"name": "My Progressive Web Application",
"icons": [
{
"src": "icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
],
"start_url": "/",
"background_color": "#ffffff",
"display": "standalone",
"scope": "/",
"theme_color": "#000000"
}
And voilà! You now have a foundational setup for your PWA. This manifest will help define your application’s appearance and how it behaves when opened on a user's device.
Key Takeaway #3: Crafting the Core Features of Your PWA
With your project set up, let’s sprinkle some enchantment to your app by incorporating core PWA features. Here are some vital components you should aim to include:
Service Workers
Service workers act like a magical middleman between your web application and the network. They allow you to intercept network requests, cache responses, and serve them even when the user is offline. With next-pwa
, your service worker will practically set itself up!
Simply add the following to your public
directory:
- Service Worker Logic:
// src/service-worker.js self.addEventListener('install', event => { console.log('Service Worker installing.'); }); self.addEventListener('activate', event => { console.log('Service Worker activating.'); }); self.addEventListener('fetch', event => { event.respondWith( caches.match(event.request).then(response => { return response || fetch(event.request); }) ); });
Offline Functionality
Now, ensure your app works seamlessly offline by using caching strategies for assets and data. For example, in your next-pwa
configuration, you can specify routes to cache:
const withPWA = require('next-pwa')({
dest: 'public',
runtimeCaching: [
{
urlPattern: /^https:\/\/yourapi.com\/.*$/,
handler: 'NetworkFirst',
},
{
urlPattern: /^https:\/\/yourassets.com\/.*$/,
handler: 'CacheFirst',
},
],
});
Push Notifications
Engaging users with timely updates makes your app more interactive. Incorporate push notifications using a server-side platform or service (like Firebase) that sends alerts directly to users’ devices—keeping them in the loop even when the app isn’t open.
Key Takeaway #4: Testing and Deploying Your PWA
Before showing off your magical creation to the world, let’s talk about testing and deployment.
Testing Your PWA
Use tools like Google's Lighthouse to assess your PWA’s performance. Open Chrome DevTools, navigate to the "Lighthouse" tab, and generate a report. This will help you review aspects such as performance, accessibility, and PWA compliance.
Deployment
To deploy your app, you can choose hosting platforms such as Vercel (the creators of Next.js) or Netlify. With just one command, you can deploy your app live into the world:
vercel
Follow the prompts, and in no time, your PWA will be soaring through cyberspace!
Conclusion: Your Magical Journey Awaits!
Congratulations, brave developer! You've delved into the enchanting world of Progressive Web Applications using Next.js! From understanding the nature of PWAs to crafting one that’s robust, offline-capable, and fast—you're well on your way to mastering the art of web wizardry.
Now, go forth and build your magical creations. The web is waiting for your spellbinding applications that can be experienced by users any time, anywhere! Happy coding! 🎩✨
Certainly! Let’s expand, explain in detail, and refine the provided content for clarity and depth.
Manifest for My Progressive Web Application
In creating a Progressive Web Application (PWA), the first essential element is the Web App Manifest, which is a JSON file that provides important metadata about your application. Here’s a breakdown of the various properties you might include in the manifest:
{
"name": "My Progressive Web Application",
"short_name": "My PWA",
"icons": [
{
"src": "icon.png",
"sizes": "192x192",
"type": "image/png"
}
],
"start_url": ".",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000"
}
Explanation of Key Properties:
-
name: This is the name of your PWA as it will appear to users. It should be descriptive and compelling so users feel intrigued to explore your application.
-
short_name: A shorter version of your application name, used when there’s limited space, such as on the home screen or task switcher.
-
icons: This array defines the app icons used in various contexts, such as on the home screen or in the browser. The
src
specifies the image file location, andsizes
indicates the dimensions of the icon, ensuring proper display on different device resolutions. Here we define a 192x192 pixel PNG icon. -
start_url: The URL that the browser loads when the app is started. The dot (
"."
) means it will start at the root of the application. -
display: This specifies the preferred display mode for the application. The
standalone
mode makes the app look and feel like a native mobile application, without browser chrome. -
background_color: The background color of your application, which is displayed during the loading process.
-
theme_color: This affects the appearance of the browser's UI elements, such as the address bar, and provides a cohesive aesthetic that aligns with your branding.
In summary, once you have configured your manifest file correctly, you have effectively laid the groundwork for your PWA!
Key Takeaway #3: Service Workers are Your New Best Friends
When it comes to increasing the efficiency and interactivity of your PWA, service workers are indispensable. You can think of them as the loyal sidekick that watches over your app, graciously performing tasks to enhance the user experience.
What are Service Workers?
Service workers are scripts that run in the background of a web browser, independently of a web page. Their core responsibilities include intercepting and managing network requests, caching important assets, and enabling offline functionality.
Imagine being on a roller coaster ride—the exhilarating rush as you go down steep drops represents the rapid speed at which your website responds to user interactions, powered by strategic caching!
Implementing Service Workers in Next.js PWA:
While the concept may sound daunting, implementing service workers in a Next.js PWA is simplified through the use of the next-pwa
package. However, there are some practices worth noting:
-
Caching Strategies: You must define caching strategies in your configuration file (
next.config.js
). This determines how resources are cached and retrieved. Here’s a sample implementation:const runtimeCaching = [ { urlPattern: /^https:\/\/yourapi\.com\/.*$/, handler: 'NetworkFirst' // Prioritize fresh content from the network first }, { urlPattern: /^https:\/\/yourcdn\.com\/.*$/, handler: 'CacheFirst' // Serve cached content if it exists }, ];
- NetworkFirst: This strategy is ideal for dynamic content, ensuring that your application always attempts to get the latest information from the server.
- CacheFirst: Use this for static assets like images or stylesheets, ensuring quick loading times as they are often cached locally.
-
Pre-caching: This refers to the process of caching designated assets when the service worker is installed. With
next-pwa
, you can easily specify routes and resources that should be pre-cached. It’s akin to ensuring your backpack is stocked with essentials before setting off on your journey!
Encourage experimentation with these strategies—imagine it as creating a treasure map where you can explore while keeping your bases covered.
Key Takeaway #4: Embracing Next.js Offline Mode & Caching
Consider the scenario: you’re on a long-haul flight, navigating through unexpected turbulence, and Wi-Fi connectivity is as unreliable as a cat chasing after its tail. This is where Next.js offline mode comes into play!
The Importance of Offline Mode:
Implementing offline mode elevates user experience dramatically. Users should feel confident that they can access your app even when connectivity is lacking—it's akin to having your favorite book with you on the go!
By utilizing service worker caching as outlined above, users can revisit previously loaded pages with ease. Here's how this functionality can be further refined:
-
Cache Preloading: With packages like
next-pwa
, you can configure specific assets to be preloaded during the first visit. This means they will be stored in the cache, creating a smoother experience on subsequent visits. Think of it as preparing your equipment before an expedition, ensuring you’re ready for adventure! -
Dynamic Caching: This method provides a seamless experience by caching dynamically fetched data. When users interact with your app, important content will be accessible without needing an internet connection every time. This adaptability ensures your application remains responsive and user-friendly no matter the circumstances.
By fully implementing service workers and caching strategies, as well as optimizing your PWA's metadata, you'll create a compelling and efficient user experience that keeps your users engaged and delighted, regardless of their internet connection. Happy coding!
Key Takeaway #5: Supercharging Next.js Web Performance
When it comes to website performance, speed isn't just a preference—it’s a necessity! In today's fast-paced digital landscape, users expect instantaneous interactions, akin to the sudden appearance of a magician's rabbit. 🐇✨ That's precisely where Next.js web performance truly excels. By implementing features that enhance speed and responsiveness, Next.js empowers developers to create high-performance applications that captivate and retain users.
Fast Facts to Turbocharge Your Application’s Performance
-
Code Splitting: One of the standout features of Next.js is its built-in code splitting. This technique divides your JavaScript code into smaller chunks that are loaded as needed rather than all at once. This not only reduces initial load times but also ensures that users only download the specific code necessary for the page they’re interacting with, leading to quicker render times and a snappier user experience.
-
Image Optimization: Visual content is critical for engaging users, but heavy images can considerably slow down your app. Next.js offers advanced image optimization capabilities. It automatically adjusts image sizes and chooses the most appropriate formats (like WEBP for compatible browsers), allowing for faster loading times while still delivering stunning visuals. This seamless process minimizes bandwidth usage and enhances load speeds, making your app light and efficient.
-
Lazy Loading: Addressing user experience is paramount, which is where lazy loading comes into play. By only loading images and other assets as they enter the viewport, you can significantly boost performance. Imagine it as a cat that waits for a treat to appear—no need for it to be on high alert until the moment it's necessary! This not only conserves resources but creates a smoother browsing experience.
-
Utilizing a CDN: A Content Delivery Network (CDN) is essential for any modern web application. Deploying your Next.js Progressive Web App (PWA) on a CDN allows you to cache content in multiple geographic locations, ensuring that users receive data from the nearest server. As a result, your app runs faster for users, no matter where they access it from, creating a seamless global experience.
Maximizing Your Next.js Lighthouse Scores
Lighthouse is a tool that serves as an authoritative guide to web performance, acting like a wise mentor in your development journey. 🦉 Regular Lighthouse audits can help you gauge the efficiency of your application. Your goal? Achieve scores of 90 or above. This threshold indicates outstanding performance and user experience, ensuring your PWA stands out in the crowded digital marketplace.
Key Takeaway #6: Next.js SEO for PWA
Visibility matters. If your PWA is tucked away in the depths of the internet, it might as well not exist at all. Properly implemented Next.js SEO for PWA is your key to ensuring that search engines can discover and rank your app appropriately.
-
Server-Side Rendering (SSR): Next.js simplifies the process of server-side rendering. This feature allows pre-rendering of pages on the server, making it easier for search engines to crawl and index your content effectively. Properly structured title tags, meta descriptions, and clean URLs become essential ingredients that enhance your site’s discoverability.
-
Structured Data: Implementing structured data is akin to providing search engines with a detailed map of your site’s content. By utilizing JSON-LD format, you can specify distinct pieces of information about your app, improving the chances of rich results in search listings. This detailing enables search engines to understand and categorize your app correctly, enhancing visibility.
-
Performance as a Ranking Factor: Google has made it clear—site speed matters. As you refine your app and achieve better Lighthouse scores, remember that these scores are tied to your search rankings. A fast, well-optimized PWA can propel you to the top of the search engine results pages (SERPs), driving traffic and engagement.
Key Takeaway #7: Mobile Optimization for Next.js
Mobile browsing is the prevailing trend, and as such, an exceptional mobile experience is crucial. With a vast majority of users now accessing content via smartphones, your Next.js mobile optimization strategy needs to be on point.
-
Responsive Design: Ensure that your PWA uses fluid, responsive design principles. From large desktops to small smartphones, your app should adapt seamlessly to any screen size, providing a consistent experience across all devices.
-
Touch-Friendly Elements: Design elements in your app must be easy to interact with on touch screens. Ensure buttons and links are large enough for users to tap comfortably. A frustrated user who struggles to click a small button might abandon your app for a competitor's.
-
Minimize Input Requirements: Streamline user interaction by minimizing typing. Implement features like auto-fill forms, checkboxes, and sliders to ease data input. This approach leads to a more user-friendly experience and encourages higher engagement rates.
Remember, turning overwhelming tasks into creative challenges can often lead to surprising results—like discovering the joy of pumpkin decorating during Halloween! 🎃
Calling All Aspiring Developers—Time to Craft Your PWA!
Congratulations, aspiring web developers! 🎉 You’ve ventured into the exciting universe of building a PWA with Next.js. You’ve gained invaluable knowledge about service workers, offline capabilities, caching strategies, mobile optimization, and the significance of SEO in driving traffic.
Final Words: Embrace the Magic!
Don’t let technical complexities deter you from exploring your full potential. Envision your PWA transforming into a high-speed, responsively designed, SEO-friendly powerhouse that dazzles users.
As you embark on this creative journey, remember to seek support when needed. Engage with community forums, inquire about best practices, and continue expanding your knowledge! If you find yourself in need of professional guidance, Prateeksha Web Design is ready to assist you. Based in Mumbai, we specialize in ecommerce website development and design, dedicated to transforming your visions into reality.
So gather your development tools and start coding! Our web design company in Mumbai is eager to help you manifest your PWA dreams. We believe in your talent—now go out there and craft something magical! ✨🚀
Call to Action
Are you ready to take the plunge and enhance your web development skills? Join us at Prateeksha Web Design as we support you in building innovative and outstanding PWAs with Next.js! Together, let's create digital experiences that not only perform but enchant users around the globe. Reach out today to begin your transformative journey in web development!
Tip
Utilize Next.js Incremental Static Regeneration (ISR): To enhance your PWA’s performance, implement ISR to regenerate static pages on demand. This feature allows your application to serve fresh content without compromising the speed of static generation, ensuring users receive the latest data quickly.
Fact
Core Web Vitals Matter: Google’s Core Web Vitals—specifically, Largest Contentful Paint (LCP), First Input Delay (FID), and Cumulative Layout Shift (CLS)—are critical metrics for measuring user experience in mobile PWAs. Optimizing for these metrics can significantly improve your site's SEO and user engagement.
Warning
Be Cautious with Service Worker Caching: While service workers boost performance by caching resources, improper configuration can lead to stale or outdated content being served to users. Always test your caching strategies and implement versioning to ensure users receive the most relevant and updated content inside your PWA.
About Prateeksha Web Design
Prateeksha Web Design specializes in crafting Progressive Web Applications (PWAs) using Next.js, ensuring a seamless and ultra-fast mobile experience. Their services include intuitive UI/UX design, efficient code optimization, and robust service worker integration to enhance performance. They provide tailored solutions that leverage Next.js’s features for smooth navigation and offline capabilities. Prateeksha prioritizes responsiveness and speed, making mobile interactions lightning-fast. With expert guidance, clients can effectively transition to PWAs for improved user engagement and retention.
Interested in learning more? Contact us today.
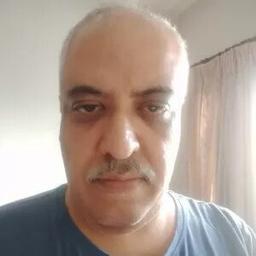