Mastering Mobile-First Web Design with Next.js: A Geek’s Guide
Welcome, budding web wizards! Today, we’re diving into the digital cauldron to brew up some magic with Next.js and explore the fascinating realm of mobile-first web design. If you're looking to develop a sleek, performance-oriented mobile website, you’re in for a treat. Our journey will uncover the secrets of crafting fast mobile websites while harnessing the Next.js mobile optimization sorcery. Let’s get started!
Why Mobile-First?
Before we leap into the nitty-gritty, let’s demystify why a mobile-first approach is the holy grail of web design today. Picture this: You're out and about, your stomach rumbling like a lion, ready to order some food. You whip out your phone and start scrolling for options. If the website looks like it was designed in the Stone Age, are you going to order from there? Nope! You’ll quickly swipe right to that shiny competitor with the responsive web design that fits your screen like that t-shirt you love.
The Rise of Mobile Usage
Statistics reveal that more than half of all web traffic now comes from mobile devices, highlighting the necessity of a mobile-first mindset. A mobile-first approach isn’t just a design trend; it’s a crucial strategy to enhance user engagement and retention. It prioritizes the mobile experience, ensuring that users can easily navigate and interact with your website, regardless of their device.
This is why a mobile-first strategy is essential. It ensures your website provides an exceptional user experience (UX) across all devices, but especially on mobile. With Next.js, you're not just keeping up with trends; you're setting the pace for a seamless experience!
Getting Started with Next.js
What is Next.js?
At its core, Next.js is a powerful React framework that simplifies the process of building server-rendered and static web applications. It offers an extensive feature set that makes it a prime choice for implementing a mobile-first design:
- Static Site Generation (SSG) allows for super-fast load times—a critical factor for Next.js performance for mobile, as users on a mobile network often experience slower speeds.
- API routes offer an effortless way to build APIs directly in your application, providing flexibility in data handling.
- Out-of-the-box routing systems that enable intuitive app navigation without congestion in your codebase, making it easy to structure your application.
This versatile framework transforms the often tedious task of site development into a more enjoyable, efficient process!
Getting Your Feet Wet
-
Set Up Your Next.js Environment
First thing's first: let’s set up your workspace. Head over to the Next.js documentation and ensure you have Node.js installed. Once you’re all set, fire up your terminal and run:
npx create-next-app@latest my-awesome-project
Congratulations! You are off to a flying start, and your future website company in Mumbai would be proud of your initiative!
-
Understand the Folder Structure
The pages directory is where the magic happens. Each JavaScript file you create in this folder corresponds to a route in your app. Easy peasy, right? Think of it like having different rooms in your online home—each page takes you to a different area!
Here's a brief overview of important directories you'll encounter:
- pages/: This folder contains your application's routes (e.g., index.js for the homepage).
- public/: For static assets like images and fonts, easily accessible from your app.
- components/: Perfect for organizing reusable UI components, keeping your code DRY.
- styles/: The go-to folder for your CSS files.
-
Leverage Built-in CSS Support
Next.js supports global CSS files and CSS modules right out of the box. You can stylishly dress up your site without breaking a sweat. You can add a global stylesheet in your
pages/_app.js
as follows:import '../styles/globals.css';
Keeping your styles organized will enhance maintainability and help prevent CSS conflicts. Trust us, your users will thank you later!
Crafting Responsive Designs
Let’s chat about responsive web design. You might be wondering, "Isn't it just about making websites look good on mobile?" Well, sort of, but there’s more to it than that.
Use Flexbox and Grid
CSS Flexbox and Grid layouts are fantastic for creating dynamic, flexible layouts. While Flexbox is your go-to for arranging elements in a row or a column, Grid shines when designing entire page layouts.
Here’s a quick analogy—picture Flexbox as arranging chairs in a cozy café (like how you squeeze in those extra seats), while Grid helps you plan the entire café layout, from the entrance to the restrooms. With both in your toolkit, your designs will be buttery smooth and effortlessly responsive.
Breakpoints and Media Queries
When dealing with responsive design, it’s essential to incorporate breakpoints and media queries. Breakpoints mark the points at which your web design will adapt to different screen sizes, ensuring that your content is presented effectively.
@media (max-width: 768px) {
.container {
flex-direction: column;
}
}
In this example, when the viewport width is less than or equal to 768 pixels, the flex container will switch from a horizontal layout to a vertical stack. This enhances accessibility and user experience, especially for mobile users where screen real estate is limited.
Optimizing Images for Mobile
Another critical aspect of mobile-first design is image optimization. Large images can drastically slow down your website performance, particularly on mobile networks. Make use of responsive image techniques such as <img srcSet>
and src
attributes, or leverage libraries like next/image
to automatically optimize your images:
import Image from 'next/image';
<Image
src="/path/to/image.jpg"
alt="Description"
width={500}
height={300}
layout="responsive"
/>
Performance Optimization with Next.js
Now that you’ve designed a visually appealing and responsive layout, it's time to boost performance even further. Next.js comes with built-in features that enhance load times and performance, vital for mobile users.
-
Automatic Code Splitting: This ensures that users only download the necessary code for the page they’re currently visiting, rather than the entire site in one go.
-
Image Optimization: Utilizing the
next/image
component significantly reduces image loading times and automatically serves the best resolution for each device. -
Prefetching: Next.js prefetches linked pages in the background, providing a snappier navigation experience for users.
-
Server-Side Rendering (SSR): You can choose to render your pages on the server, which can speed up initial loading times for complex applications.
-
Static Generation (SSG): For primarily content-driven sites, generating static pages during build time can provide unparalleled performance.
Final Touches
Before you launch your masterfully crafted mobile-first website, conduct thorough testing across various devices and browsers. Tools like Google Lighthouse can help you assess performance, accessibility, and best practices. Don’t forget to optimize for SEO by ensuring proper meta tags, descriptions, and alt tags for images.
Conclusion: Embrace the Mobile-First Revolution
In summary, embracing a mobile-first design approach using Next.js empowers you to create dynamic, user-friendly websites that outperform competitors. By prioritizing mobile users’ experience, leveraging Next.js features, and utilizing modern CSS techniques, you're not only enhancing user engagement but also ensuring your website stands the test of time.
Now go forth, beloved code sorcerers! With your newfound powers, enchant the web with stunning, swift, and intuitive mobile experiences that leave users coming back for more. Happy coding!
To effectively embrace a mobile-first design philosophy, one of the most powerful tools at your disposal is the implementation of media queries. Media queries allow you to create responsive styles based on the characteristics of the device displaying your site, particularly the width of the screen. This means you can dictate how your website appears on different screens, ensuring an optimal user experience across all devices. For example, you can instruct your website: "If this screen width is less than 768 pixels, please apply these specific styles!"
Here's a quick demonstration using CSS:
@media (max-width: 768px) {
body {
background-color: lightblue;
}
}
In this snippet, when a user's screen is 768 pixels wide or narrower, the background color of the website will change to light blue. This isn't just about aesthetics; it's about being user-centric and responsive to the technology your users are utilizing. This simple implementation showcases your consideration for users and enhances usability on mobile devices—kudos to you for that!
Optimize Images for Mobile
Now, let’s talk about a common pain point for mobile users: slow-loading images. Large images can significantly slow down load times, especially on mobile networks. By optimizing images, you not only increase the loading speed of your pages but also enhance the overall user experience.
Next.js provides a robust solution with its Image Component, which automatically optimizes images for different devices and screen sizes. Here’s how you can use it:
import Image from 'next/image';
export default function MyProfile() {
return (
<Image
src="/my-image.jpg"
alt="My Profile Picture"
width={500}
height={500}
/>
);
}
With this implementation, your images will load quickly and efficiently on mobile devices, providing a seamless browsing experience while conserving users' precious data. It's a win-win!
Next.js Mobile Optimization Techniques
Now that we’ve covered some key basics, let’s delve into some advanced Next.js mobile optimization techniques. Mastering these will ensure that your website performs smoothly, even under the constraints of mobile networks.
Prefetch Links
One powerful feature of Next.js is its ability to automatically prefetch links. This means as users hover over a link, their browser begins loading that page in the background. This preemptive measure significantly improves the transition speed between pages—no more impatient waiting! Here's how you can implement prefetching for your links:
<Link href="/about" prefetch>
<a>About Us</a>
</Link>
By using prefetching, you can enhance navigational speed and create a more fluid experience for your users. Think of it as a friendly gesture that anticipates user actions.
Page Segmentation
When creating mobile content, consider breaking down your information into smaller, digestible segments. Long pages can lead to scrolling fatigue, making it essential to structure your content in a way that maintains user interest without overwhelming them. This approach is similar to how episodic content on streaming platforms engages viewers—each episode maintains interest and eager anticipation for more.
Minimize the JavaScript Bundle Size
Embarking on a journey to minimize your JavaScript bundle size is not only a good practice but can also have a dramatic impact on performance. Techniques like tree-shaking—which removes unused code from your bundles—are automatically handled by Next.js. However, it’s beneficial to regularly audit your dependencies and assess whether there are any unnecessary libraries or components.
You can analyze your bundle sizes and dependencies using tools like:
- Webpack Bundle Analyzer – This tool gives a visual representation of your bundle size, helping you identify which modules are taking up the most space.
- Next.js built-in analysis – You can enable
next build --analyze
to get insights directly in your terminal.
By employing these strategies and features, you will not only enhance the performance of your mobile site on Next.js but also create a more engaging and efficient user experience.
Overall, embedding mobile-first design principles into your web development process ensures that you’re catering to the growing number of mobile users and optimizing their experience effectively.
Certainly! Let’s expand, detail, and refine your content to provide an enriched understanding of optimizing a Next.js site with emphasis on speed, user experience, and best practices.
npm run analyze
When you run the above command in your Next.js application, it triggers a powerful analysis tool that provides insights into your application's performance, bundling, and potential areas for optimization. By leveraging this analysis, you can ensure your website operates efficiently, aiming to be the lean, mean machine you've envisioned.
Optimize Your Next.js Application with Caching and CDN
One of the key advantages of using Next.js is its seamless compatibility with Content Delivery Networks (CDNs). CDNs expedite file delivery to users by caching static assets across various global locations, effectively minimizing latency and enhancing load times.
Implementing Caching Strategy
To effectively leverage caching, ensure that you store static files like images, stylesheets, and scripts in the CDN. This enables your repeat visitors to benefit from drastically improved loading times, which translates to increased user satisfaction and engagement.
Here's an example of how to fetch data in a server-side environment while benefiting from caching:
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/data');
if (!res.ok) {
return {
notFound: true,
}
}
const data = await res.json();
return {
props: { data },
}
}
By implementing caching strategies and optimizing server-side fetching, you not only enhance performance but also elevate the user experience. Remember, every second saved is an invitation for potential users to engage with your content!
Elevating User Experience through Best Practices in Next.js
As you embark on building an engaging web ecosystem, it's crucial to adopt methodologies that prioritize user experience (UX). Here are essential Next.js UX practices to consider:
Usability Testing
Conducting usability tests with real users is pivotal. Engage with your audience to uncover what resonates with them. By observing their interactions with your design, you can identify pain points and refine those areas until your interface delights users.
Accessibility Matters
Creating an accessible website should be a priority for any web developer. Start with semantic HTML, which not only aids in SEO but also helps screen readers interpret content more effectively. Providing comprehensive alt text
for images and ensuring proper color contrast not only broadens your audience demographic but fulfills ethical standards that enhance overall satisfaction.
Continuous Performance Monitoring
Regularly monitor the performance of your website using tools such as Google Lighthouse and WebPageTest. These tools act like fitness trackers for your website, allowing you to benchmark progress and identify areas needing improvement. Implement periodic evaluations to ensure your site consistently meets performance goals, retaining users in the fast-paced digital landscape.
Frequently Asked Questions
What Makes Next.js Ideal for Mobile Development?
Next.js shines in mobile development thanks to its static rendering capabilities and extensive built-in optimizations. By serving pre-rendered pages, Next.js ensures that mobile users receive fast, lightweight, and responsive experiences, resulting in a smooth interaction no matter the device.
Is Next.js Suitable for E-commerce Websites?
Absolutely! With features such as easy API integrations, dynamic routes, and a performance-focused architecture, Next.js is highly advantageous for e-commerce platforms. If you're curating an e-commerce website in Mumbai, Next.js serves as a robust foundation, ensuring fast load times and seamless user experiences.
How Can I Discover a Top-notch Web Design Agency in Mumbai?
For those in search of excellence in web design, Prateeksha Web Design is a noteworthy choice. Renowned for their expertise in e-commerce website development and innovative design approaches, they are well-equipped to transform your vision into a sophisticated digital reality.
Conclusion
Congratulations! You’ve successfully navigated through the world of Next.js and modern mobile-first web design principles. Equipped with insights into responsive design, Next.js performance optimization, and valuable UX practices, you are empowered to thrive in the mobile market.
The web design landscape is constantly evolving, driven by user preferences and technological advancements. Stay informed, remain curious, and embrace experimentation to remain ahead of the curve. If you’re ready to take your web projects to the next level, consider partnering with Prateeksha Web Design, a leading web development company in Mumbai. With their guidance, you can craft a website that is functional, aesthetically pleasing, and delivers a delightful experience for every mobile user.
This refined content emphasizes detailed understanding while maintaining clarity and engagement for readers interested in Next.js and web design.
Tip:
Utilize Responsive Design Principles: When using Next.js, leverage CSS frameworks like Tailwind CSS or Bootstrap for responsive utility classes. Prioritize a mobile-first approach by designing your layouts and components for smaller screens before scaling up to larger devices. This effectively helps in creating a seamless user experience across various screen sizes.
Fact:
SEO Benefits of Server-Side Rendering: One of the strengths of Next.js is its capability for server-side rendering (SSR). This feature not only improves the loading speed of your mobile-first web applications but also enhances search engine optimization (SEO), as search engines can easily crawl and index the content of your site, significantly improving visibility on mobile searches.
Warning:
Be Cautious with Excessive Dependencies: While Next.js offers many powerful features, avoiding excessive reliance on third-party libraries is crucial. Too many dependencies can bloat your mobile application's bundle size, slowing down performance and negatively impacting the user experience on mobile devices. Always review and optimize your dependencies to maintain efficiency.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services for mastering mobile-first web design using Next.js, focusing on responsive layouts and optimal performance. Their approach emphasizes user experience across devices, ensuring fast loading times and intuitive navigation. The team integrates modern design principles with advanced features of Next.js to create visually appealing and functional websites. They provide tailored solutions for businesses looking to enhance their online presence in a mobile-centric world. Continuous support and updates ensure that clients stay ahead in the ever-evolving digital landscape.
Interested in learning more? Contact us today.
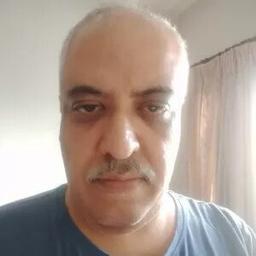