtime crashes, particularly in large and complex systems where undetected bugs can have severe consequences.
- Real-Life Impact: Consider a financial application where incorrect data types could lead to erroneous calculations. Static typing ensures such bugs are caught during development.
2. Better Tooling and IDE Support
- How It Works: Statically typed languages are compatible with powerful Integrated Development Environments (IDEs) like IntelliJ IDEA, Eclipse, or Visual Studio Code. These tools can:
- Offer code completion suggestions based on declared types.
- Highlight type mismatches in real-time.
- Provide refactoring support, making it easier to rename variables or change types across a large codebase.
- Why It’s Important: These features save time and reduce the risk of introducing errors during code updates.
- Example: In TypeScript, if you change a class property’s type, the IDE can instantly highlight all impacted parts of the code.
3. Improved Code Readability
- How It Works: Explicit type declarations act as self-documenting code. A developer reading the code can immediately understand what kind of data a variable is supposed to hold.
- Why It’s Important: In large teams or when working on long-term projects, code readability is crucial. It makes onboarding new developers easier and ensures that code is maintainable.
- Example:
Contrast this with dynamically typed code, where the type isn’t explicitly declared and might need to be inferred.int employeeCount = 50; // Clear indication that employeeCount is an integer
4. Optimized Performance
- How It Works: In statically typed languages, the compiler performs type checks during compilation, which means there’s no need for additional type-checking logic at runtime.
- Why It’s Important: This optimization results in faster execution times, especially for performance-critical applications like gaming engines or real-time systems.
- Example: A game engine written in C++ (statically typed) typically outperforms one written in Python (dynamically typed) because C++ avoids runtime type checks.
Cons of Static Typing
1. Inflexibility
- How It Works: Static typing enforces strict rules about what types can be assigned to variables, which can limit creative problem-solving.
- Why It’s a Drawback: In fast-paced environments, such rigidity might slow down experimentation and prototyping.
- Example: In TypeScript, if you’ve declared a variable as a
number
, you can’t later assign it astring
, even if it makes sense in the context:let userInput: number = 42; userInput = "Invalid input"; // Error: Type mismatch
2. Verbose Code
- How It Works: Statically typed languages require explicit type declarations, which can result in verbose code. While modern languages like TypeScript offer some degree of type inference, many still require manual type specification.
- Why It’s a Drawback: This verbosity can make code harder to write and understand, especially for beginners or in projects where simplicity is valued.
- Example: Compare Java (statically typed) with Python (dynamically typed):
int count = 10; // Java count += 1;
count = 10 # Python (shorter and simpler) count += 1
3. Longer Development Time
- How It Works: The strictness of static typing can slow down development, particularly for small teams or projects where speed is more important than precision.
- Why It’s a Drawback: Developers must spend extra time declaring types, resolving type mismatches, and ensuring all type requirements are met.
- Example: When building a prototype, declaring types for every variable in a language like C# can be time-consuming compared to the freedom offered by Python or JavaScript.
The Pros and Cons of Dynamic Typing: In-Depth Analysis
Dynamic typing is a more flexible paradigm that determines variable types at runtime. Let’s dive into its advantages and disadvantages.
Pros of Dynamic Typing
1. Ease of Use
- How It Works: Developers don’t need to worry about type declarations or enforcement. The interpreter dynamically assigns types based on the value assigned to a variable.
- Why It’s Important: This simplicity makes dynamic typing a favorite among beginners and those working on projects where speed is a priority.
- Example:
value = 42 value = "A string value" # Perfectly valid in Python
2. Rapid Prototyping
- How It Works: Without the need to define types, developers can focus on functionality rather than type rules, speeding up the development process.
- Why It’s Important: Startups and MVP (Minimum Viable Product) projects often need to get ideas to market quickly, and dynamic typing is perfect for this.
- Example: A web app written in JavaScript can be quickly tested and iterated upon without worrying about type safety.
3. Less Boilerplate Code
- How It Works: Dynamic languages often require fewer lines of code since there’s no need for explicit type declarations.
- Why It’s Important: This makes codebases shorter and easier to read.
- Example:
Compare this with the statically typed equivalent:let user = "John"; // Dynamic typing
let user: string = "John"; // Static typing
4. Flexibility
- How It Works: Variables can hold any type, and their type can change during execution.
- Why It’s Important: This allows developers to solve problems creatively and adapt to changing requirements without being constrained by type rules.
- Example:
let item = 42; item = "Now it's a string"; // Completely valid in JavaScript
Cons of Dynamic Typing
While dynamic typing offers flexibility and simplicity, it has its drawbacks:
1. Runtime Errors
- Without compile-time type checks, errors might only surface during execution, potentially causing crashes or bugs.
- Example: A typo in JavaScript might lead to undefined variables, which could break your app.
2. Debugging Challenges
- Debugging type-related errors can be tricky because the root cause may not be apparent until the program runs.
3. Performance Overheads
- Runtime type checking introduces additional processing, making dynamically typed languages slower than their statically typed counterparts.
Cons of Dynamic Typing: In-Depth Explanation
Dynamic typing offers unparalleled flexibility and ease of use, but it also has some notable drawbacks that can affect the stability, maintainability, and performance of your application. Let’s explore these challenges in detail.
1. Runtime Errors
How It Works:
In dynamically typed languages, type checks are performed during runtime instead of compile time. While this provides flexibility during development, it also means that the interpreter won’t catch type-related errors until the program is executed.
Why It’s a Problem:
- Errors that could have been caught early in a statically typed language remain hidden in dynamically typed code until specific parts of the program are run. This can lead to unexpected crashes or unintended behavior.
- These errors can be subtle and occur in edge cases, which might not be immediately evident during testing.
Example:
Here’s a simple example in JavaScript:
function addNumbers(a, b) {
return a + b;
}
// Works fine with numbers
console.log(addNumbers(5, 10)); // Output: 15
// Causes unexpected behavior
console.log(addNumbers(5, "10")); // Output: "510" (string concatenation)
In the second call, instead of adding the numbers, JavaScript concatenates them because one of the inputs is a string. A statically typed language like TypeScript would catch this issue during compilation.
Real-World Impact:
- In production systems, a type error might lead to application downtime or data corruption.
- In critical domains like finance or healthcare, such errors can have severe consequences, such as incorrect calculations or data loss.
2. Debugging Challenges
How It Works:
Dynamic typing allows variable types to change at runtime, making it harder to track the flow of data and debug issues. Unlike statically typed languages, which enforce type constraints and provide clear error messages during compilation, dynamically typed languages rely on runtime execution to expose issues.
Why It’s a Problem:
- Debugging type-related errors in dynamic languages can be challenging, especially in large codebases where variables pass through multiple functions and contexts.
- Without explicit type annotations, it’s harder to trace what kind of data a variable should hold, leading to confusion when something goes wrong.
Example:
Imagine a Python program that processes user data:
def process_user_data(user):
print(user['name']) # Assumes 'user' is a dictionary
# Works fine
process_user_data({'name': 'Alice'})
# Raises a runtime error
process_user_data('Alice') # TypeError: string indices must be integers
In this example:
- The function assumes
user
is a dictionary, but there’s no enforcement of that assumption. - Passing a string instead of a dictionary results in a TypeError during runtime.
Real-World Impact:
- Debugging such errors requires tracing the program’s execution and identifying where the wrong type was introduced.
- For teams working on large projects, inconsistent type usage can lead to miscommunication and delays in debugging.
3. Performance Overheads
How It Works:
In dynamically typed languages, the interpreter must perform type checking and type inference at runtime. This adds an additional layer of processing compared to statically typed languages, where type information is already resolved during compilation.
Why It’s a Problem:
- The continuous type checking at runtime makes dynamically typed languages slower in execution, especially in performance-critical applications like games, high-frequency trading platforms, or real-time systems.
- Statically typed languages like C++ and Java are optimized for performance since type information is baked into the compiled code.
Example:
Let’s consider a Python loop that processes a large dataset:
numbers = [1, 2, 3, 4, 5]
result = 0
for num in numbers:
result += num
In Python:
- Each iteration requires the interpreter to determine the type of
result
andnum
dynamically. - This type-checking process adds overhead compared to a statically typed equivalent in C++:
int numbers[] = {1, 2, 3, 4, 5};
int result = 0;
for (int num : numbers) {
result += num;
}
In C++, type information is resolved at compile time, allowing the loop to execute faster.
Real-World Impact:
- Dynamic languages like Python or JavaScript may struggle to handle large-scale computations efficiently.
- In industries requiring high performance, such as gaming or data processing, this overhead can make dynamically typed languages unsuitable.
Summary: The Challenges of Dynamic Typing
Challenge | Explanation | Impact |
---|---|---|
Runtime Errors | Errors only surface when the program runs, leading to potential crashes or incorrect behavior. | Makes testing and bug resolution more critical to avoid issues in production. |
Debugging Challenges | Lack of type enforcement makes it harder to identify and fix issues, especially in large codebases. | Debugging becomes time-consuming, requiring careful tracing of the program’s execution. |
Performance Overheads | Runtime type checking adds extra processing, making dynamic languages slower than their statically typed counterparts. | Limits the use of dynamic languages in performance-critical applications like gaming or real-time systems. |
Choosing the Right Typing for Your Project
Now that you understand the pros and cons of both paradigms, how do you decide what’s best for your project? Let’s break it down based on use cases and requirements.
1. Project Type
- Static Typing: Large-scale applications, systems with strict safety requirements (e.g., banking software), and long-term projects that involve multiple developers.
- Dynamic Typing: Startups, MVPs, small-scale applications, and projects requiring frequent changes.
2. Team Expertise
- Static Typing: If your team is experienced in strongly typed languages, the structured nature of static typing will enhance productivity.
- Dynamic Typing: Ideal for teams that prioritize flexibility or consist of developers familiar with dynamic languages.
3. Development Speed
- Static Typing: Slower initially but reduces debugging time later.
- Dynamic Typing: Faster development in the early stages, but debugging might take longer.
4. Performance
- Static Typing: If performance is critical (e.g., gaming engines, real-time systems), go static.
- Dynamic Typing: For scripts or non-performance-critical tasks, dynamic is sufficient.
Real-World Examples
Static Typing in Action
At Prateeksha Web Design, we’ve used TypeScript to build a robust e-commerce platform for a retail giant. The type safety ensured smooth integration with payment gateways and third-party APIs, eliminating runtime surprises.
Dynamic Typing in Action
For a startup’s MVP, we leveraged Python and JavaScript to rapidly build a prototype. Dynamic typing allowed us to iterate quickly, adding features on the go without worrying about rigid type systems.
Prateeksha Web Design’s Approach
At Prateeksha Web Design, we understand that every project is unique. Here’s how we help our clients decide between static and dynamic typing:
- Project Analysis: We assess the scope, complexity, and performance requirements of your project.
- Team Collaboration: We work with your team to determine their expertise and preferences.
- Future-Proofing: We consider the long-term implications of the chosen typing paradigm, ensuring scalability and maintainability.
The Verdict
So, which is right for your project—static typing or dynamic typing? The answer isn’t one-size-fits-all. Instead, it depends on your project’s specific needs, team expertise, and goals.
TL;DR
- Go static if you need reliability, performance, and long-term maintainability.
- Go dynamic if you need speed, flexibility, and ease of use.
If you’re still unsure, reach out to Prateeksha Web Design. Our team has the experience and expertise to guide you through the decision-making process and deliver a solution tailored to your needs.
Whether you’re building a startup MVP or a full-fledged enterprise application, the choice between static and dynamic typing can shape the future of your project. Choose wisely, and happy coding!
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services that clarify the differences between static and dynamic typing, helping clients choose the best approach for their projects. With a focus on project requirements, they provide tailored solutions that enhance performance and maintainability. Their team of experts guides clients through the pros and cons of each typing method, ensuring informed decisions. Whether you need a straightforward static site or a feature-rich dynamic application, Prateeksha Web Design delivers customized web development services. Trust them to optimize your project for scalability and efficiency.
Interested in learning more? Contact us today.
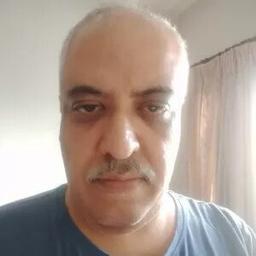